How to Get Vendors with the Sage 100 API in PHP
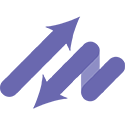
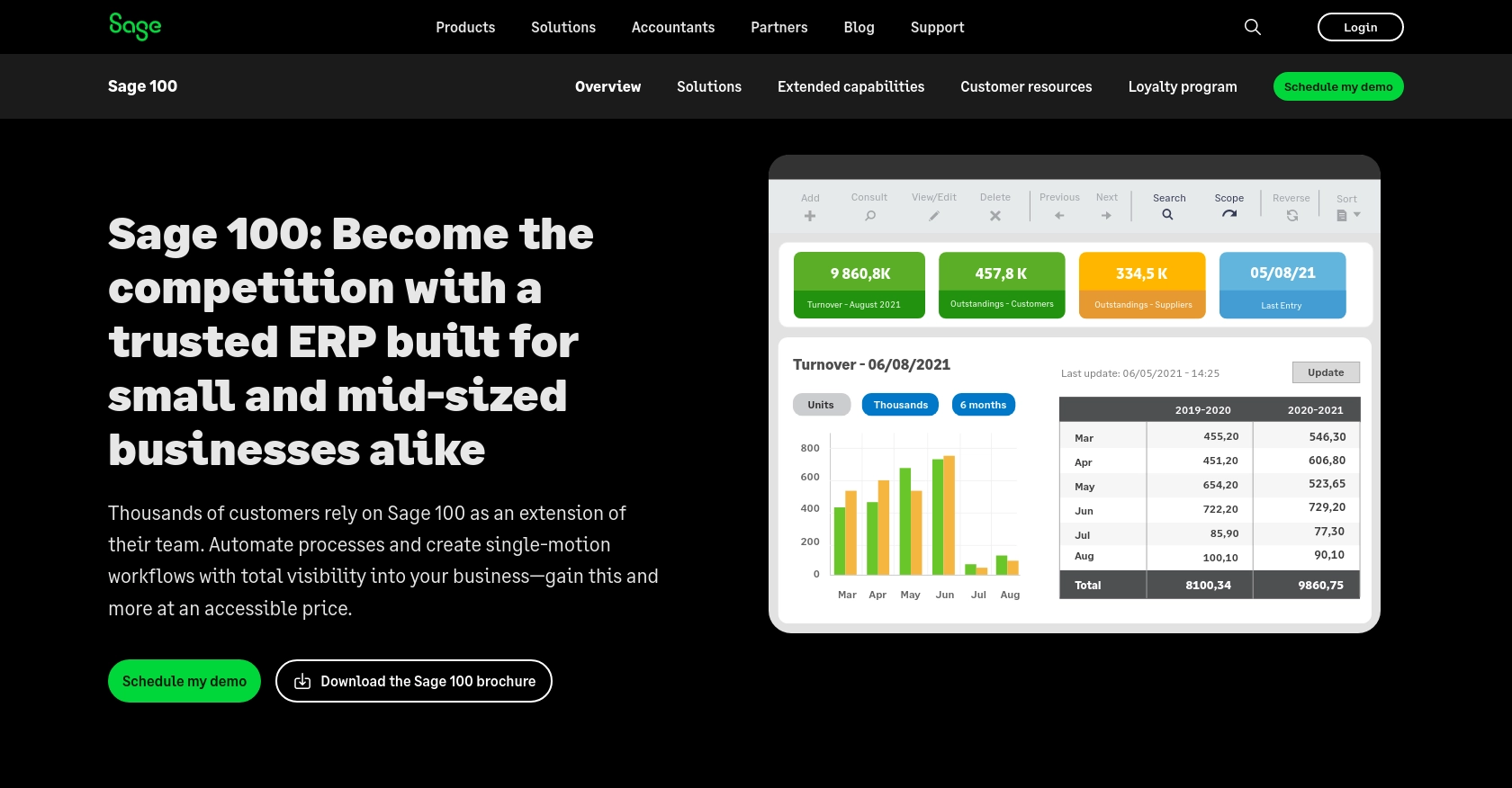
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution tailored for small to medium-sized businesses, offering robust features for accounting, inventory management, and customer relationship management. Its flexibility and scalability make it a popular choice for businesses looking to streamline their operations.
Integrating with the Sage 100 API allows developers to automate and enhance various business processes. For example, accessing vendor information through the Sage 100 API can help streamline procurement workflows, enabling businesses to efficiently manage vendor relationships and transactions.
This article will guide you through the process of retrieving vendor data using the Sage 100 API with PHP, providing a step-by-step approach to setting up and executing API calls effectively.
Setting Up Your Sage 100 Test Account for API Integration
Before you can start interacting with the Sage 100 API using PHP, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data, ensuring a smooth development process.
Installing and Configuring the Sage 100 ODBC Driver
To connect to the Sage 100 database, you need to install and configure the Sage 100 ODBC driver. Follow these steps to set up the driver:
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Refer to the official Sage 100 documentation for detailed instructions on configuring the ODBC driver.
Creating a Sage 100 Test Environment
Once the ODBC driver is configured, you can set up a test environment:
- Ensure you have access to a Sage 100 test server or request a sandbox account from your Sage 100 administrator.
- Verify that the test environment mirrors your production setup to accurately test API interactions.
Configuring Authentication for Sage 100 API Access
Sage 100 uses a custom authentication method. Follow these steps to configure authentication:
- In the Sage 100 Advanced server, open the Server Manager and configure the Sage 100 Client Server ODBC Driver Service.
- Ensure the service is set to start automatically and uses a domain administrator-level account for login.
- Test the ODBC connection to confirm successful configuration.
With your Sage 100 test account and ODBC driver configured, you're ready to start making API calls using PHP. This setup ensures a robust environment for developing and testing your integration solutions.
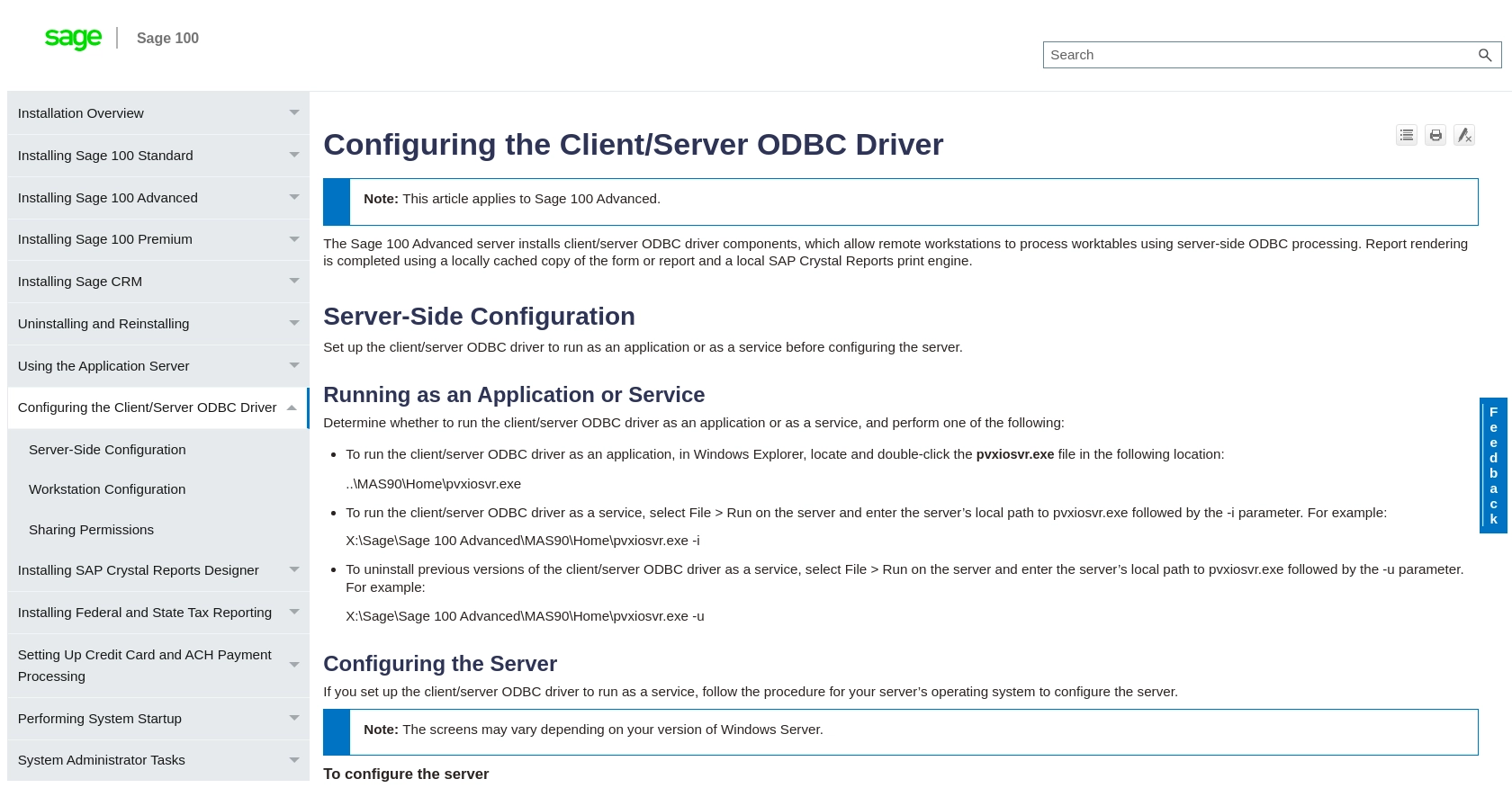
sbb-itb-96038d7
Making API Calls to Retrieve Vendor Data from Sage 100 Using PHP
To interact with the Sage 100 API and retrieve vendor data, you'll need to use PHP to establish a connection and execute the necessary API calls. This section will guide you through the process, including setting up your PHP environment and writing the code to fetch vendor information.
Setting Up Your PHP Environment for Sage 100 API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Install PHP 7.4 or later on your system.
- Ensure the ODBC extension is enabled in your
php.ini
file. You can do this by uncommenting the lineextension=odbc
. - Restart your web server to apply the changes.
Writing PHP Code to Connect to Sage 100 and Retrieve Vendor Data
With your environment set up, you can now write the PHP code to connect to the Sage 100 database and retrieve vendor data:
<?php
// Define the DSN and credentials
$dsn = 'DSN=Sage100DSN';
$username = 'your_username';
$password = 'your_password';
// Establish the ODBC connection
$conn = odbc_connect($dsn, $username, $password);
if (!$conn) {
die('Connection failed: ' . odbc_errormsg());
}
// Define the SQL query to retrieve vendor data
$query = "SELECT VendorNo, VendorName, AddressLine1, City, State, ZipCode FROM AP_Vendor";
// Execute the query
$result = odbc_exec($conn, $query);
if (!$result) {
die('Query failed: ' . odbc_errormsg());
}
// Fetch and display the vendor data
while ($row = odbc_fetch_array($result)) {
echo "Vendor No: " . $row['VendorNo'] . "<br>";
echo "Vendor Name: " . $row['VendorName'] . "<br>";
echo "Address: " . $row['AddressLine1'] . ", " . $row['City'] . ", " . $row['State'] . " " . $row['ZipCode'] . "<br><br>";
}
// Close the connection
odbc_close($conn);
?>
In this code, we start by defining the Data Source Name (DSN) and credentials required to connect to the Sage 100 database. We then establish an ODBC connection using odbc_connect()
. If the connection is successful, we execute a SQL query to fetch vendor data from the AP_Vendor
table. The results are displayed in a readable format, and finally, the connection is closed using odbc_close()
.
Verifying Successful API Calls and Handling Errors
After running the PHP script, verify the output to ensure the vendor data is correctly retrieved. If you encounter any errors, check the following:
- Ensure the DSN, username, and password are correct.
- Verify that the Sage 100 ODBC driver is properly configured.
- Check for any syntax errors in your SQL query.
Handling errors gracefully is crucial. Use try-catch blocks to manage exceptions and provide meaningful error messages to help diagnose issues quickly.
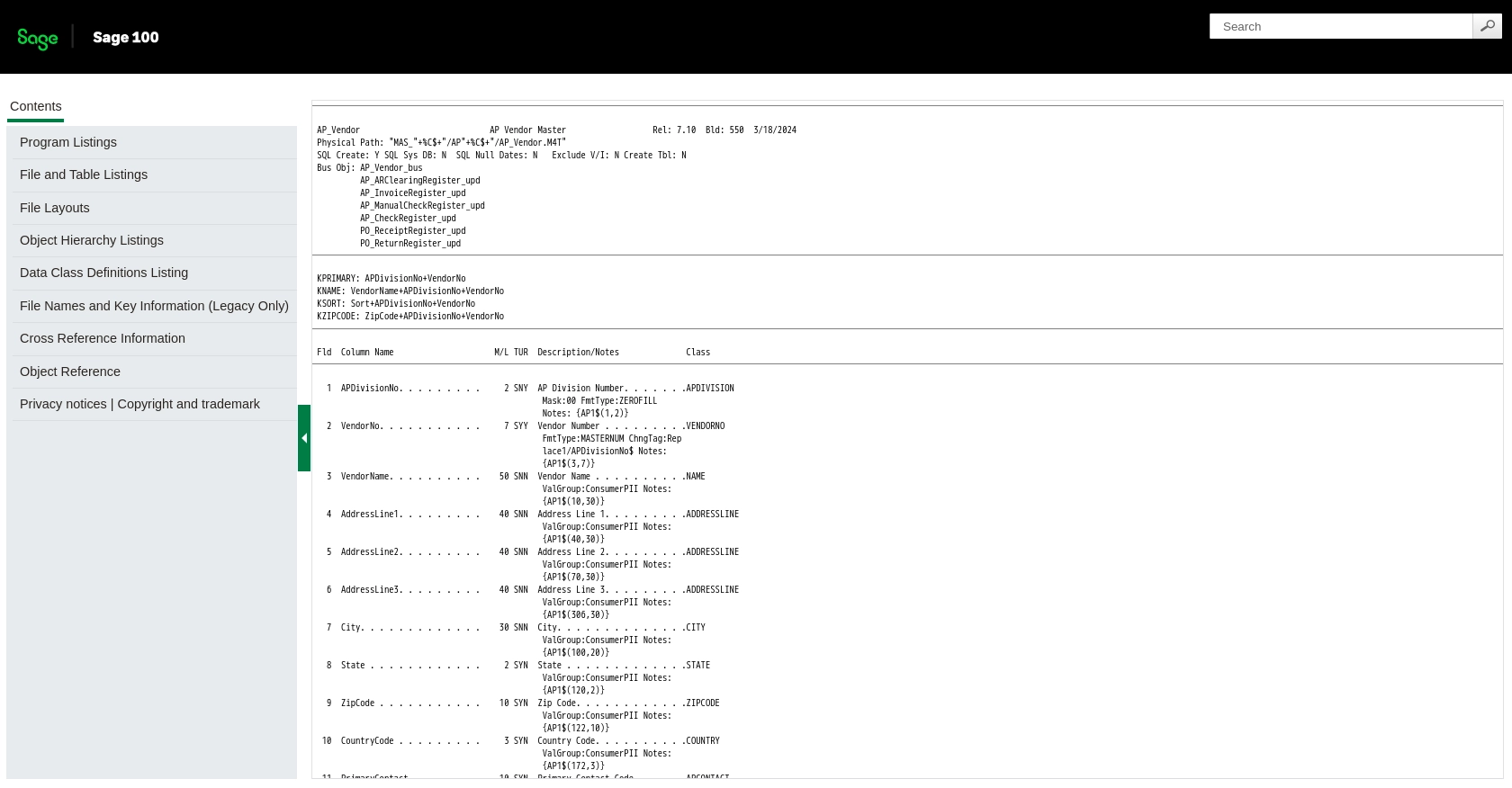
Conclusion: Best Practices for Sage 100 API Integration in PHP
Integrating with the Sage 100 API using PHP can significantly enhance your business processes by automating data retrieval and management tasks. To ensure a successful integration, consider the following best practices:
Securely Storing Sage 100 User Credentials
Always store user credentials securely. Use environment variables or secure vaults to manage sensitive information like usernames and passwords. Avoid hardcoding credentials directly in your code.
Handling Sage 100 API Rate Limiting and Errors
While Sage 100 documentation does not specify rate limits, it's essential to implement error handling and retry logic to manage potential API call failures. Use try-catch blocks to handle exceptions and provide informative error messages for troubleshooting.
Standardizing and Transforming Vendor Data
When retrieving vendor data, ensure that the data is standardized and transformed to meet your application's requirements. This might involve formatting addresses or normalizing vendor names for consistency across your system.
Leverage Endgrate for Streamlined Integrations
Consider using Endgrate to simplify your integration process. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Sage 100, offering an intuitive integration experience for your customers.
By following these best practices, you can ensure a robust and efficient integration with the Sage 100 API, enhancing your business operations and vendor management capabilities.
Read More
Ready to get started?