Using the Mixpanel API to Get Annotations (with PHP examples)
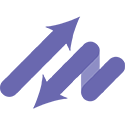
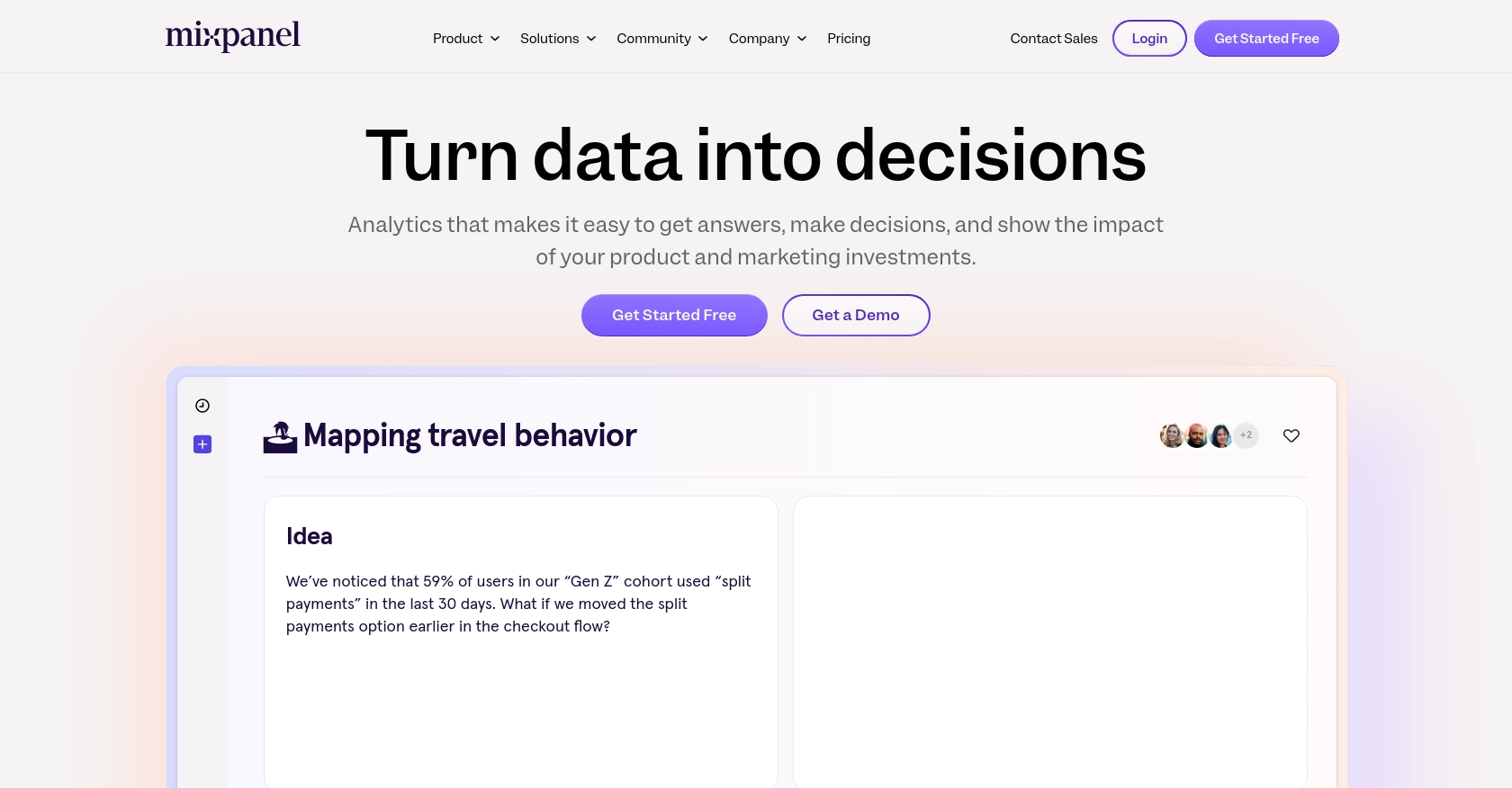
Introduction to Mixpanel API Integration
Mixpanel is a powerful analytics platform that helps businesses understand user behavior across their applications. By providing detailed insights into user interactions, Mixpanel enables companies to make data-driven decisions to enhance user engagement and improve product offerings.
Integrating with Mixpanel's API allows developers to access and manage various analytics data, such as annotations, which are notes or comments added to specific data points to provide context. For example, a developer might want to retrieve annotations to analyze trends or correlate specific events with marketing campaigns.
This article will guide you through using PHP to interact with the Mixpanel API to retrieve annotations, providing a step-by-step approach to setting up and executing API calls effectively.
Setting Up a Mixpanel Test Account for API Integration
Before you can start interacting with the Mixpanel API to retrieve annotations, you'll need to set up a test account. This involves creating a service account within Mixpanel, which will allow you to authenticate your API requests securely.
Creating a Mixpanel Service Account
Mixpanel uses service accounts to authenticate API requests. A service account is a special type of user account that represents a non-human entity, such as a script or backend service. Follow these steps to create a service account:
- Log in to your Mixpanel account and navigate to the Organization Settings.
- In the Service Accounts tab, click on Create Service Account.
- Select the appropriate role and projects for the service account. Ensure that it has access to the projects you intend to work with.
- Once created, immediately store the service account's secret in a secure location, as you won't be able to access it again later.
For more details on managing service accounts, refer to the Mixpanel Service Accounts documentation.
Authenticating with Mixpanel Using Service Accounts
Mixpanel's API requires authentication via HTTP Basic Auth using the service account's username and secret. Here's how you can authenticate your requests:
$serviceAccountUsername = 'your_service_account_username';
$serviceAccountSecret = 'your_service_account_secret';
// Base64 encode the credentials
$credentials = base64_encode("$serviceAccountUsername:$serviceAccountSecret");
// Set the authorization header
$headers = [
'Authorization: Basic ' . $credentials
];
// Example of making an authenticated request
$ch = curl_init('https://mixpanel.com/api/app/me');
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Replace your_service_account_username
and your_service_account_secret
with your actual service account credentials.
For more information on authentication, visit the Mixpanel Authentication documentation.
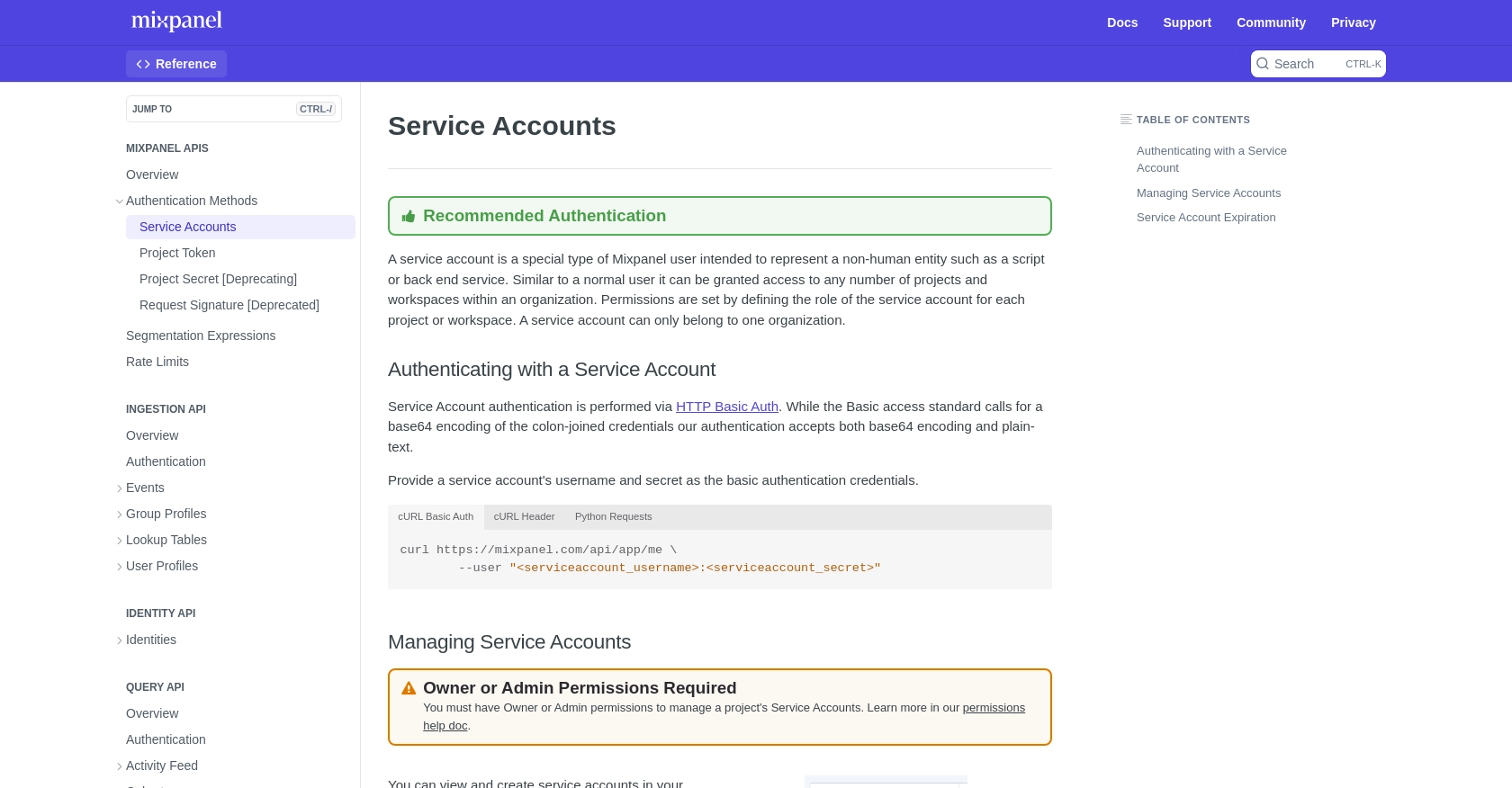
sbb-itb-96038d7
Executing Mixpanel API Calls to Retrieve Annotations Using PHP
Once you have set up your Mixpanel service account and authenticated your requests, you can proceed to interact with the Mixpanel API to retrieve annotations. This section will guide you through the process of making API calls using PHP, ensuring you can efficiently access the data you need.
Prerequisites for PHP Integration with Mixpanel API
Before making API calls, ensure you have the following prerequisites installed on your system:
- PHP 7.4 or higher
- cURL extension enabled in PHP
These components are essential for executing HTTP requests and handling API responses.
Making a GET Request to List Annotations in Mixpanel
To retrieve annotations from Mixpanel, you'll need to make a GET request to the appropriate endpoint. Below is a PHP example demonstrating how to achieve this:
$serviceAccountUsername = 'your_service_account_username';
$serviceAccountSecret = 'your_service_account_secret';
$projectId = 'your_project_id';
// Base64 encode the credentials
$credentials = base64_encode("$serviceAccountUsername:$serviceAccountSecret");
// Set the authorization header
$headers = [
'Authorization: Basic ' . $credentials
];
// Define the API endpoint
$url = "https://mixpanel.com/api/app/projects/$projectId/annotations";
// Initialize cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the JSON response
$annotations = json_decode($response, true);
// Output the annotations
foreach ($annotations as $annotation) {
echo "Annotation: " . $annotation['text'] . "\n";
}
Replace your_service_account_username
, your_service_account_secret
, and your_project_id
with your actual Mixpanel credentials and project ID.
Verifying Successful API Requests in Mixpanel
After executing the API call, you should verify that the request was successful by checking the response. A successful request will return a list of annotations associated with your project. If the request fails, ensure that your credentials and project ID are correct.
Handling Errors and Understanding Mixpanel API Error Codes
When interacting with the Mixpanel API, you may encounter various error codes. It's crucial to handle these errors gracefully in your application. Common error codes include:
- 401 Unauthorized: Check your authentication credentials.
- 404 Not Found: Verify the endpoint URL and project ID.
- 429 Too Many Requests: You have hit the rate limit. Refer to the Mixpanel Rate Limits documentation for more details.
Implement error handling in your code to manage these scenarios effectively and ensure a robust integration.
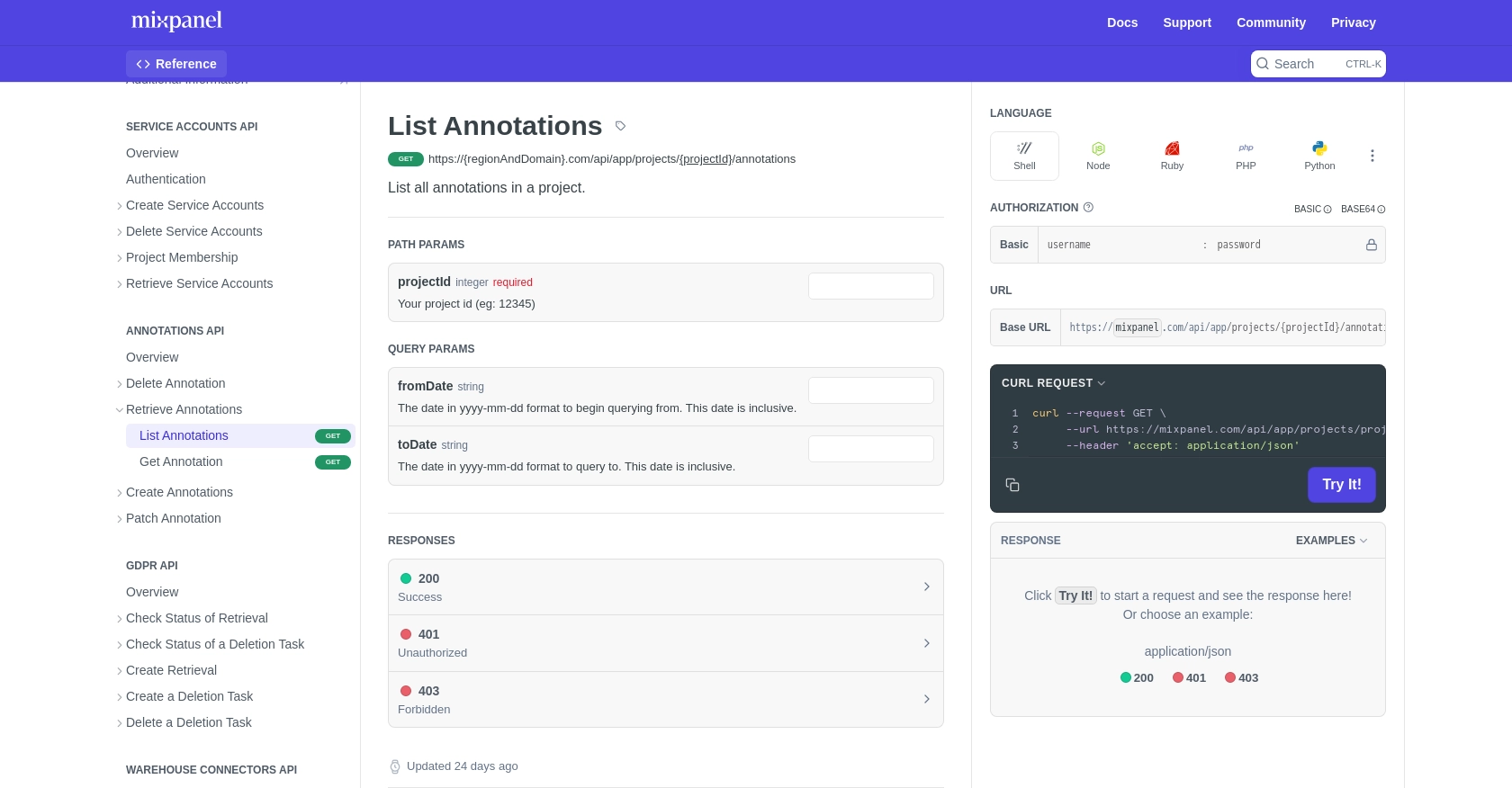
Conclusion and Best Practices for Using Mixpanel API with PHP
Integrating with the Mixpanel API to retrieve annotations using PHP can significantly enhance your ability to analyze and leverage user data effectively. By following the steps outlined in this guide, you can set up a robust integration that allows you to access valuable insights and make data-driven decisions.
Best Practices for Secure and Efficient Mixpanel API Integration
- Secure Credential Storage: Always store your service account credentials securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Mixpanel's rate limits to avoid disruptions. Implement logic to handle
429 Too Many Requests
errors by spreading out requests or consolidating queries. Refer to the Mixpanel Rate Limits documentation for more information. - Data Standardization: Ensure that the data retrieved from Mixpanel is standardized and transformed as needed for your application. This will facilitate easier analysis and integration with other systems.
- Error Handling: Implement comprehensive error handling to manage potential API errors gracefully. This will help maintain a seamless user experience and ensure the reliability of your integration.
Streamlining Integrations with Endgrate
While integrating with Mixpanel's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Mixpanel. This allows you to build once for each use case and streamline the integration process, saving time and resources.
Explore how Endgrate can simplify your integration needs and enhance your product offerings by visiting Endgrate. Focus on your core product while Endgrate handles the complexities of integration.
Read More
Ready to get started?