Using the Sugar Market API to Create Or Update Contacts (with Python examples)
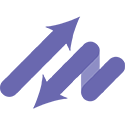
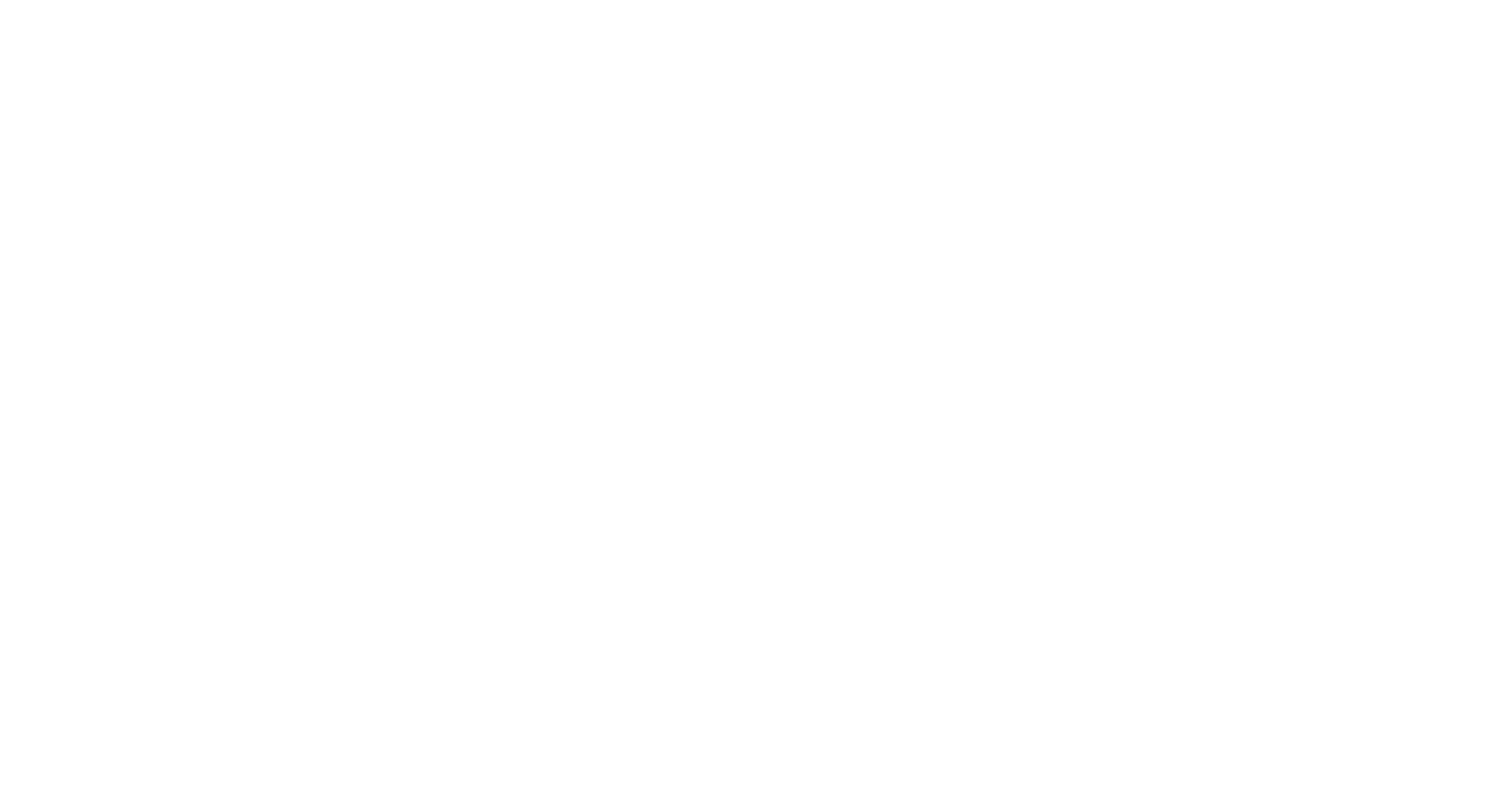
Introduction to Sugar Market API
Sugar Market, part of the SugarCRM suite, is a powerful marketing automation platform designed to help businesses streamline their marketing efforts. It offers a range of features including email marketing, lead nurturing, and campaign management, making it an essential tool for businesses looking to enhance their marketing strategies.
Developers may want to integrate with the Sugar Market API to automate and manage contact data efficiently. For example, using the Sugar Market API, a developer can create or update contact information directly from their application, ensuring that the marketing team always has access to the most current data.
This article will guide you through using Python to interact with the Sugar Market API, specifically focusing on creating or updating contacts. By following this tutorial, you will learn how to leverage the API to enhance your marketing automation processes.
Setting Up Your Sugar Market Test/Sandbox Account
Before you can start interacting with the Sugar Market API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting any live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or demo account on the Sugar Market website. Follow the instructions provided to create your account and gain access to the platform's features.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the integrations or developer tools menu.
- Create a new API application by providing the necessary details such as application name and description.
- Once the application is created, you will receive a client ID and client secret. Make sure to store these securely as they will be used for authenticating your API requests.
Configuring OAuth for Sugar Market API
To interact with the Sugar Market API, you'll need to configure OAuth authentication. Follow these steps to set up OAuth:
- In your application settings, locate the OAuth configuration section.
- Enter the redirect URI that your application will use to handle the OAuth callback.
- Save the configuration and note the authorization URL provided by Sugar Market.
With your API credentials and OAuth configuration in place, you're ready to start making API calls to Sugar Market. In the next section, we'll explore how to use Python to create or update contacts using the Sugar Market API.
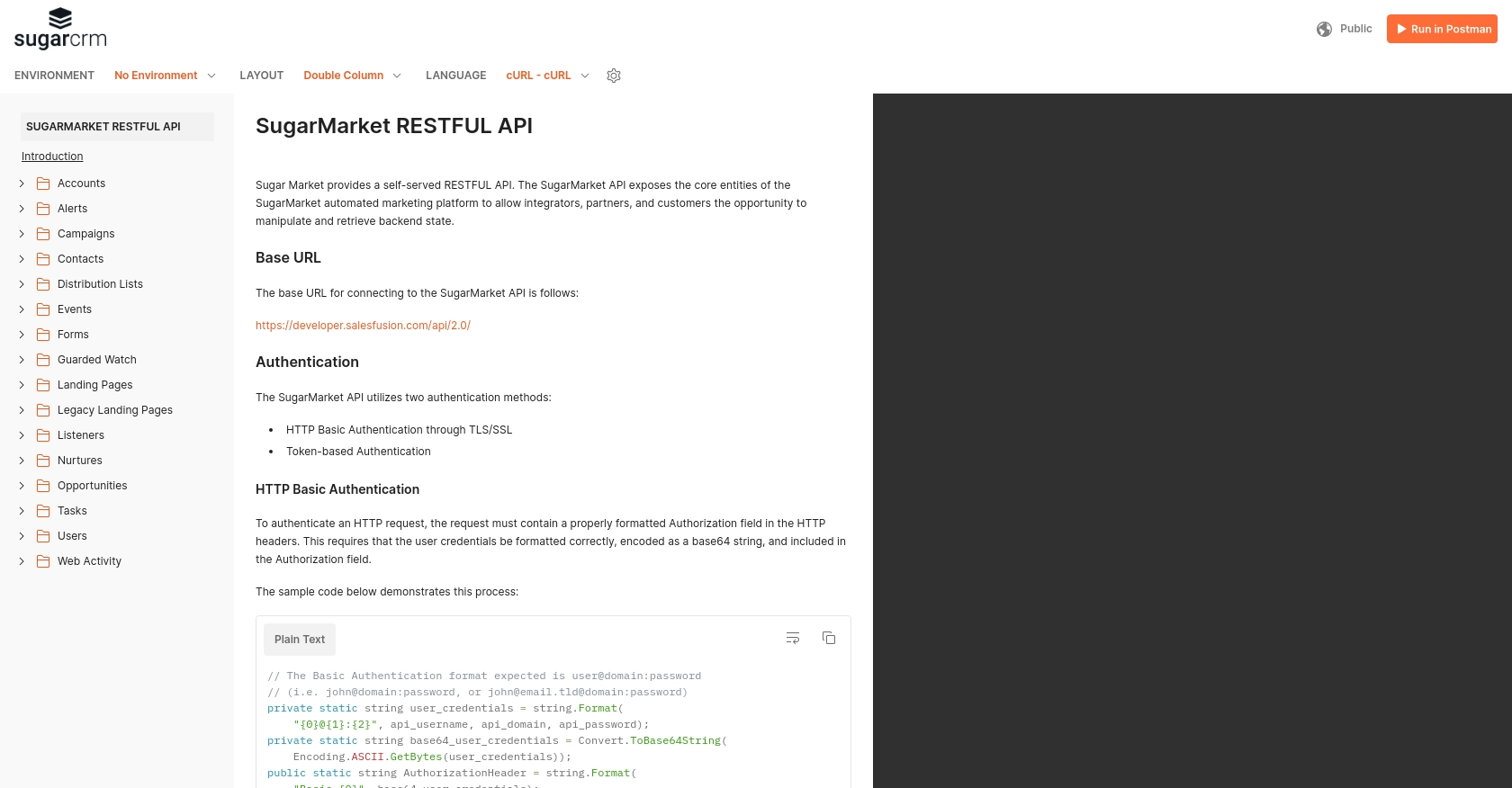
sbb-itb-96038d7
Making API Calls to Sugar Market Using Python
To interact with the Sugar Market API for creating or updating contacts, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Sugar Market API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
pip install requests
Creating or Updating Contacts with Sugar Market API
Let's create a Python script to interact with the Sugar Market API. This example demonstrates how to create or update a contact.
import requests
# Define the API endpoint and headers
url = "https://api.sugarmarket.com/v1/contacts"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
# Define the contact data
contact_data = {
"email": "example@sugarmarket.com",
"firstName": "John",
"lastName": "Doe"
}
# Send a POST request to create or update the contact
response = requests.post(url, json=contact_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Contact created or updated successfully.")
else:
print(f"Failed to create or update contact. Status code: {response.status_code}")
Replace YOUR_ACCESS_TOKEN
with the token obtained from your Sugar Market account. This script sends a POST request to the Sugar Market API to create or update a contact with the specified email, first name, and last name.
Verifying API Call Success in Sugar Market
After running the script, you can verify the success of the API call by checking the contact list in your Sugar Market sandbox account. If the contact appears with the provided details, the API call was successful.
Handling Errors and Error Codes in Sugar Market API
When making API calls, it's crucial to handle potential errors. The Sugar Market API may return various status codes indicating success or failure. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Ensure your code checks the response status and handles errors appropriately to provide a seamless user experience.
For more detailed information on error codes, refer to the Sugar Market API documentation.
Conclusion and Best Practices for Using Sugar Market API with Python
Integrating with the Sugar Market API allows developers to efficiently manage contact data, enhancing marketing automation processes. By following this guide, you have learned how to create or update contacts using Python, ensuring that your marketing team has access to the most current information.
Best Practices for Secure and Efficient Sugar Market API Integration
- Securely Store Credentials: Always store your API credentials, such as client ID and client secret, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sugar Market API to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized and validated before sending them to the API. This helps maintain data integrity and reduces the risk of errors.
- Implement Error Handling: Properly handle API errors by checking response status codes and implementing appropriate error messages or fallback mechanisms.
Enhance Your Integration Strategy with Endgrate
While integrating with individual APIs like Sugar Market is beneficial, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Sugar Market.
With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case instead of multiple times for different integrations, and offer your customers an intuitive integration experience.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate today.
Read More
Ready to get started?