Using the Postgres API to Create or Update Records in Python
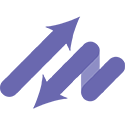
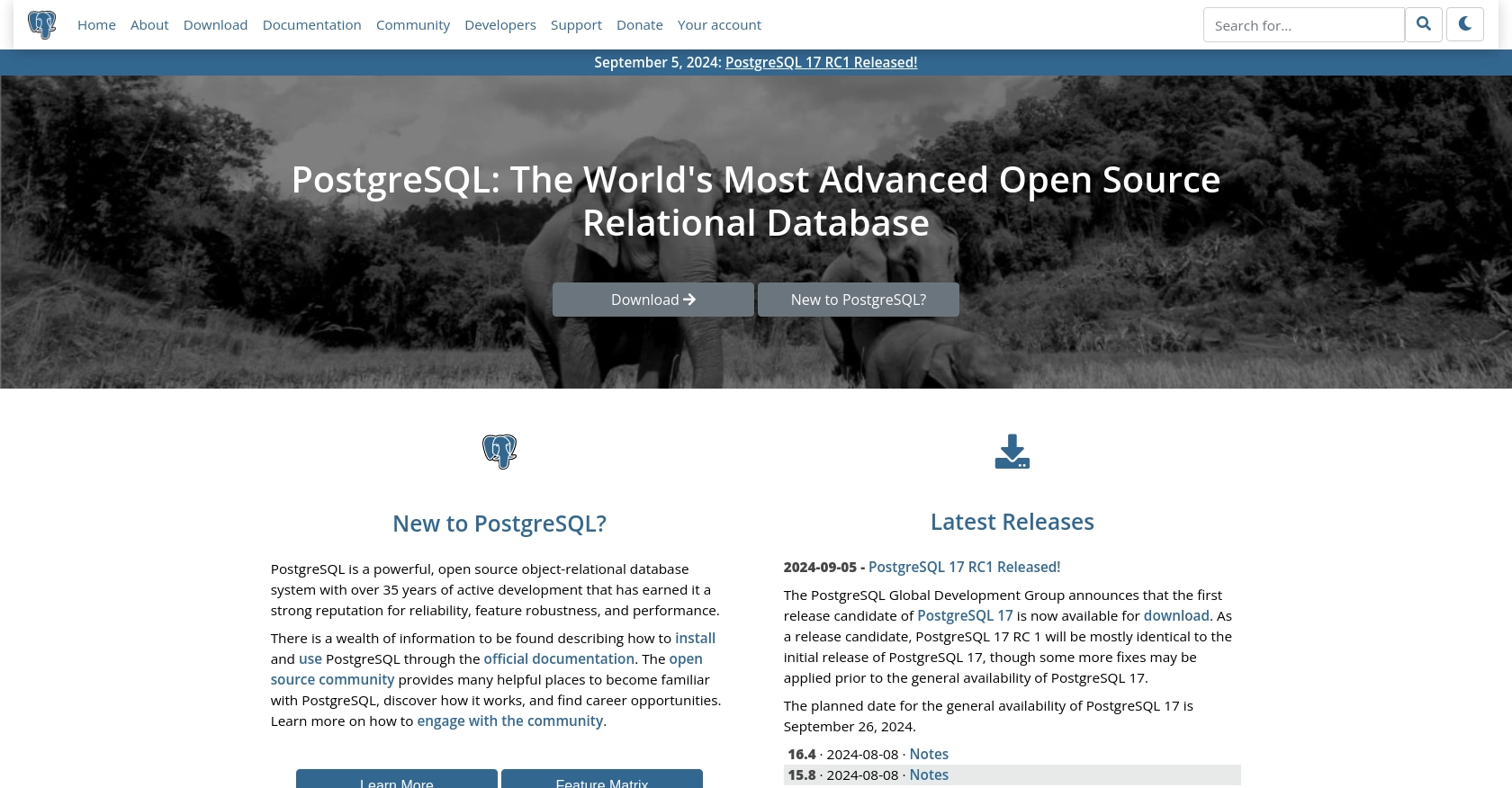
Introduction to PostgreSQL API Integration
PostgreSQL, often referred to as Postgres, is a powerful, open-source relational database management system known for its robustness, scalability, and compliance with SQL standards. It is widely used by developers and organizations to manage and store data efficiently, offering advanced features such as full-text search, custom data types, and concurrency control.
Integrating with the PostgreSQL API allows developers to automate and streamline database operations, such as creating or updating records programmatically. For example, a developer might use the PostgreSQL API to update customer information in a database whenever a user updates their profile on a web application, ensuring data consistency across platforms.
Setting Up a PostgreSQL Test Environment for API Integration
Before diving into creating or updating records using the PostgreSQL API, it's crucial to set up a test environment. This allows developers to experiment and test their code without affecting production data. PostgreSQL offers a robust setup process that can be tailored to your development needs.
Installing PostgreSQL Locally
To begin, you'll need to install PostgreSQL on your local machine. Follow these steps to set up a local PostgreSQL server:
- Visit the PostgreSQL download page and select the appropriate version for your operating system.
- Follow the installation instructions provided for your OS. Ensure that you install the PostgreSQL server and command-line tools.
- Once installed, initialize the database cluster using the command line:
initdb -D /usr/local/pgsql/data
This command sets up the necessary data directory for PostgreSQL.
Creating a Test Database and User
With PostgreSQL installed, the next step is to create a test database and user:
- Start the PostgreSQL server:
pg_ctl -D /usr/local/pgsql/data -l logfile start
- Access the PostgreSQL command line interface (CLI) using the following command:
psql postgres
- Create a new database for testing:
CREATE DATABASE test_db;
- Create a new user with access to the test database:
CREATE USER test_user WITH PASSWORD 'securepassword';
- Grant privileges to the new user:
GRANT ALL PRIVILEGES ON DATABASE test_db TO test_user;
Configuring Client Authentication
To allow your application to connect to the PostgreSQL server, configure the client authentication settings:
- Open the
pg_hba.conf
file located in the data directory. - Add the following line to allow password authentication:
host test_db test_user 127.0.0.1/32 md5
Save the changes and restart the PostgreSQL server to apply the new settings.
Connecting to the Test Database
With the test environment set up, you can now connect to the test database using Python. Ensure you have the psycopg2
library installed:
pip install psycopg2
Use the following Python code to establish a connection:
import psycopg2
try:
connection = psycopg2.connect(
dbname="test_db",
user="test_user",
password="securepassword",
host="127.0.0.1",
port="5432"
)
print("Connection to PostgreSQL successful")
except Exception as e:
print(f"Error connecting to PostgreSQL: {e}")
This setup ensures you have a safe environment to test your PostgreSQL API interactions, allowing you to create or update records without impacting live data.
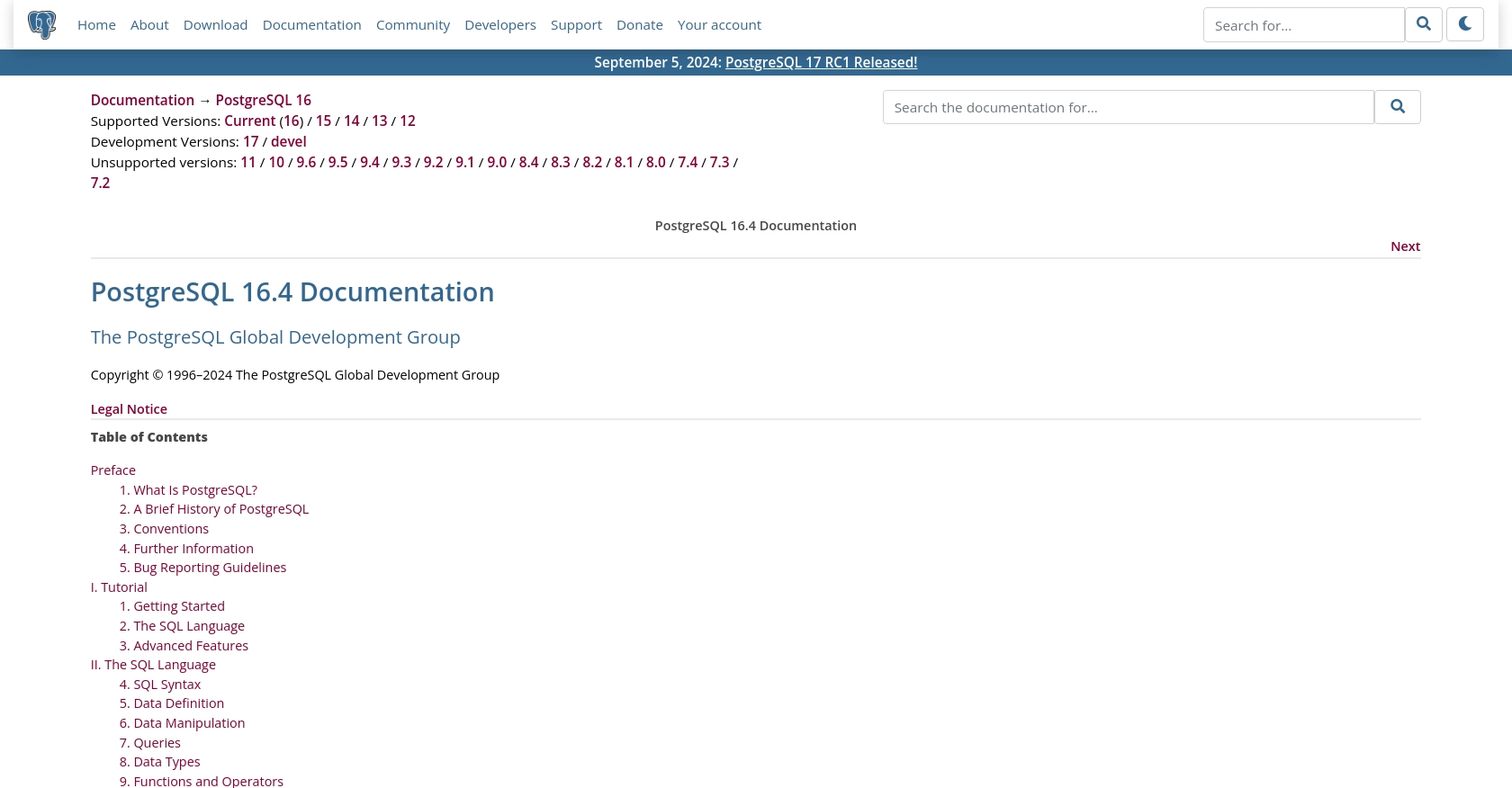
sbb-itb-96038d7
Making API Calls to Create or Update Records in PostgreSQL Using Python
To interact with PostgreSQL and perform operations like creating or updating records, you'll need to use Python's psycopg2
library. This library provides a robust interface for connecting to PostgreSQL databases and executing SQL commands.
Setting Up Your Python Environment for PostgreSQL API Integration
Before making any API calls, ensure your Python environment is set up correctly. You'll need Python 3.x and the psycopg2
library installed. If you haven't installed psycopg2
yet, use the following command:
pip install psycopg2
Connecting to PostgreSQL Database Using Python
To connect to your PostgreSQL test database, use the following Python code. This example demonstrates how to establish a connection and handle potential errors:
import psycopg2
try:
connection = psycopg2.connect(
dbname="test_db",
user="test_user",
password="securepassword",
host="127.0.0.1",
port="5432"
)
cursor = connection.cursor()
print("Connected to PostgreSQL database successfully")
except Exception as e:
print(f"Error connecting to PostgreSQL: {e}")
This code snippet connects to the test_db
database using the credentials specified. If the connection is successful, a cursor object is created for executing SQL commands.
Creating Records in PostgreSQL Using Python
To create a new record in your PostgreSQL database, use the following code. This example inserts a new customer record into a hypothetical customers
table:
try:
insert_query = """
INSERT INTO customers (name, email, age)
VALUES (%s, %s, %s)
"""
cursor.execute(insert_query, ("John Doe", "john.doe@example.com", 30))
connection.commit()
print("Record inserted successfully")
except Exception as e:
print(f"Error inserting record: {e}")
This code uses a parameterized query to insert data, which helps prevent SQL injection attacks. After executing the query, the transaction is committed to the database.
Updating Records in PostgreSQL Using Python
To update an existing record, modify the SQL query as shown below. This example updates the email of a customer based on their name:
try:
update_query = """
UPDATE customers
SET email = %s
WHERE name = %s
"""
cursor.execute(update_query, ("new.email@example.com", "John Doe"))
connection.commit()
print("Record updated successfully")
except Exception as e:
print(f"Error updating record: {e}")
Again, parameterized queries are used to ensure security. The transaction is committed after the update operation.
Handling Errors and Verifying Success in PostgreSQL API Calls
It's important to handle errors gracefully when interacting with databases. The examples above include basic error handling using try-except blocks. Additionally, verify the success of your operations by checking the affected rows or querying the database to confirm changes.
After executing any SQL command, you can use cursor.rowcount
to check how many rows were affected:
print(f"Number of rows affected: {cursor.rowcount}")
This provides a quick way to verify that your insert or update operations were successful.
Conclusion and Best Practices for PostgreSQL API Integration
Integrating with the PostgreSQL API using Python provides developers with a powerful toolset to automate and streamline database operations. By following the steps outlined in this guide, you can efficiently create and update records in your PostgreSQL database, ensuring data consistency and reliability across your applications.
Best Practices for Secure and Efficient PostgreSQL API Usage
- Secure Credential Storage: Always store database credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Although PostgreSQL does not impose rate limits, ensure your application handles database connections efficiently to avoid performance bottlenecks.
- Use Parameterized Queries: Always use parameterized queries to protect against SQL injection attacks and ensure data integrity.
- Monitor Database Performance: Regularly monitor your database's performance and optimize queries to maintain efficient operations.
Streamlining Integrations with Endgrate
While integrating with PostgreSQL directly is powerful, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including PostgreSQL. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover an intuitive integration experience that scales with your business needs.
Read More
Ready to get started?