How to Create or Update Customers with the Zoho Books API in Python
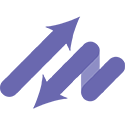
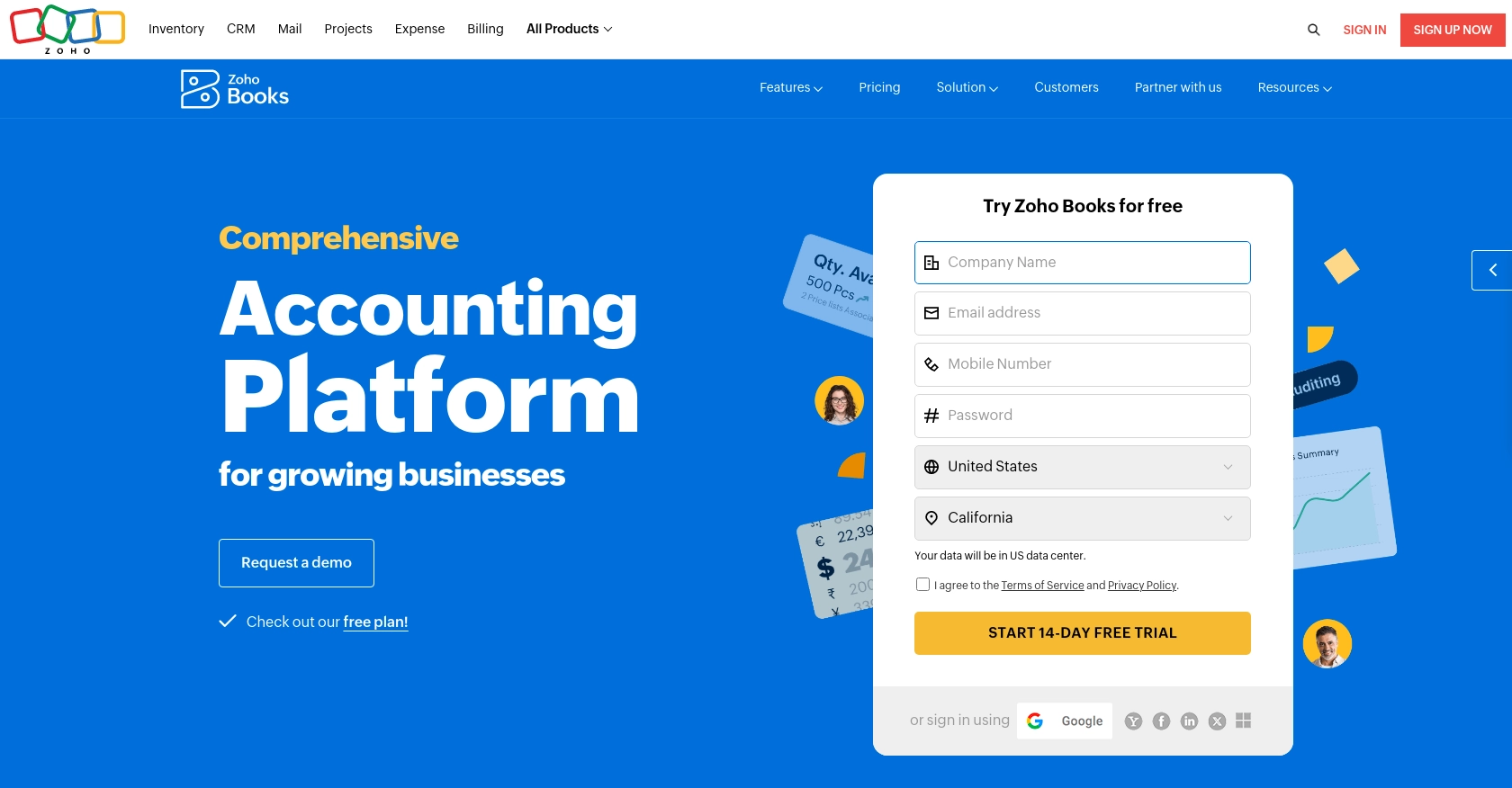
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to streamline financial operations by automating tasks such as creating or updating customer records. For example, you can use the API to automatically update customer information from your CRM system into Zoho Books, ensuring that your financial data is always up-to-date and accurate.
Setting Up Your Zoho Books Test Account for API Integration
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Step 1: Sign Up for a Zoho Books Account
- Visit the Zoho Books website and sign up for a free trial or create a demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is set up, log in to access the Zoho Books dashboard.
Step 2: Create a Zoho Books Developer App for OAuth Authentication
Zoho Books uses OAuth 2.0 for authentication, ensuring secure access to your data. Here's how to set up OAuth:
- Go to the Zoho Developer Console.
- Click on "Add Client ID" to register your application.
- Provide the necessary details, such as the app name and redirect URI, and submit the form.
- After registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are required for API access.
Step 3: Generate OAuth Tokens
To interact with the Zoho Books API, you need to generate access and refresh tokens:
- Redirect users to the following URL to obtain a grant token:
- After user consent, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for access and refresh tokens by making a POST request:
- Store the access and refresh tokens securely for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
import requests
url = "https://accounts.zoho.com/oauth/v2/token"
payload = {
'code': 'YOUR_CODE',
'client_id': 'YOUR_CLIENT_ID',
'client_secret': 'YOUR_CLIENT_SECRET',
'redirect_uri': 'YOUR_REDIRECT_URI',
'grant_type': 'authorization_code'
}
response = requests.post(url, data=payload)
tokens = response.json()
print(tokens)
With your Zoho Books test account and OAuth setup complete, you're ready to start making API calls to create or update customer records.
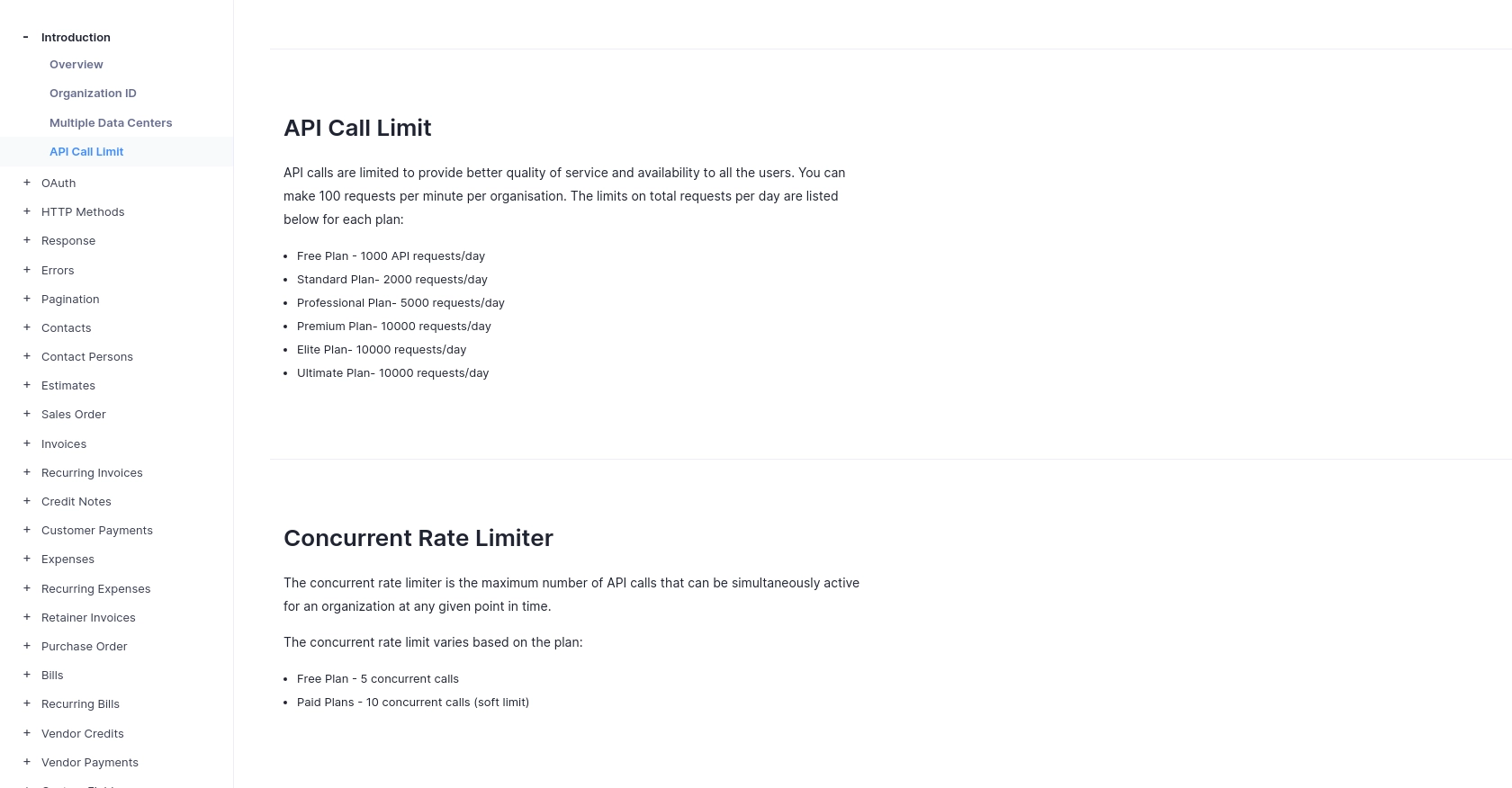
sbb-itb-96038d7
Making API Calls to Create or Update Customers in Zoho Books Using Python
With your Zoho Books test account and OAuth setup complete, you can now proceed to make API calls to create or update customer records. This section will guide you through the necessary steps and provide example code to help you interact with the Zoho Books API using Python.
Prerequisites for Zoho Books API Integration with Python
Before making API calls, ensure you have the following prerequisites:
- Python 3.11.1 installed on your machine.
- The
requests
library for handling HTTP requests. Install it using the command:
pip install requests
Creating a New Customer in Zoho Books
To create a new customer in Zoho Books, you'll need to make a POST request to the Zoho Books API. Here's a step-by-step guide:
- Set the API endpoint and headers:
- Define the customer data you want to create:
- Make the POST request to create the customer:
import requests
url = "https://www.zohoapis.com/books/v3/contacts?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
customer_data = {
"contact_name": "John Doe",
"company_name": "Doe Enterprises",
"contact_type": "customer",
"billing_address": {
"attention": "John Doe",
"address": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "12345",
"country": "USA"
}
}
response = requests.post(url, headers=headers, json=customer_data)
if response.status_code == 201:
print("Customer created successfully:", response.json())
else:
print("Failed to create customer:", response.json())
Updating an Existing Customer in Zoho Books
To update an existing customer, you'll need to make a PUT request. Here's how:
- Set the API endpoint for the specific customer you want to update:
- Define the updated customer data:
- Make the PUT request to update the customer:
contact_id = "EXISTING_CONTACT_ID"
url = f"https://www.zohoapis.com/books/v3/contacts/{contact_id}?organization_id=YOUR_ORG_ID"
updated_data = {
"contact_name": "John Doe Updated",
"company_name": "Doe Enterprises Updated"
}
response = requests.put(url, headers=headers, json=updated_data)
if response.status_code == 200:
print("Customer updated successfully:", response.json())
else:
print("Failed to update customer:", response.json())
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and errors appropriately. Zoho Books API uses standard HTTP status codes:
- 200 - OK: The request was successful.
- 201 - Created: The resource was successfully created.
- 400 - Bad Request: The request was invalid.
- 401 - Unauthorized: Authentication failed.
- 404 - Not Found: The resource was not found.
- 429 - Rate Limit Exceeded: Too many requests.
For more detailed error handling, refer to the Zoho Books API error documentation.
Verifying API Call Success in Zoho Books
To verify that your API calls have succeeded, check the Zoho Books dashboard. Newly created or updated customers should appear in your contacts list. If you encounter issues, review the API response messages for guidance.
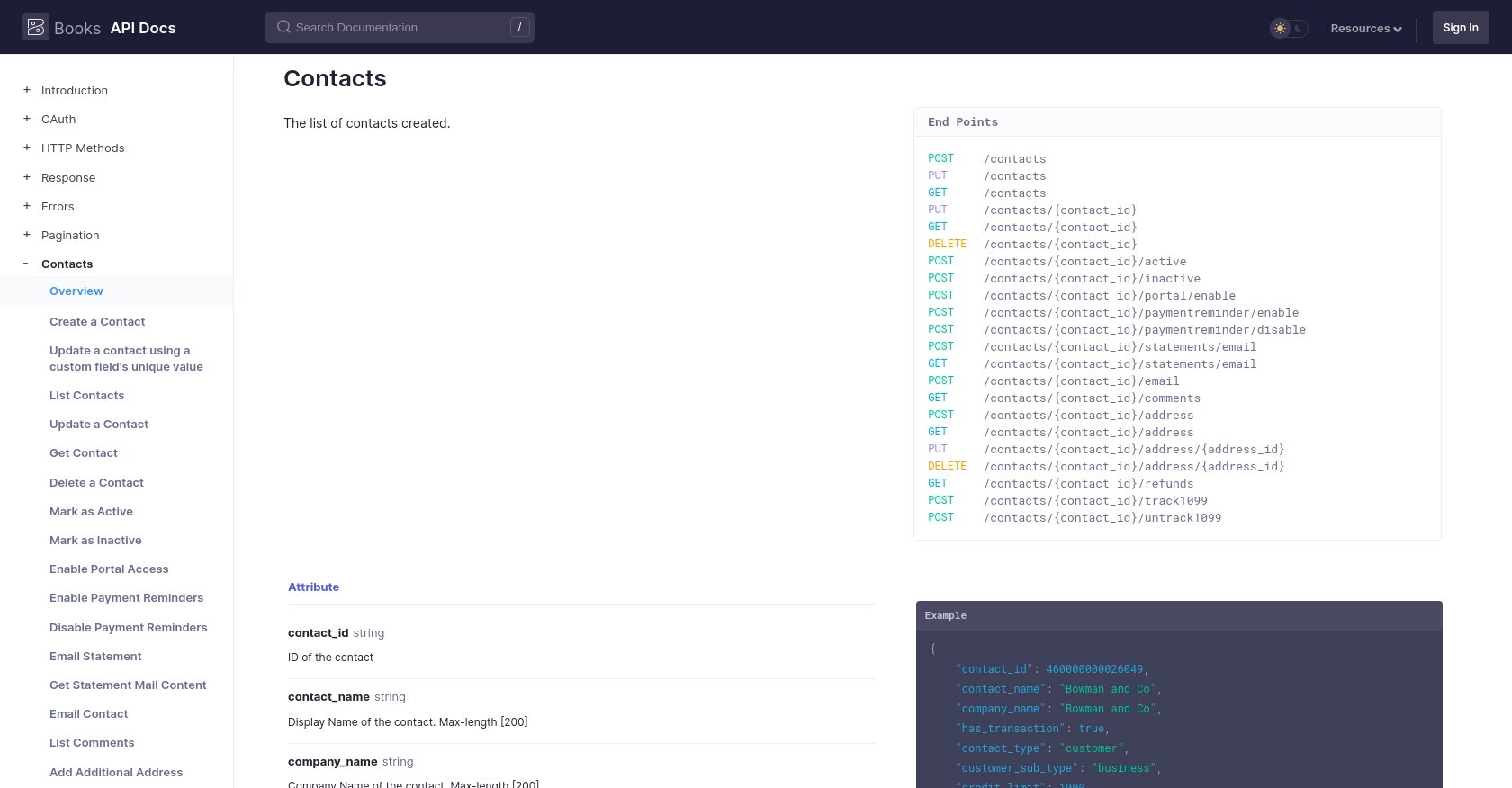
Conclusion: Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using Python can significantly streamline your financial operations by automating customer management tasks. Here are some best practices to ensure a smooth integration process:
Securely Store Zoho Books API Credentials
Always store your OAuth tokens, Client ID, and Client Secret securely. Use environment variables or secure vaults to prevent unauthorized access to your credentials.
Handle Zoho Books API Rate Limits
Zoho Books imposes rate limits to ensure fair usage. You can make up to 100 requests per minute per organization. Plan your API calls accordingly to avoid exceeding these limits. For more details, refer to the API call limit documentation.
Implement Robust Error Handling
Utilize the HTTP status codes provided by the Zoho Books API to handle errors effectively. Implement retry logic for transient errors and ensure your application can gracefully handle failures.
Data Standardization and Transformation
Ensure that the data you send to Zoho Books is standardized and transformed as needed. This includes formatting addresses, names, and other fields to match Zoho Books' requirements.
Leverage Endgrate for Efficient Integration Management
Consider using Endgrate to manage your integrations more efficiently. With Endgrate, you can save time and resources by outsourcing integration tasks, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies interactions with multiple platforms, including Zoho Books.
By following these best practices, you can ensure a successful and efficient integration with the Zoho Books API, enhancing your business's financial management capabilities.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/contacts/#overview
Ready to get started?