How to Get Page Views with the Pendo API in Javascript
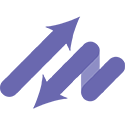
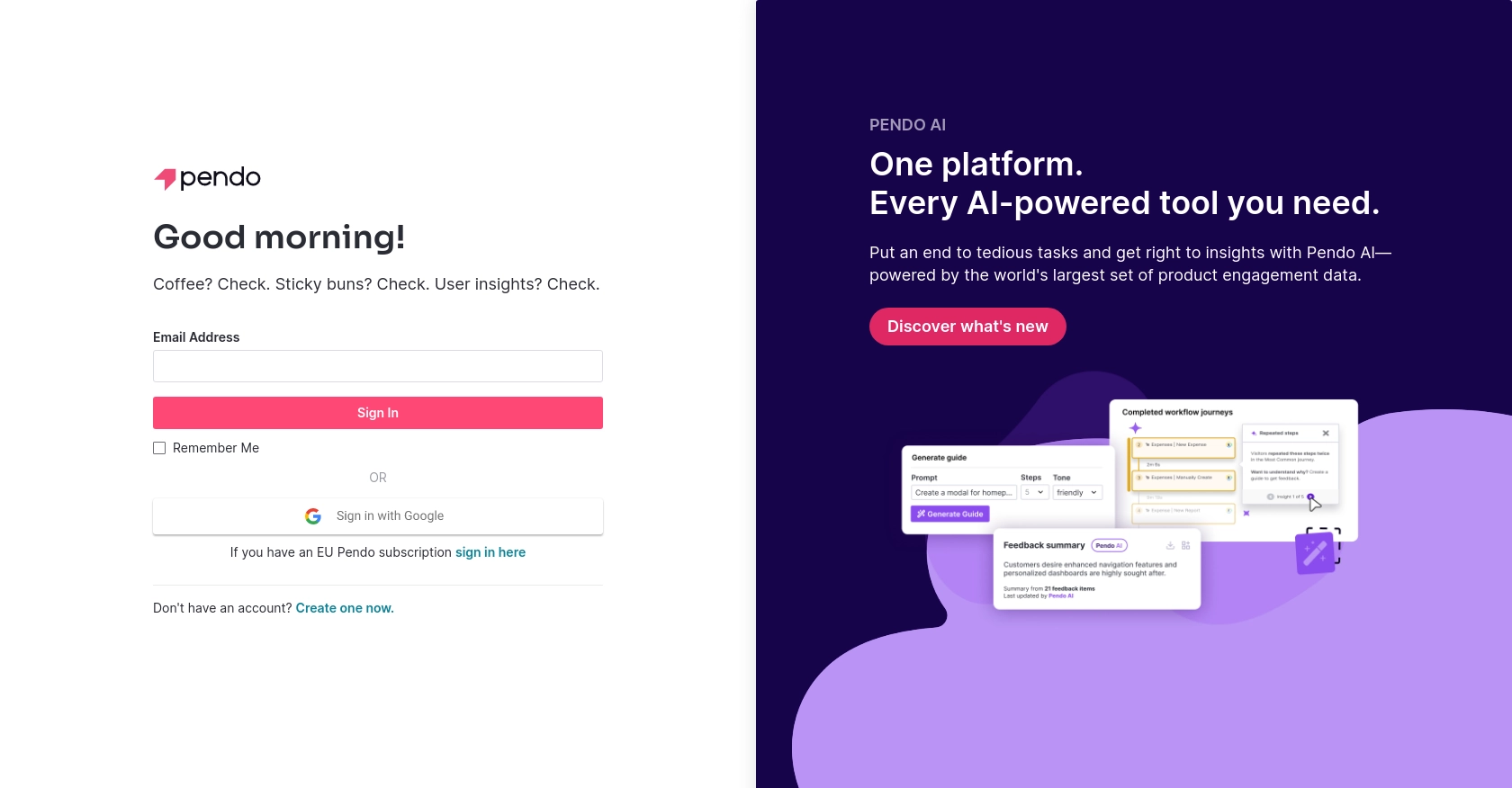
Introduction to Pendo
Pendo is a powerful product analytics and user feedback platform designed to help businesses understand and improve their user experience. By providing insights into user behavior, Pendo enables companies to make data-driven decisions to enhance product engagement and customer satisfaction.
Developers may want to integrate with Pendo's API to access detailed analytics, such as page views, to better understand how users interact with their applications. For example, a developer could use the Pendo API to retrieve page view data and analyze user engagement trends, helping to optimize the user journey and improve feature adoption.
Setting Up Your Pendo Test Account for API Access
Before you can start retrieving page view data using the Pendo API, you'll need to set up a Pendo account. Pendo offers a free trial, which is perfect for testing and development purposes.
Creating a Pendo Account
If you don't already have a Pendo account, follow these steps to create one:
- Visit the Pendo website and click on the "Start Free Trial" button.
- Fill out the registration form with your details and submit it to create your account.
- Once your account is created, log in to access the Pendo dashboard.
Generating Your Pendo API Key
Pendo uses API key-based authentication to allow developers to access their API. Follow these steps to generate your API key:
- Navigate to the "Settings" section in the Pendo dashboard.
- Under "Integrations," find the API key management section.
- Click on "Generate API Key" and provide a name for your key to identify it later.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
With your Pendo account set up and your API key in hand, you're ready to start making API calls to retrieve page view data. In the next section, we'll guide you through the process of making these calls using JavaScript.
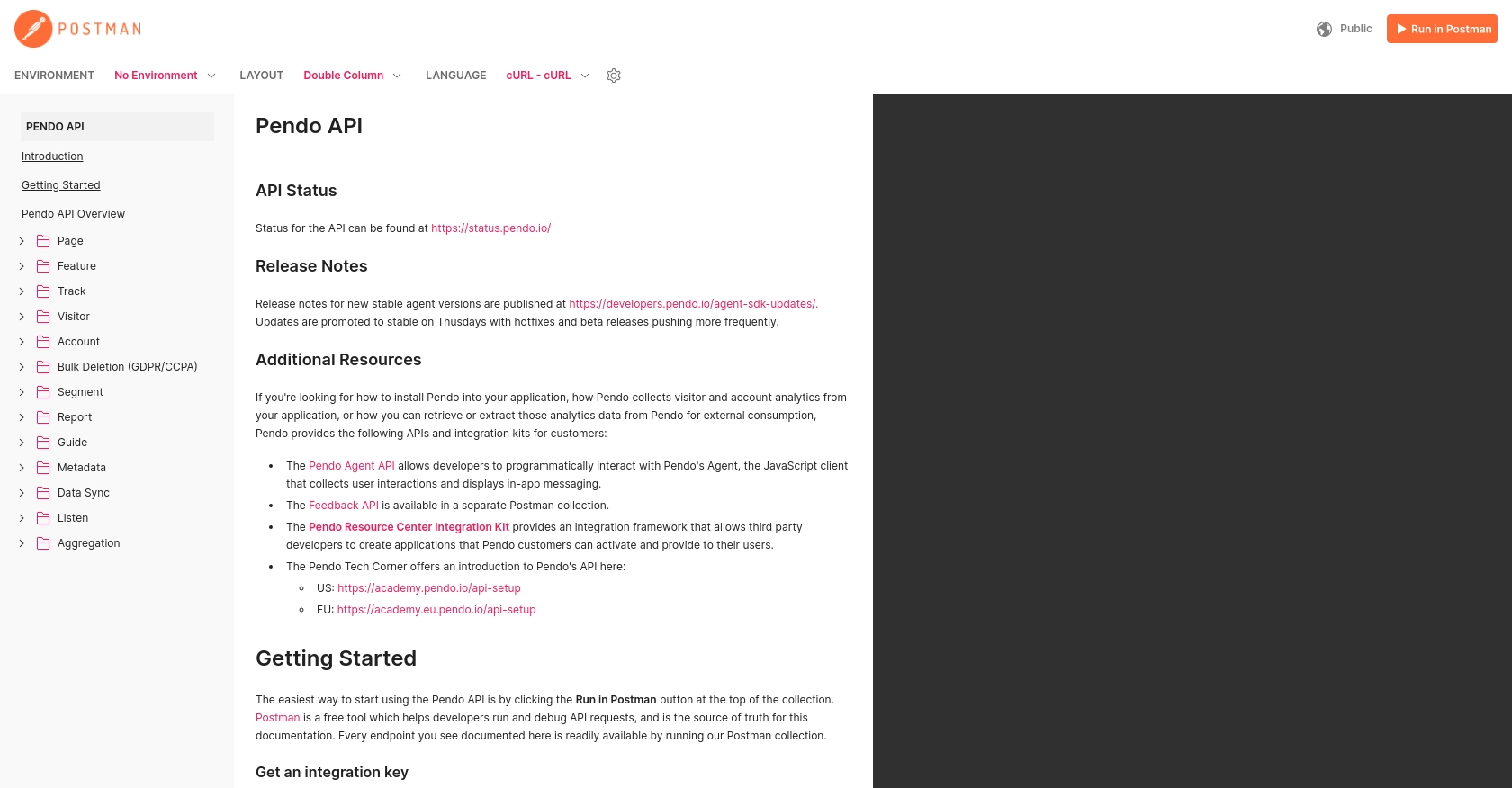
sbb-itb-96038d7
Making API Calls to Retrieve Page Views with Pendo in JavaScript
To effectively retrieve page view data from Pendo using JavaScript, you need to ensure you have the right setup and understand the necessary steps to make API calls. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Pendo API Integration
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or a browser-based setup. For this tutorial, we'll focus on using Node.js.
- Ensure you have Node.js installed. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the Axios library for making HTTP requests by running
npm install axios
.
Writing JavaScript Code to Fetch Page Views from Pendo
With your environment set up, you can now write the JavaScript code to make an API call to Pendo and retrieve page view data. Follow these steps:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://engageapi.pendo.io/api/v1/pageViews';
const headers = {
'Authorization': 'Bearer Your_API_Key',
'Content-Type': 'application/json'
};
// Function to get page views
async function getPageViews() {
try {
const response = await axios.get(endpoint, { headers });
const data = response.data;
// Process and display the page view data
console.log('Page Views:', data);
} catch (error) {
console.error('Error fetching page views:', error.response ? error.response.data : error.message);
}
}
// Call the function
getPageViews();
Replace Your_API_Key
with the API key you generated from your Pendo account. This code uses Axios to send a GET request to the Pendo API endpoint for page views. If successful, it logs the page view data to the console.
Verifying API Call Success and Handling Errors
After running your code, check the console output to verify that the page view data is retrieved successfully. If the request fails, the error message will be logged, helping you diagnose the issue.
Common error codes include:
- 401 Unauthorized: Check if your API key is correct and has the necessary permissions.
- 404 Not Found: Ensure the endpoint URL is correct.
- 500 Internal Server Error: This might be an issue on Pendo's end; try again later.
For more detailed information on error codes, refer to the Pendo API documentation.
Best Practices for Using the Pendo API in JavaScript
When integrating with the Pendo API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key considerations:
- Securely Store API Keys: Always store your API keys securely, such as in environment variables, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by Pendo. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Standardize and transform the retrieved data as needed to fit your application's requirements, ensuring consistency across your systems.
Enhance Your Integration Experience with Endgrate
Building and maintaining multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Pendo. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing their overall satisfaction.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?