Using the Zoho Books API to Get Sales Orders in Javascript
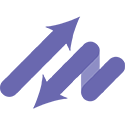
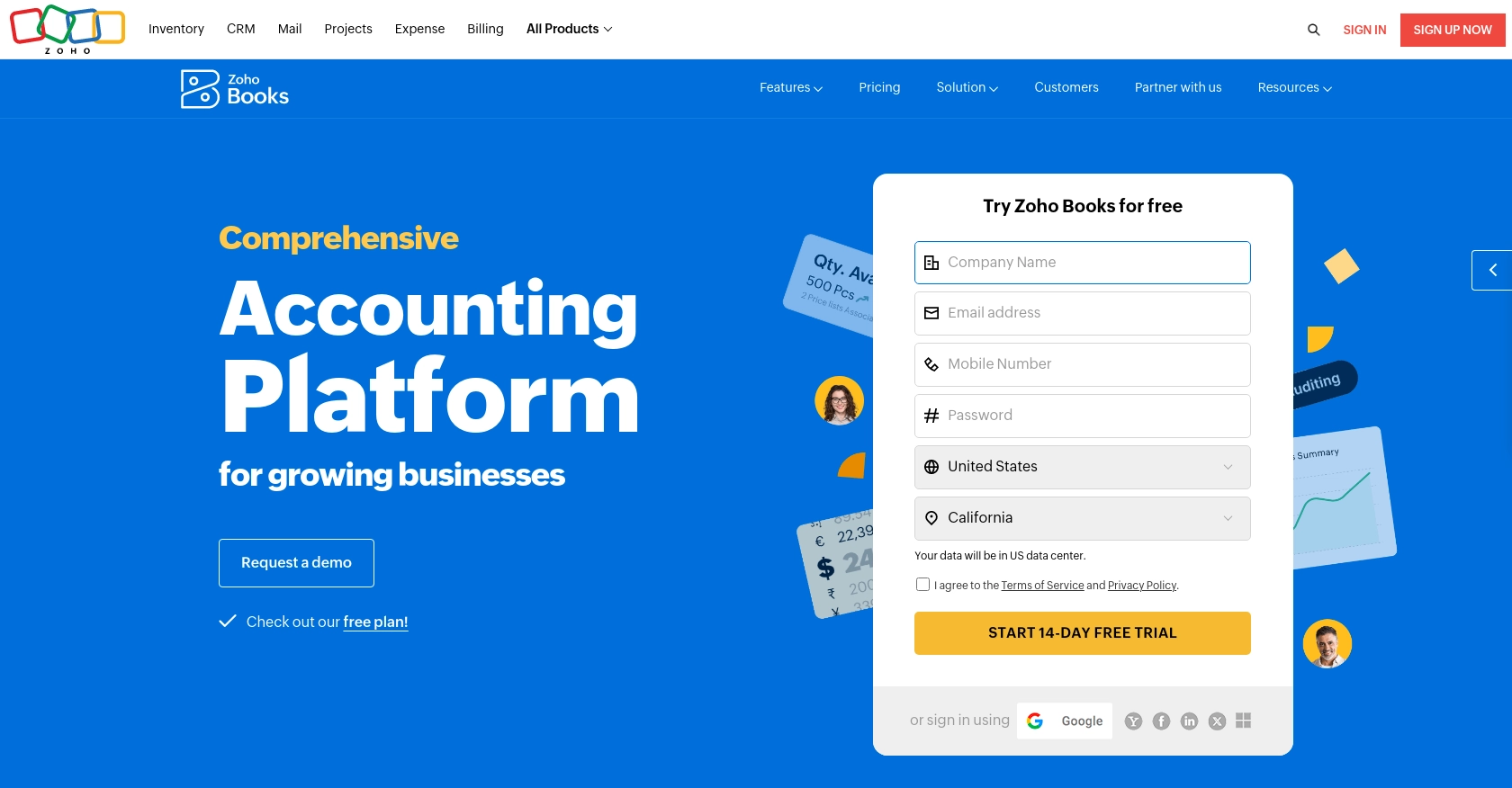
Introduction to Zoho Books API for Sales Orders
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. With its robust set of features, Zoho Books is a preferred choice for businesses looking to streamline their accounting processes.
For developers, integrating with the Zoho Books API opens up a world of possibilities to automate and enhance financial operations. One such integration is accessing sales orders, which can be crucial for businesses to track and manage their sales pipeline effectively.
By using the Zoho Books API, developers can automate the retrieval of sales orders, allowing for seamless integration with other systems such as CRM or inventory management. For example, a developer might want to fetch sales orders to update a dashboard that provides real-time insights into sales performance.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start interacting with the Zoho Books API to retrieve sales orders, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, follow these steps to create one:
- Visit the Zoho Books website and click on the "Sign Up" button.
- Fill in the required details, such as your name, email, and password, to create a free trial account.
- Once your account is created, log in to access the Zoho Books dashboard.
Setting Up OAuth Authentication for Zoho Books API
The Zoho Books API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth authentication:
- Go to the Zoho Developer Console and log in with your Zoho account credentials.
- Click on "Add Client ID" to register a new application.
- Provide the necessary details such as client name, homepage URL, and authorized redirect URIs.
- After registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are needed for API authentication.
Generating OAuth Tokens for Zoho Books API Access
To interact with the Zoho Books API, you need to generate access and refresh tokens. Here's how:
- Redirect users to the following authorization URL to obtain a grant token:
- Once the user authorizes the application, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for access and refresh tokens by making a POST request:
- Store the access and refresh tokens securely for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.salesorders.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
With your Zoho Books account and OAuth setup complete, you're ready to start making API calls to retrieve sales orders using JavaScript.
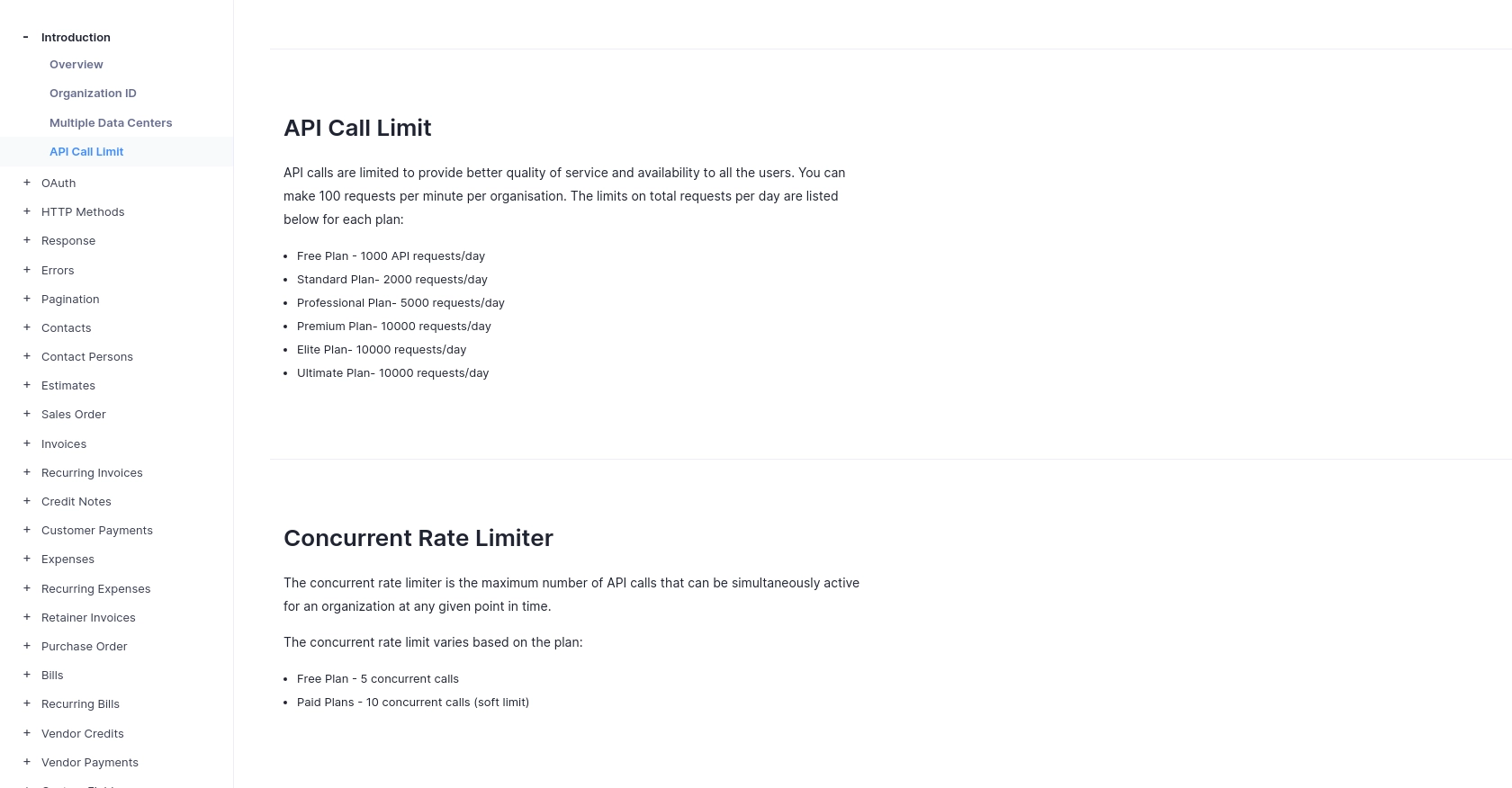
sbb-itb-96038d7
Making API Calls to Retrieve Sales Orders from Zoho Books Using JavaScript
Now that you have set up your Zoho Books account and OAuth authentication, it's time to make API calls to retrieve sales orders using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Zoho Books API
Before making API calls, ensure you have the necessary tools and libraries installed:
- Node.js: Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Fetch API: We'll use the Fetch API, which is built into modern browsers and Node.js environments, to make HTTP requests.
Writing JavaScript Code to Fetch Sales Orders from Zoho Books
Follow these steps to write the JavaScript code for retrieving sales orders:
- Create a new JavaScript file named
getSalesOrders.js
and open it in your code editor. - Add the following code to make a GET request to the Zoho Books API:
- Replace
YOUR_ACCESS_TOKEN
andYOUR_ORGANIZATION_ID
with your actual access token and organization ID.
const fetch = require('node-fetch');
const accessToken = 'YOUR_ACCESS_TOKEN';
const organizationId = 'YOUR_ORGANIZATION_ID';
const endpoint = `https://www.zohoapis.com/books/v3/salesorders?organization_id=${organizationId}`;
const headers = {
'Authorization': `Zoho-oauthtoken ${accessToken}`,
'Content-Type': 'application/json'
};
fetch(endpoint, { headers })
.then(response => response.json())
.then(data => {
console.log('Sales Orders:', data.salesorders);
})
.catch(error => console.error('Error fetching sales orders:', error));
Running the JavaScript Code and Handling Responses
To execute the code and retrieve sales orders, follow these steps:
- Open your terminal or command prompt.
- Navigate to the directory where your
getSalesOrders.js
file is located. - Run the script using Node.js:
- Upon successful execution, you should see the list of sales orders printed in the console.
node getSalesOrders.js
Handling Errors and Verifying API Call Success
It's important to handle potential errors and verify the success of your API calls:
- Check the HTTP status code in the response to ensure the request was successful. A status code of 200 indicates success.
- Handle error codes such as 400 (Bad Request), 401 (Unauthorized), and 429 (Rate Limit Exceeded) by implementing appropriate error handling logic.
- Verify the retrieved sales orders by cross-referencing them with the data in your Zoho Books sandbox account.
For more information on error codes, refer to the Zoho Books API documentation.
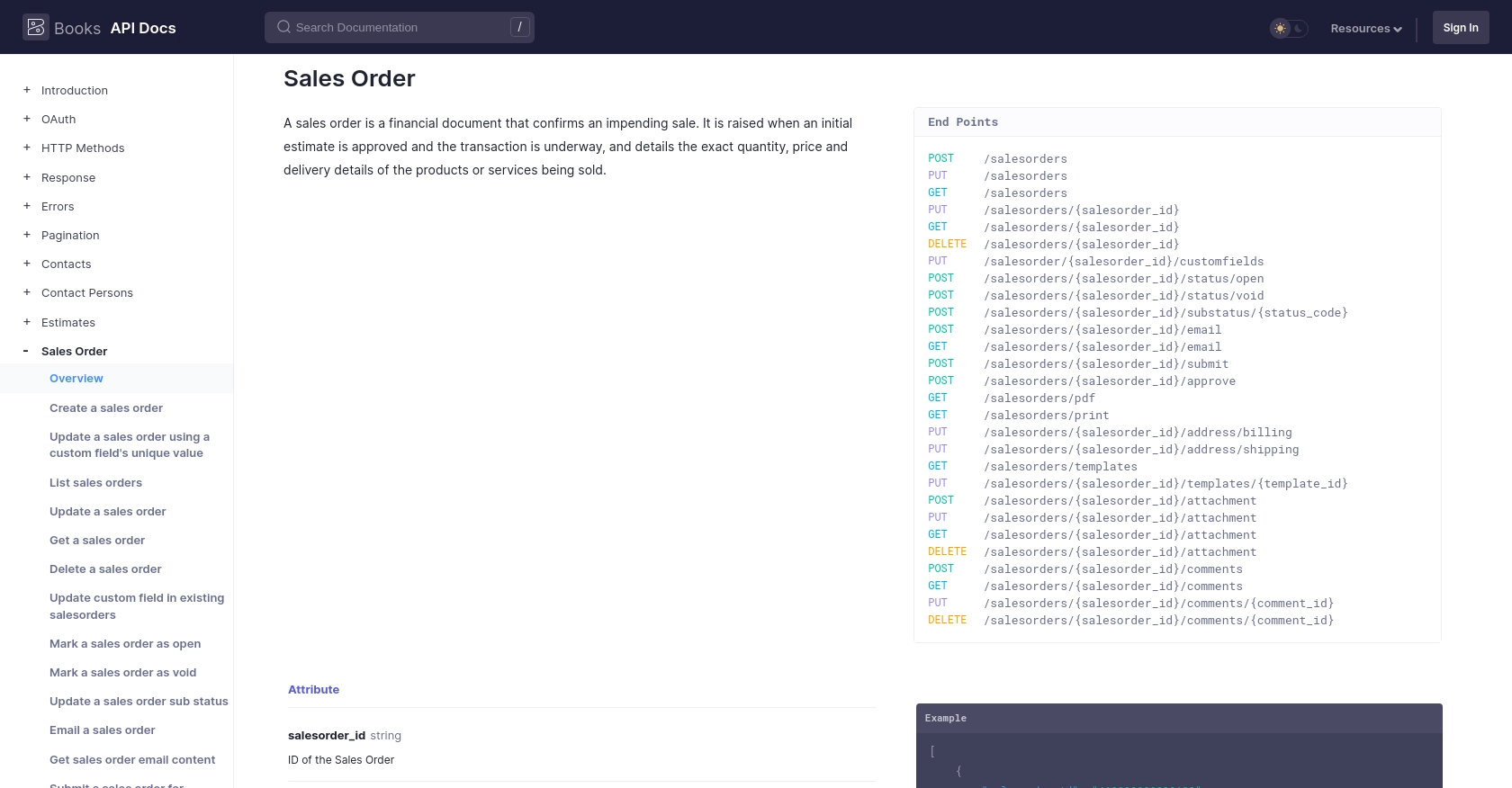
Conclusion and Best Practices for Using Zoho Books API in JavaScript
Integrating with the Zoho Books API to retrieve sales orders using JavaScript can significantly enhance your business's financial operations. By automating the process of fetching sales orders, you can streamline workflows and improve data accuracy across your systems.
Best Practices for Secure and Efficient Zoho Books API Integration
- Securely Store Credentials: Always store your OAuth tokens and client credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books API allows 100 requests per minute per organization. Implement logic to handle rate limits gracefully by queuing requests or implementing exponential backoff strategies. For more details, refer to the API call limit documentation.
- Data Standardization: Ensure that the data retrieved from Zoho Books is standardized and transformed as needed to fit your application's requirements. This can help maintain consistency across different systems.
- Error Handling: Implement robust error handling to manage different HTTP status codes and API-specific errors. This will ensure that your application can recover gracefully from unexpected issues.
Enhancing Your Integration Strategy with Endgrate
While integrating with Zoho Books API can provide significant benefits, managing multiple integrations can be complex and time-consuming. This is where Endgrate can help. By using Endgrate, you can:
- Outsource integrations to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/sales-order/#overview
Ready to get started?