Using the Intercom API to Create Notes (with Python examples)
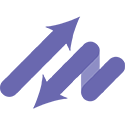
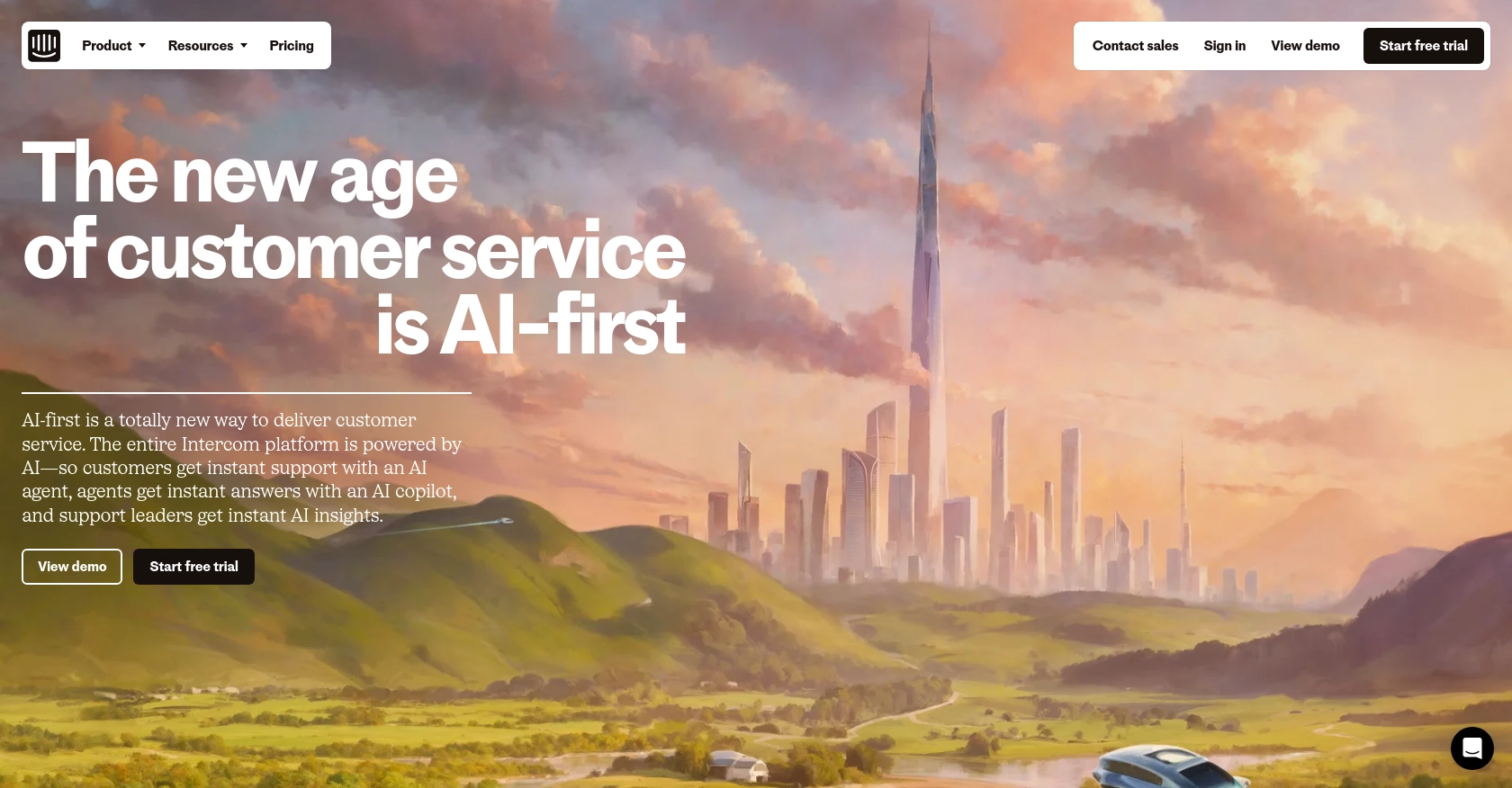
Introduction to Intercom API Integration
Intercom is a powerful customer communication platform that enables businesses to connect with their users through messaging, email, and more. It offers a suite of tools designed to enhance customer engagement, support, and retention.
Developers may want to integrate with Intercom's API to automate and streamline customer interactions. For example, using the Intercom API, a developer can create notes on customer profiles to keep track of interactions and provide personalized support. This can be particularly useful for customer service teams looking to maintain a comprehensive history of customer communications.
Setting Up Your Intercom Test Account for API Integration
Before you can start using the Intercom API to create notes, you'll need to set up a test account. This involves creating a development workspace and configuring OAuth for authentication. Follow these steps to get started:
Create a Development Workspace on Intercom
To begin, you'll need a development workspace on Intercom. This workspace allows you to test your integration without affecting live data. Here's how to set it up:
- Visit the Intercom Developer Hub and sign up for a free account if you haven't already.
- Once logged in, navigate to the Developer Hub and create a new app. Make sure it's associated with your main Intercom workspace.
- Follow the prompts to set up your development workspace. This will give you access to necessary tools and resources for building your integration.
Configure OAuth for Secure Authentication
Intercom uses OAuth for secure authentication, allowing your app to access user data. Follow these steps to configure OAuth:
- In the Developer Hub, go to the Authentication section and enable the "Use OAuth" option.
- Provide the necessary information, including Redirect URLs, which must use HTTPS for secure communication.
- Select the permissions your app requires. For creating notes, ensure you have the appropriate scopes for accessing contact and note data.
- Save your settings to generate your
client_id
andclient_secret
, which you'll use to obtain an access token.
For detailed instructions, refer to the Intercom OAuth setup guide.
Generate an Access Token
With OAuth configured, you can now generate an access token to authenticate API requests:
- Redirect users to the following URL to obtain an authorization code:
https://app.intercom.com/oauth?client_id=YOUR_CLIENT_ID&state=YOUR_STATE
. - Once the user authorizes the app, they'll be redirected to your specified URL with a code parameter.
- Exchange this code for an access token by making a POST request to
https://api.intercom.io/auth/eagle/token
with yourclient_id
,client_secret
, and the authorization code.
For more details, see the Intercom API documentation.
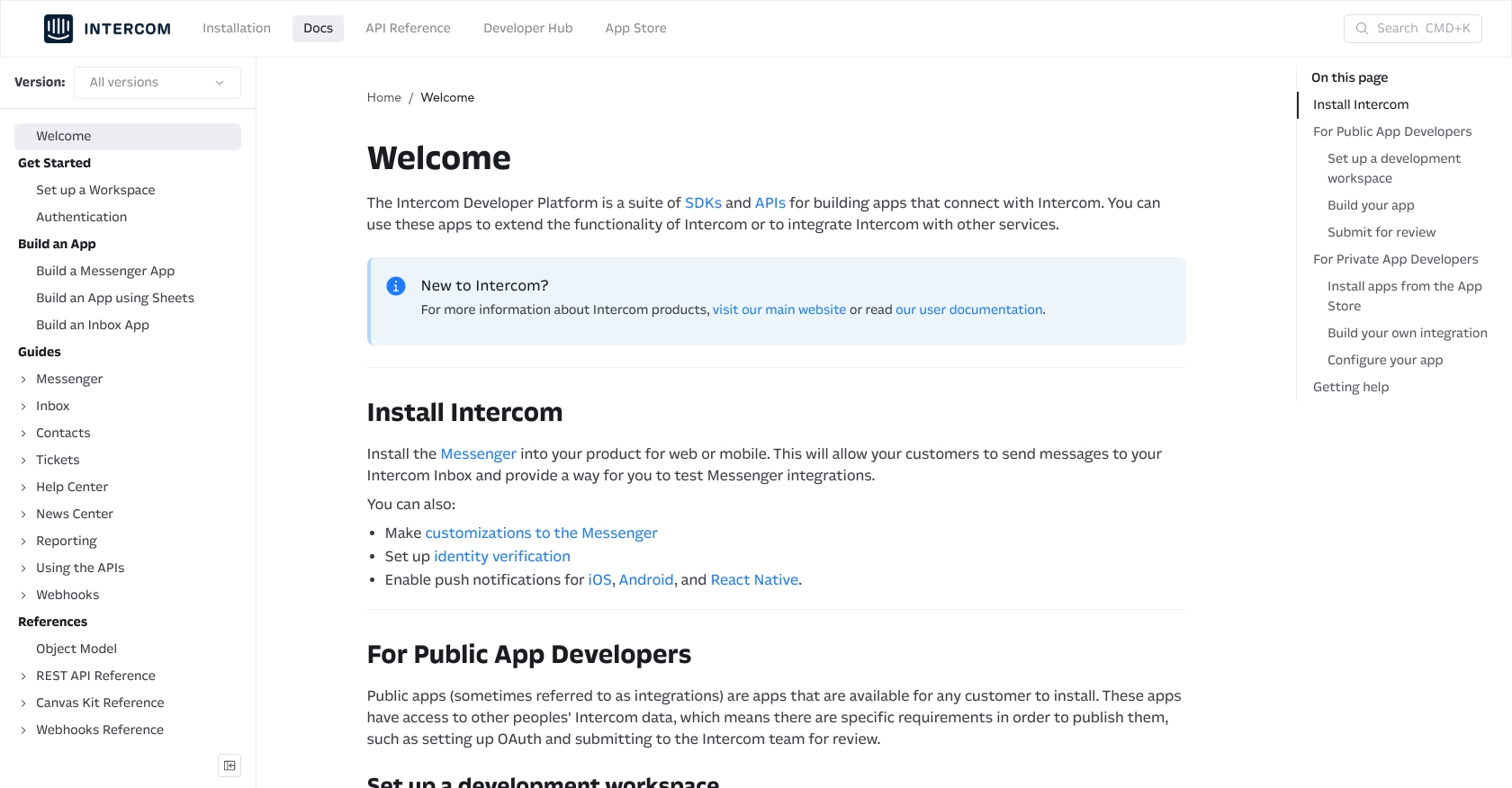
sbb-itb-96038d7
Making API Calls to Create Notes with Intercom API Using Python
Now that you have set up your Intercom development workspace and configured OAuth, you can proceed to make API calls to create notes on customer profiles using Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Intercom API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer,
pip
Next, install the requests
library, which will be used to make HTTP requests to the Intercom API:
pip install requests
Writing Python Code to Create Notes with Intercom API
With your environment set up, you can now write the Python code to create notes. Create a file named create_intercom_note.py
and add the following code:
import requests
# Set the API endpoint
url = "https://api.intercom.io/contacts/{contact_id}/notes"
# Set the request headers
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json",
"Intercom-Version": "2.11"
}
# Set the note data
note_data = {
"contact_id": "CONTACT_ID",
"admin_id": "ADMIN_ID",
"body": "This is a note created via the Intercom API."
}
# Make the POST request to create a note
response = requests.post(url, json=note_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Note created successfully:", response.json())
else:
print("Failed to create note:", response.status_code, response.text)
Replace YOUR_ACCESS_TOKEN
, CONTACT_ID
, and ADMIN_ID
with your actual access token, contact ID, and admin ID, respectively.
Verifying Successful Note Creation in Intercom
After running the script, you should see a success message if the note was created successfully. To verify, log in to your Intercom dashboard and check the contact profile to see the newly created note.
Handling Errors and Common Error Codes in Intercom API
When making API calls, it's essential to handle potential errors. Common error codes you might encounter include:
- 401 Unauthorized: Ensure your access token is valid and has the necessary permissions.
- 404 Not Found: Verify that the contact ID and admin ID are correct.
- 400 Bad Request: Check the request payload for any missing or incorrect fields.
For more detailed error handling, refer to the Intercom API documentation.
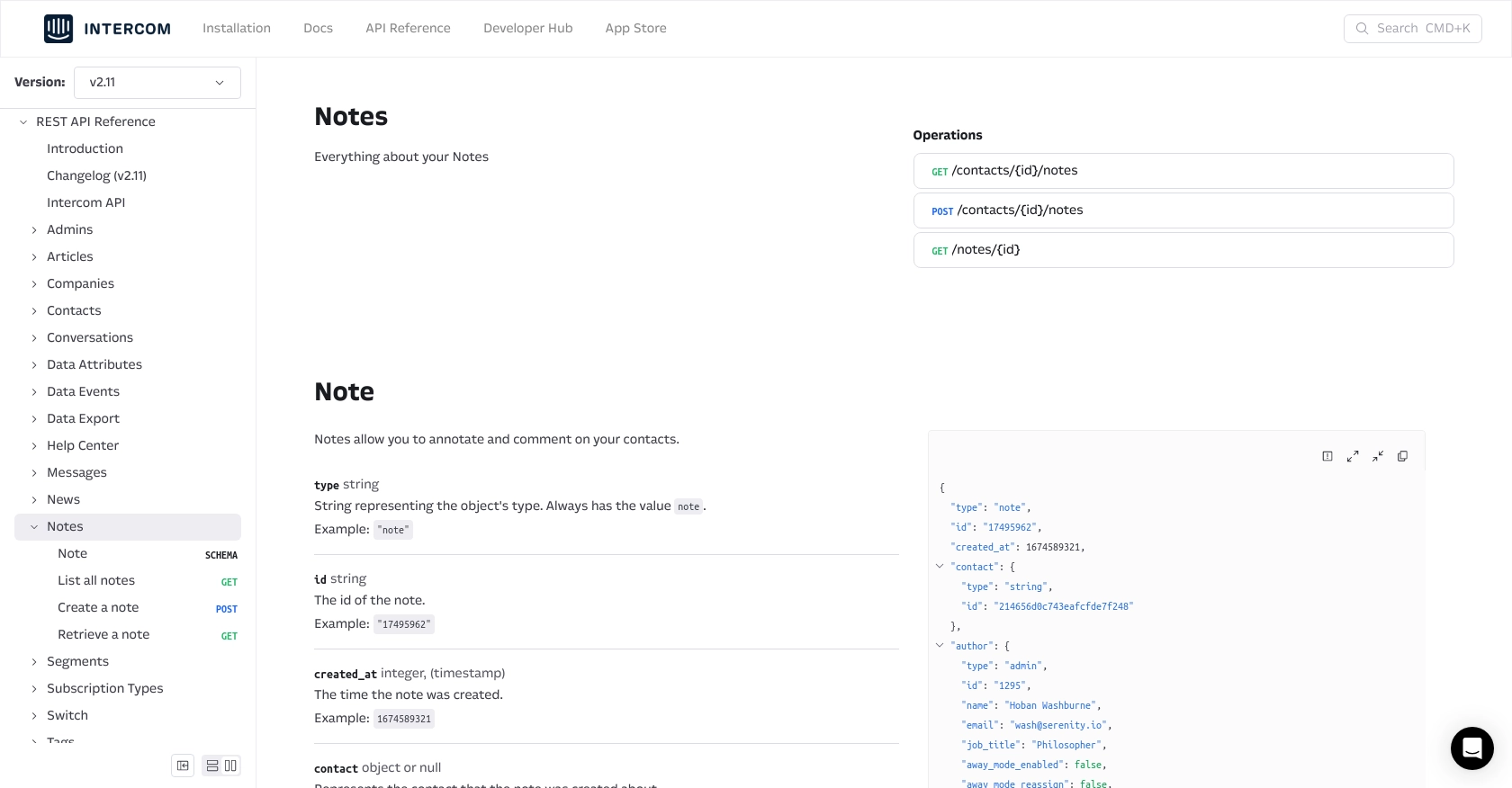
Conclusion and Best Practices for Intercom API Integration
Integrating with the Intercom API to create notes can significantly enhance your customer interaction capabilities, allowing for more personalized and efficient communication. By following the steps outlined in this guide, you can seamlessly set up your development environment, authenticate using OAuth, and execute API calls using Python.
Best Practices for Secure and Efficient API Usage
- Securely Store Credentials: Always store your
client_id
,client_secret
, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information. - Handle Rate Limiting: Be aware of Intercom's API rate limits to avoid service interruptions. Implement retry logic and exponential backoff strategies to manage request limits effectively.
- Data Standardization: Ensure that the data you send and receive is standardized to maintain consistency across your applications. This includes formatting dates, names, and other fields according to your business requirements.
Streamlining Integrations with Endgrate
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Intercom. This allows you to focus on your core product while outsourcing the integration process.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate.
Read More
Ready to get started?