How to Create Tasks with the Sugar Market API in PHP
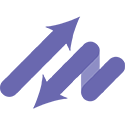
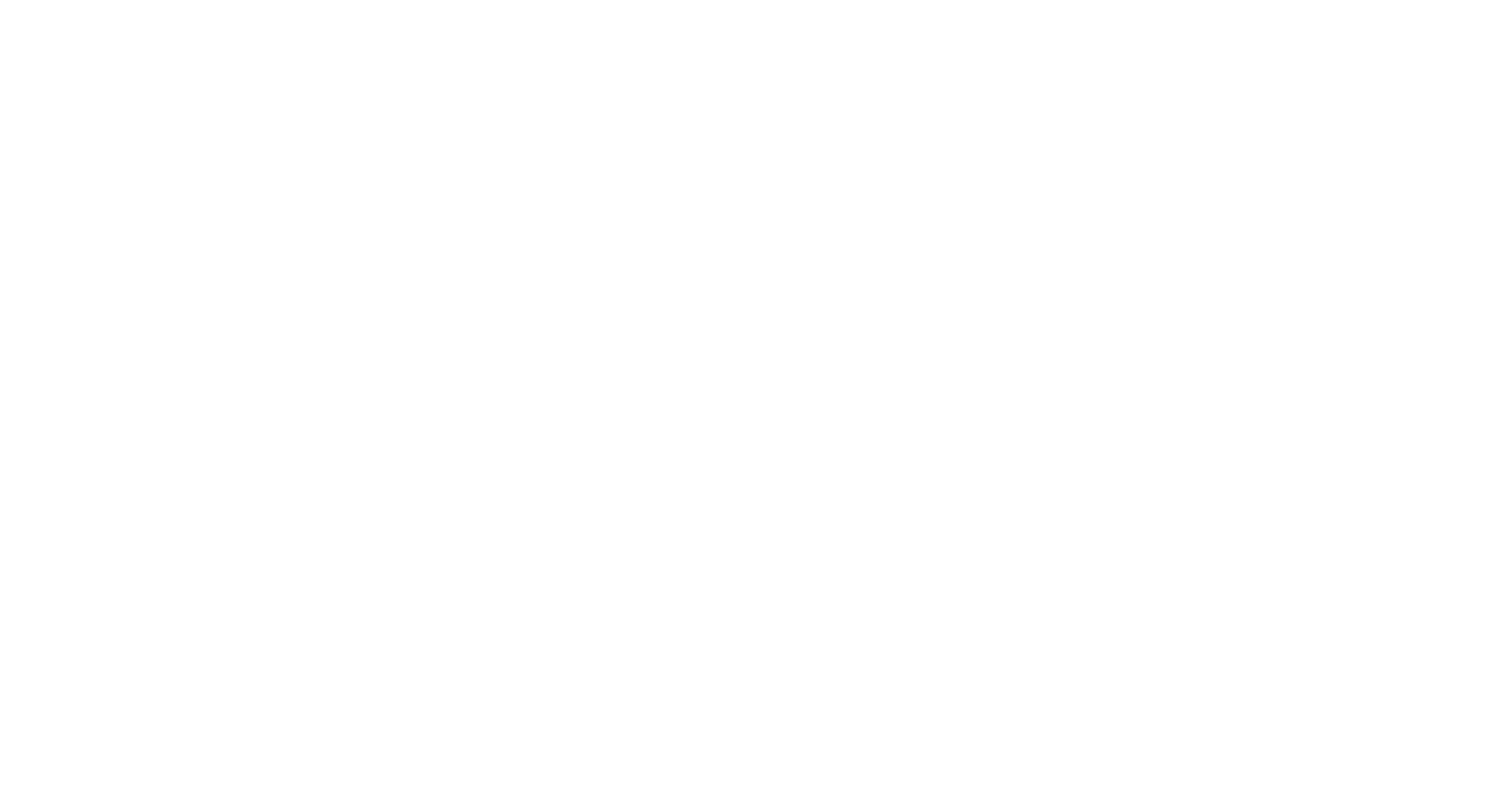
Introduction to Sugar Market
Sugar Market is a powerful marketing automation platform designed to help businesses streamline their marketing efforts and drive growth. With features like email marketing, lead nurturing, and analytics, Sugar Market provides a comprehensive solution for managing marketing campaigns and engaging with customers effectively.
Developers may want to integrate with the Sugar Market API to automate and enhance marketing workflows. For example, creating tasks programmatically can help teams manage marketing activities more efficiently, ensuring that no critical tasks are overlooked. By leveraging the Sugar Market API, developers can seamlessly integrate task creation into their existing systems, optimizing productivity and collaboration.
Setting Up a Sugar Market Test/Sandbox Account for API Integration
Before you can start creating tasks with the Sugar Market API in PHP, you need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or demo account on the Sugar Market website. This will give you access to the platform's features and allow you to test API integrations.
- Visit the Sugar Market website and navigate to the sign-up page.
- Follow the instructions to create your account. You'll need to provide some basic information, such as your name, email address, and company details.
- Once your account is created, log in to access the Sugar Market dashboard.
Generating API Credentials for Sugar Market
To interact with the Sugar Market API, you'll need to generate API credentials. These credentials will authenticate your requests and allow you to access the API securely.
- Log in to your Sugar Market account and navigate to the API settings section.
- Follow the instructions to create a new API application. You'll need to provide a name and description for your application.
- Once your application is created, you'll receive a client ID and client secret. Keep these credentials secure, as you'll need them to authenticate your API requests.
Understanding Custom Authentication for Sugar Market API
The Sugar Market API uses a custom authentication method. Ensure you follow the specific guidelines provided in the Sugar Market API documentation to authenticate your requests properly.
Typically, this involves including your client ID and client secret in the headers of your API requests. Make sure to review the documentation for any additional authentication requirements or updates.
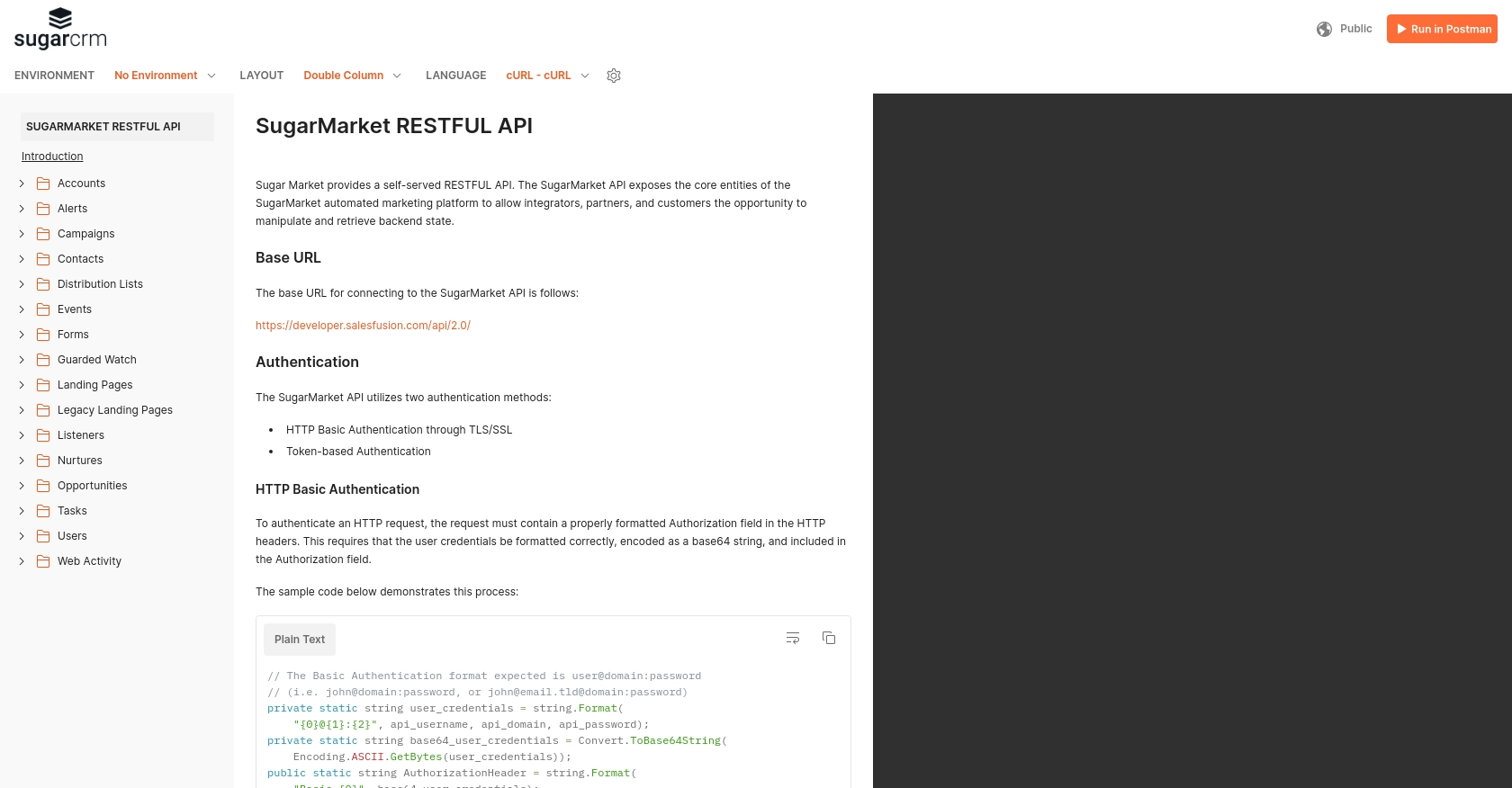
sbb-itb-96038d7
Making API Calls to Create Tasks with Sugar Market API in PHP
To create tasks using the Sugar Market API in PHP, you need to set up your development environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up PHP, installing dependencies, and writing the code to make API calls.
Setting Up Your PHP Environment for Sugar Market API Integration
Before you start coding, ensure that you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, open your terminal and navigate to your project directory. Use Composer to install the Guzzle HTTP client, which will help you make HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Create Tasks with Sugar Market API
Now that your environment is set up, you can write the PHP code to create tasks using the Sugar Market API. Create a new PHP file named create_task.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle client
$client = new Client();
// Define the API endpoint and your credentials
$endpoint = 'https://api.sugarmarket.com/v1/tasks';
$clientId = 'Your_Client_ID';
$clientSecret = 'Your_Client_Secret';
// Set up the request headers
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Basic ' . base64_encode("$clientId:$clientSecret")
];
// Define the task data
$taskData = [
'title' => 'New Marketing Task',
'description' => 'Description of the task',
'due_date' => '2023-12-31'
];
// Make the POST request to create a task
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $taskData
]);
// Check the response status
if ($response->getStatusCode() == 201) {
echo "Task created successfully!";
} else {
echo "Failed to create task. Status code: " . $response->getStatusCode();
}
Replace Your_Client_ID
and Your_Client_Secret
with the credentials you obtained from the Sugar Market API settings.
Verifying Successful Task Creation in Sugar Market
After running the script, you should see a message indicating whether the task was created successfully. To verify, log in to your Sugar Market account and check the tasks section to see if the new task appears.
Handling Errors and Troubleshooting API Requests
If you encounter errors, check the response status code and message. Common HTTP status codes include:
- 400 Bad Request: The request was invalid. Check your request data and headers.
- 401 Unauthorized: Authentication failed. Verify your client ID and secret.
- 500 Internal Server Error: An error occurred on the server. Try again later or contact support.
For more detailed error information, refer to the Sugar Market API documentation.
Best Practices for Using Sugar Market API in PHP
When working with the Sugar Market API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store API Credentials: Always store your client ID and client secret securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data you send and receive is consistent with your application's data structures. This will help maintain data integrity across systems.
Enhance Your Integration Experience with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sugar Market.
By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration for multiple platforms, reducing development overhead.
- Offer a Seamless Experience: Provide your customers with an intuitive integration experience without the hassle of managing multiple APIs.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?