Using the Pendo API to Get Page Accounts in Javascript
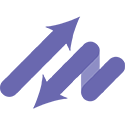
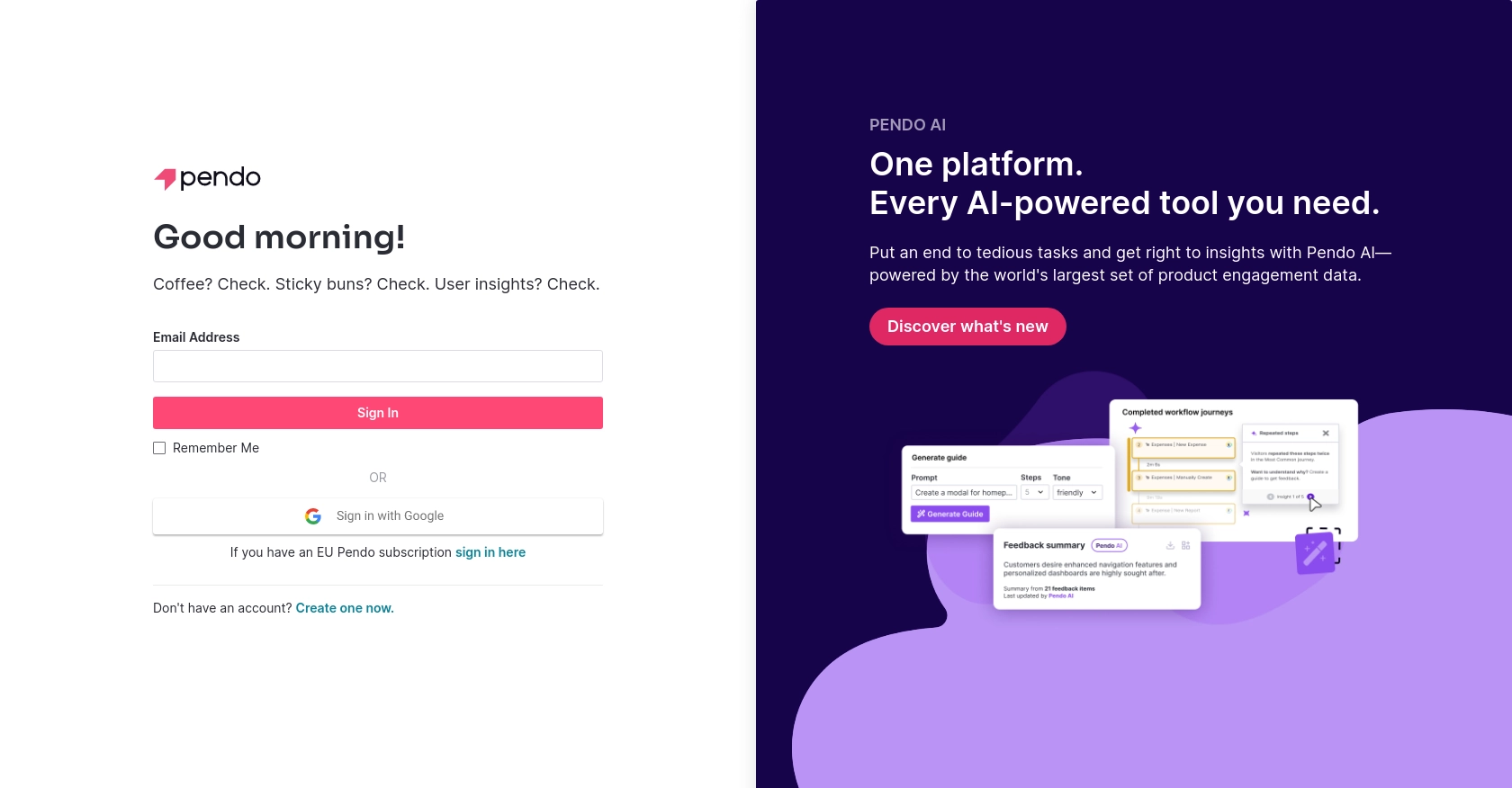
Introduction to Pendo API Integration
Pendo is a powerful platform that provides product teams with insights into user behavior, helping them create more engaging and effective software experiences. With features like in-app messaging, user feedback collection, and detailed analytics, Pendo enables businesses to understand and improve their product usage.
Developers may want to integrate with Pendo's API to access detailed analytics and user data, such as page accounts, to enhance their applications. For example, by retrieving page account information, a developer can analyze user interactions on specific pages and tailor the user experience accordingly.
Setting Up Your Pendo Test Account for API Integration
Before you can start using the Pendo API to retrieve page account information, you'll need to set up a Pendo test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Pendo Test Account
If you don't already have a Pendo account, you can sign up for a free trial on the Pendo website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Pendo website and click on the sign-up button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Pendo dashboard.
Generating Your Pendo API Key
To authenticate your API requests, you'll need to generate an API key. Pendo uses API key-based authentication, which is straightforward to set up.
- Navigate to the settings section of your Pendo dashboard.
- Locate the API key management section.
- Click on the option to generate a new API key.
- Copy the generated API key and store it securely, as you'll need it for making API calls.
With your test account and API key ready, you're now set to begin interacting with the Pendo API using JavaScript. In the next section, we'll walk through the process of making API calls to retrieve page account data.
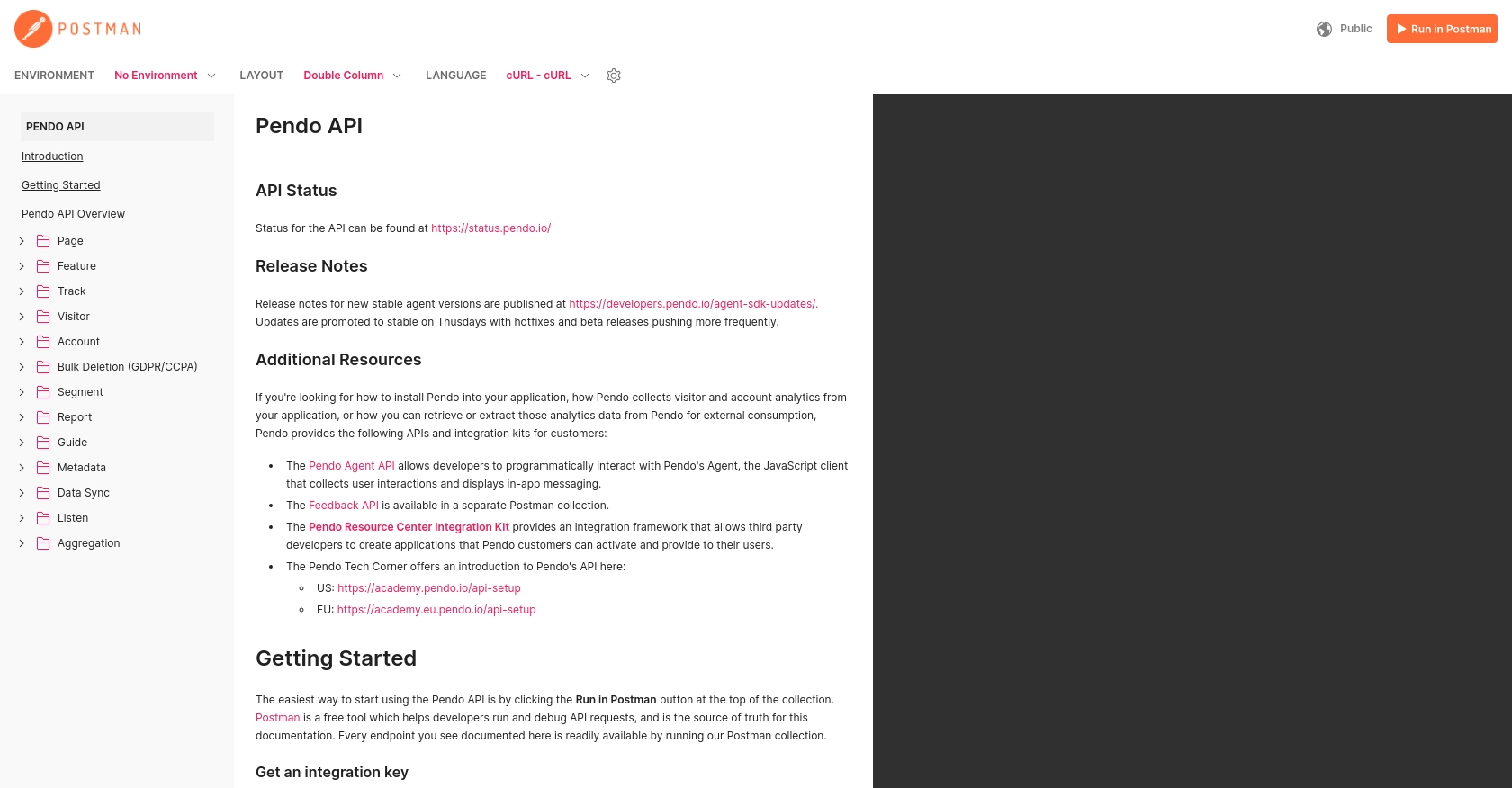
sbb-itb-96038d7
Making API Calls to Retrieve Pendo Page Accounts Using JavaScript
To interact with the Pendo API and retrieve page account data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment and writing the code to fetch the desired data.
Setting Up Your JavaScript Environment for Pendo API Integration
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js. Make sure you have Node.js installed on your machine.
- Download and install Node.js if you haven't already.
- Initialize a new project by running
npm init -y
in your terminal. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Fetch Pendo Page Accounts
With your environment set up, you can now write the code to make API calls to Pendo. The following example demonstrates how to retrieve page account data using the axios
library.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://engageapi.pendo.io/api/v1/pages';
const headers = {
'Authorization': 'Bearer YOUR_API_KEY',
'Content-Type': 'application/json'
};
// Function to get page accounts
async function getPageAccounts() {
try {
const response = await axios.get(endpoint, { headers });
const pageAccounts = response.data;
console.log('Page Accounts:', pageAccounts);
} catch (error) {
console.error('Error fetching page accounts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getPageAccounts();
Replace YOUR_API_KEY
with the API key you generated from your Pendo account. This code sets up the API endpoint and headers, makes a GET request to the Pendo API, and logs the retrieved page accounts to the console.
Verifying Successful API Requests and Handling Errors
After running the code, you should see the page account data printed in your console. If the request is successful, the data will match the page accounts in your Pendo test account.
To handle errors, the code checks for response errors and logs them. Common error codes include:
- 401 Unauthorized: Check your API key and ensure it's correctly included in the headers.
- 404 Not Found: Verify the API endpoint URL.
- 500 Internal Server Error: This may indicate an issue with the Pendo server; try again later.
For more details on error handling, refer to the Pendo API documentation.
Conclusion and Best Practices for Using Pendo API with JavaScript
Integrating with the Pendo API using JavaScript allows developers to access valuable insights into user interactions and page accounts, enabling them to enhance user experiences and optimize product features. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate requests, and handle API interactions.
Best Practices for Secure and Efficient Pendo API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Pendo's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Data Standardization: Transform and standardize data fields to ensure consistency across different integrations and systems.
- Error Handling: Implement robust error handling to manage API response errors gracefully and provide meaningful feedback to users.
By adhering to these best practices, you can ensure a secure and efficient integration with the Pendo API, allowing you to leverage its full potential in your applications.
Streamline Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Pendo, allowing you to focus on your core product while outsourcing complex integrations.
With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can save you time and resources.
Read More
Ready to get started?