How to Get Records with the VTiger API in Javascript
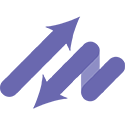
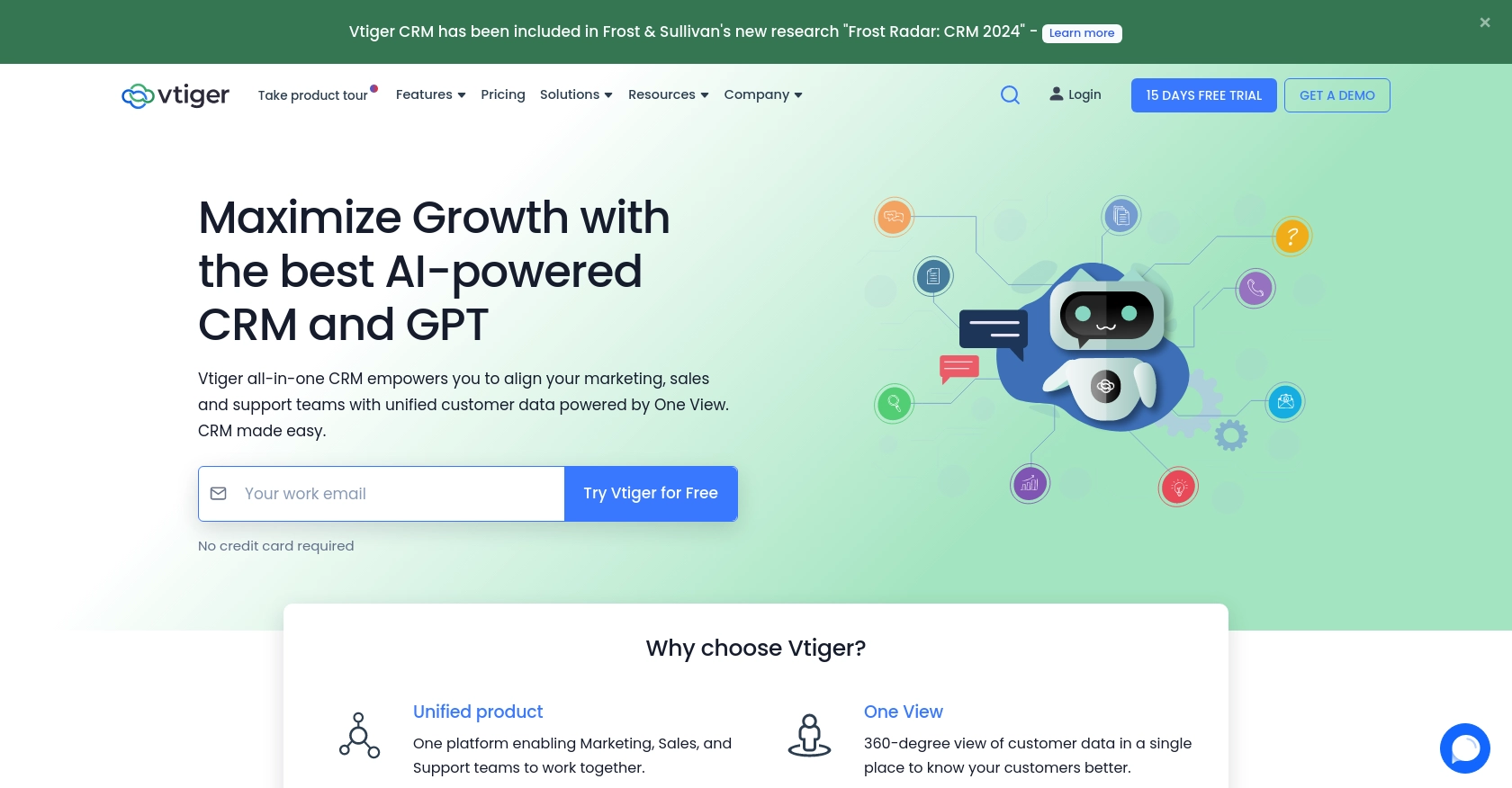
Introduction to VTiger CRM API Integration
VTiger CRM is a versatile customer relationship management platform that offers a wide range of tools to help businesses manage their sales, marketing, and support operations. With its robust features and user-friendly interface, VTiger is a popular choice for organizations looking to streamline their customer interactions and improve overall efficiency.
Developers may want to integrate with VTiger's API to automate data retrieval and management tasks. For example, you can use the VTiger API to fetch records from the CRM, allowing you to synchronize customer data with other applications or generate reports for business analysis.
This article will guide you through the process of using JavaScript to interact with the VTiger API, enabling you to efficiently retrieve records and integrate them into your applications.
Setting Up Your VTiger CRM Test Account for API Integration
Before you can start interacting with the VTiger API using JavaScript, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. VTiger offers a REST-friendly API that requires basic authentication using a username and access key.
Creating a VTiger CRM Account
If you don't already have a VTiger account, you can sign up for a free trial on the VTiger website. Follow the instructions to create your account and log in to the VTiger CRM dashboard.
Generating Your VTiger API Access Key
To authenticate API requests, you'll need an access key. Follow these steps to generate your access key:
- Log in to your VTiger CRM account.
- Navigate to My Preferences in the user menu.
- Locate the Access Key section. Here, you'll find a randomly generated token that serves as your access key.
- Copy the access key and store it securely, as you'll need it for API authentication.
Understanding VTiger API Authentication
VTiger's API uses HTTP Basic Auth for authentication. This means you'll need to include your username and access key in the request headers. Here's a brief overview of how to set up authentication:
const username = 'your_username';
const accessKey = 'your_access_key';
const authHeader = 'Basic ' + btoa(username + ':' + accessKey);
Replace your_username
and your_access_key
with your actual VTiger credentials.
Testing Your VTiger API Connection
To ensure your setup is correct, you can test the connection by making a simple API call. Use the following JavaScript code to verify your authentication:
fetch('https://your_instance.odx.vtiger.com/restapi/v1/vtiger/default/me', {
method: 'GET',
headers: {
'Authorization': authHeader
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
If the authentication is successful, you should receive a JSON response with your user details. This confirms that your VTiger API setup is ready for further integration tasks.
For more detailed information on VTiger API authentication, refer to the VTiger REST API Manual.
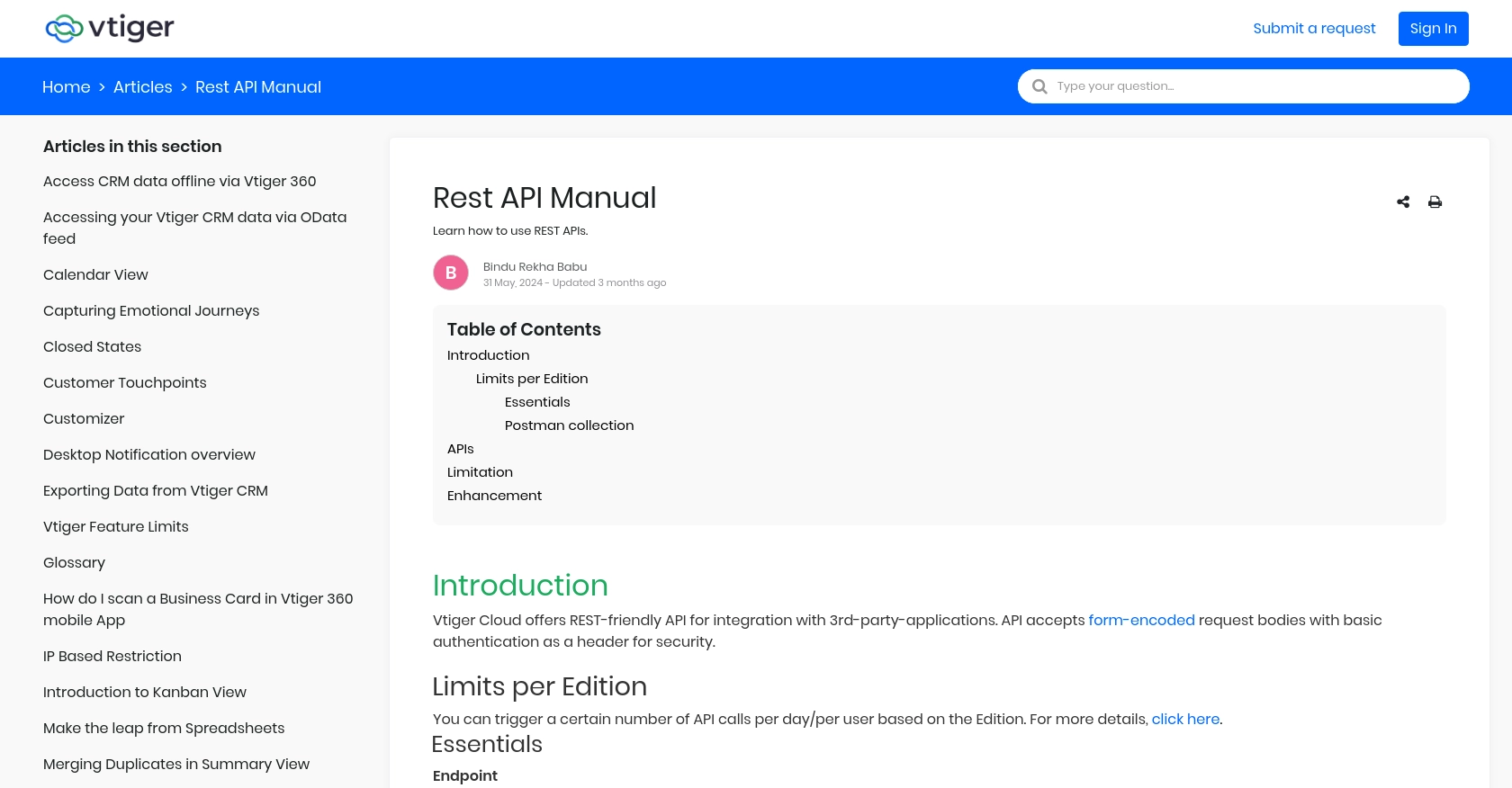
sbb-itb-96038d7
Executing VTiger API Calls Using JavaScript
To interact with the VTiger API using JavaScript, you need to make HTTP requests to the API endpoints. This section will guide you through the process of fetching records from VTiger CRM using JavaScript, ensuring you have the right setup and understanding to perform these operations efficiently.
Setting Up Your JavaScript Environment for VTiger API
Before making API calls, ensure you have a modern JavaScript environment. You can use Node.js or a browser-based environment to execute the code. Additionally, you'll need to use the fetch
API or a library like Axios to handle HTTP requests.
Fetching VTiger CRM Records with JavaScript
To retrieve records from VTiger, you'll use the query
endpoint. This allows you to specify the module and fields you want to access. Here's a step-by-step guide to making a query:
// Define your VTiger instance and authentication details
const domain = 'your_instance.odx.vtiger.com';
const username = 'your_username';
const accessKey = 'your_access_key';
const authHeader = 'Basic ' + btoa(username + ':' + accessKey);
// Define the module you want to query
const objectType = 'Contacts'; // Example module
const query = `select * from ${objectType} limit 100`;
// Construct the API URL
const url = `https://${domain}/restapi/v1/vtiger/default/query?query=${encodeURIComponent(query)}`;
// Make the API call
fetch(url, {
method: 'GET',
headers: {
'Authorization': authHeader
}
})
.then(response => response.json())
.then(data => {
if (data.success) {
console.log('Records:', data.result);
} else {
console.error('Error fetching records:', data.error);
}
})
.catch(error => console.error('Network Error:', error));
Replace your_instance
, your_username
, and your_access_key
with your actual VTiger credentials. The above code fetches records from the Contacts module, but you can modify the objectType
variable to query different modules.
Handling VTiger API Responses and Errors
When making API calls, it's crucial to handle responses and errors effectively. A successful response will have a success
property set to true
, and the records will be available in the result
property. If the API call fails, check the error
property for details.
Refer to the VTiger REST API Manual for more information on error codes and handling.
Verifying VTiger API Call Success
After executing the API call, verify the success by checking the returned data. If successful, the records should match those in your VTiger CRM sandbox. This ensures your API integration is functioning correctly.
By following these steps, you can efficiently retrieve records from VTiger CRM using JavaScript, enabling seamless integration with your applications.
Conclusion and Best Practices for VTiger API Integration with JavaScript
Integrating with the VTiger API using JavaScript provides a powerful way to automate and streamline data management tasks within your CRM. By following the steps outlined in this guide, you can efficiently retrieve records and enhance your application's capabilities.
Best Practices for Secure and Efficient VTiger API Usage
- Secure Storage of Credentials: Always store your VTiger username and access key securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of VTiger's API rate limits to avoid service disruptions. Implement retry logic with exponential backoff to manage rate limits effectively.
- Data Standardization: Ensure that data retrieved from VTiger is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API response errors gracefully. Refer to the VTiger REST API Manual for detailed error code information.
Streamlining Integrations with Endgrate
While integrating with VTiger's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including VTiger. This allows you to build once for each use case and streamline your integration processes.
By leveraging Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your customers. Visit Endgrate to learn more about how you can simplify your integration strategy.
Read More
Ready to get started?