How to Get Notes with the Endear API in PHP
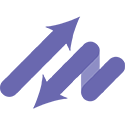
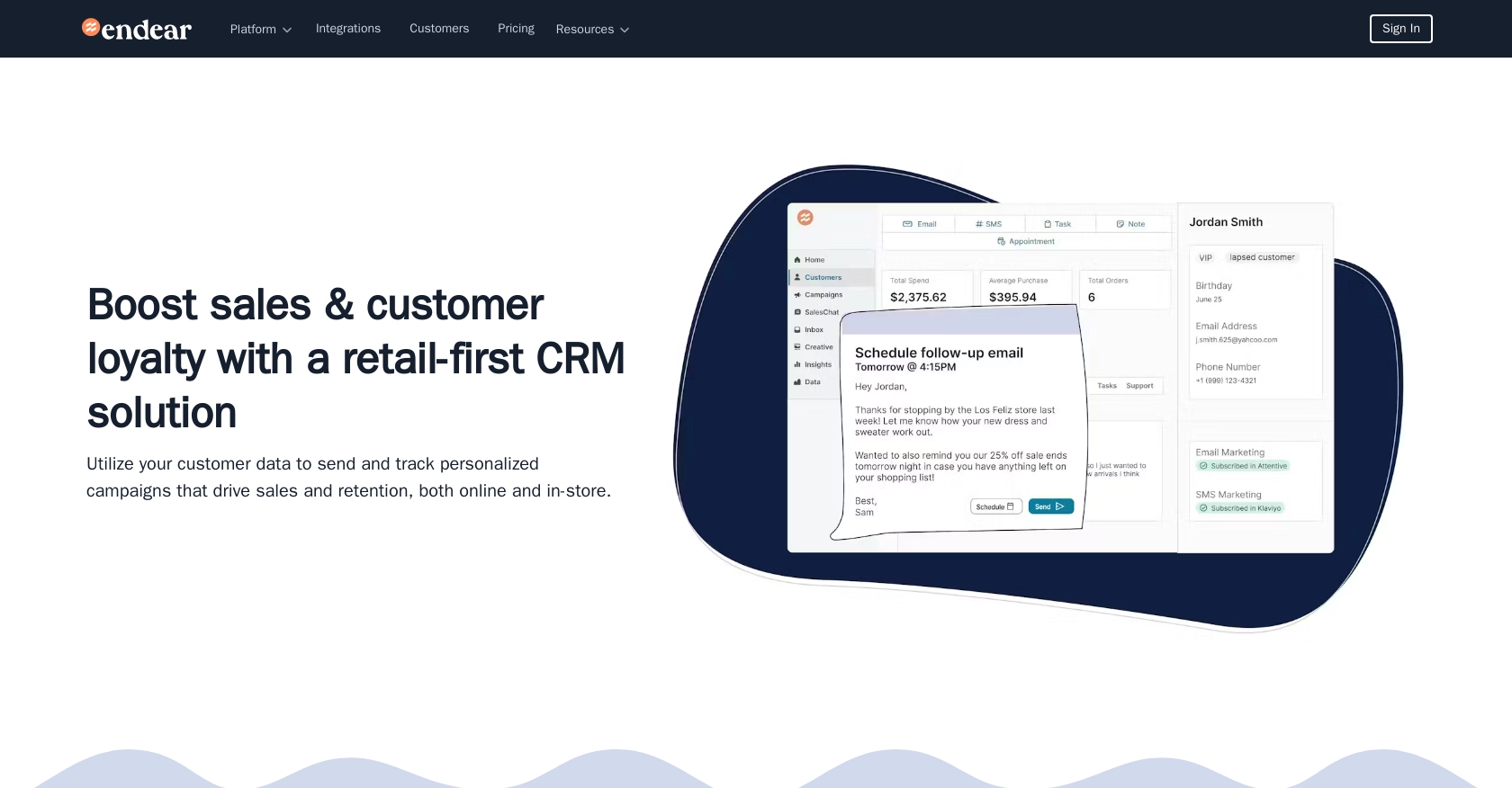
Introduction to Endear API for Note Management
Endear is a powerful CRM platform designed to enhance customer engagement and streamline communication for businesses. It offers a robust suite of tools that allow companies to manage customer interactions, track sales, and analyze data effectively.
Developers may want to integrate with Endear's API to access and manage notes, which are crucial for maintaining detailed records of customer interactions. For example, a developer might use the Endear API to retrieve notes related to customer feedback, enabling the company to tailor its services based on customer insights.
This article will guide you through the process of using PHP to interact with the Endear API, specifically focusing on retrieving notes. By following this tutorial, you'll learn how to efficiently access and manage notes within the Endear platform using PHP.
Setting Up Your Endear Test/Sandbox Account for API Access
Before you can start interacting with the Endear API to retrieve notes, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating an Endear Account
If you don't already have an Endear account, follow these steps to create one:
- Visit the Endear signup page.
- Fill in the required details to create your account.
- Once your account is set up, log in to access the dashboard.
Generating an API Key for Endear Integration
To authenticate your API requests, you'll need to generate an API key. Follow these steps to obtain your API key:
- Navigate to the settings section of your Endear account.
- Click on Integrations and then select Add Integration.
- Choose API from the options provided.
- Fill in the necessary details and generate your API key.
- Make sure to copy and securely store your API key, as you'll need it for authentication.
For more detailed instructions, refer to the Endear authentication documentation.
Authenticating API Requests with Your API Key
Once you have your API key, you can authenticate your API requests by including it in the request headers. Here's how you can do it:
// Set the API endpoint and headers
$endpoint = "https://api.endearhq.com/graphql";
$headers = [
"Content-Type: application/json",
"X-Endear-Api-Key: YOUR_API_KEY"
];
// Example of making a POST request
$options = [
'http' => [
'header' => $headers,
'method' => 'POST',
'content' => json_encode(['query' => 'query { currentIntegration { id } }'])
]
];
$context = stream_context_create($options);
$result = file_get_contents($endpoint, false, $context);
Replace YOUR_API_KEY
with the API key you generated earlier. This setup will allow you to authenticate and interact with the Endear API effectively.
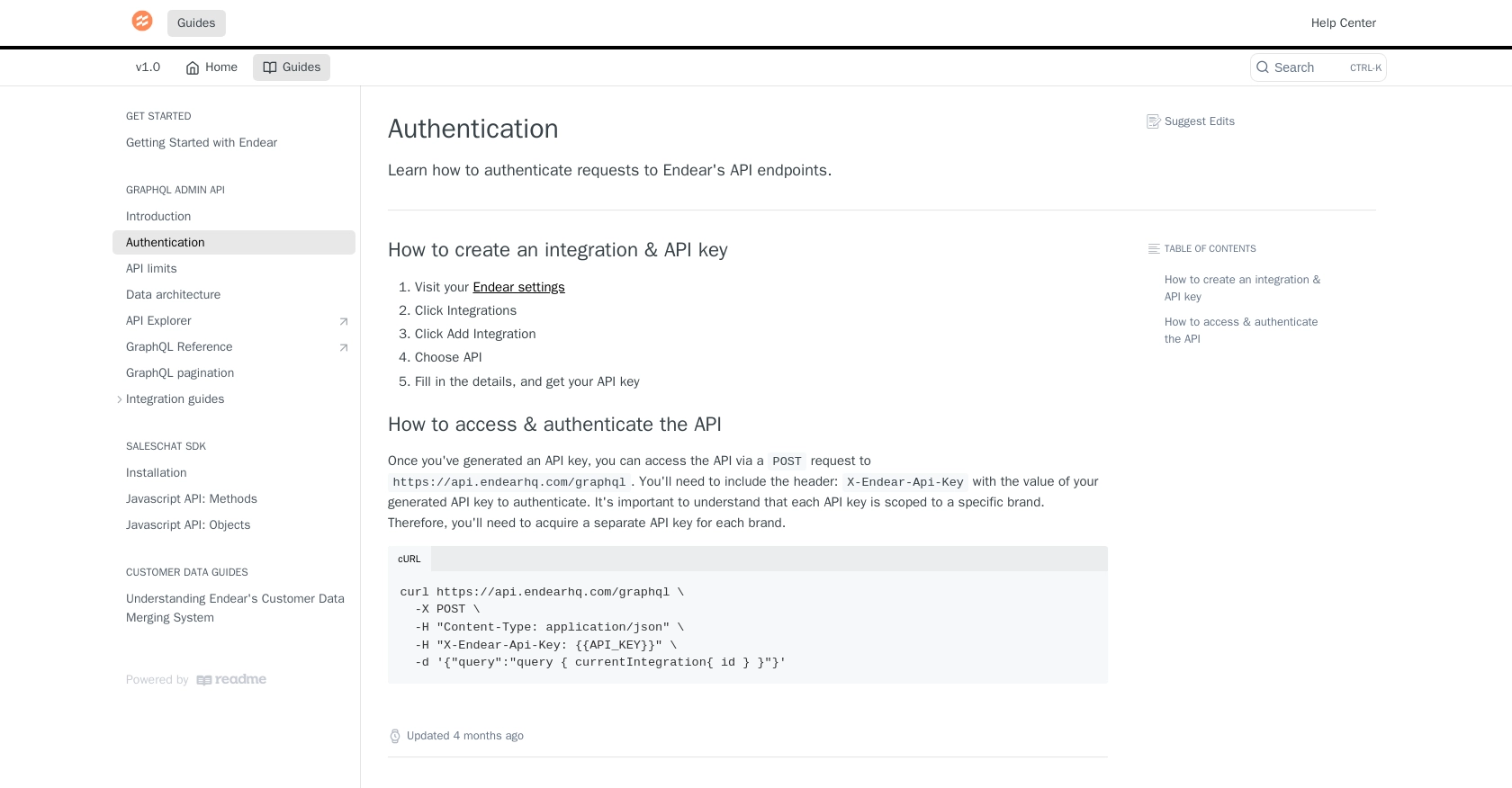
sbb-itb-96038d7
Making API Calls to Retrieve Notes with the Endear API in PHP
To interact with the Endear API and retrieve notes, you'll need to make a POST request to the GraphQL endpoint. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Endear API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL
extension enabled. You can verify this by running the following command:
php -v
To install the cURL
extension, use the following command:
sudo apt-get install php-curl
Installing Required PHP Dependencies
For making HTTP requests, we'll use the GuzzleHTTP
library. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Notes from Endear
Now, let's write the PHP code to make a POST request to the Endear API and retrieve notes. Create a file named get_notes.php
and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle HTTP client
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://api.endearhq.com/graphql';
$headers = [
'Content-Type' => 'application/json',
'X-Endear-Api-Key' => 'YOUR_API_KEY'
];
// Define the GraphQL query
$query = [
'query' => '{
searchNotes {
edges {
node {
id
description
title
creator {
full_name
}
}
}
pageInfo {
endCursor
hasNextPage
}
}
}'
];
// Make the POST request
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $query
]);
// Parse the response
$data = json_decode($response->getBody(), true);
// Display the notes
foreach ($data['data']['searchNotes']['edges'] as $note) {
echo 'Note ID: ' . $note['node']['id'] . "\n";
echo 'Title: ' . $note['node']['title'] . "\n";
echo 'Description: ' . $note['node']['description'] . "\n";
echo 'Creator: ' . $note['node']['creator']['full_name'] . "\n\n";
}
Replace YOUR_API_KEY
with the API key you generated earlier. This script initializes a Guzzle client, sets up the necessary headers, and sends a POST request with the GraphQL query to retrieve notes.
Running the PHP Script and Verifying Results
Execute the script from the command line:
php get_notes.php
You should see the notes retrieved from your Endear account displayed in the terminal. Verify the results by checking the notes in your Endear dashboard.
Handling Errors and Understanding Endear API Rate Limits
It's important to handle potential errors when making API calls. The Endear API returns HTTP status codes to indicate success or failure. For example, a 429 status code indicates that you've hit the rate limit of 120 requests per minute. Implement error handling to manage these scenarios:
if ($response->getStatusCode() !== 200) {
echo 'Error: ' . $response->getStatusCode() . ' - ' . $response->getReasonPhrase();
exit;
}
For more details on rate limits and error codes, refer to the Endear rate limits and error codes documentation.
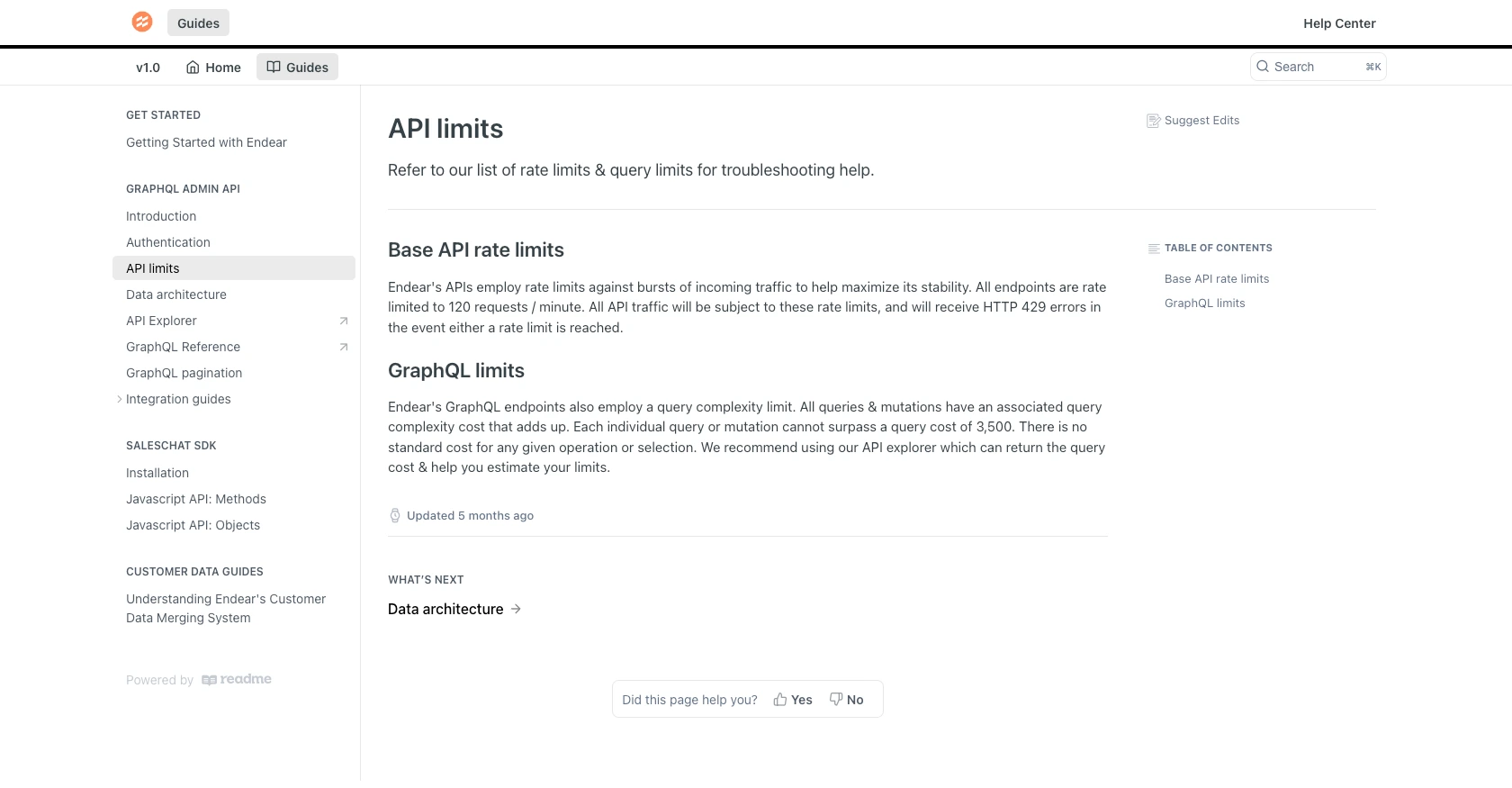
Conclusion and Best Practices for Using Endear API in PHP
Integrating with the Endear API to manage notes can significantly enhance your ability to maintain detailed records of customer interactions. By following the steps outlined in this article, you can efficiently retrieve notes using PHP, allowing your business to leverage customer insights for improved service delivery.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Implement Rate Limiting: Be mindful of Endear's rate limit of 120 requests per minute. Implement logic to handle HTTP 429 errors gracefully and retry requests as needed.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as necessary to fit your application's data model.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and ensure robust application performance.
Streamline Your Integrations with Endgrate
While integrating with the Endear API provides powerful capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Endear.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website today.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Note
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?