Using the Pipedrive API to Create or Update Organizations (with Javascript examples)
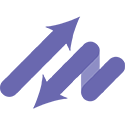
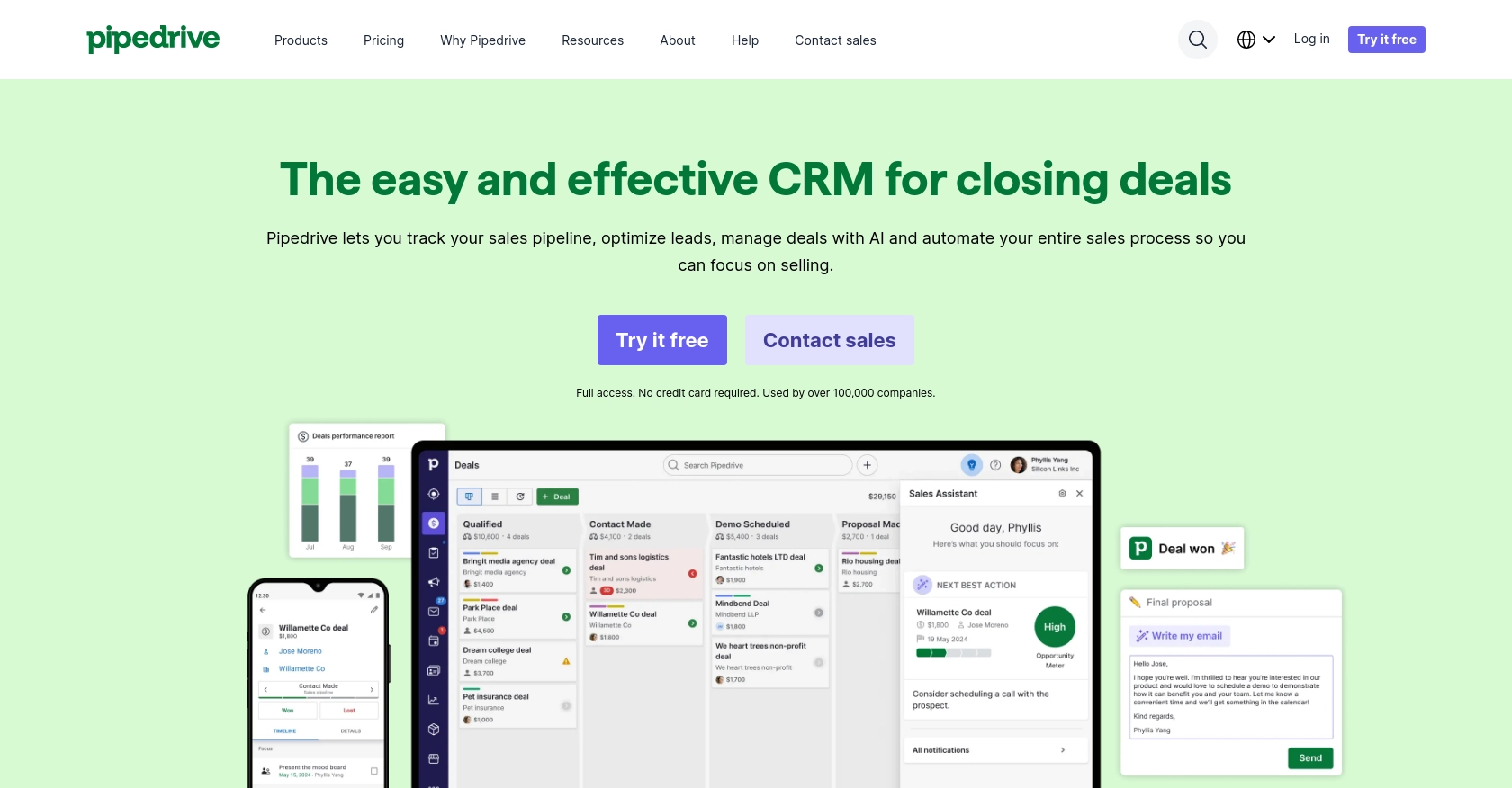
Introduction to Pipedrive CRM and API Integration
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. Known for its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage deals, and automate workflows, making it a popular choice for businesses aiming to enhance their sales efficiency.
Integrating with Pipedrive's API allows developers to automate and streamline various sales operations, such as managing organizations within the CRM. For example, a developer might use the Pipedrive API to create or update organization records automatically, ensuring that the sales team always has access to the most current information without manual data entry.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you'll need to set up a developer sandbox account. This account provides a safe environment for testing and development, ensuring that your integration works seamlessly without affecting live data.
Steps to Create a Pipedrive Developer Sandbox Account
- Sign Up for a Sandbox Account: Visit the Pipedrive Developer Sandbox page and fill out the form to request access to a sandbox account. This account mimics a regular Pipedrive company account and is limited to five seats.
- Access Developer Hub: Once your sandbox account is set up, you can access the Developer Hub. This is where you can manage your apps and obtain the necessary credentials for OAuth 2.0 authentication.
- Import Sample Data: To familiarize yourself with Pipedrive's features, you can import sample data. Navigate to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app to upload template spreadsheets available in English or Portuguese.
Creating an App for OAuth Authentication
To interact with the Pipedrive API, you'll need to create an app that uses OAuth 2.0 for authentication. Follow these steps to set up your app:
- Register Your App: In the Developer Hub, register your app to obtain the client ID and client secret. These credentials are essential for implementing the OAuth flow.
- Define Scopes and Permissions: Choose the necessary scopes for your app. Ensure that you request only the permissions required for your specific use case to streamline the user experience.
- Implement OAuth Flow: Add server-side code to handle the OAuth flow, allowing users to authorize your app to access their Pipedrive data securely.
For more detailed information on creating an app and managing OAuth authentication, refer to the Pipedrive App Creation Guide.
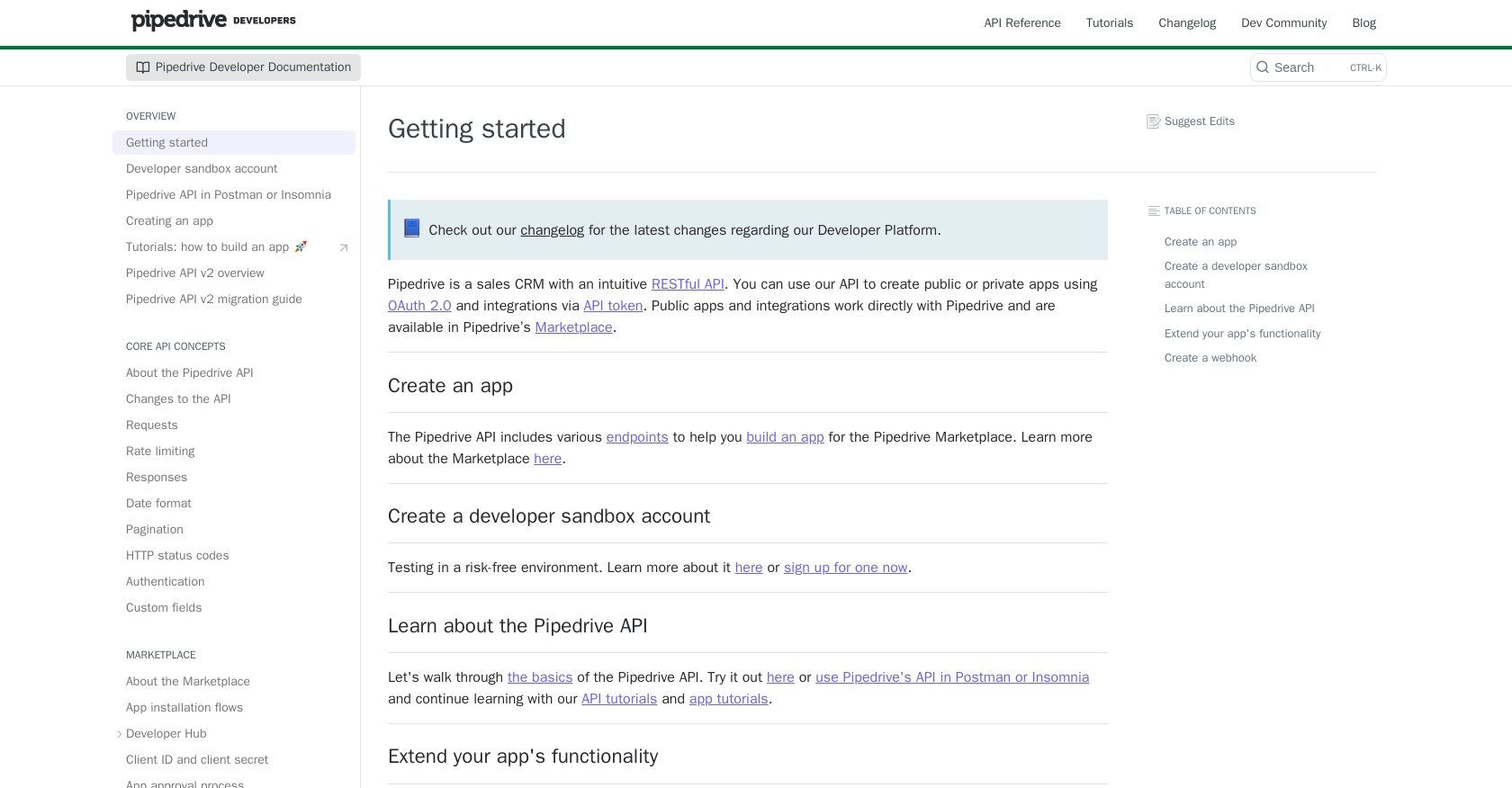
sbb-itb-96038d7
Making API Calls to Pipedrive for Creating or Updating Organizations Using JavaScript
To interact with the Pipedrive API for creating or updating organizations, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Pipedrive API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside a browser. Additionally, you'll need the axios
library to handle HTTP requests.
- Install Node.js: Download and install Node.js from the official website.
-
Install Axios: Open your terminal and run the following command to install Axios:
npm install axios
Writing JavaScript Code to Create or Update Organizations in Pipedrive
With your environment set up, you can now write JavaScript code to interact with the Pipedrive API. Below is an example of how to create or update an organization using Axios.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.pipedrive.com/v1/organizations';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
};
// Function to create or update an organization
async function createOrUpdateOrganization(orgData) {
try {
const response = await axios.post(endpoint, orgData, { headers });
console.log('Organization created/updated successfully:', response.data);
} catch (error) {
if (error.response) {
console.error('Error:', error.response.data);
} else {
console.error('Error:', error.message);
}
}
}
// Example organization data
const organizationData = {
name: 'New Organization',
owner_id: 123456
};
// Call the function
createOrUpdateOrganization(organizationData);
Replace YOUR_ACCESS_TOKEN
with the token obtained during the OAuth authentication process. The organizationData
object contains the details of the organization you wish to create or update.
Verifying API Call Success in Pipedrive Sandbox
After executing the code, verify the success of your API call by checking the Pipedrive sandbox account. Navigate to the Organizations section to see if the new or updated organization appears as expected.
Handling Errors and Understanding Pipedrive API Response Codes
When making API calls, it's crucial to handle potential errors. The Pipedrive API uses standard HTTP status codes to indicate the success or failure of a request:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 429 Too Many Requests: Rate limit exceeded. Consider implementing rate limiting strategies.
For more detailed information on error codes, refer to the Pipedrive HTTP Status Codes Documentation.
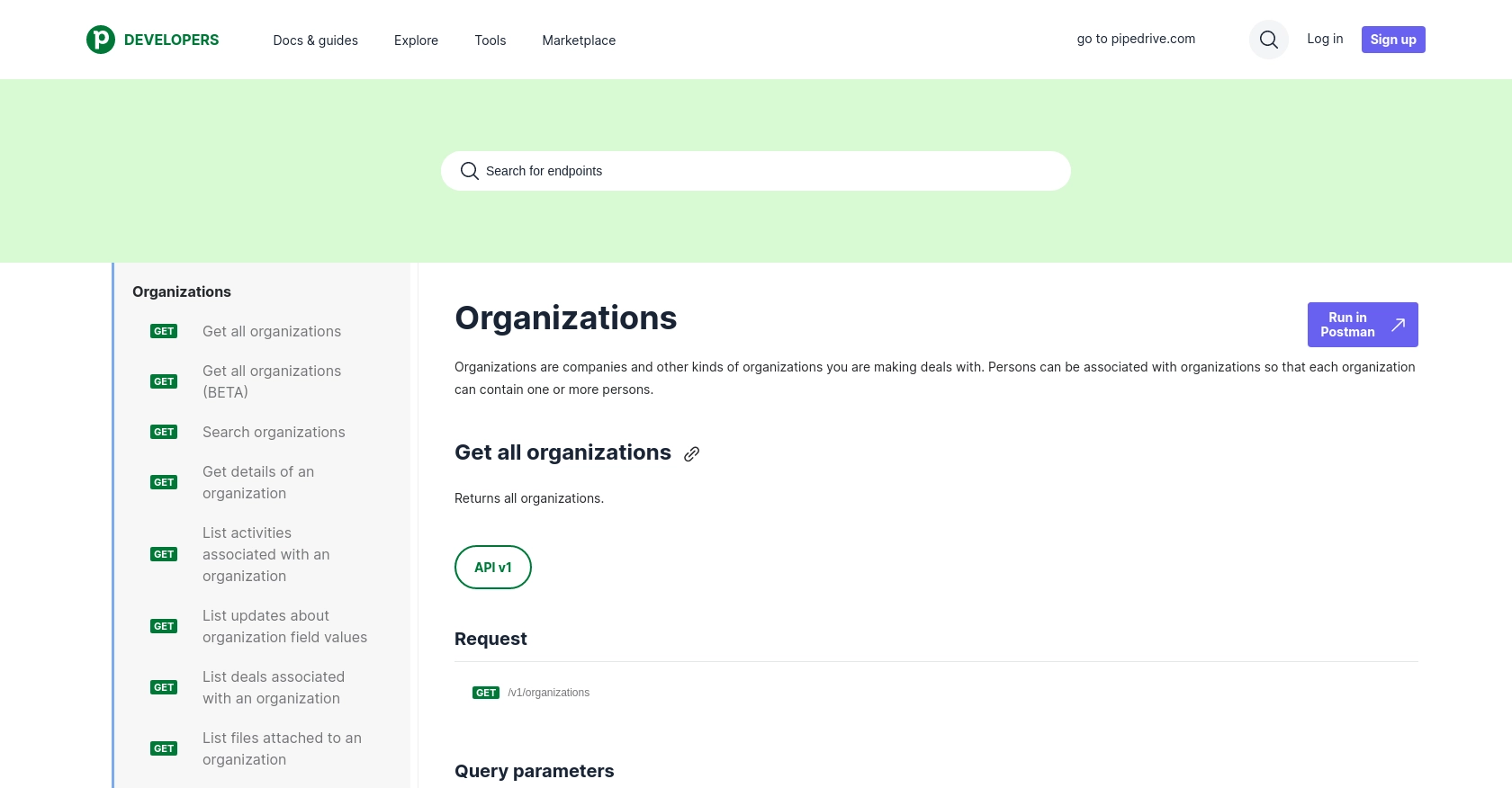
Conclusion and Best Practices for Pipedrive API Integration
Integrating with the Pipedrive API to create or update organizations using JavaScript can significantly enhance your sales operations by automating data management and ensuring accuracy. By following the steps outlined in this guide, you can efficiently set up your development environment, authenticate using OAuth, and make API calls to manage organizations within Pipedrive.
Best Practices for Secure and Efficient Pipedrive API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Implement Rate Limiting: Be mindful of Pipedrive's rate limits, which allow up to 80 requests per 2 seconds per access token for the Essential plan. Implement strategies to handle rate limiting, such as exponential backoff or queuing requests.
- Standardize Data Fields: Ensure that data fields are standardized across your applications to maintain consistency and avoid errors when integrating with Pipedrive.
Enhance Your Integration Strategy with Endgrate
While integrating with Pipedrive directly can be powerful, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including Pipedrive.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a seamless integration experience for your users. Explore how Endgrate can streamline your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Organizations
- https://developers.pipedrive.com/docs/api/v1/OrganizationFields
- https://developers.pipedrive.com/docs/api/v1/OrganizationRelationships
Ready to get started?