How to Create Opportunities with the Sugar Market API in Python
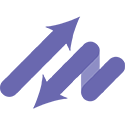
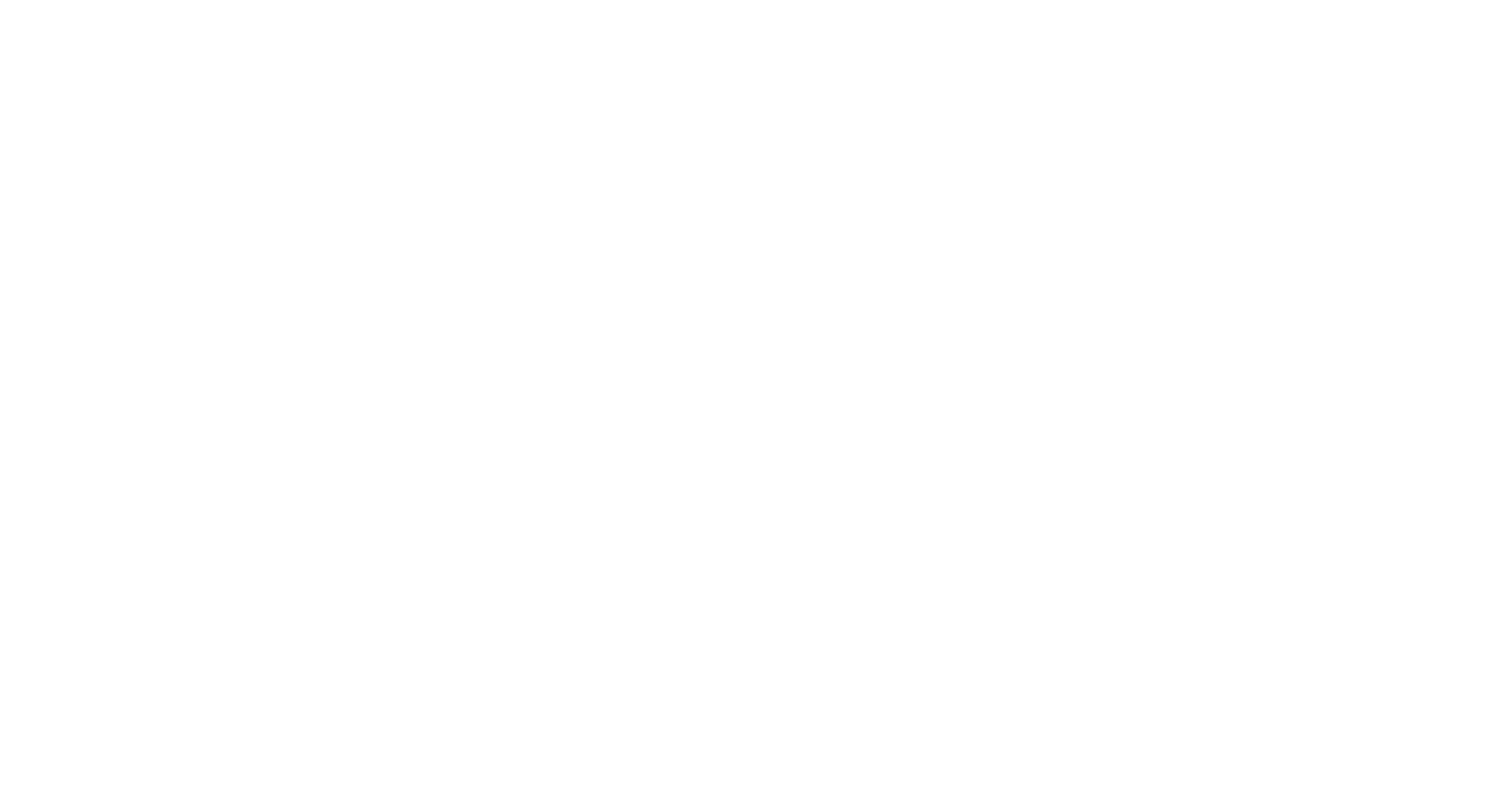
Introduction to Sugar Market API
Sugar Market is a robust marketing automation platform designed to enhance marketing efforts for B2B businesses. It offers a comprehensive suite of tools that help streamline marketing campaigns, lead nurturing, and analytics, making it a popular choice for businesses looking to optimize their marketing strategies.
Developers might want to integrate with the Sugar Market API to automate and manage marketing opportunities effectively. For example, using the Sugar Market API, a developer can create opportunities directly from a CRM system, ensuring that sales teams have up-to-date information on potential leads and can act swiftly to convert them.
Setting Up Your Sugar Market Test/Sandbox Account
Before you can start creating opportunities with the Sugar Market API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Sugar Market Sandbox Account
To begin, visit the Sugar Market API documentation and sign up for a sandbox account. This account will provide you with access to the necessary tools and resources to test API interactions.
- Navigate to the Sugar Market website and click on the sign-up link for a sandbox account.
- Fill out the registration form with your details and submit it.
- Once registered, you will receive an email with instructions to activate your account.
Generating API Credentials for Sugar Market
With your sandbox account set up, the next step is to generate the API credentials needed for authentication. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market sandbox account.
- Navigate to the API settings section in your account dashboard.
- Click on "Create New API Key" and fill in the required information.
- After creating the API key, you will receive a client ID and client secret. Make sure to store these securely as they will be used for authenticating your API requests.
Configuring OAuth for Sugar Market API
To authenticate API requests, you will need to configure OAuth using the client ID and client secret obtained earlier:
- In your development environment, set up a configuration file to store your client ID and client secret.
- Use these credentials to request an access token from the Sugar Market authorization server.
- Once you have the access token, include it in the headers of your API requests to authenticate them.
With your sandbox account and API credentials ready, you are now set to start making API calls to create opportunities in Sugar Market using Python.
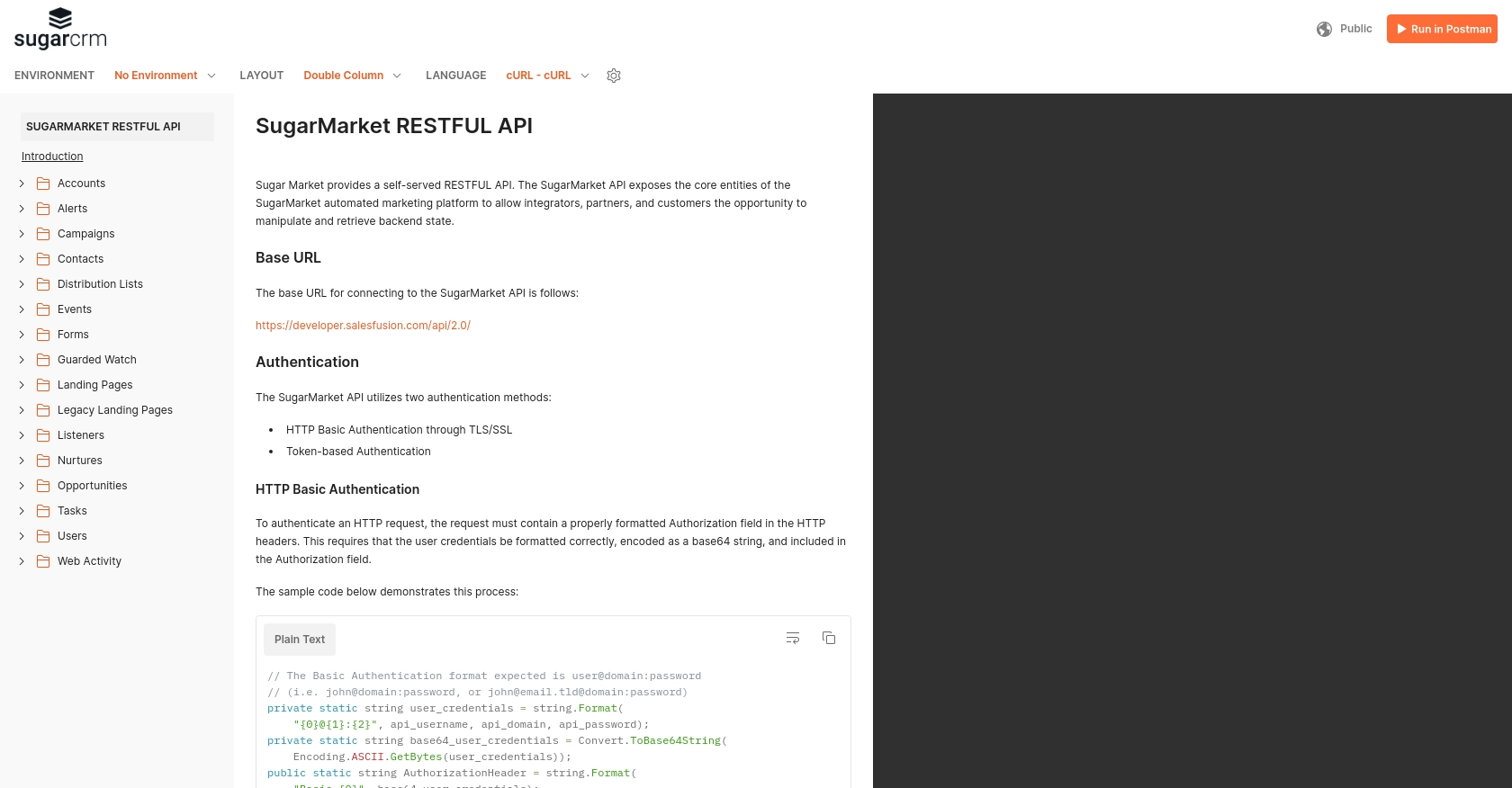
sbb-itb-96038d7
Making API Calls to Create Opportunities with Sugar Market in Python
To interact with the Sugar Market API and create opportunities, you'll need to use Python, a versatile and widely-used programming language. Ensure you have Python 3.11.1 installed on your machine, along with the necessary dependencies.
Setting Up Your Python Environment for Sugar Market API
Before making API calls, you need to set up your Python environment. Install the required libraries using pip, the Python package installer:
pip install requests
The requests
library will help you make HTTP requests to the Sugar Market API.
Creating Opportunities with Sugar Market API Using Python
Now that your environment is ready, you can proceed to create opportunities in Sugar Market. Follow these steps to make the API call:
import requests
# Define the API endpoint for creating opportunities
url = "https://api.sugarmarket.com/v1/opportunities"
# Set the request headers, including the access token for authentication
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the opportunity data
opportunity_data = {
"name": "New Business Opportunity",
"amount": 5000,
"close_date": "2023-12-31",
"stage": "Prospecting"
}
# Make the POST request to create the opportunity
response = requests.post(url, json=opportunity_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Opportunity created successfully!")
else:
print(f"Failed to create opportunity: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth configuration. The code above sends a POST request to the Sugar Market API to create a new opportunity with specified details such as name, amount, close date, and stage.
Verifying the API Call and Handling Errors
After running the script, check your Sugar Market sandbox account to verify that the opportunity has been created. If the request is successful, you should see the new opportunity listed in your account.
In case of errors, the response will include an error code and message. Common error codes include:
- 400 Bad Request: The request was invalid. Check the data format and required fields.
- 401 Unauthorized: Authentication failed. Ensure your access token is correct.
- 500 Internal Server Error: An error occurred on the server. Try again later.
Refer to the Sugar Market API documentation for more details on error handling and troubleshooting.
Best Practices for Using Sugar Market API
When working with the Sugar Market API, it's essential to follow best practices to ensure efficient and secure integration. Here are some key recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid being throttled. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Check the Sugar Market API documentation for specific rate limit details.
- Standardize Data Fields: Ensure that the data you send to the API is standardized and validated. This helps prevent errors and ensures consistency in your marketing data.
- Monitor API Usage: Regularly monitor your API usage and logs to identify any anomalies or issues. This proactive approach helps maintain the health of your integration.
Streamlining Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex, especially when dealing with multiple platforms. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sugar Market.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case, rather than multiple times for different integrations.
- Offer an easy and intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined integration process.
Read More
Ready to get started?