Using the Pipeliner CRM API to Create Or Update Companies in Javascript
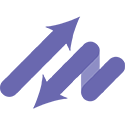
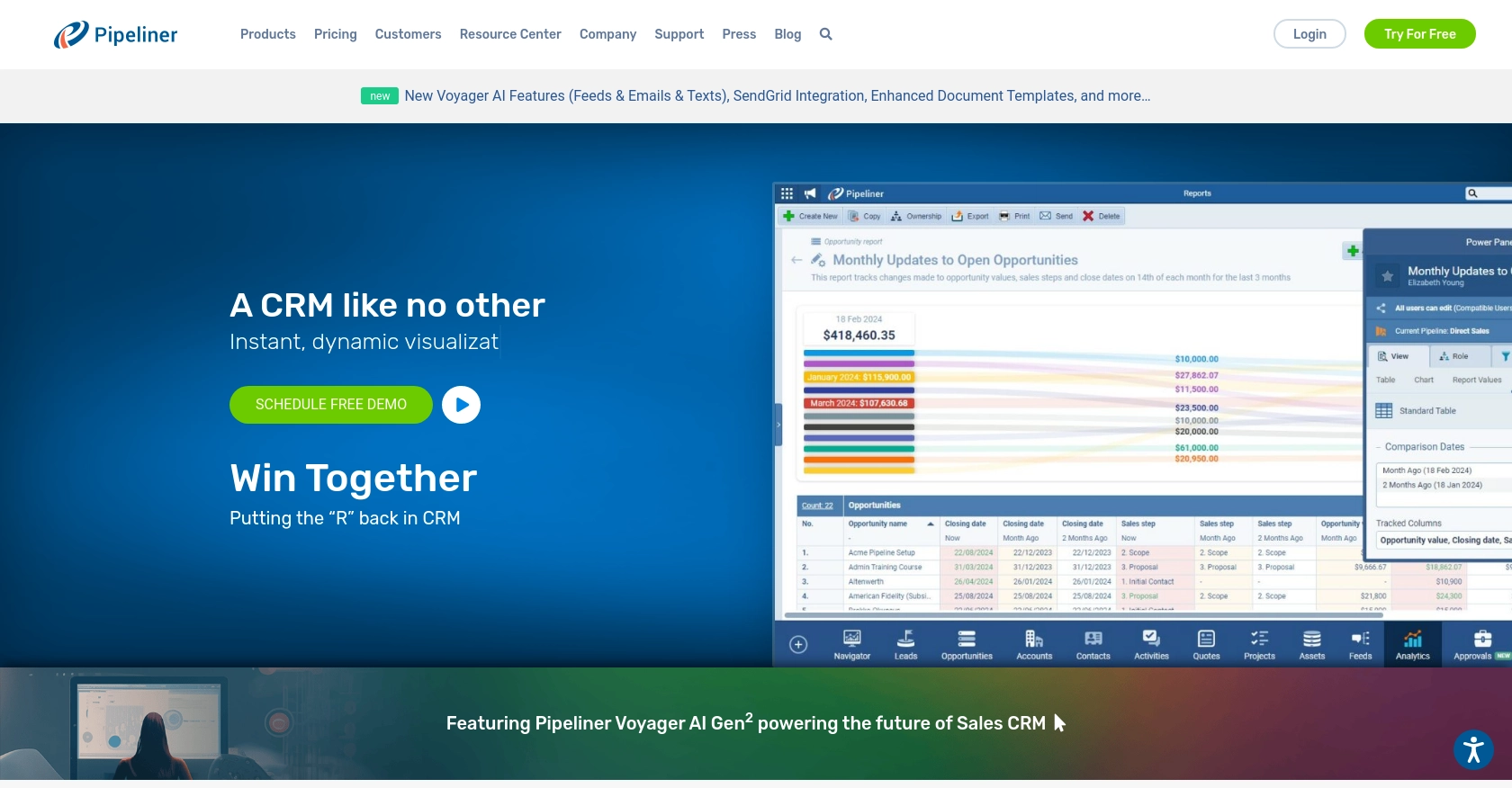
Introduction to Pipeliner CRM
Pipeliner CRM is a robust customer relationship management platform designed to enhance sales processes and improve team collaboration. With its intuitive interface and comprehensive features, Pipeliner CRM empowers businesses to manage leads, track opportunities, and streamline customer interactions effectively.
Developers may want to integrate with Pipeliner CRM's API to automate and optimize business workflows. For example, using the Pipeliner CRM API, developers can create or update company records seamlessly, ensuring that the CRM data remains accurate and up-to-date. This integration can be particularly useful for synchronizing data between Pipeliner CRM and other business applications, enhancing productivity and data consistency.
Setting Up Your Pipeliner CRM Test Account
Before you can start integrating with the Pipeliner CRM API, you'll need to set up a test account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to create a sandbox environment and obtain the necessary credentials for authentication.
Creating a Pipeliner CRM Account
If you don't already have a Pipeliner CRM account, you can sign up for one by visiting the Pipeliner CRM website. Follow the on-screen instructions to create your account and log in.
Accessing the Administration Panel
Once logged in, select your team space and navigate to the administration panel. This is where you'll manage applications and obtain API access credentials.
Generating API Keys for Authentication
- In the administration panel, go to the Unit, Users & Roles tab.
- Select Applications and create a new application.
- Click on Show API Access to reveal your API username and password.
These credentials are used for Basic Authentication and are generated only once, so be sure to store them securely.
Understanding Space ID and Service URL
Every API request requires a Space ID and Service URL, which are unique to your team space. Ensure you have these details handy as they are essential for formatting your API requests.
For more detailed information on authentication, refer to the Pipeliner CRM Authentication Documentation.
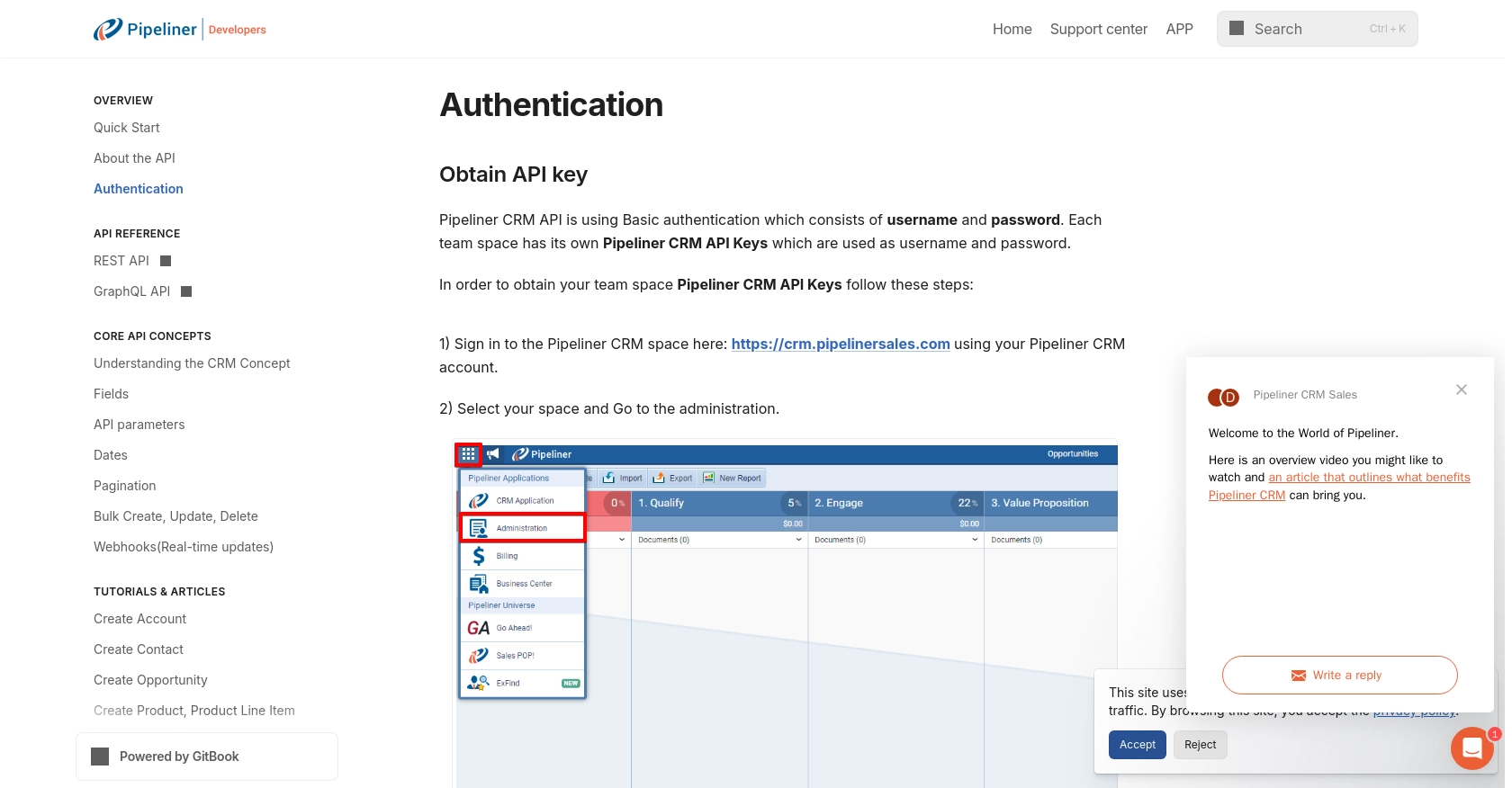
sbb-itb-96038d7
Making API Calls to Pipeliner CRM Using JavaScript
In this section, we'll explore how to interact with the Pipeliner CRM API using JavaScript to create or update company records. This involves setting up the environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Additionally, you'll need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Creating or Updating Companies with Pipeliner CRM API
To create or update company records in Pipeliner CRM, you'll need to use the appropriate API endpoints. Below is a sample code snippet demonstrating how to perform these actions using JavaScript and the axios
library.
const axios = require('axios');
// Define your API credentials and endpoint
const username = 'Your_API_Username';
const password = 'Your_API_Password';
const spaceId = 'Your_Space_ID';
const serviceUrl = 'https://api.pipelinersales.com';
// Function to create or update a company
async function createOrUpdateCompany(companyData) {
try {
const response = await axios({
method: 'post', // Use 'put' for updating
url: `${serviceUrl}/entities/Companies`,
auth: {
username: username,
password: password
},
data: companyData
});
if (response.status === 200 || response.status === 201) {
console.log('Company record successfully created or updated:', response.data);
} else {
console.log('Failed to create or update company record:', response.status, response.statusText);
}
} catch (error) {
console.error('Error making API call:', error.response ? error.response.data : error.message);
}
}
// Sample company data
const companyData = {
name: 'Example Company',
industry: 'Technology',
website: 'https://example.com'
};
// Call the function
createOrUpdateCompany(companyData);
Verifying API Call Success in Pipeliner CRM
After executing the API call, you can verify the success by checking the Pipeliner CRM dashboard. Navigate to the Companies section to see if the new or updated company record appears as expected.
Handling Errors and API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The Pipeliner CRM API may return various HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
For more detailed information on API calls, refer to the Pipeliner CRM API Documentation.
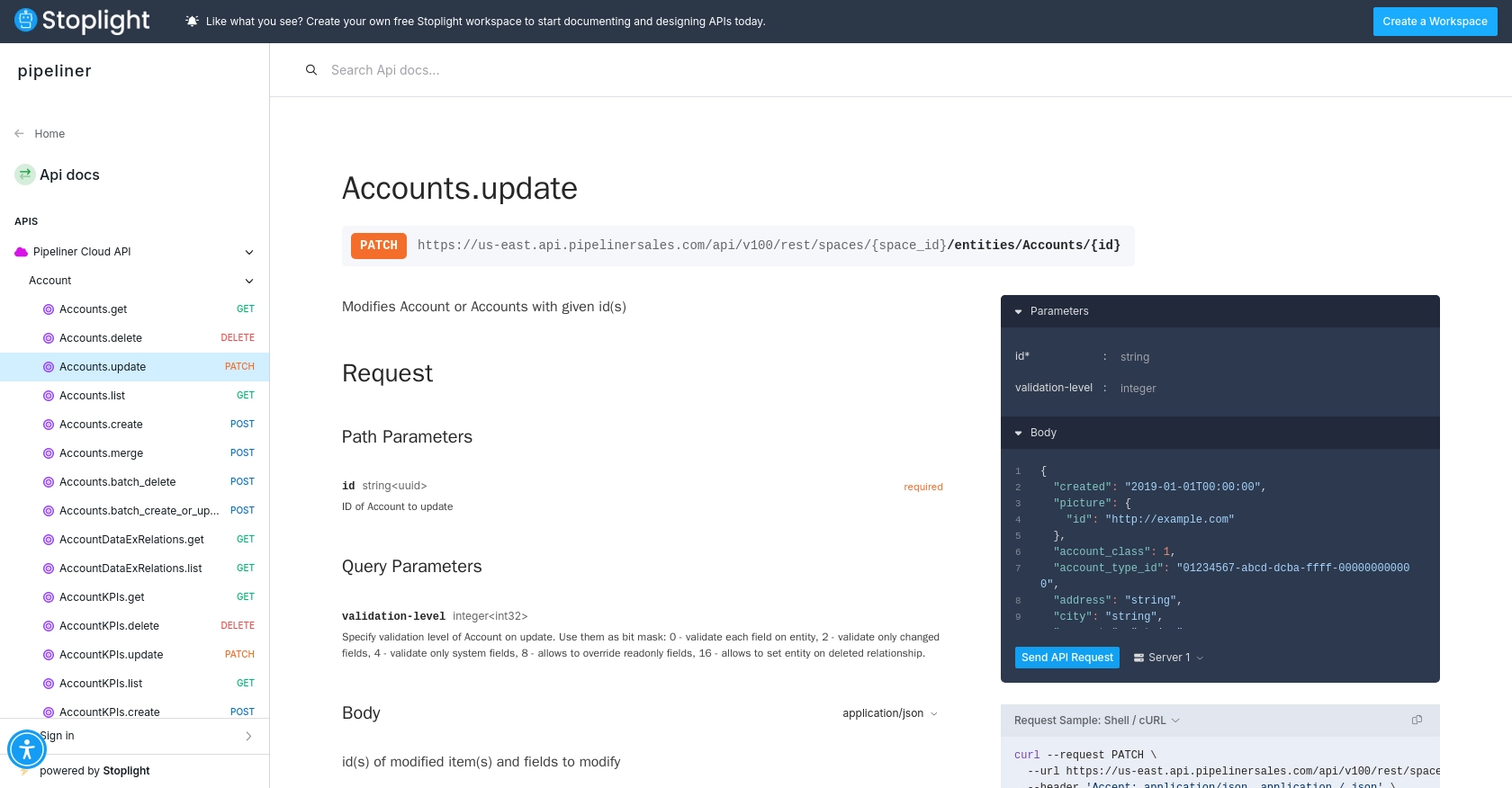
Best Practices for Pipeliner CRM API Integration
When integrating with the Pipeliner CRM API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key considerations:
- Secure Storage of Credentials: Store your API username and password securely, using environment variables or a secure vault, to prevent unauthorized access.
- Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Standardize and transform data fields to match your application's requirements, ensuring consistency across systems.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide informative feedback to users.
- Pagination: Use pagination effectively to manage large datasets, leveraging the end_cursor and has_next_page fields as described in the Pipeliner CRM Pagination Documentation.
Streamlining Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Pipeliner CRM. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while outsourcing integration complexities to Endgrate.
- Build Once, Deploy Everywhere: Create a single integration for multiple platforms, reducing development efforts.
- Enhance User Experience: Offer your customers an intuitive and seamless integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website today.
Read More
- https://endgrate.com/provider/pipelinercrm
- https://developers.pipelinersales.com/api-docs/overview/authentication
- https://developers.pipelinersales.com/api-docs/core-api-concepts/pagination
- https://pipeliner.stoplight.io/docs/api-docs/p5b3cyeorbick-accounts-update
- https://pipeliner.stoplight.io/docs/api-docs/89p8xi32mv7cj-accounts-create
Ready to get started?