Using the Quickbooks API to Get Products in Python
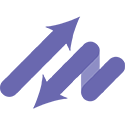
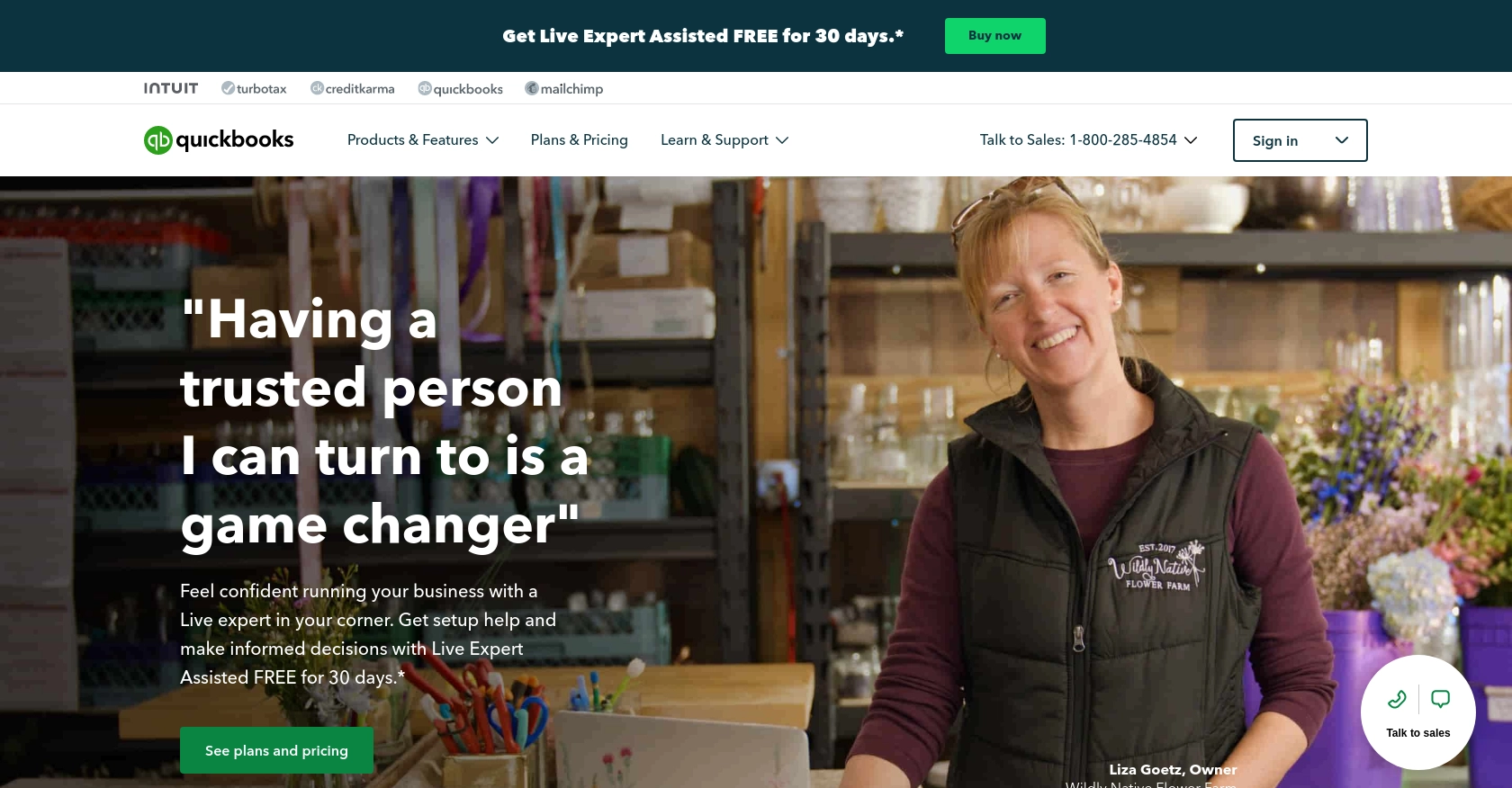
Introduction to Quickbooks API Integration
Quickbooks is a widely-used accounting software that offers a comprehensive suite of tools for managing financial operations. It is particularly popular among small to medium-sized businesses due to its user-friendly interface and robust features, including invoicing, payroll, and expense tracking.
Integrating with the Quickbooks API allows developers to automate and streamline financial processes, such as retrieving product information. For example, a developer might use the Quickbooks API to fetch product details and synchronize them with an inventory management system, ensuring accurate and up-to-date records across platforms.
Setting Up Your Quickbooks Sandbox Account for API Integration
Before you can start interacting with the Quickbooks API, you'll need to set up a sandbox account. This allows you to test API calls without affecting live data, providing a safe environment to develop and debug your integration.
Creating a Quickbooks Developer Account
If you don't already have a Quickbooks Developer account, you'll need to create one. Follow these steps to get started:
- Visit the Quickbooks Developer Portal.
- Click on "Sign Up" and fill in the required information to create your developer account.
- Once registered, log in to your account to access the developer dashboard.
Setting Up a Quickbooks Sandbox Company
After creating your developer account, you can set up a sandbox company to test your API integration:
- Navigate to the "Sandbox" section in the developer dashboard.
- Click on "Add Sandbox" to create a new sandbox company.
- Choose the type of company you want to simulate and complete the setup process.
Creating a Quickbooks App for OAuth Authentication
To interact with the Quickbooks API, you'll need to create an app and obtain OAuth credentials:
- Go to the "My Apps" section in your developer dashboard.
- Click on "Create an App" and select "Quickbooks Online and Payments" as the platform.
- Fill in the app details, including the name and description.
- Once the app is created, navigate to the "Keys & OAuth" section to find your Client ID and Client Secret.
These credentials will be used to authenticate your API requests. Make sure to store them securely.
Configuring OAuth Scopes and Redirect URIs
To ensure your app has the necessary permissions, configure the OAuth scopes:
- In the "Keys & OAuth" section, scroll down to "Scopes" and select the required permissions for accessing product data.
- Set up the redirect URIs that will be used during the OAuth flow.
For more detailed instructions, refer to the Quickbooks OAuth Documentation.
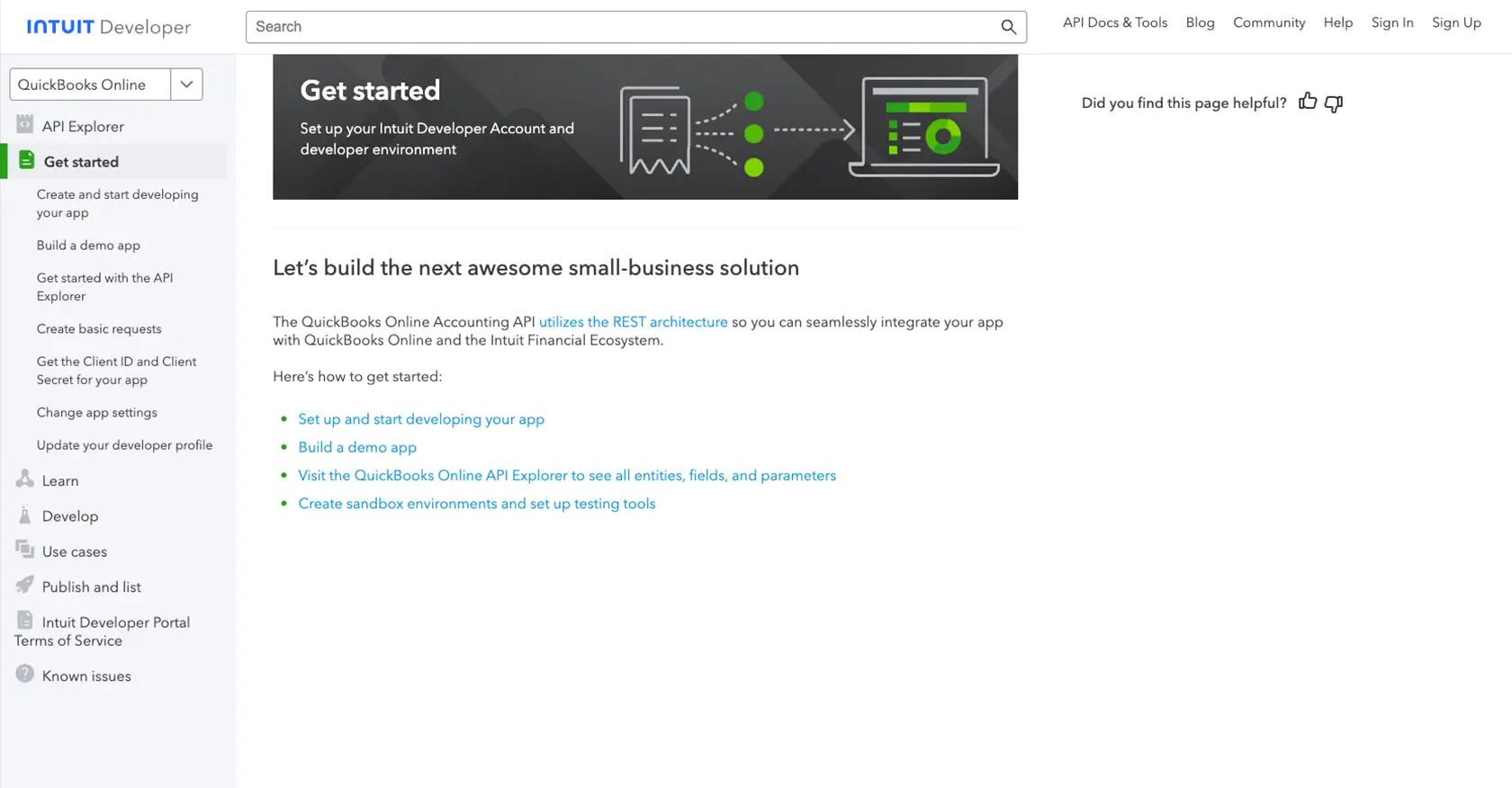
sbb-itb-96038d7
Making API Calls to Retrieve Products from Quickbooks Using Python
To interact with the Quickbooks API and retrieve product information, you'll need to set up your Python environment and write code to make the necessary API calls. This section will guide you through the process, including setting up Python, installing dependencies, and writing the code to fetch product data.
Setting Up Your Python Environment for Quickbooks API Integration
Before you begin, ensure you have the following installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Fetch Products from Quickbooks API
Create a new Python file named get_quickbooks_products.py
and add the following code:
import requests
import json
# Set the API endpoint and headers
endpoint = "https://quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/item"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Print the product information
for item in data.get("Item", []):
print(f"Product Name: {item['Name']}, Price: {item['UnitPrice']}")
else:
print(f"Failed to retrieve products. Status Code: {response.status_code}")
print(f"Error: {response.text}")
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual Quickbooks company ID and access token obtained during the OAuth setup.
Running the Python Script and Verifying API Call Success
Run the script from your terminal or command prompt using the following command:
python get_quickbooks_products.py
If successful, you should see a list of products with their names and prices printed in the console. This indicates that the API call was successful and the data was retrieved correctly.
Handling Errors and Common Quickbooks API Error Codes
When making API calls, it's essential to handle potential errors gracefully. The Quickbooks API may return various error codes, such as:
- 401 Unauthorized: Indicates that the access token is invalid or expired. Ensure your token is correct and refresh it if necessary.
- 403 Forbidden: The request is valid, but the server is refusing action. Check your app's permissions and scopes.
- 404 Not Found: The requested resource could not be found. Verify the endpoint URL and resource ID.
For more detailed information on error codes, refer to the Quickbooks API Documentation.
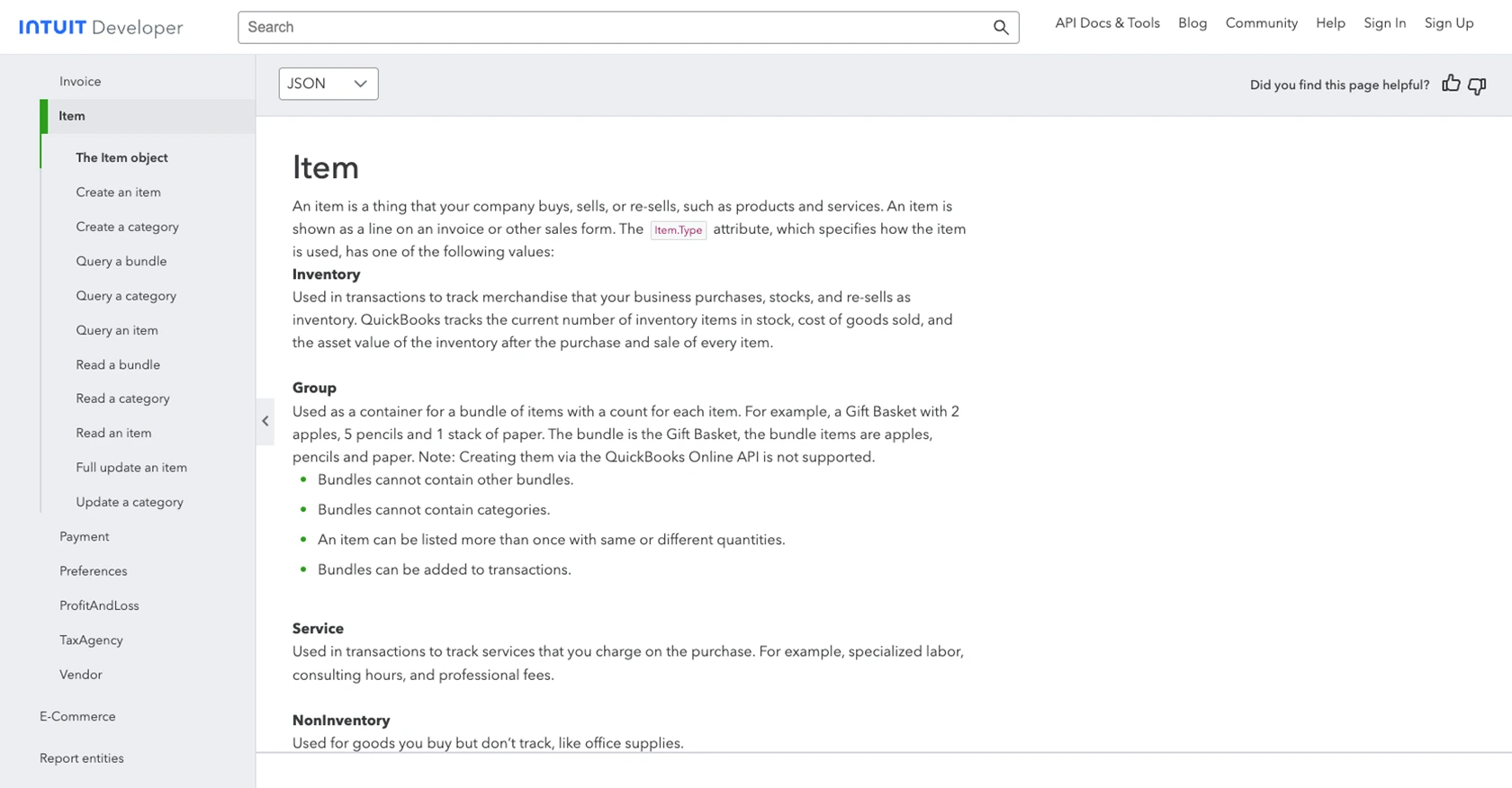
Best Practices for Quickbooks API Integration in Python
When integrating with the Quickbooks API, it's crucial to follow best practices to ensure a secure and efficient implementation. Here are some key considerations:
- Securely Store OAuth Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Quickbooks API has rate limits to prevent abuse. Monitor the response headers for rate limit information and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Quickbooks is transformed and standardized to match your application's data structures. This will help maintain consistency across different systems.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Quickbooks. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/item
Ready to get started?