How to Get Calls with the Salesloft API in Python
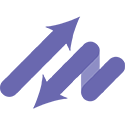
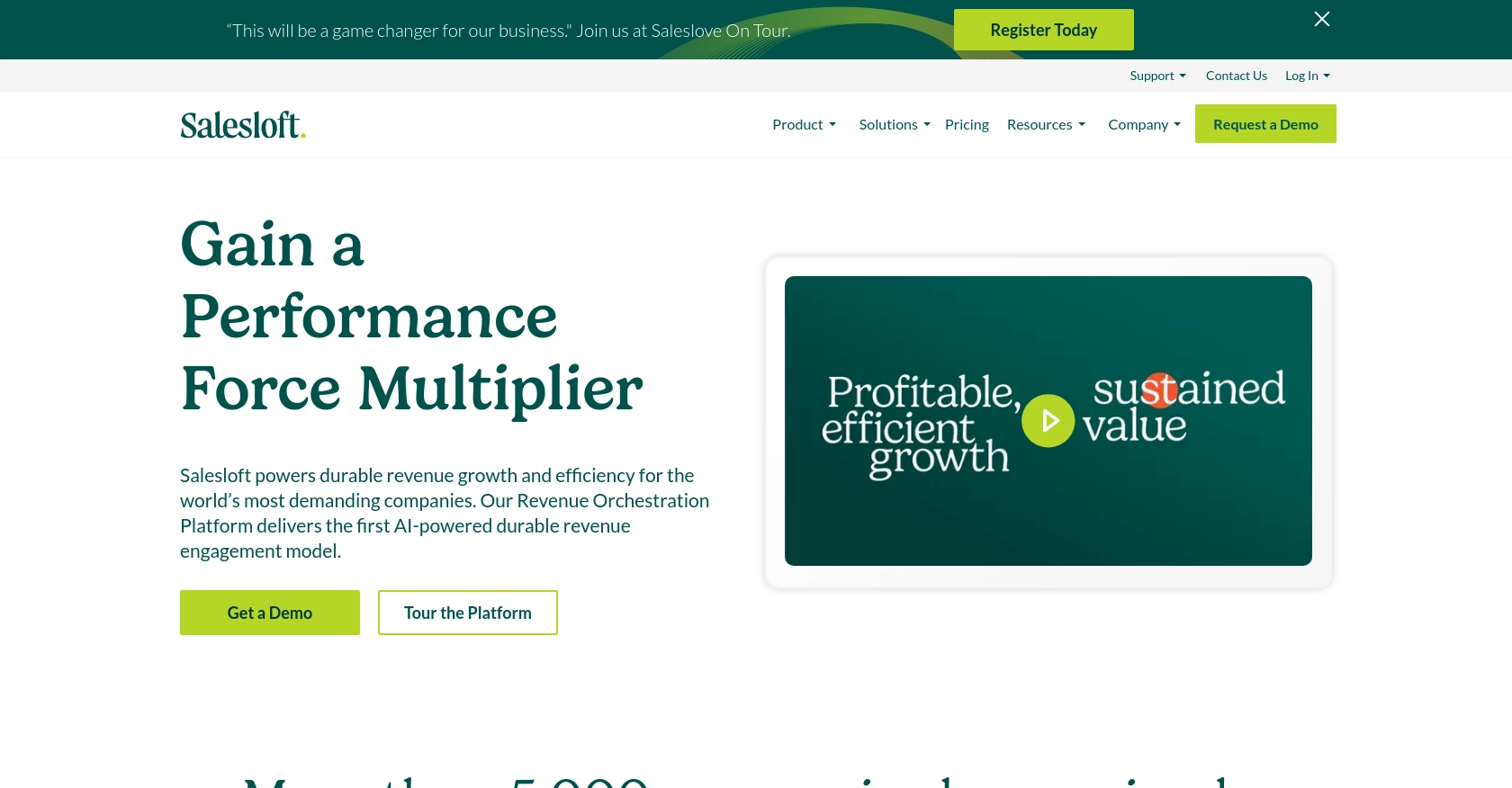
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform designed to enhance the efficiency and effectiveness of sales teams. It offers a suite of tools that streamline communication, automate tasks, and provide valuable insights into sales activities.
Integrating with the Salesloft API allows developers to access and manage call data, which can be crucial for analyzing sales performance and improving customer interactions. For example, a developer might use the Salesloft API to retrieve call records and analyze call durations and outcomes to optimize sales strategies.
Setting Up Your Salesloft Test/Sandbox Account
Before you can start interacting with the Salesloft API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial or demo account on the Salesloft website. Follow the instructions to create your account and log in.
Creating a Salesloft OAuth App for API Access
Since the Salesloft API uses OAuth 2.0 for authentication, you'll need to create an OAuth app to obtain the necessary credentials.
- Navigate to Your Applications in your Salesloft account.
- Select OAuth Applications and click on Create New.
- Fill in the required fields and click Save.
- After saving, you'll receive your Application Id (Client Id), Secret (Client Secret), and Redirect URI (Callback URL). Keep these credentials secure as they will be used for authorization.
Obtaining Authorization Code and Access Tokens
To interact with the Salesloft API, you'll need to obtain an access token using the authorization code grant type.
- Generate a request to the authorization endpoint using your Client Id and Redirect URI:
- Authorize the application when prompted. Upon approval, you'll receive a code in the redirect URI.
- Exchange this code for an access token by making a POST request:
- Store the access_token and refresh_token from the response securely for future API calls.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "YOUR_AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
With your Salesloft sandbox account and OAuth app set up, you're ready to start making API calls to retrieve call data.
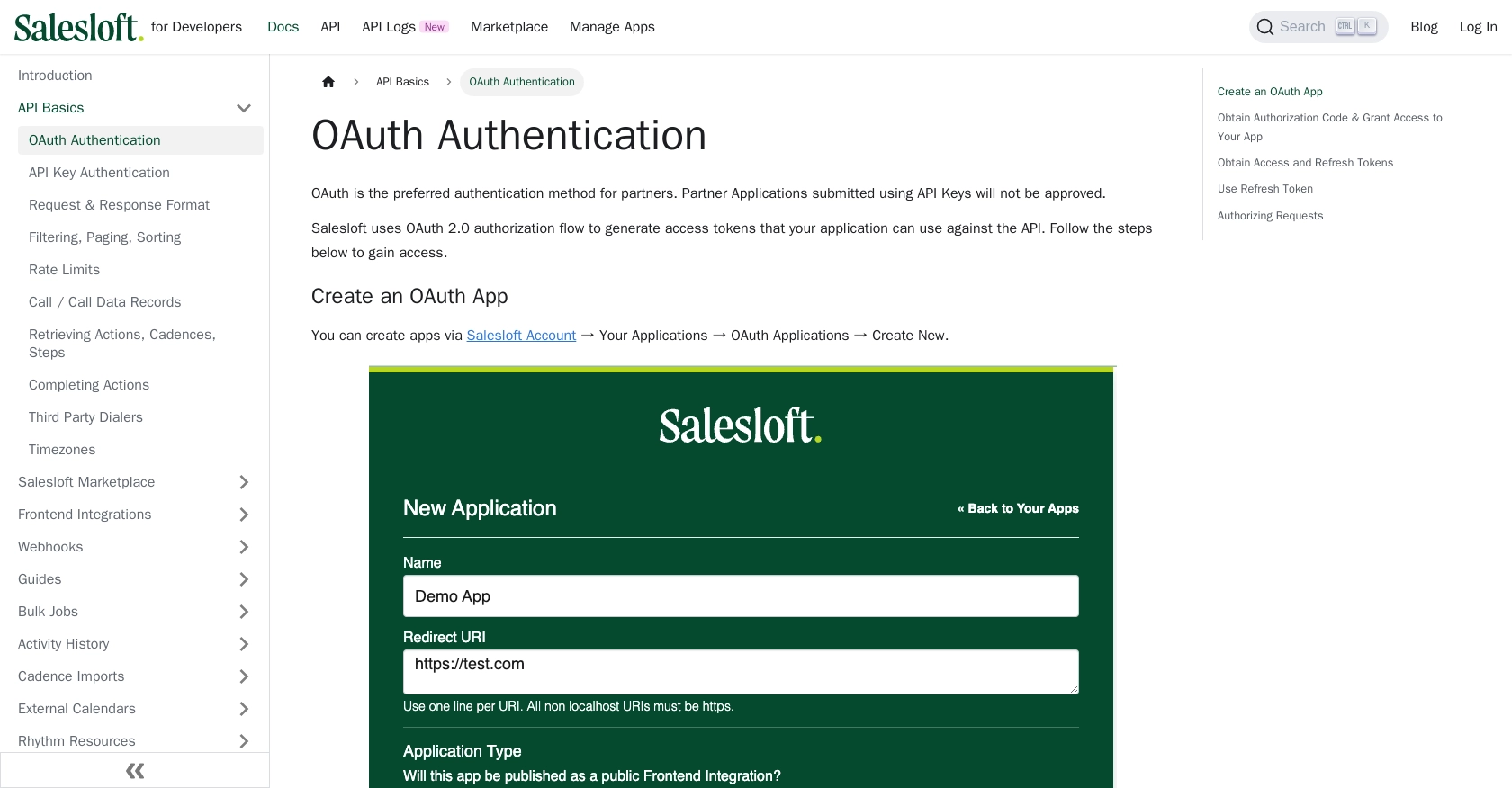
sbb-itb-96038d7
Making API Calls to Retrieve Salesloft Call Data Using Python
To interact with the Salesloft API and retrieve call data, you'll need to set up your Python environment and make the necessary API calls. This section will guide you through the process of fetching call records using Python.
Setting Up Your Python Environment for Salesloft API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Install the
requests
library using pip:
pip install requests
Fetching Call Records from Salesloft API
With your environment set up, you can now write a Python script to retrieve call records from Salesloft. Follow these steps:
- Create a new Python file named
get_salesloft_calls.py
and add the following code: - Replace
YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. - Run the script using the command:
import requests
# Define the API endpoint and headers
endpoint = "https://api.salesloft.com/v2/activities/calls"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the Salesloft API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Iterate through the call records and print details
for call in data['data']:
print(f"Call ID: {call['id']}, To: {call['to']}, Duration: {call['duration']} seconds")
else:
print(f"Failed to retrieve calls: {response.status_code} - {response.text}")
python get_salesloft_calls.py
Upon successful execution, the script will output call details such as call ID, recipient, and duration. If the request fails, it will print an error message with the status code and response text.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The Salesloft API provides error codes to help diagnose issues:
- 403 Forbidden: Access is denied. Check your access token and permissions.
- 404 Not Found: The requested resource doesn't exist.
- 422 Unprocessable Entity: Invalid parameters were provided.
Verify the success of your API calls by checking the Salesloft sandbox account for the retrieved call records. Ensure the data matches the expected output.
For more detailed information on error handling, refer to the Salesloft API documentation.
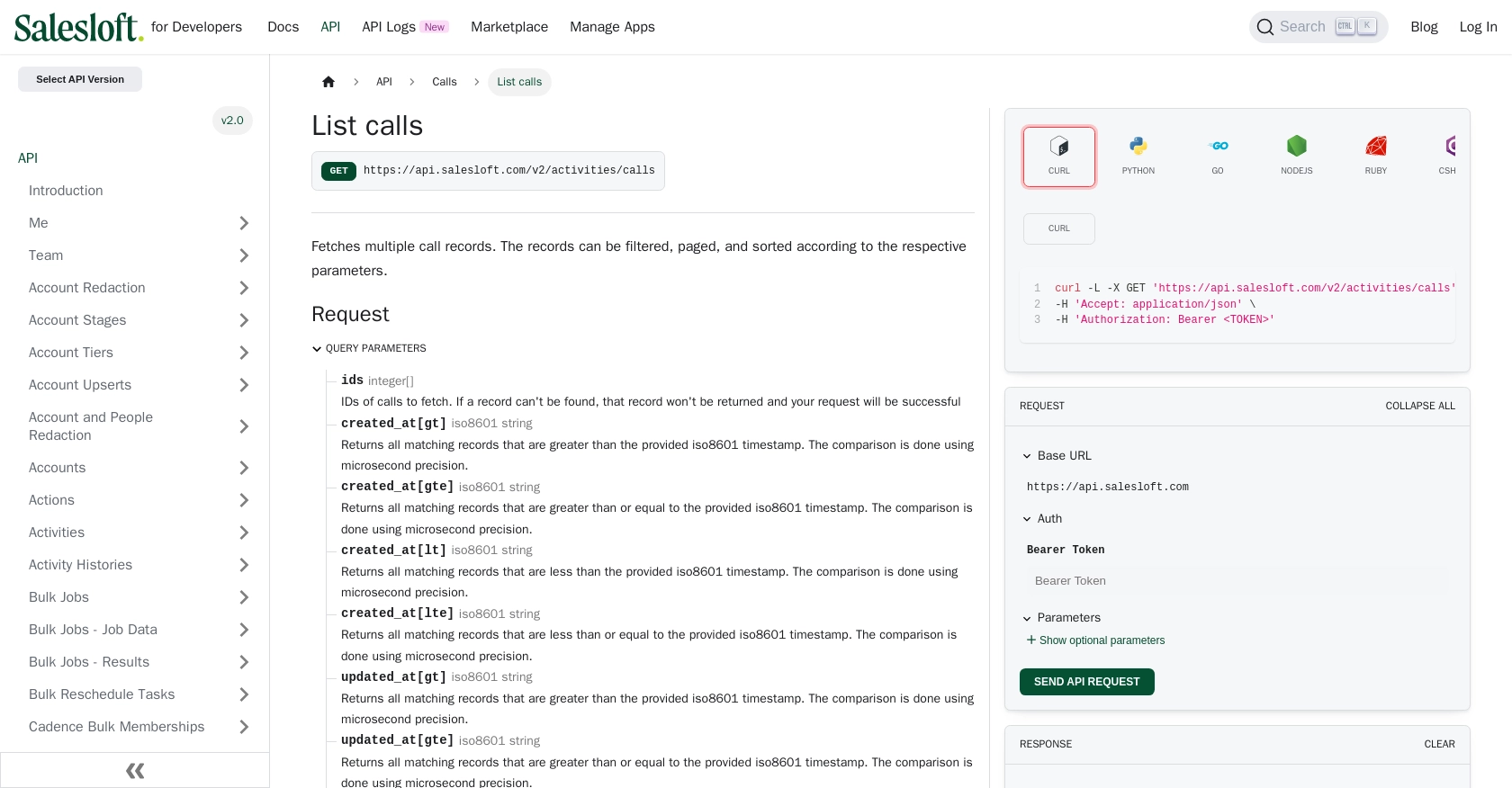
Conclusion and Best Practices for Using Salesloft API in Python
Integrating with the Salesloft API using Python provides a powerful way to access and analyze call data, enhancing your sales strategies and customer interactions. By following the steps outlined in this guide, you can efficiently retrieve call records and handle potential errors effectively.
Best Practices for Secure and Efficient Salesloft API Integration
- Secure Storage of Credentials: Always store your access and refresh tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handling Rate Limits: Be mindful of the Salesloft API rate limit of 600 requests per minute. Implement logic to handle rate limiting by checking the
x-ratelimit-remaining-minute
header and pausing requests if necessary. For more details, refer to the Salesloft API rate limits documentation. - Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
Enhancing Integration Capabilities with Endgrate
While integrating with Salesloft is a valuable step, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint for various platforms, including Salesloft. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can simplify your integration processes and enhance your application's capabilities by visiting Endgrate.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/activities-calls-index/
Ready to get started?