Using the BigCommerce API to Get Products (with PHP examples)
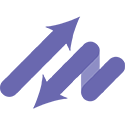
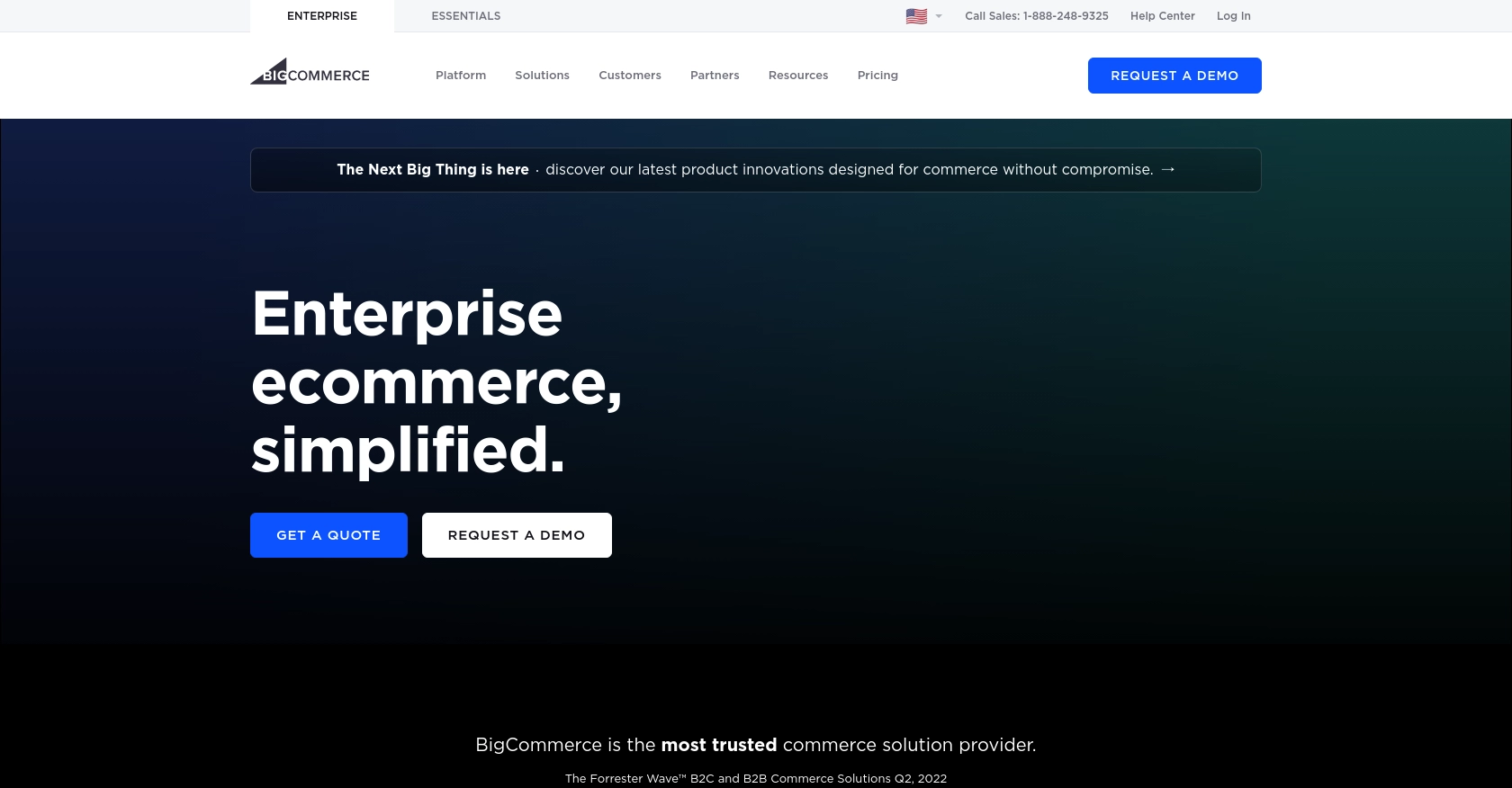
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a comprehensive suite of tools for managing products, orders, and customer relationships, making it an ideal choice for businesses looking to scale their online presence.
Integrating with the BigCommerce API allows developers to automate and enhance various aspects of store management. For example, using the API to retrieve product information can streamline inventory management, enabling real-time updates and synchronization with other systems.
This article will guide you through using PHP to interact with the BigCommerce API, specifically focusing on retrieving product data. By the end of this tutorial, you'll be equipped to efficiently access and manage product information within your BigCommerce store.
Setting Up Your BigCommerce Test or Sandbox Account
Before diving into the integration process with the BigCommerce API, it's essential to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting your live store data, ensuring a safe and controlled development process.
Creating a BigCommerce Account
If you don't already have a BigCommerce account, you can sign up for a free trial on the BigCommerce website. This trial will provide you with access to a sandbox environment where you can test API interactions.
- Visit the BigCommerce website and click on "Start Your Free Trial."
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access your store's dashboard.
Generating API Credentials
To interact with the BigCommerce API, you'll need to generate API credentials. These credentials will allow your application to authenticate and make requests to the BigCommerce servers.
- Navigate to the "Advanced Settings" section in your BigCommerce dashboard.
- Select "API Accounts" and click on "Create API Account."
- Fill in the necessary details, such as the name and description of your API account.
- Set the OAuth scopes required for your application. For retrieving product data, ensure you have the appropriate read permissions.
- Click "Save" to generate your API credentials, including the client ID, client secret, and access token.
- Store these credentials securely, as you'll need them to authenticate your API requests.
Understanding BigCommerce Authentication
BigCommerce uses OAuth-based authentication for its API. You'll need to include the access token in the X-Auth-Token
header of your API requests. For more details on authentication, refer to the BigCommerce authentication documentation.
With your test account and API credentials ready, you can now proceed to make API calls to retrieve product data, as detailed in the following sections of this guide.
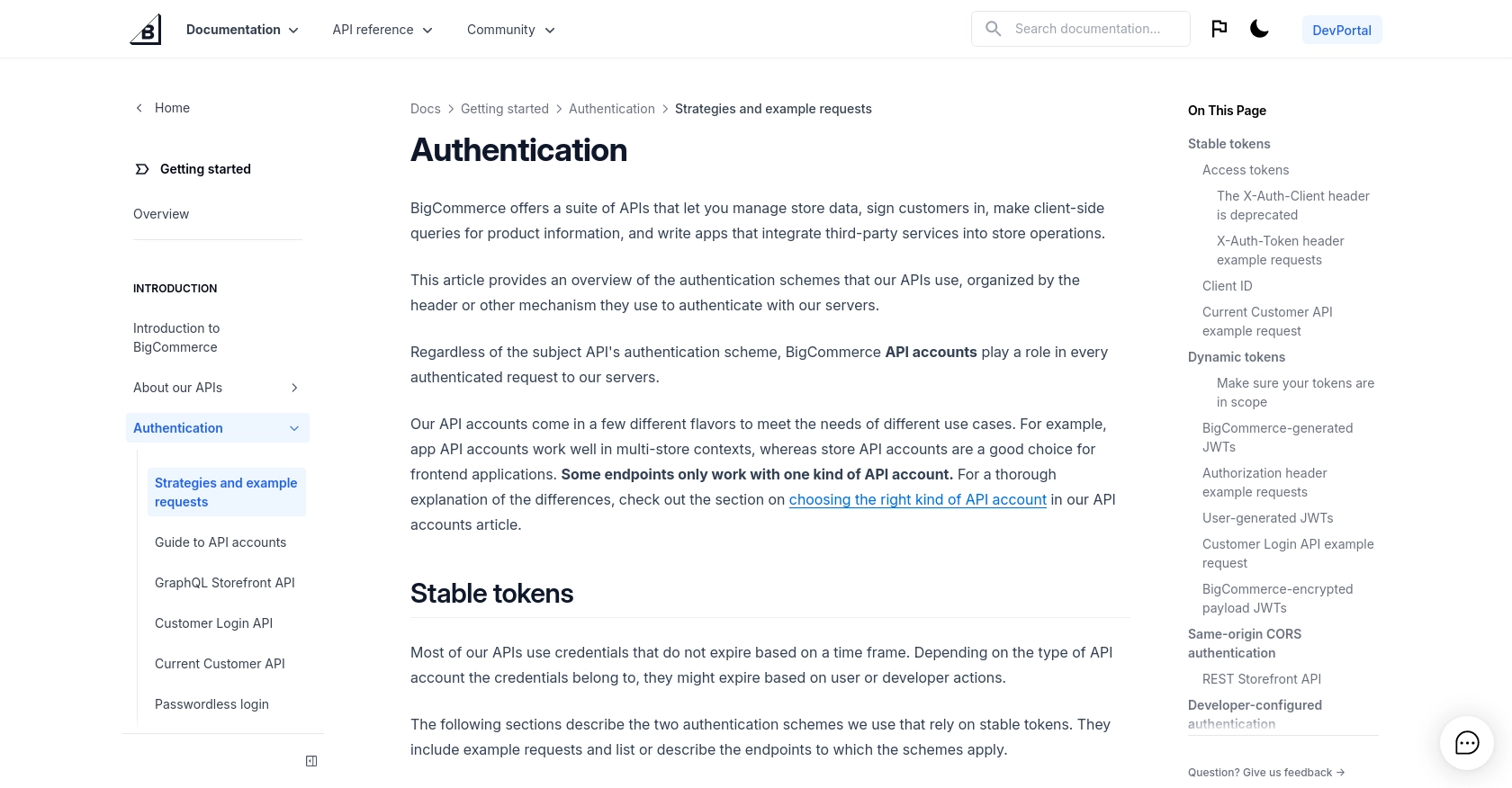
sbb-itb-96038d7
Making API Calls to Retrieve Products from BigCommerce Using PHP
To effectively interact with the BigCommerce API and retrieve product data, you'll need to set up your PHP environment and make authenticated requests. This section will guide you through the process of making API calls using PHP, ensuring you can access and manage product information seamlessly.
Setting Up Your PHP Environment for BigCommerce API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP version 7.4 or higher and the cURL extension enabled to handle HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m
to list installed modules.
Installing Required PHP Dependencies
To simplify the process of making HTTP requests, you can use the Guzzle HTTP client. Install it via Composer by running the following command:
composer require guzzlehttp/guzzle
Example Code to Retrieve Products from BigCommerce
With your environment set up, you can now write PHP code to retrieve product data from BigCommerce. The following example demonstrates how to make a GET request to the BigCommerce API to fetch all products:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$storeHash = 'your_store_hash';
$accessToken = 'your_access_token';
$response = $client->request('GET', "https://api.bigcommerce.com/stores/{$storeHash}/v3/catalog/products", [
'headers' => [
'X-Auth-Token' => $accessToken,
'Accept' => 'application/json',
]
]);
$products = json_decode($response->getBody(), true);
foreach ($products['data'] as $product) {
echo "Product Name: " . $product['name'] . "\n";
echo "SKU: " . $product['sku'] . "\n";
echo "Price: $" . $product['price'] . "\n\n";
}
Replace your_store_hash
and your_access_token
with your actual store hash and access token. This script uses Guzzle to send a GET request to the BigCommerce API, retrieves the product data, and prints out the name, SKU, and price of each product.
Verifying Successful API Requests in BigCommerce
After running the script, verify the successful retrieval of product data by checking the output in your terminal. The product details should match those in your BigCommerce store.
Handling Errors and Common Error Codes in BigCommerce API
When making API calls, it's crucial to handle potential errors gracefully. Common HTTP status codes you might encounter include:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
Use try-catch blocks in your PHP code to handle exceptions and log errors for troubleshooting.
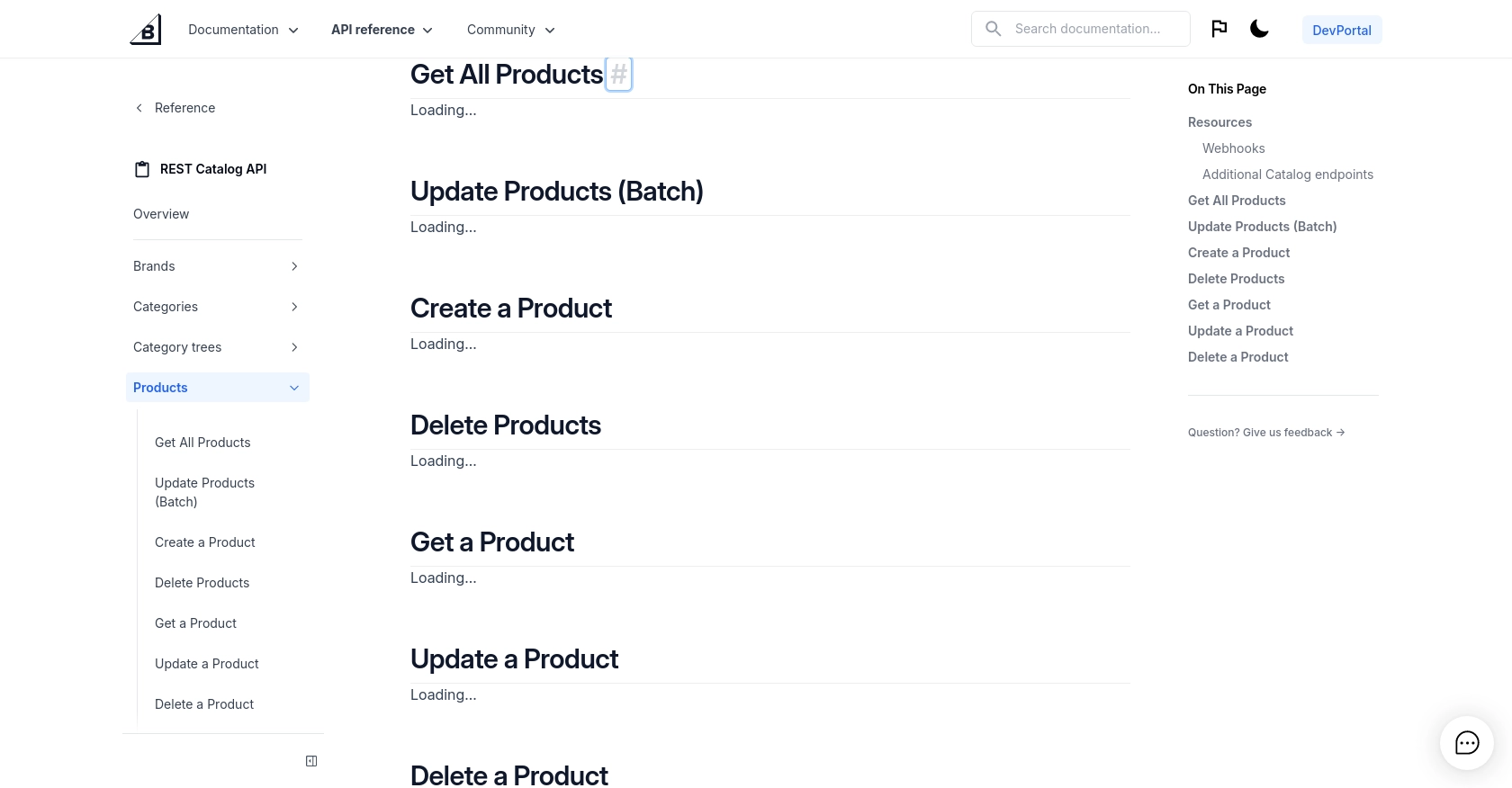
Conclusion and Best Practices for BigCommerce API Integration Using PHP
Integrating with the BigCommerce API using PHP allows developers to efficiently manage and retrieve product data, enhancing the overall functionality and automation of e-commerce operations. By following the steps outlined in this guide, you can seamlessly access product information and ensure your store's data is synchronized with other systems.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store Credentials: Always store your API credentials, such as the access token, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Optimize Data Handling: When retrieving large datasets, use pagination to manage data efficiently and reduce the load on your application and BigCommerce servers.
- Log and Monitor API Calls: Implement logging for your API requests and responses to monitor performance and troubleshoot issues effectively.
Enhancing Your Integration Strategy with Endgrate
While integrating with the BigCommerce API directly offers flexibility, using a tool like Endgrate can further streamline your integration process. Endgrate provides a unified API endpoint that connects to multiple platforms, allowing you to manage various integrations with ease. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can simplify your integration strategy by visiting Endgrate's website and discover how it can enhance your e-commerce operations.
Read More
Ready to get started?