Using the Insightly API to Create or Update Contacts (with Javascript examples)
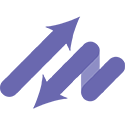
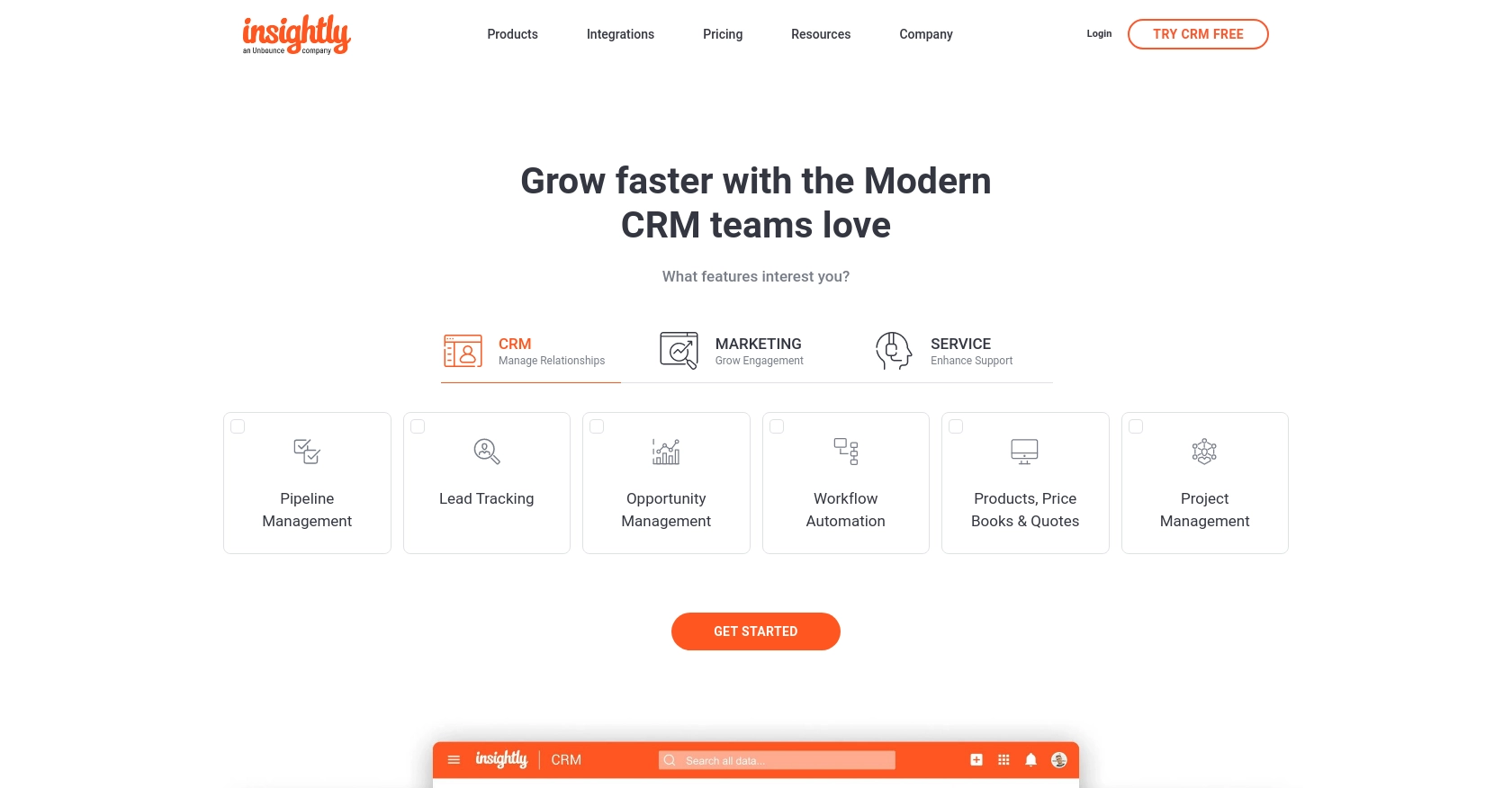
Introduction to Insightly CRM Integration
Insightly is a robust CRM platform that offers a suite of tools to help businesses manage customer relationships and streamline their sales processes. With features like contact management, project tracking, and workflow automation, Insightly is a popular choice for organizations looking to enhance their customer engagement strategies.
Integrating with the Insightly API allows developers to automate and manage contact data efficiently. For example, you can use the API to create or update contact information directly from your application, ensuring that your CRM data is always up-to-date and accurate.
This article will guide you through using JavaScript to interact with the Insightly API, focusing on creating and updating contacts. By the end of this tutorial, you'll be equipped to seamlessly integrate Insightly's powerful CRM capabilities into your own applications.
Setting Up Your Insightly API Test Account
Before you can start integrating with the Insightly API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on the Insightly website. Follow the on-screen instructions to create your account. Once your account is set up, log in to access the Insightly dashboard.
Generating Your Insightly API Key
Insightly uses HTTP Basic authentication, which requires an API key to authenticate requests. Follow these steps to obtain your API key:
- Log in to your Insightly account.
- Navigate to your user profile by clicking on your profile icon in the upper right corner.
- Select User Settings from the dropdown menu.
- In the API Key and URL section, you'll find your API key. If you need to generate a new key, click on Generate New API Key.
For more details, refer to the Insightly API key documentation.
Configuring Your API Key for Authentication
Once you have your API key, you need to configure it for use in your API requests. Insightly requires the API key to be included as the Base64-encoded username in the Authorization header, leaving the password blank. Here's how you can set it up in your JavaScript code:
// Example of setting up the Authorization header with the API key
const apiKey = 'your_api_key_here';
const encodedApiKey = btoa(apiKey + ':');
const headers = {
'Authorization': 'Basic ' + encodedApiKey,
'Content-Type': 'application/json'
};
Replace your_api_key_here
with the API key you obtained from your Insightly account.
Testing Your API Setup
With your API key configured, you can now test your setup by making a simple API call to retrieve contacts. This will ensure that your authentication is working correctly:
// Example API call to retrieve contacts
fetch('https://api.na1.insightly.com/v3.1/Contacts', {
method: 'GET',
headers: headers
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
If the request is successful, you should see a list of contacts in the console. This confirms that your API setup is correct and ready for further integration.
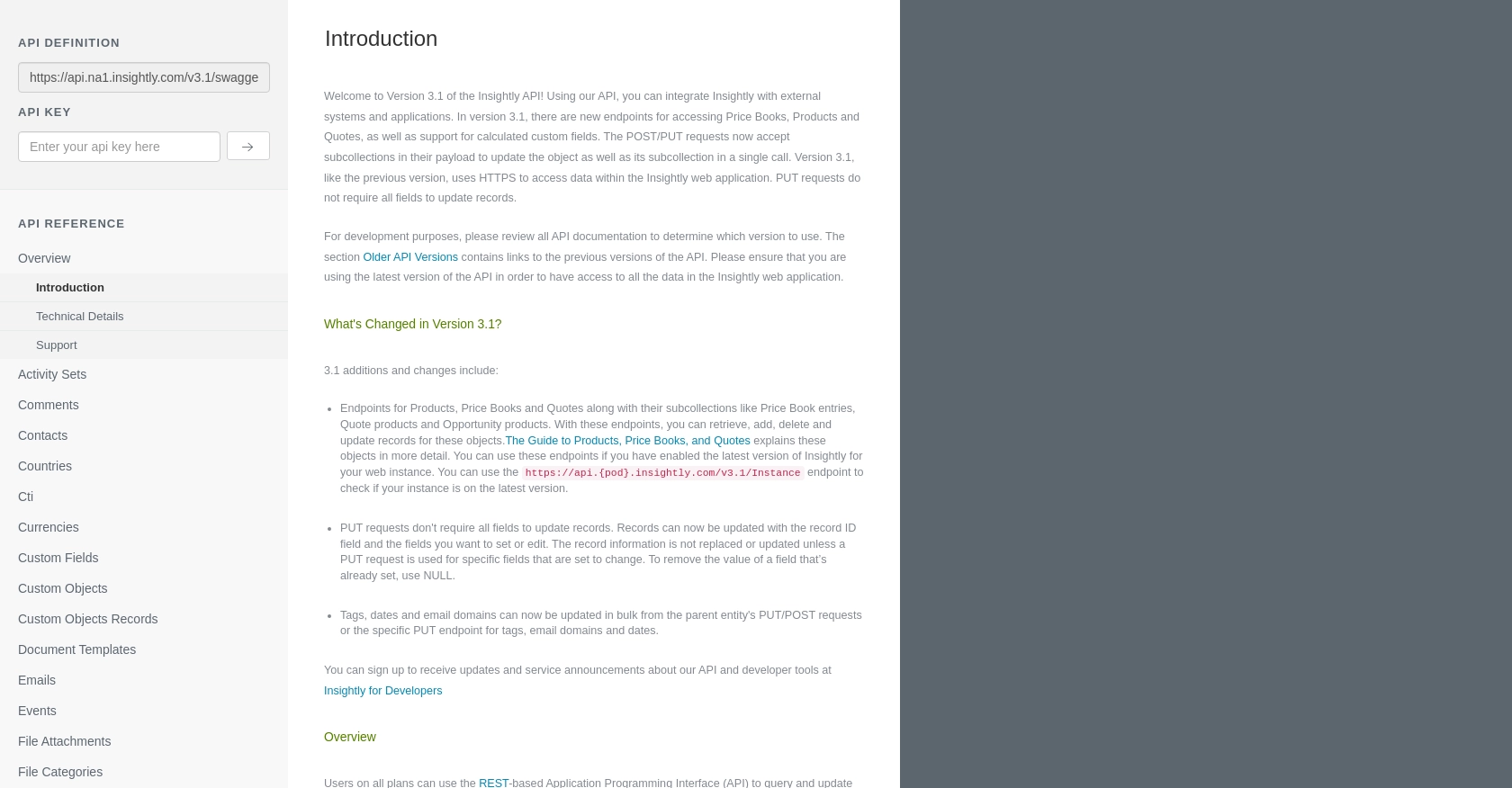
sbb-itb-96038d7
Making API Calls to Insightly for Contact Management Using JavaScript
Now that you have your Insightly API key set up, it's time to dive into making API calls to create or update contacts. This section will guide you through the process using JavaScript, ensuring you can efficiently manage contact data within your application.
Setting Up JavaScript Environment for Insightly API Integration
Before making API calls, ensure your JavaScript environment is ready. You'll need a modern browser or a Node.js setup to execute the code. Additionally, make sure you have access to the fetch
API, which is commonly available in most environments.
Creating a New Contact in Insightly Using JavaScript
To create a new contact in Insightly, you'll use a POST request. Here's a step-by-step guide:
// Define the API endpoint for creating contacts
const createContactUrl = 'https://api.na1.insightly.com/v3.1/Contacts';
// Define the contact data
const newContact = {
"FIRST_NAME": "John",
"LAST_NAME": "Doe",
"EMAIL_ADDRESS": "john.doe@example.com"
};
// Make the POST request to create a new contact
fetch(createContactUrl, {
method: 'POST',
headers: headers,
body: JSON.stringify(newContact)
})
.then(response => response.json())
.then(data => console.log('Contact Created:', data))
.catch(error => console.error('Error:', error));
Replace the contact details with the information you want to add. If successful, the response will include the newly created contact's details.
Updating an Existing Contact in Insightly Using JavaScript
To update an existing contact, you'll need the contact's ID. Use a PUT request to modify the contact information:
// Define the API endpoint for updating contacts
const updateContactUrl = 'https://api.na1.insightly.com/v3.1/Contacts/{CONTACT_ID}';
// Define the updated contact data
const updatedContact = {
"FIRST_NAME": "Jane",
"LAST_NAME": "Doe",
"EMAIL_ADDRESS": "jane.doe@example.com"
};
// Make the PUT request to update the contact
fetch(updateContactUrl.replace('{CONTACT_ID}', '123456'), {
method: 'PUT',
headers: headers,
body: JSON.stringify(updatedContact)
})
.then(response => response.json())
.then(data => console.log('Contact Updated:', data))
.catch(error => console.error('Error:', error));
Replace {CONTACT_ID}
with the actual ID of the contact you wish to update. The response will confirm the update with the contact's new details.
Handling API Response and Error Codes for Insightly
When interacting with the Insightly API, it's crucial to handle responses and potential errors effectively. Here are some tips:
- Check the HTTP status code in the response to determine success or failure.
- Insightly's API may return a
429
status code if you exceed rate limits. Refer to the Insightly API documentation for rate limit details. - Use the
catch
block to handle network errors or unexpected issues.
By following these guidelines, you can ensure robust and reliable integration with Insightly's API.
Conclusion and Best Practices for Insightly API Integration
Integrating with the Insightly API using JavaScript allows developers to efficiently manage contact data, automate workflows, and enhance CRM capabilities within their applications. By following the steps outlined in this guide, you can create and update contacts seamlessly, ensuring your CRM data remains accurate and up-to-date.
Best Practices for Secure and Efficient Insightly API Usage
- Secure API Key Storage: Always store your API key securely and avoid hardcoding it in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of Insightly's rate limits, which are 10 requests per second and vary daily based on your plan. Implement retry logic and exponential backoff strategies to handle
429
status codes gracefully. - Data Transformation: Standardize and transform data fields as needed to ensure consistency across different systems and applications.
- Error Handling: Implement robust error handling to manage network issues and API errors effectively. Use the response status codes to guide your error management strategies.
Streamlining Integrations with Endgrate
While integrating with Insightly's API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Insightly.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can manage multiple integrations efficiently, providing an intuitive experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, scalable integration solution.
Read More
Ready to get started?