How to Get Companies with the PipelineCRM API in Python
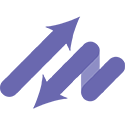
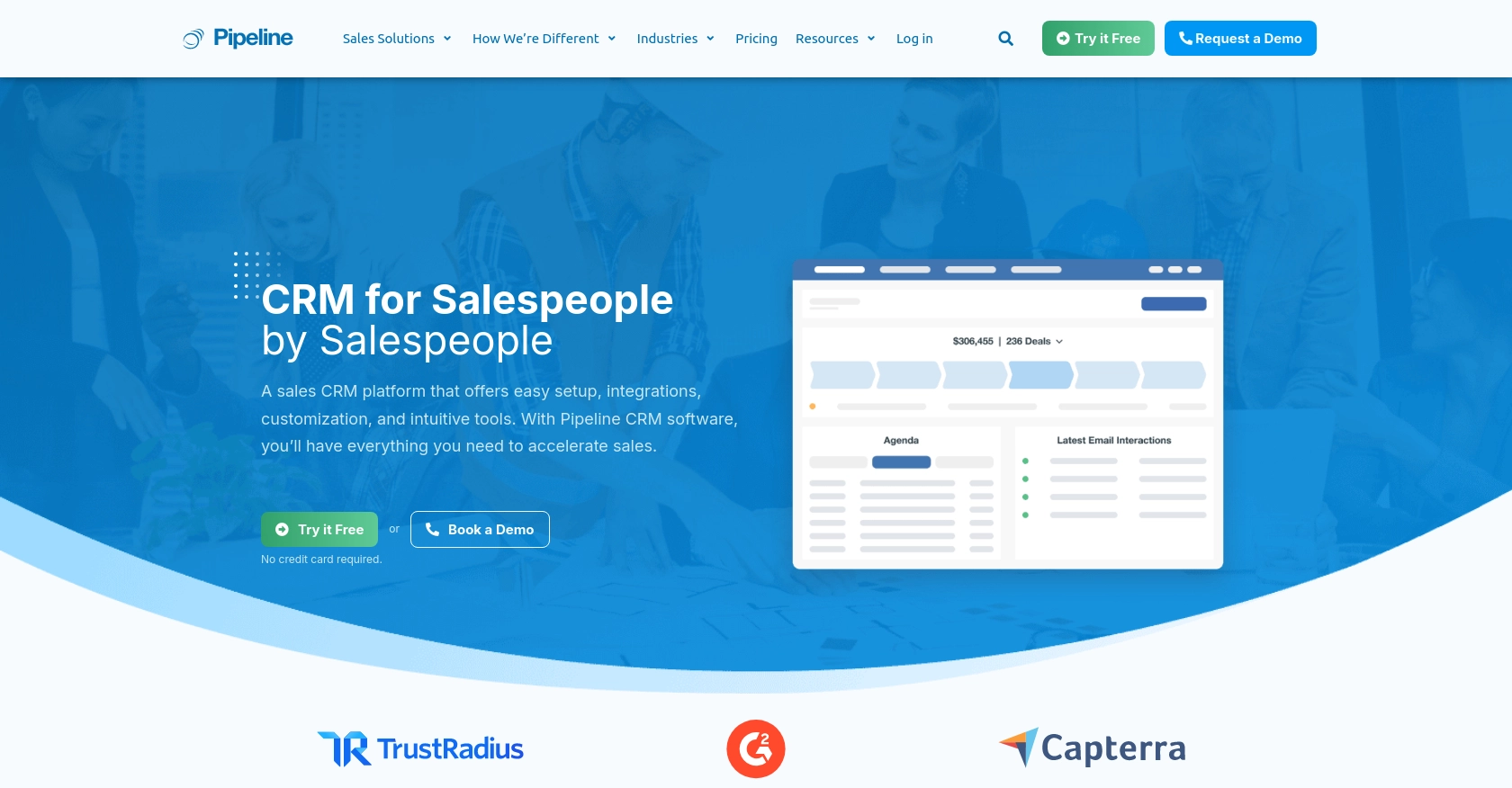
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. With features such as contact management, sales automation, and reporting, PipelineCRM is a valuable tool for businesses looking to streamline their sales operations.
Developers may want to integrate with PipelineCRM's API to access and manage company data, enabling seamless synchronization with other business applications. For example, a developer might use the PipelineCRM API to retrieve a list of companies and integrate this data into a custom reporting tool, enhancing the sales team's ability to track and analyze client interactions.
Setting Up Your PipelineCRM Test Account
Before you can start integrating with the PipelineCRM API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial or use the free version available on the PipelineCRM website. Follow the on-screen instructions to create your account and log in.
Generating Your API Key for PipelineCRM
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account.
- Navigate to the Settings section, usually found in the top navigation bar.
- Under the API Access section, find the option to generate a new API key.
- Click on Generate API Key and copy the key provided. Make sure to store it securely, as you will need it to authenticate your API requests.
Configuring Your Development Environment
With your API key ready, you can now configure your development environment to interact with the PipelineCRM API. Ensure you have Python installed on your machine, along with the necessary libraries for making HTTP requests.
pip install requests
This command will install the requests
library, which you'll use to make API calls in Python.
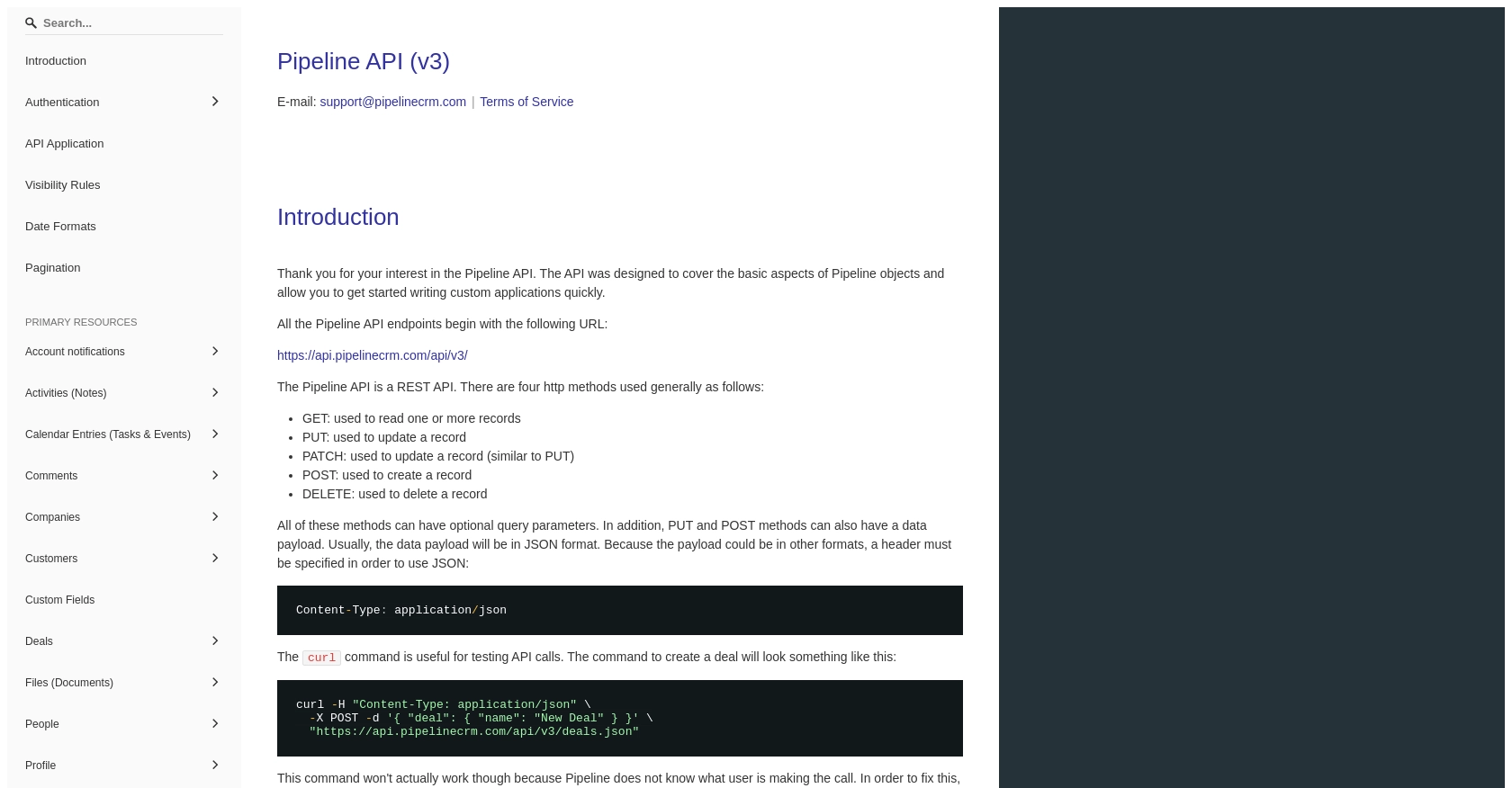
sbb-itb-96038d7
Making API Calls to Retrieve Companies with PipelineCRM in Python
Understanding Python and Required Libraries for PipelineCRM API Integration
To interact with the PipelineCRM API using Python, ensure you have Python 3.x installed on your system. You will also need the requests
library to handle HTTP requests. If you haven't installed it yet, you can do so using the following command:
pip install requests
This library simplifies the process of making API calls and handling responses.
Writing Python Code to Fetch Companies from PipelineCRM
Once your environment is set up, you can proceed to write the Python script to retrieve company data from PipelineCRM. Create a new Python file named get_pipelinecrm_companies.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://api.pipelinecrm.com/v3/companies"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_API_Key"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
companies = response.json()
for company in companies:
print(f"Company Name: {company['name']}")
else:
print(f"Failed to retrieve companies. Status Code: {response.status_code}")
Replace Your_API_Key
with the API key you generated from your PipelineCRM account. This script sends a GET request to the PipelineCRM API to fetch a list of companies. If the request is successful, it prints the name of each company.
Running the Python Script and Verifying the Output
Execute the script from your terminal or command line using the following command:
python get_pipelinecrm_companies.py
You should see a list of company names printed in your terminal, confirming that the API call was successful. If there are any issues, the script will output the status code, which can help in troubleshooting.
Handling Errors and Understanding PipelineCRM API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The PipelineCRM API may return various status codes indicating the result of your request:
- 200 OK: The request was successful, and the response contains the requested data.
- 401 Unauthorized: The API key is missing or invalid. Ensure your API key is correct.
- 404 Not Found: The requested resource could not be found. Verify the endpoint URL.
- 500 Internal Server Error: An error occurred on the server. Try again later or contact support.
By checking the status code, you can implement logic to handle these scenarios and provide meaningful feedback to users.
Conclusion and Best Practices for Integrating with PipelineCRM API
Integrating with the PipelineCRM API using Python allows developers to efficiently manage and synchronize company data across various business applications. By following the steps outlined in this guide, you can seamlessly retrieve company information and enhance your sales processes.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the PipelineCRM API. Implement logic to handle rate limit responses and retry requests after the appropriate wait time.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized to match your application's data structures for consistency and ease of use.
Streamline Your Integrations with Endgrate
While integrating with individual APIs like PipelineCRM can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including PipelineCRM. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?