How to Create or Update Leads with the Pipedrive API in Javascript
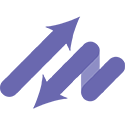
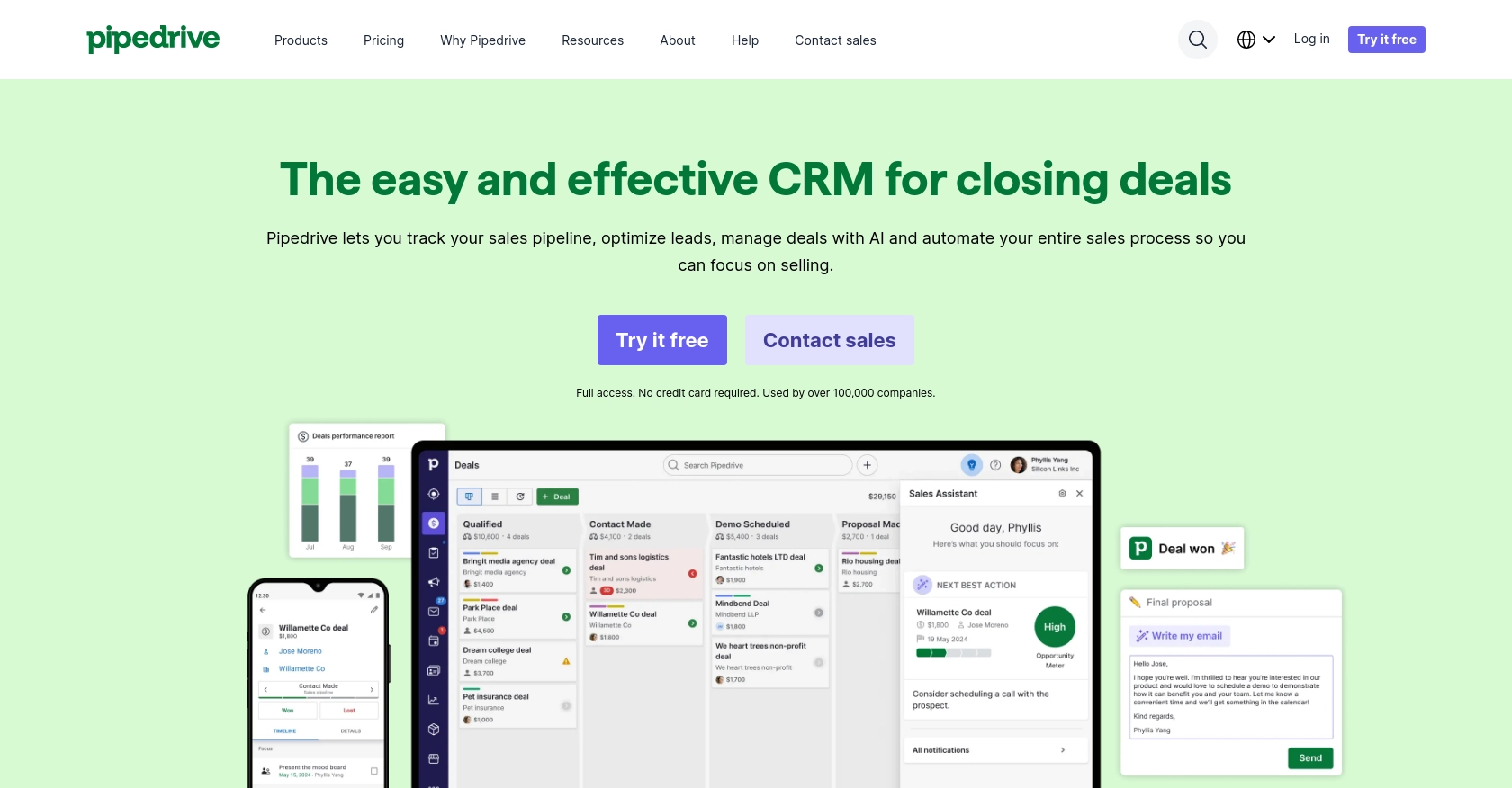
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage deals, and streamline their sales pipeline.
Integrating with Pipedrive's API allows developers to automate and enhance sales operations. For example, you can create or update leads directly from your application, ensuring that your sales team has the most up-to-date information at their fingertips. This integration can significantly improve efficiency and accuracy in managing potential deals.
Setting Up Your Pipedrive Developer Sandbox Account
Before you begin integrating with the Pipedrive API, it's essential to set up a developer sandbox account. This account provides a risk-free environment to test and develop your application without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Pipedrive Developer Sandbox page.
- Fill out the form to request access to a sandbox account.
- Once approved, you'll receive access to a sandbox environment that mimics a regular Pipedrive account.
This sandbox account allows you to perform testing and development in a safe environment, ensuring your app functions correctly before going live.
Generating OAuth Credentials for Pipedrive API
Pipedrive uses OAuth 2.0 for authentication. To interact with the API, you'll need to create an app and obtain the necessary credentials:
- Log in to your Pipedrive sandbox account.
- Navigate to the Developer Hub and register your app.
- During registration, you'll receive a client ID and client secret, which are essential for the OAuth flow.
Ensure you store these credentials securely, as they are required for authenticating API requests.
Configuring OAuth Scopes and Permissions
When setting up your app, you'll need to define the scopes and permissions it requires:
- Choose only the necessary scopes to minimize security risks.
- Ensure your app's installation flows are smooth and handle various user scenarios.
Proper configuration of scopes ensures your app has the right level of access to perform its functions.
With your sandbox account and OAuth credentials ready, you're now set to start integrating with the Pipedrive API using JavaScript.
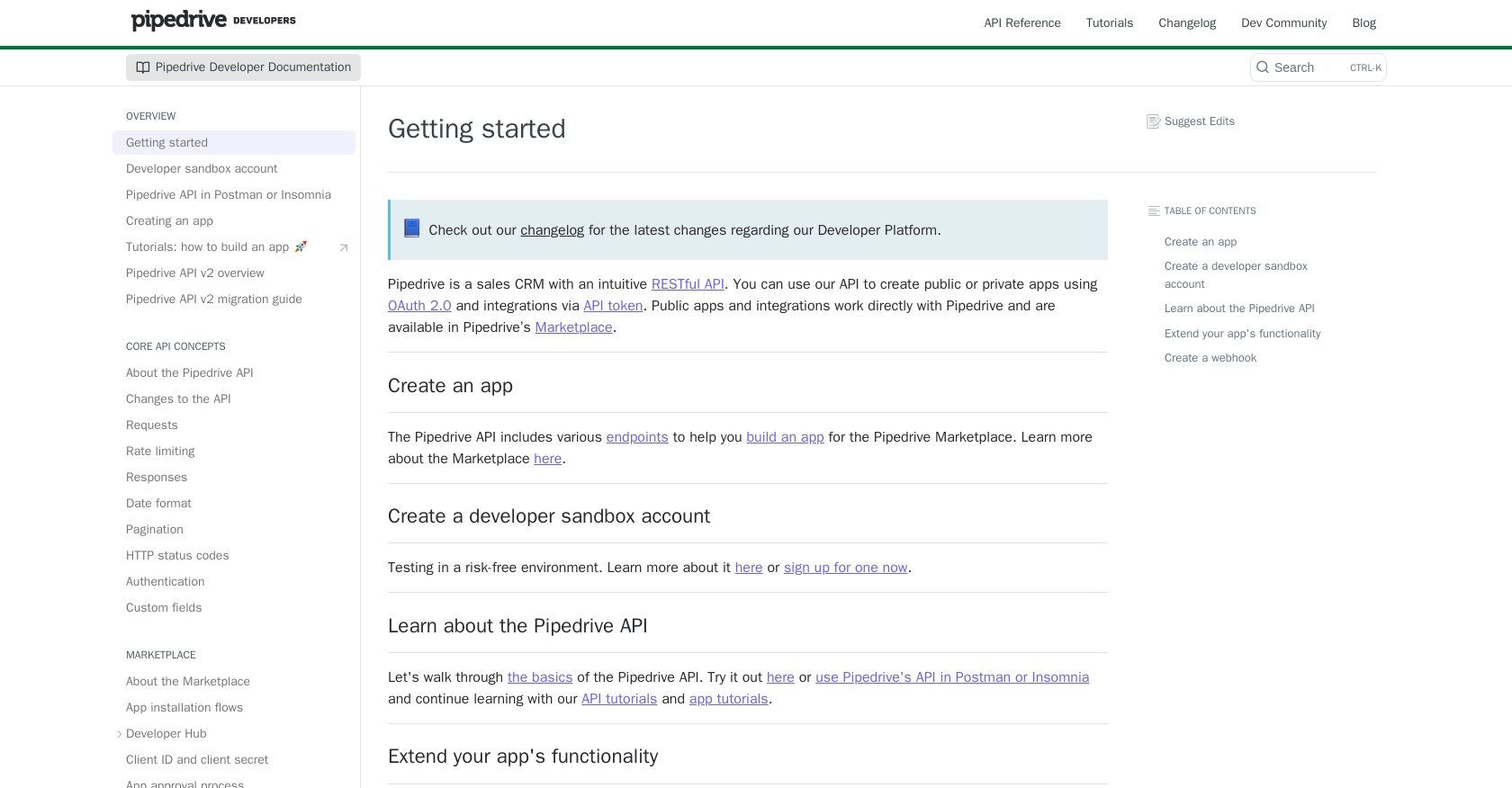
sbb-itb-96038d7
Making API Calls to Create or Update Leads in Pipedrive Using JavaScript
To interact with the Pipedrive API and manage leads, you'll need to use JavaScript to make HTTP requests. This section will guide you through setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Pipedrive API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a browser.
You'll also need the axios
library to simplify HTTP requests. Install it using npm:
npm install axios
Creating a Lead with Pipedrive API Using JavaScript
To create a lead, you'll send a POST request to the Pipedrive API. Here's a sample code snippet to create a lead:
const axios = require('axios');
const createLead = async () => {
const url = 'https://api.pipedrive.com/v1/leads';
const token = 'Your_Access_Token'; // Replace with your OAuth access token
const leadData = {
title: 'New Lead',
person_id: 123, // Replace with actual person ID
organization_id: 456, // Replace with actual organization ID
value: { amount: 500, currency: 'USD' }
};
try {
const response = await axios.post(url, leadData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Lead Created:', response.data);
} catch (error) {
console.error('Error Creating Lead:', error.response.data);
}
};
createLead();
Replace Your_Access_Token
with your actual OAuth access token. The person_id
and organization_id
should be valid IDs from your Pipedrive account.
Updating a Lead with Pipedrive API Using JavaScript
To update an existing lead, use a PATCH request. Here's how you can update a lead:
const updateLead = async (leadId) => {
const url = `https://api.pipedrive.com/v1/leads/${leadId}`;
const token = 'Your_Access_Token'; // Replace with your OAuth access token
const updateData = {
title: 'Updated Lead Title',
value: { amount: 1000, currency: 'EUR' }
};
try {
const response = await axios.patch(url, updateData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Lead Updated:', response.data);
} catch (error) {
console.error('Error Updating Lead:', error.response.data);
}
};
updateLead('lead_id_here');
Replace lead_id_here
with the ID of the lead you wish to update. Adjust the updateData
object as needed to reflect the changes you want to make.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors. Successful requests will return a status code of 200 or 201, while errors might return codes like 400 (Bad Request) or 401 (Unauthorized). Refer to the Pipedrive HTTP status codes documentation for more details.
In the examples above, error handling is done using try-catch blocks, which log errors to the console. You can expand this to include more sophisticated error handling as needed.
By following these steps, you can efficiently create and update leads in Pipedrive using JavaScript, enhancing your sales operations through automation.
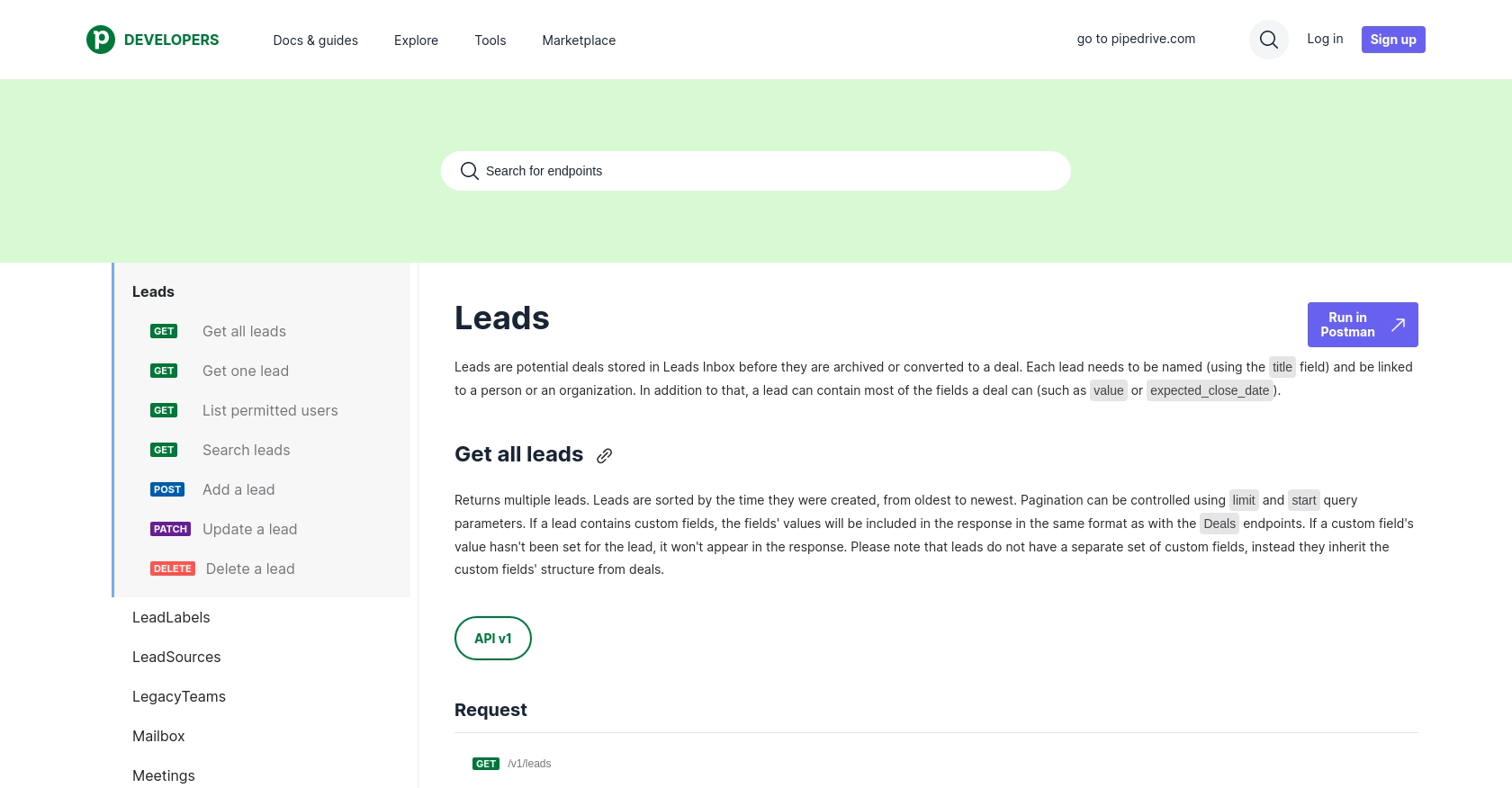
Conclusion and Best Practices for Pipedrive API Integration
Integrating with the Pipedrive API using JavaScript offers a powerful way to automate and enhance your sales processes. By creating and updating leads programmatically, you can ensure that your sales team always has access to the most current and accurate information, improving efficiency and decision-making.
Best Practices for Secure and Efficient Pipedrive API Usage
- Secure Storage of Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handling Rate Limits: Be mindful of Pipedrive's rate limits, which allow up to 80 requests per 2 seconds per access token for the Essential plan. Implement logic to handle HTTP 429 errors gracefully and consider using webhooks to reduce the need for frequent polling. For more details, refer to the Pipedrive rate limiting documentation.
- Data Standardization: Ensure consistent data formats when creating or updating leads. This includes standardizing currency formats and date fields to match Pipedrive's requirements.
Enhancing Your Integration with Endgrate
While building integrations with Pipedrive can greatly enhance your sales operations, managing multiple integrations can become complex and time-consuming. This is where Endgrate can help. By using Endgrate, you can streamline your integration processes, allowing you to focus on your core product development. Endgrate provides a unified API endpoint that simplifies the integration experience, enabling you to build once and deploy across multiple platforms effortlessly.
Explore how Endgrate can save you time and resources by visiting Endgrate's website and discover the benefits of a seamless integration experience.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Leads
Ready to get started?