How to Get Payments with the Chargebee API in PHP
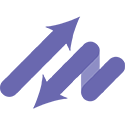
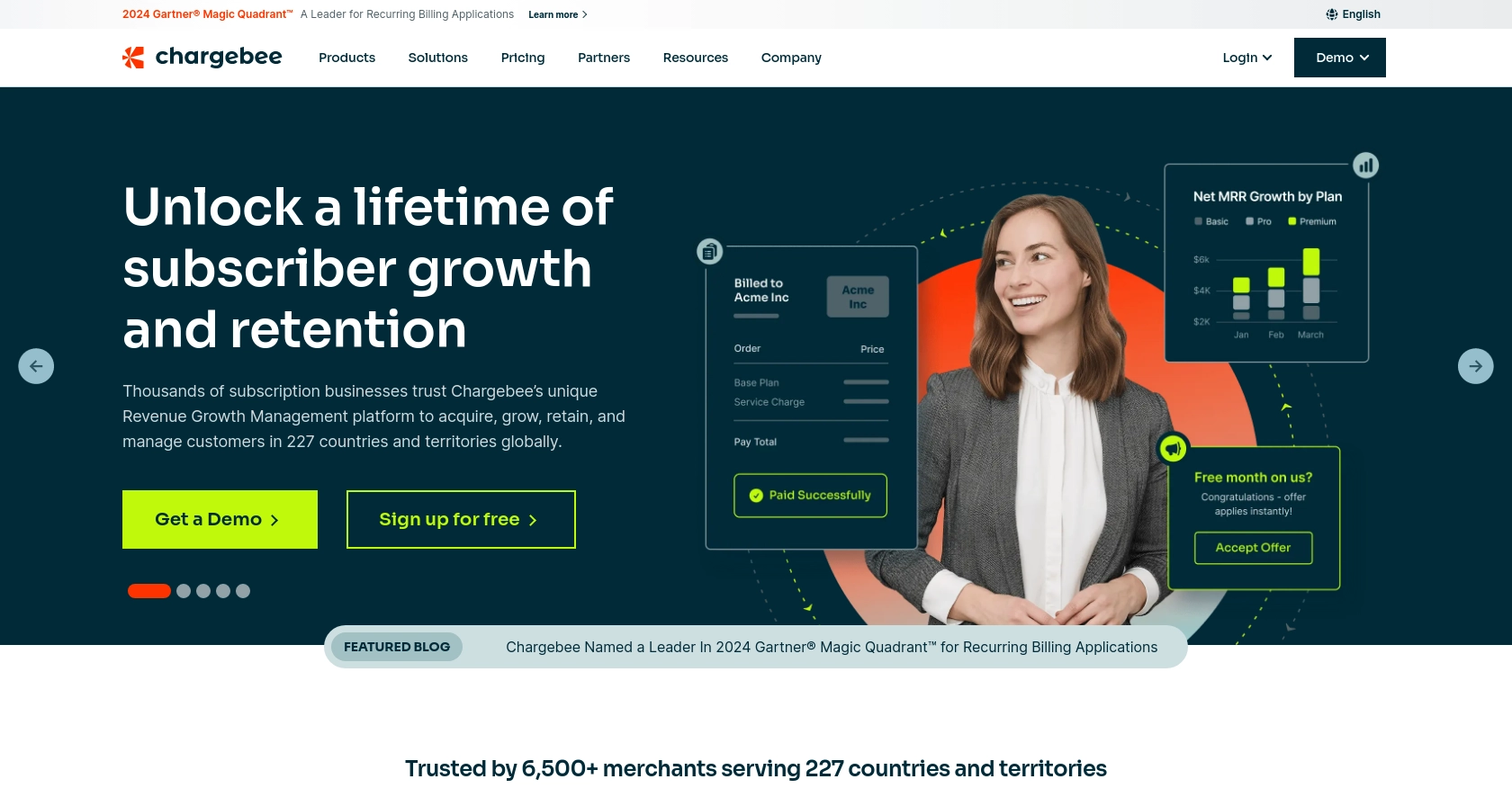
Introduction to Chargebee and Its API for Payment Processing
Chargebee is a robust subscription billing and revenue management platform designed to simplify the complexities of recurring billing for businesses. It offers a comprehensive suite of tools to manage subscriptions, invoicing, payments, and more, making it a preferred choice for SaaS companies and other subscription-based businesses.
Developers may want to integrate with Chargebee's API to streamline payment processing and automate financial operations. By connecting with Chargebee, you can efficiently manage transactions, handle refunds, and generate invoices, all while maintaining a seamless user experience.
For example, a developer might use the Chargebee API to retrieve payment transactions and display them in a custom dashboard, providing real-time insights into the business's financial health.
Setting Up Your Chargebee Test/Sandbox Account for API Integration
Before you can start interacting with the Chargebee API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data, making it ideal for development and testing purposes.
Creating a Chargebee Test Account
If you don't already have a Chargebee account, follow these steps to create a test account:
- Visit the Chargebee signup page and register for a free trial account.
- Once registered, log in to your Chargebee dashboard.
- Navigate to the "Test Site" section to access your sandbox environment.
Generating API Keys for Chargebee Authentication
Chargebee uses HTTP Basic authentication for API calls, where the username is your API key and the password is left empty. Follow these steps to generate your API keys:
- In the Chargebee dashboard, go to Settings > API Keys.
- Click on Create a Key to generate a new API key for your test site.
- Copy the generated API key and store it securely. This key will be used for authenticating your API requests.
Note: Ensure you use the API key specific to your test site to avoid affecting live data.
Configuring Chargebee API Access in PHP
To interact with the Chargebee API using PHP, you'll need to set up your environment accordingly:
- Ensure you have PHP installed on your machine. It's recommended to use PHP 7.4 or later.
- Install the necessary PHP libraries for making HTTP requests, such as
cURL
orGuzzle
.
Example: Setting Up a Basic PHP Script for Chargebee API
Here's a simple example of how to set up a PHP script to authenticate and make a basic API call to Chargebee:
<?php
// Set your Chargebee site and API key
$site = 'your-site';
$apiKey = 'your-api-key';
// Initialize cURL session
$ch = curl_init();
// Set cURL options
curl_setopt($ch, CURLOPT_URL, "https://$site.chargebee.com/api/v2/transactions");
curl_setopt($ch, CURLOPT_USERPWD, "$apiKey:");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
echo 'Response:' . $response;
}
// Close cURL session
curl_close($ch);
?>
Replace your-site
and your-api-key
with your Chargebee site name and API key. This script initializes a cURL session, sets the necessary options for authentication, and executes a request to list transactions.
By following these steps, you can effectively set up your Chargebee test account and begin integrating with the API using PHP.
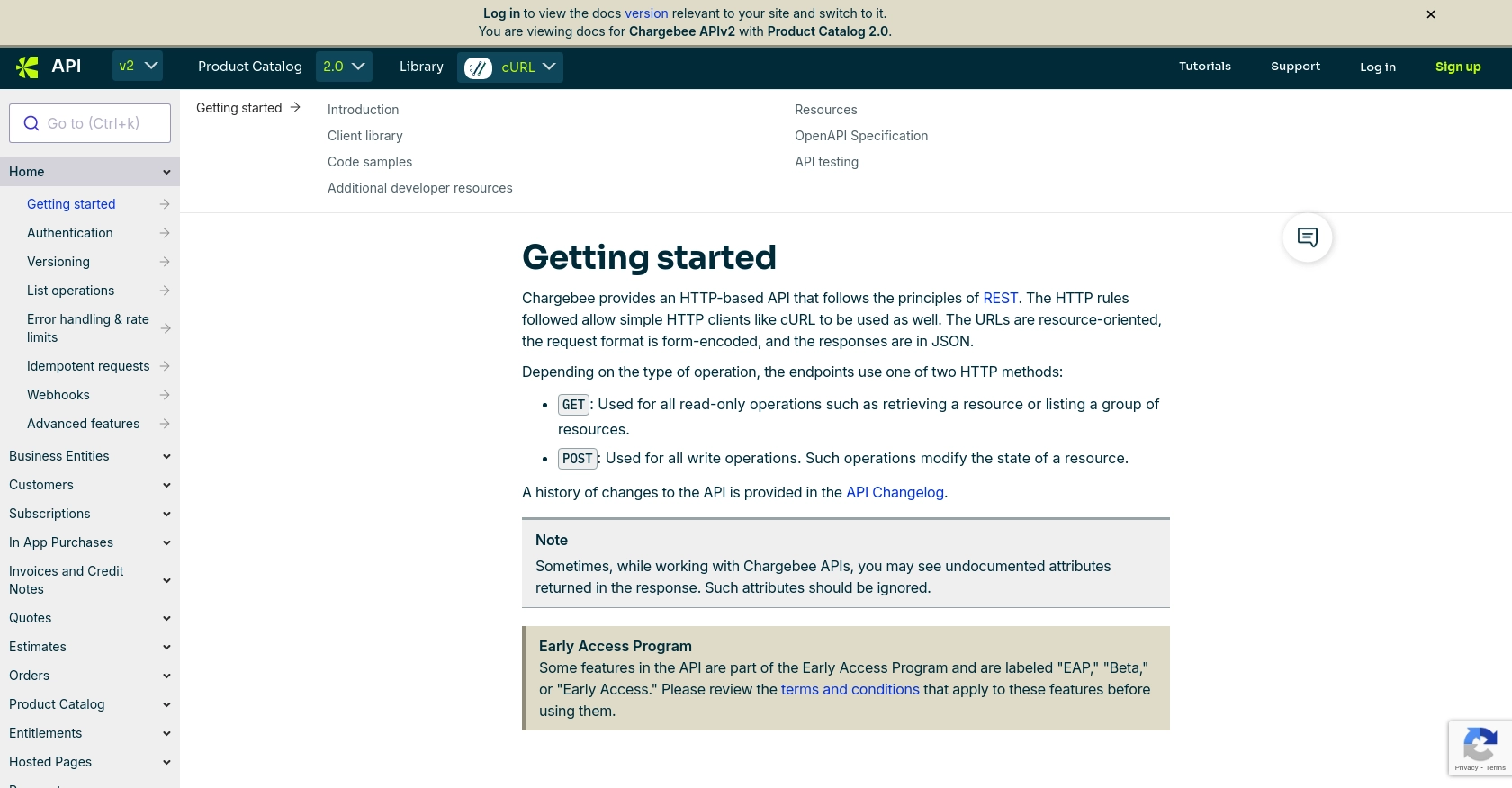
sbb-itb-96038d7
Making API Calls to Retrieve Payments with Chargebee in PHP
To interact with the Chargebee API and retrieve payment transactions using PHP, you'll need to follow a series of steps to ensure your environment is correctly set up and your API calls are properly authenticated. This section will guide you through the process of making API calls to Chargebee, handling responses, and managing potential errors.
Setting Up Your PHP Environment for Chargebee API Integration
Before making API calls, ensure your PHP environment is ready:
- Ensure PHP 7.4 or later is installed on your system.
- Install the
cURL
extension for PHP, which is essential for making HTTP requests.
Example Code: Retrieving Payment Transactions from Chargebee
Below is a PHP script example that demonstrates how to retrieve payment transactions from Chargebee:
<?php
// Set your Chargebee site and API key
$site = 'your-site';
$apiKey = 'your-api-key';
// Initialize cURL session
$ch = curl_init();
// Set cURL options for the API call
curl_setopt($ch, CURLOPT_URL, "https://$site.chargebee.com/api/v2/transactions");
curl_setopt($ch, CURLOPT_USERPWD, "$apiKey:");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPGET, true);
// Execute the request and capture the response
$response = curl_exec($ch);
// Check for errors in the cURL request
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response
$transactions = json_decode($response, true);
// Display the transactions
foreach ($transactions['list'] as $transaction) {
echo 'Transaction ID: ' . $transaction['transaction']['id'] . '<br>';
echo 'Amount: ' . $transaction['transaction']['amount'] . '<br>';
echo 'Status: ' . $transaction['transaction']['status'] . '<br><br>';
}
}
// Close cURL session
curl_close($ch);
?>
Replace your-site
and your-api-key
with your Chargebee site name and API key. This script initializes a cURL session, sets the necessary options for authentication, and executes a GET request to list transactions.
Handling API Responses and Errors
After executing the API call, it's crucial to handle the response correctly:
- Check for cURL errors using
curl_errno()
andcurl_error()
. - Decode the JSON response using
json_decode()
to access transaction details. - Display relevant transaction information, such as transaction ID, amount, and status.
For more detailed error handling, refer to the Chargebee API documentation on error codes and responses: Chargebee Error Handling.
Verifying API Call Success in Chargebee Sandbox
To ensure your API call was successful, verify the transactions in your Chargebee test/sandbox account:
- Log in to your Chargebee dashboard.
- Navigate to the "Transactions" section to view the list of transactions retrieved by your API call.
By following these steps, you can effectively retrieve payment transactions from Chargebee using PHP, ensuring a seamless integration with your application.
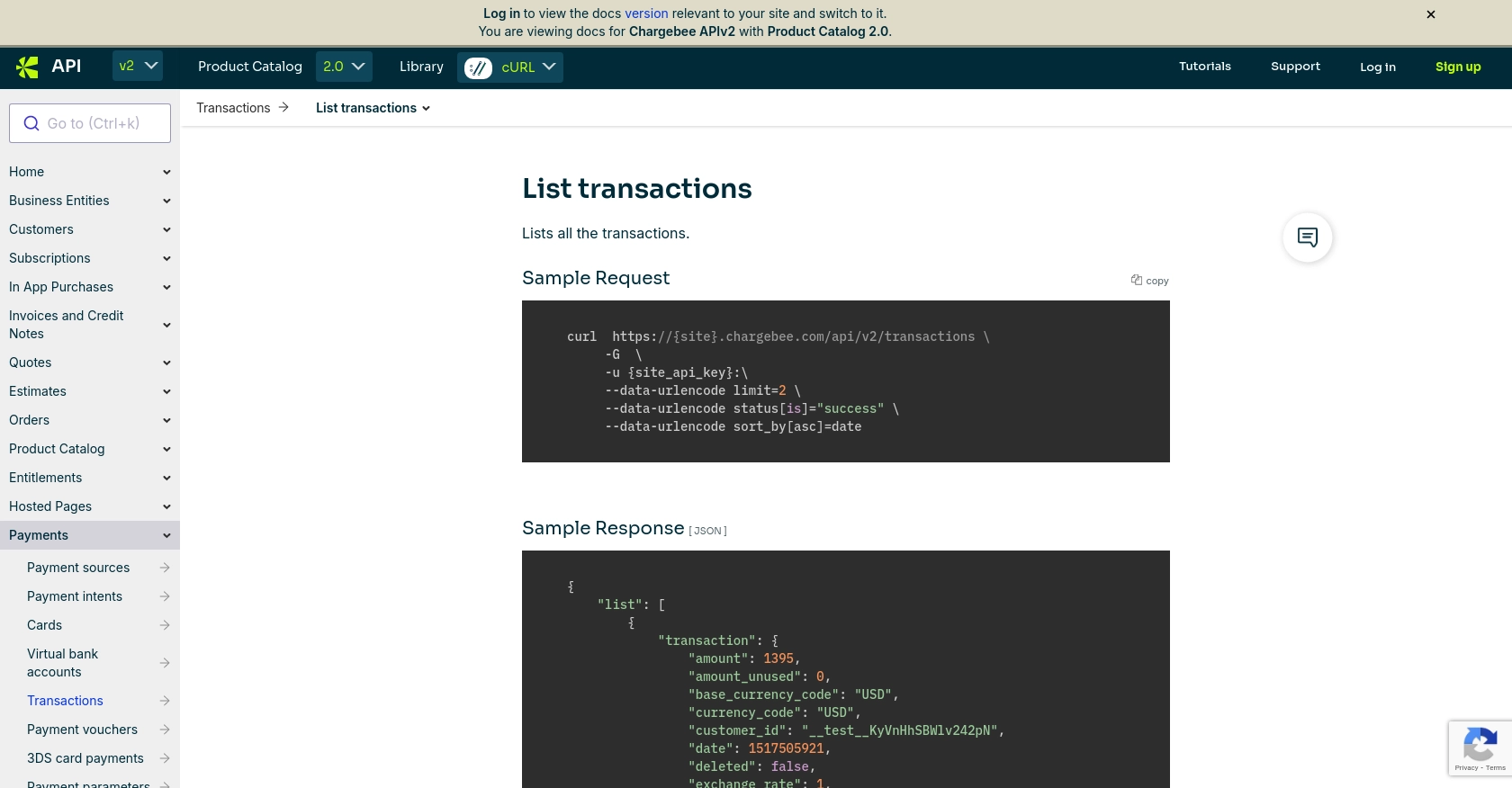
Conclusion and Best Practices for Integrating Chargebee API in PHP
Integrating with the Chargebee API using PHP offers a powerful way to manage payment transactions, automate billing processes, and enhance your application's financial operations. By following the steps outlined in this guide, you can efficiently retrieve and handle payment data, ensuring a seamless user experience.
Best Practices for Secure and Efficient Chargebee API Integration
- Secure API Keys: Store your API keys securely and avoid hardcoding them in your source files. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Chargebee imposes rate limits on API requests. Implement exponential backoff strategies to handle HTTP 429 errors gracefully. For more details, refer to the Chargebee API documentation on rate limits.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your application's data structures.
- Error Handling: Implement robust error handling by checking HTTP status codes and parsing error messages. This will help in diagnosing issues quickly and improving user experience.
Streamlining Integrations with Endgrate
While integrating with Chargebee API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Chargebee. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case, reducing the need for multiple integration efforts.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate.
By adhering to these best practices and utilizing tools like Endgrate, you can ensure a robust and efficient integration with Chargebee, driving your business's success in managing subscriptions and payments.
Read More
Ready to get started?