Using the Typeform API to Get Responses in Javascript
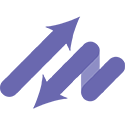
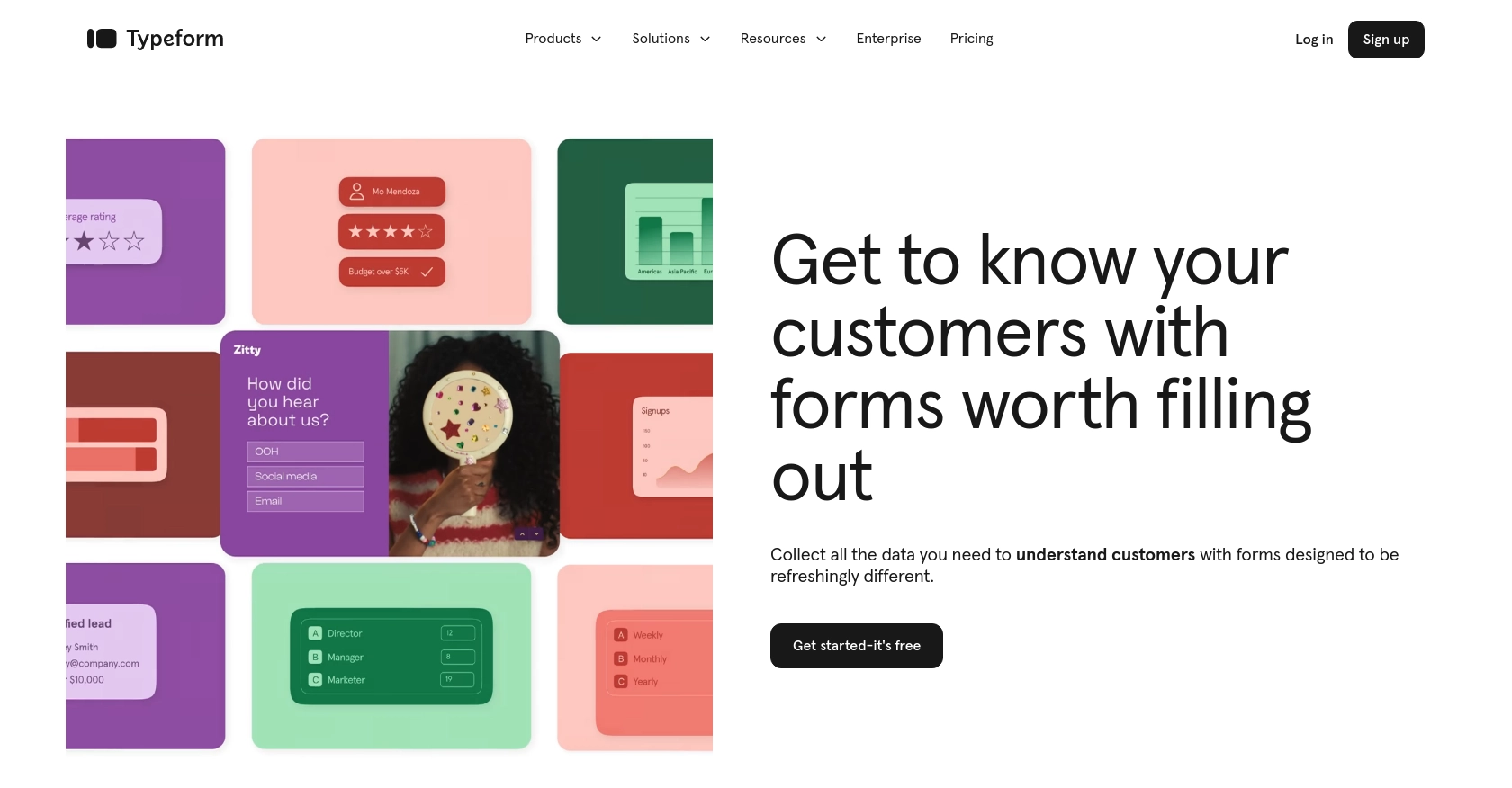
Introduction to Typeform API Integration
Typeform is a versatile online form builder that allows businesses to create engaging and interactive forms, surveys, and quizzes. Its user-friendly design and powerful features make it a popular choice for collecting data and feedback from users.
Integrating with Typeform's API enables developers to automate data retrieval and processing, enhancing the efficiency of data-driven applications. For example, you can use the Typeform API to fetch survey responses and analyze them to gain insights into customer preferences or feedback.
This article will guide you through using JavaScript to interact with the Typeform API, specifically focusing on retrieving form responses. By the end of this tutorial, you'll be able to seamlessly integrate Typeform data into your applications, streamlining your data management processes.
Setting Up Your Typeform Developer Account and Application
Before you can start interacting with the Typeform API using JavaScript, you'll need to set up a developer account and create an application to obtain the necessary credentials for OAuth 2.0 authentication. This process involves registering your application within the Typeform admin panel to generate a client ID and client secret.
Step-by-Step Guide to Creating a Typeform Developer Account
-
Sign Up or Log In:
If you don't have a Typeform account, visit the Typeform website and sign up for a free account. If you already have an account, simply log in.
-
Access Developer Apps:
Once logged in, navigate to the upper-left corner of the dashboard and click the icon drop-down menu next to your organization name. Under the Organization section, select Developer Apps.
-
Register a New Application:
Click on Register a new app. Fill in the required fields, including the App Name, App Website, and Redirect URI(s). The Redirect URI is where users will be redirected after they log in and grant access to your app.
-
Save Your Credentials:
After registering your app, you'll receive a client_id and client_secret. Make sure to copy and store these credentials securely, as you'll need them for OAuth 2.0 authentication.
Configuring OAuth 2.0 for Typeform API Access
Typeform uses OAuth 2.0 to secure its API endpoints. To interact with the API, you need to generate an access token using your client credentials.
-
Authorization Request:
Direct users to the following URL to authorize your application:
https://api.typeform.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&scope=responses:read&response_type=code
Replace YOUR_CLIENT_ID and YOUR_REDIRECT_URI with your actual client ID and redirect URI.
-
Exchange Authorization Code for Access Token:
Once the user authorizes the app, you'll receive a temporary authorization code. Use this code to request an access token:
fetch('https://api.typeform.com/oauth/token', { method: 'POST', headers: { 'Content-Type': 'application/x-www-form-urlencoded' }, body: new URLSearchParams({ grant_type: 'authorization_code', code: 'AUTHORIZATION_CODE', client_id: 'YOUR_CLIENT_ID', client_secret: 'YOUR_CLIENT_SECRET', redirect_uri: 'YOUR_REDIRECT_URI' }) }) .then(response => response.json()) .then(data => console.log(data));
Replace AUTHORIZATION_CODE, YOUR_CLIENT_ID, YOUR_CLIENT_SECRET, and YOUR_REDIRECT_URI with your actual values.
For more detailed information on setting up OAuth 2.0 with Typeform, refer to the official documentation: Typeform Applications and OAuth 2.0 Scopes.
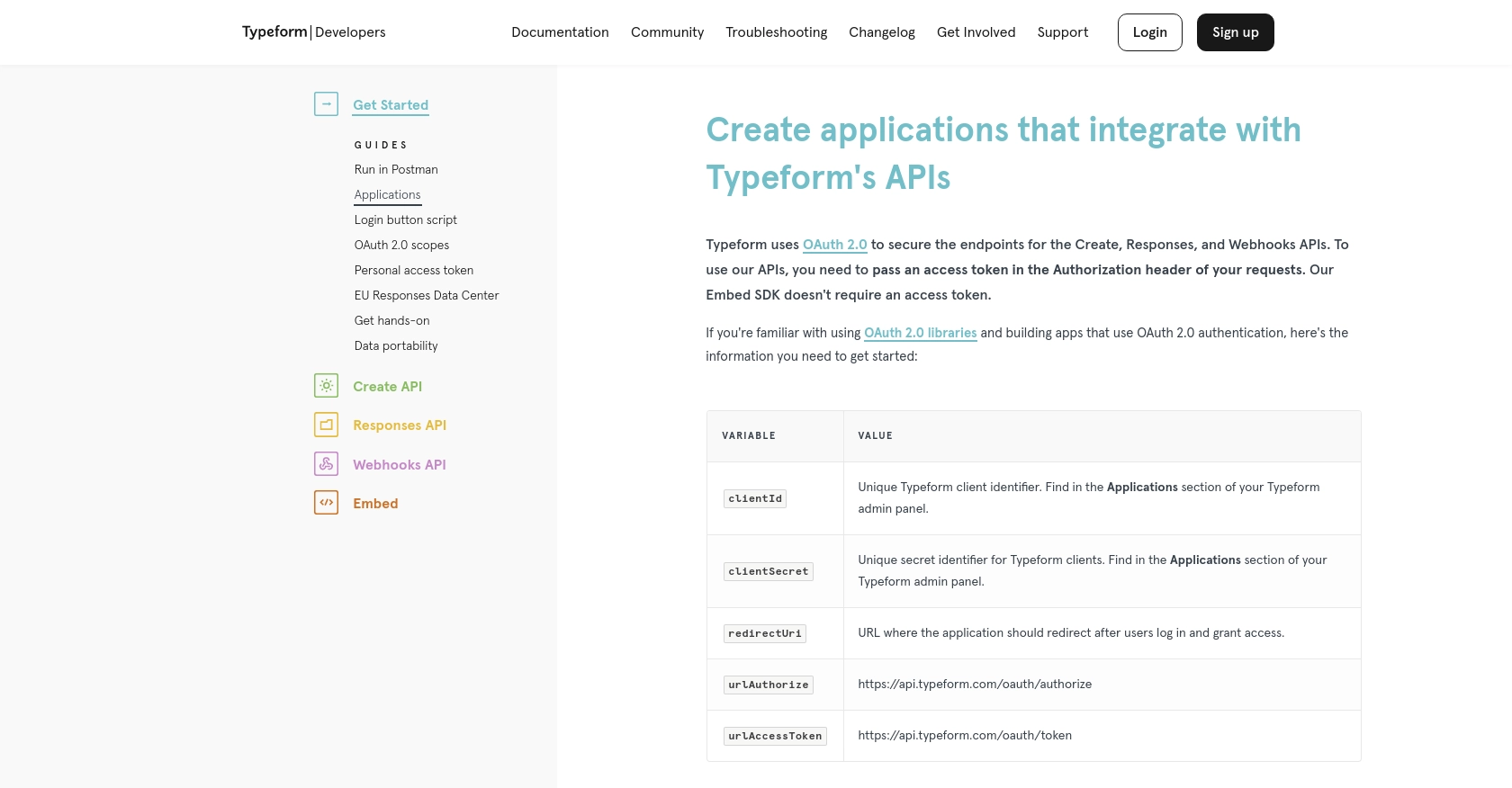
sbb-itb-96038d7
Making API Calls to Retrieve Typeform Responses Using JavaScript
Now that you have set up your Typeform application and obtained the necessary OAuth 2.0 credentials, it's time to make API calls to retrieve form responses using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Typeform API Integration
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or a browser-based setup. For this tutorial, we'll assume you're using Node.js.
-
Install Node.js:
Download and install Node.js from the official website if you haven't already.
-
Install Axios:
We'll use Axios, a promise-based HTTP client, to make API requests. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch Typeform Responses
With your environment set up, you can now write the JavaScript code to interact with the Typeform API and retrieve form responses.
const axios = require('axios');
// Replace with your actual access token and form ID
const accessToken = 'YOUR_ACCESS_TOKEN';
const formId = 'YOUR_FORM_ID';
// Set the API endpoint
const endpoint = `https://api.typeform.com/forms/${formId}/responses`;
// Make a GET request to the Typeform API
axios.get(endpoint, {
headers: {
'Authorization': `Bearer ${accessToken}`
}
})
.then(response => {
console.log('Form Responses:', response.data.items);
})
.catch(error => {
console.error('Error fetching responses:', error.response ? error.response.data : error.message);
});
Replace YOUR_ACCESS_TOKEN and YOUR_FORM_ID with your actual access token and form ID. This code sets up the API endpoint and makes a GET request to fetch the responses. If successful, it logs the responses to the console.
Handling Errors and Verifying API Call Success
It's crucial to handle errors gracefully and verify the success of your API calls. Here are some common error codes you might encounter:
- 400 Bad Request: Indicates a malformed request. Check your query parameters and request format.
- 401 Unauthorized: Indicates invalid or expired access token. Ensure your token is correct and hasn't expired.
- 405 Method Not Allowed: Indicates an incorrect HTTP method. Ensure you're using GET for retrieving responses.
- 500 Internal Server Error: Indicates a server-side issue. Try again later or contact Typeform support.
For more detailed error handling, refer to the Typeform API documentation.
By following these steps, you can efficiently retrieve and manage Typeform responses using JavaScript, enhancing your application's data processing capabilities.
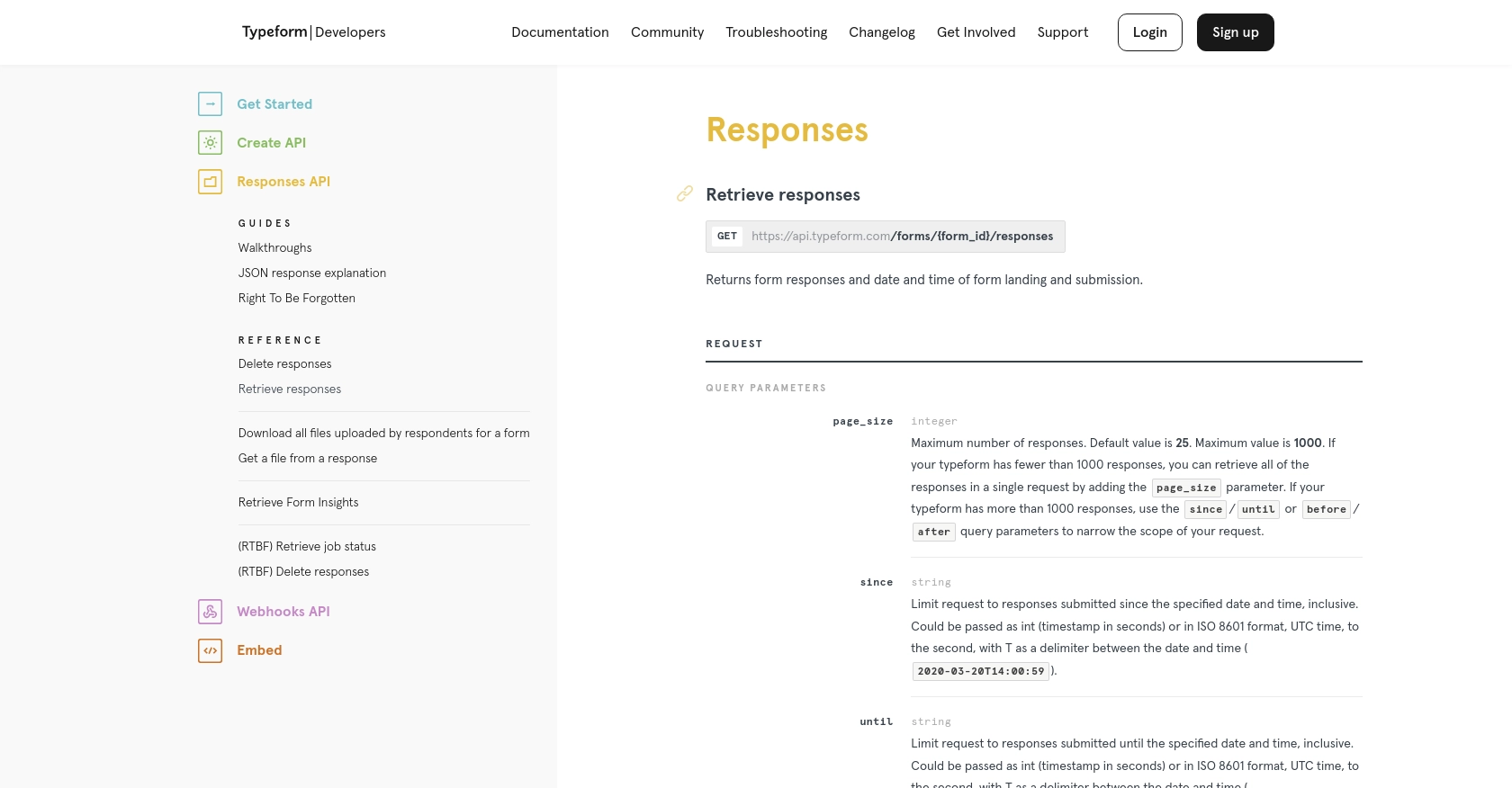
Conclusion and Best Practices for Typeform API Integration
Integrating with the Typeform API using JavaScript provides a powerful way to automate and streamline data collection processes. By following the steps outlined in this guide, you can efficiently retrieve and manage form responses, enhancing your application's data processing capabilities.
Best Practices for Secure and Efficient Typeform API Usage
- Secure Storage of Credentials: Always store your client_id, client_secret, and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Typeform's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Typeform is transformed and standardized to fit your application's data model, improving consistency and usability.
- Regular Token Refresh: Implement a mechanism to refresh access tokens regularly to maintain uninterrupted access to the Typeform API.
Streamline Your Integrations with Endgrate
While integrating with Typeform is a valuable endeavor, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Typeform. By using Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your users.
Explore how Endgrate can help you save time and resources by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?