Using the OnePageCRM API to Get Companies in Javascript
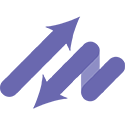
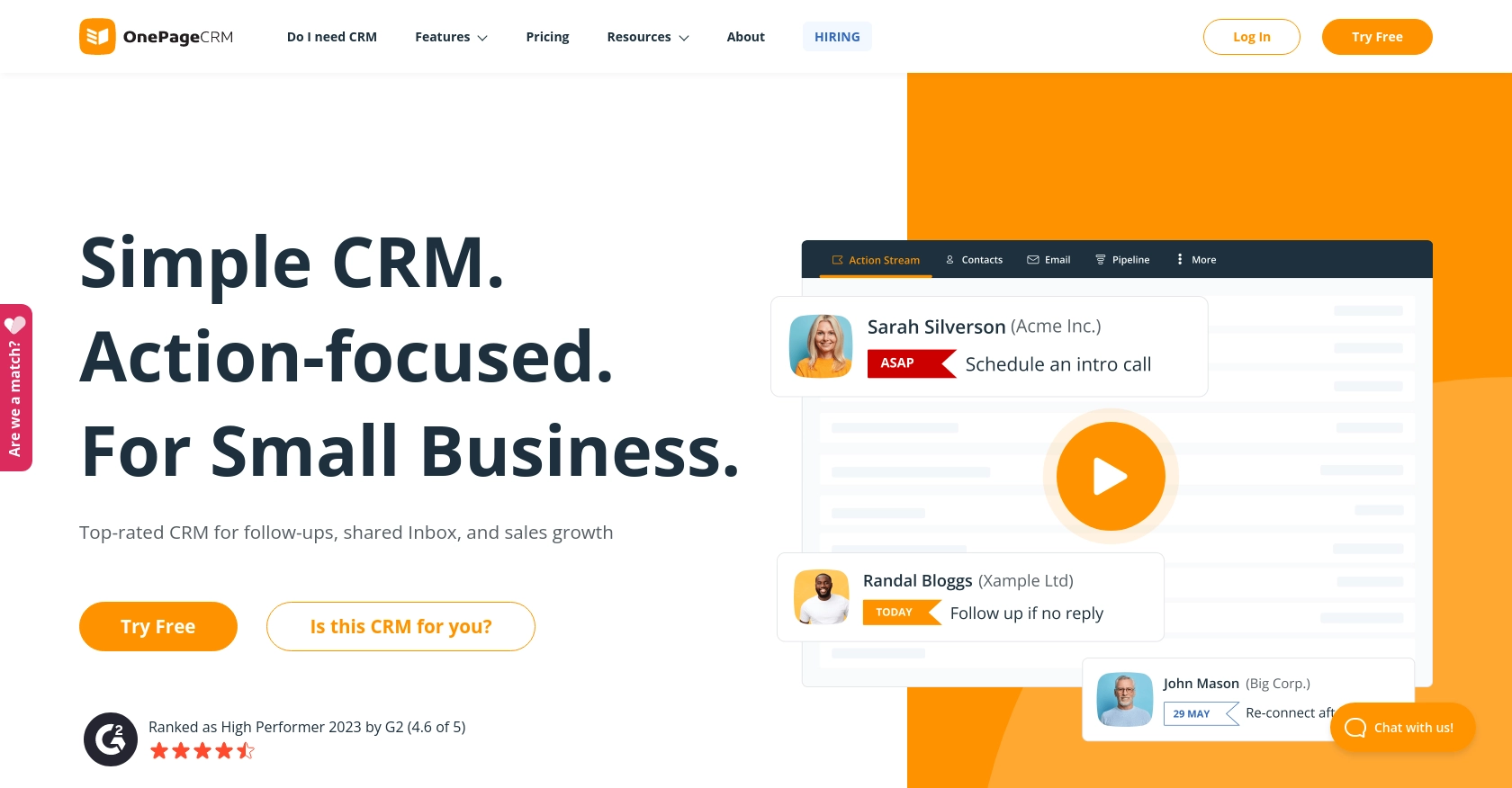
Introduction to OnePageCRM
OnePageCRM is a dynamic customer relationship management (CRM) platform designed to simplify sales processes for businesses. It offers a streamlined approach to managing contacts, tasks, and sales pipelines, making it an ideal choice for small to medium-sized enterprises looking to enhance their sales efficiency.
Integrating with the OnePageCRM API allows developers to access and manage company data programmatically. For example, a developer might use the OnePageCRM API to retrieve a list of companies, enabling seamless integration of CRM data into custom applications or dashboards. This can help automate workflows and provide real-time insights into company interactions.
Setting Up Your OnePageCRM Test Account
Before diving into the OnePageCRM API, you'll need to set up a test account to experiment with API calls. OnePageCRM offers a free trial that allows developers to explore its features without any initial cost.
Creating a OnePageCRM Free Trial Account
To get started, visit the OnePageCRM website and sign up for a free trial. Follow the on-screen instructions to create your account. Once your account is set up, you'll have access to the OnePageCRM dashboard where you can manage your CRM data.
Generating API Credentials for OnePageCRM
OnePageCRM uses a custom authentication method to secure API requests. To interact with the API, you'll need to generate API credentials.
- Log in to your OnePageCRM account.
- Navigate to the Settings section in the dashboard.
- Under API & Integrations, find the option to create a new API key.
- Follow the prompts to generate your API key and secret. Make sure to store these credentials securely, as you'll need them to authenticate your API requests.
Configuring Your Development Environment
With your API credentials ready, you can now configure your development environment to make API calls. Ensure you have a JavaScript runtime environment set up, such as Node.js, to execute your scripts.
You'll also need to install any necessary dependencies for making HTTP requests. A popular choice is the axios
library, which can be installed using npm:
npm install axios
With your OnePageCRM test account and API credentials in place, you're ready to start making API calls to retrieve company data.
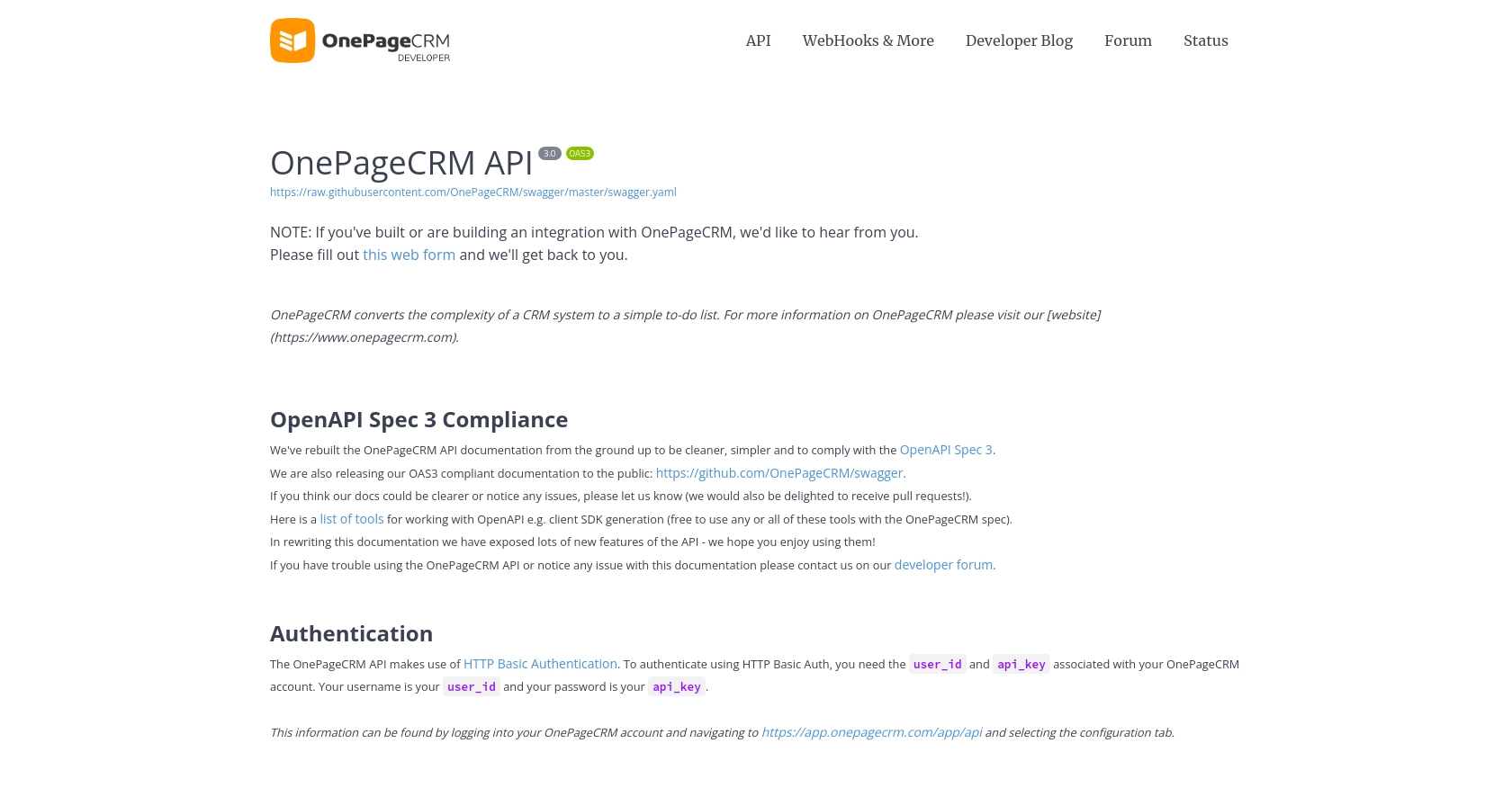
sbb-itb-96038d7
Making API Calls to Retrieve Companies from OnePageCRM Using JavaScript
With your OnePageCRM test account and API credentials set up, you can now proceed to make API calls to retrieve company data. This section will guide you through the process of using JavaScript to interact with the OnePageCRM API.
Setting Up Your JavaScript Environment for OnePageCRM API Calls
Ensure that you have Node.js installed on your machine, as it provides the runtime environment needed to execute JavaScript outside of a browser. Additionally, you'll need the axios
library to handle HTTP requests. If you haven't already installed it, run the following command:
npm install axios
Writing JavaScript Code to Retrieve Companies from OnePageCRM
Now that your environment is ready, you can write the JavaScript code to make a GET request to the OnePageCRM API to retrieve a list of companies. Create a new file named get_companies.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://app.onepagecrm.com/api/v3/companies';
const headers = {
'Authorization': 'Bearer Your_API_Key',
'Content-Type': 'application/json'
};
// Function to get companies
async function getCompanies() {
try {
const response = await axios.get(endpoint, { headers });
const companies = response.data;
console.log('Retrieved Companies:', companies);
} catch (error) {
console.error('Error fetching companies:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getCompanies();
Replace Your_API_Key
with the API key you generated earlier. This code sets up the API endpoint and headers, then makes an asynchronous GET request to retrieve the companies. If successful, it logs the list of companies to the console. In case of an error, it logs the error message.
Running the JavaScript Script to Fetch Companies
To execute the script, open your terminal and run the following command:
node get_companies.js
You should see the list of companies printed in the console, confirming that the API call was successful.
Handling Errors and Verifying API Call Success
It's important to handle potential errors when making API calls. The OnePageCRM API may return various response codes, such as 400 for a bad request or 401 for unauthorized access. Ensure your code checks for these errors and handles them gracefully.
To verify the success of your API call, you can cross-check the retrieved data with the companies listed in your OnePageCRM test account. This ensures that the data is accurate and up-to-date.
Conclusion and Best Practices for Using OnePageCRM API with JavaScript
Integrating with the OnePageCRM API using JavaScript allows developers to efficiently manage and retrieve company data, enhancing the functionality of custom applications and dashboards. By following the steps outlined in this guide, you can seamlessly connect to OnePageCRM and automate workflows to gain real-time insights into your CRM data.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Credentials: Always store your API key and secret securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of any rate limits imposed by the OnePageCRM API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized to fit your application's requirements. This helps maintain data consistency across different systems.
- Error Handling: Implement robust error handling to manage different response codes effectively. This ensures that your application can recover gracefully from unexpected issues.
Streamlining Integrations with Endgrate
While integrating with OnePageCRM is straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including OnePageCRM. By using Endgrate, you can save time and resources, allowing your team to focus on core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?