Using the Monday.com API to Get Users in Python
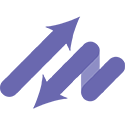
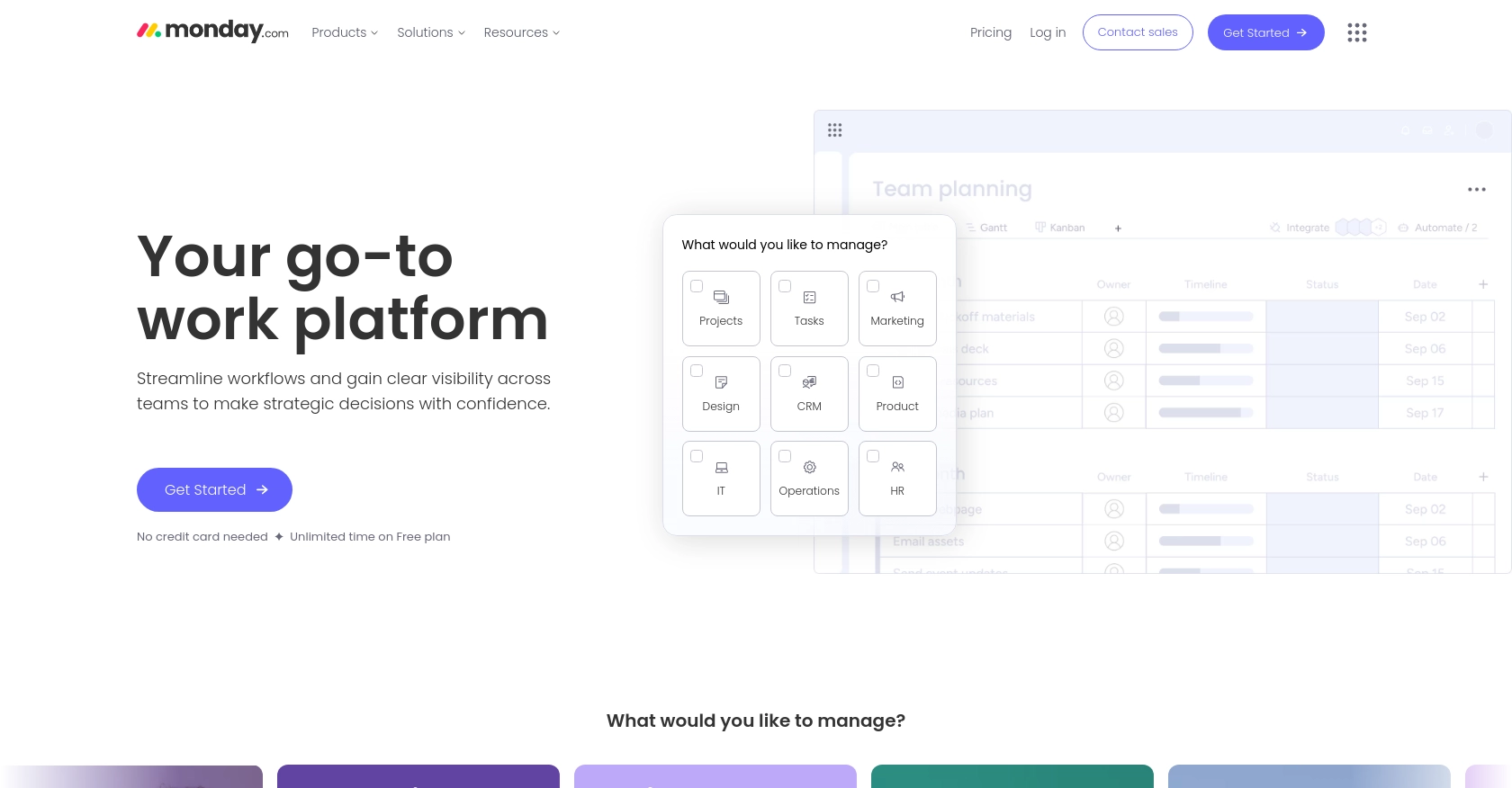
Introduction to Monday.com API Integration
Monday.com is a versatile work operating system that empowers teams to run projects and workflows with confidence. Its intuitive interface and robust features make it a popular choice for businesses seeking to enhance collaboration and productivity.
Developers may want to integrate with Monday.com's API to access and manage user data, enabling seamless synchronization with other applications. For example, retrieving user information using the Monday.com API in Python can help automate user management tasks, such as updating user roles or syncing user data across platforms.
Setting Up Your Monday.com API Test Account
Before diving into the integration with Monday.com, you'll need to set up a test account to experiment with the API. This will allow you to safely test your API calls without affecting live data.
Create a Monday.com Account
- Visit the Monday.com website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the Monday.com dashboard.
Generate Your Monday.com API Token
To interact with the Monday.com API, you'll need an API token for authentication. Here's how to obtain it:
- Log into your Monday.com account.
- Click on your profile picture in the top right corner and select Administration if you're an admin, or Developers if you're a member user.
- Navigate to the API section under Connections or My Access Tokens.
- Copy your personal API token. Remember, you can regenerate a new token if needed, but this will invalidate the previous one.
For more details, refer to the Monday.com authentication documentation.
Understanding OAuth Authentication
Monday.com uses OAuth for secure API access. Ensure your application is set up to handle OAuth tokens if you're planning to deploy beyond personal use. This involves creating an app in the Monday.com Developer Center and managing client credentials.
With your test account and API token ready, you're all set to start making API calls to Monday.com. In the next section, we'll explore how to perform these calls using Python.
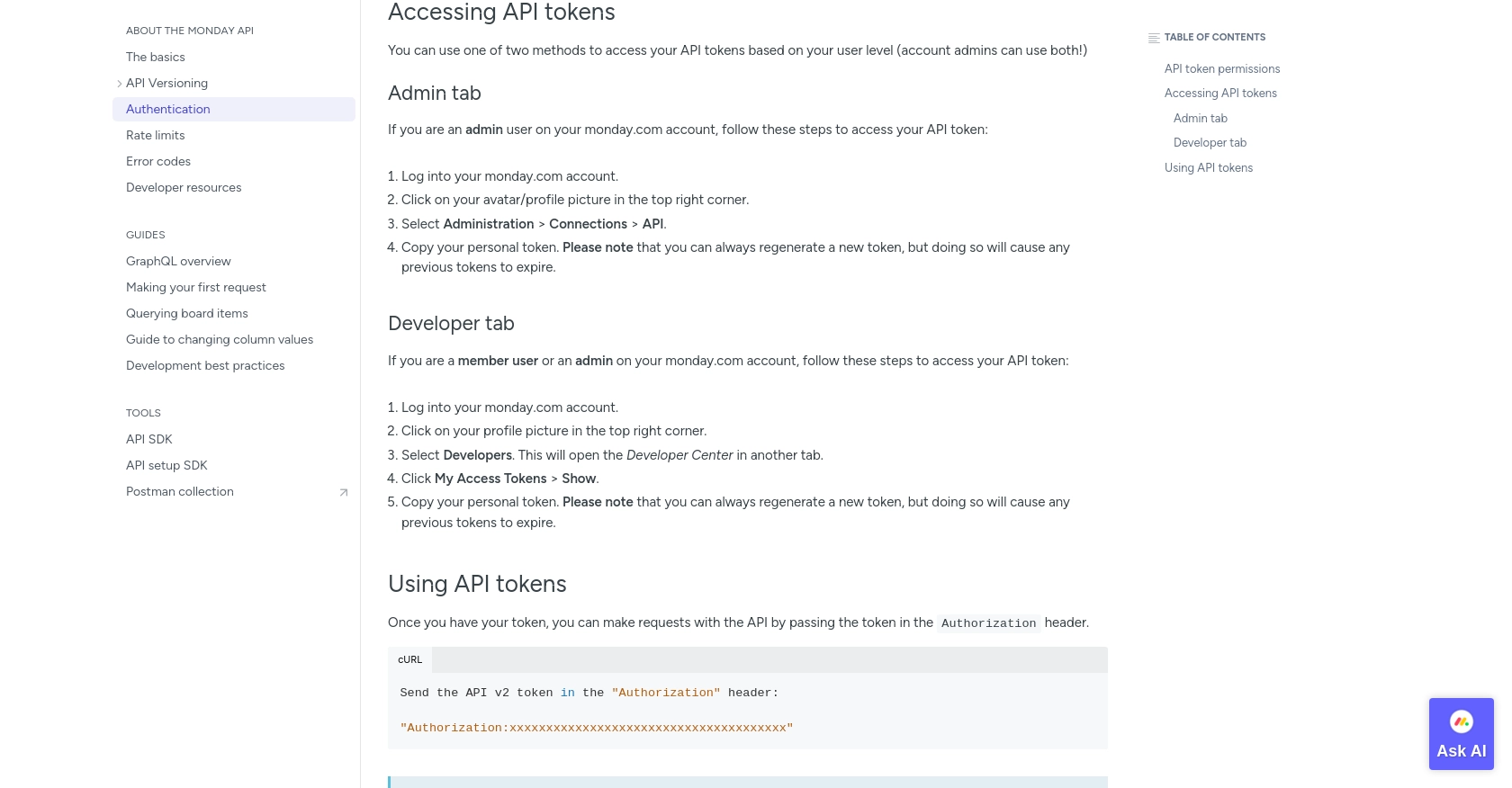
sbb-itb-96038d7
Making API Calls to Retrieve Users from Monday.com Using Python
To interact with the Monday.com API and retrieve user data, you'll need to use Python. This section will guide you through setting up your environment, making the API call, and handling the response.
Setting Up Your Python Environment for Monday.com API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Install the
requests
library using pip:
pip install requests
Writing the Python Script to Call the Monday.com API
Now that your environment is ready, you can write a Python script to retrieve users from Monday.com. Create a file named get_monday_users.py
and add the following code:
import requests
# Set the API endpoint and headers
url = "https://api.monday.com/v2"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_API_TOKEN"
}
# Define the GraphQL query
query = """
{
users (limit: 50) {
id
name
email
created_at
}
}
"""
# Make the POST request to the API
response = requests.post(url, headers=headers, json={"query": query})
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for user in data["data"]["users"]:
print(f"ID: {user['id']}, Name: {user['name']}, Email: {user['email']}")
else:
print(f"Failed to retrieve users: {response.status_code}")
Replace YOUR_API_TOKEN
with the API token you obtained earlier. This script sends a GraphQL query to the Monday.com API to fetch user details such as ID, name, and email.
Running the Script and Verifying the Output
Execute the script from your terminal or command line:
python get_monday_users.py
If successful, the script will output a list of users with their IDs, names, and emails. Verify the data by cross-referencing with your Monday.com test account.
Handling Errors and Understanding Monday.com API Error Codes
It's crucial to handle potential errors when making API calls. The Monday.com API may return various error codes, such as:
- 401 Unauthorized: Ensure your API token is valid and included in the request headers.
- 429 Rate Limit Exceeded: Reduce the number of requests per minute. The limit is 5,000 requests per minute. Refer to the rate limits documentation for more details.
- 500 Internal Server Error: Check your query for any invalid arguments or malformed JSON.
For a comprehensive list of error codes, visit the Monday.com error codes documentation.
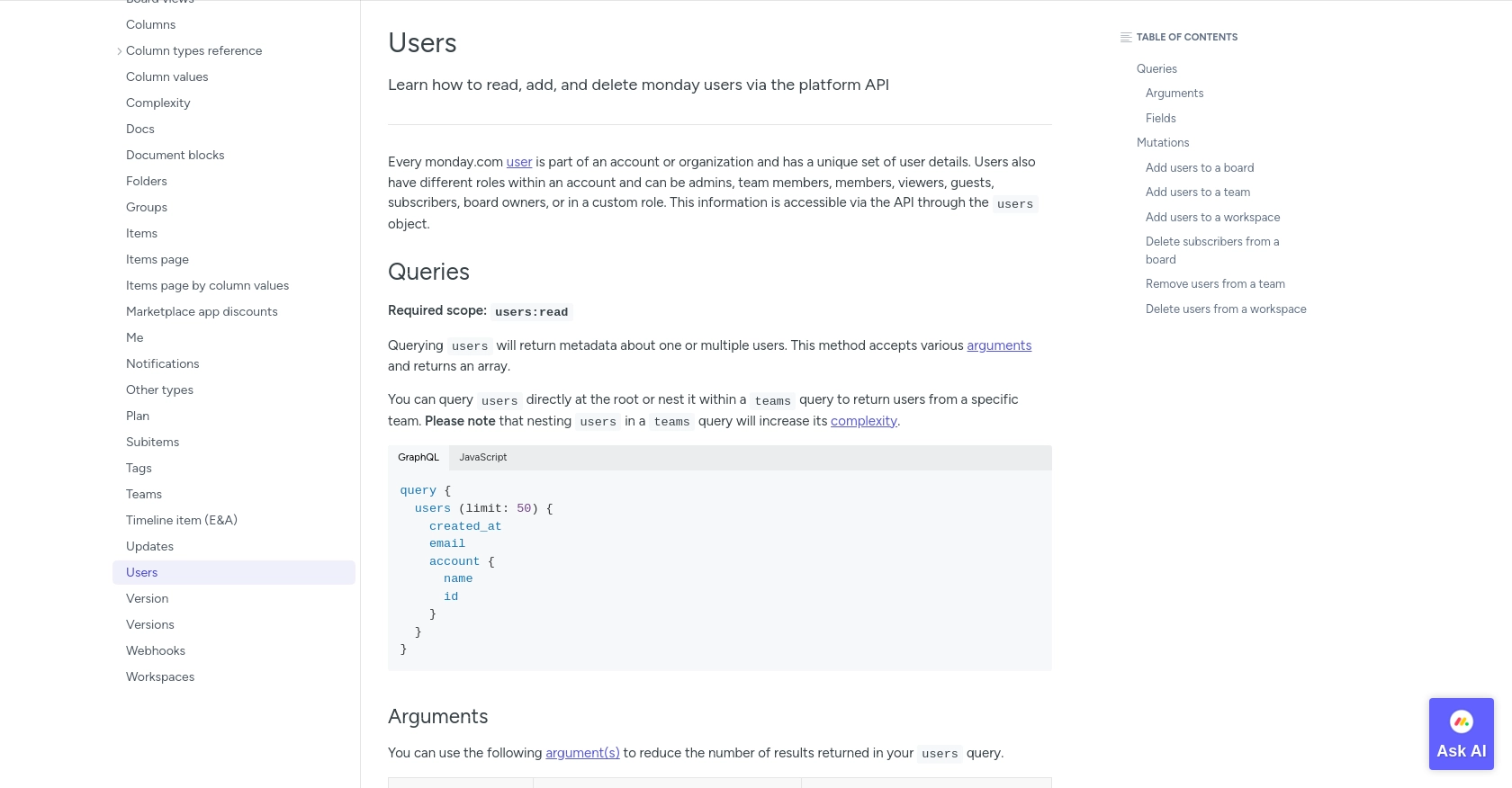
Conclusion and Best Practices for Monday.com API Integration
Integrating with the Monday.com API using Python allows developers to efficiently manage user data and automate workflows. By following the steps outlined in this guide, you can seamlessly retrieve user information and enhance your application's functionality.
Best Practices for Secure and Efficient Monday.com API Usage
- Securely Store API Tokens: Always store your API tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of the rate limits set by Monday.com, which allow up to 5,000 requests per minute. Implement retry mechanisms and optimize your queries to avoid exceeding these limits. For more details, refer to the rate limits documentation.
- Optimize Query Complexity: Use pagination and limit the fields you request to reduce query complexity and improve performance.
- Error Handling: Implement robust error handling to manage potential issues such as unauthorized access or malformed requests. Consult the error codes documentation for guidance.
Enhance Your Integration Strategy with Endgrate
While integrating with Monday.com is a powerful way to enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Monday.com.
With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?