How to Create Notes with the Endear API in Python
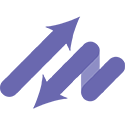
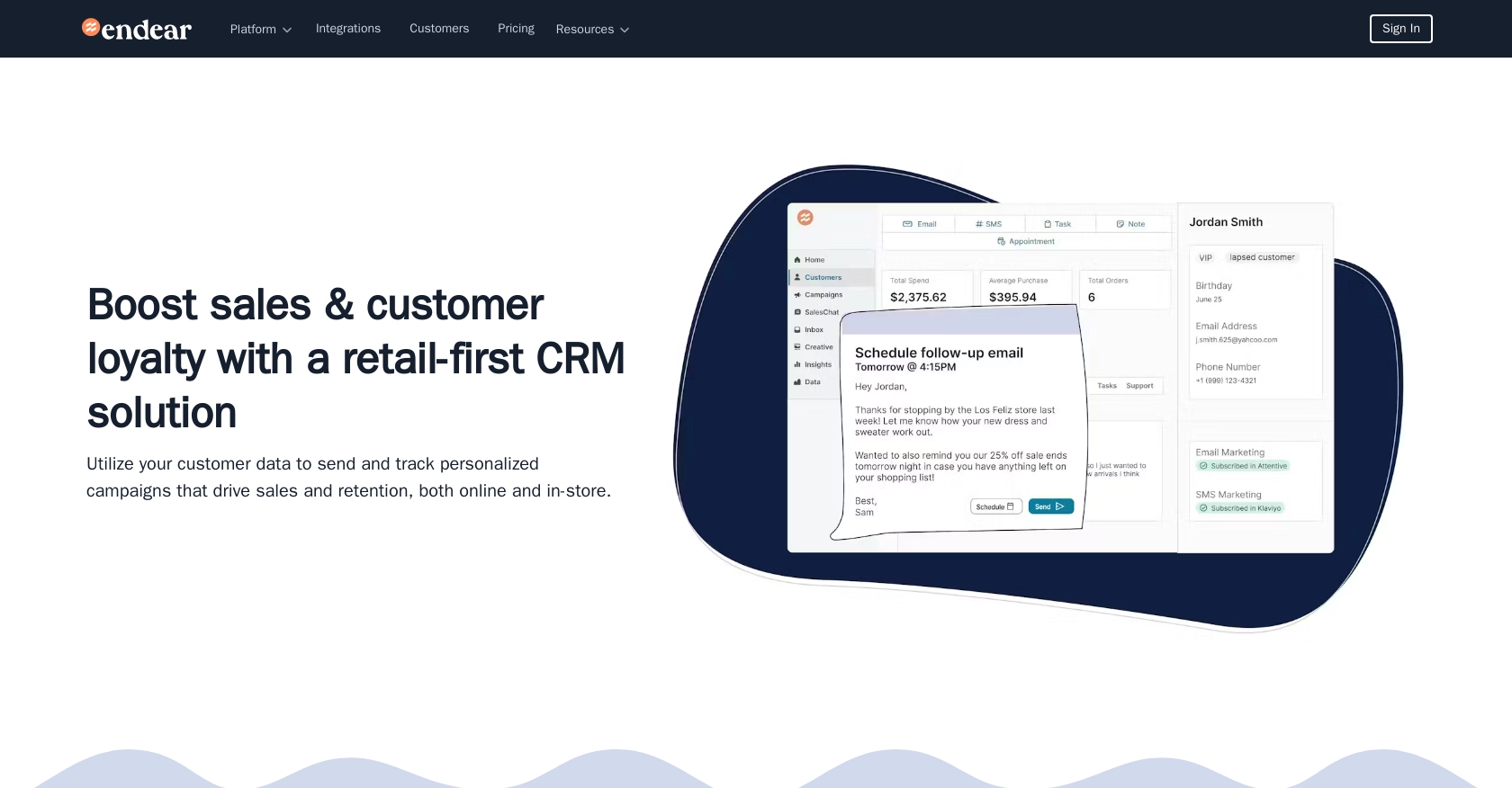
Introduction to Endear API for Creating Notes
Endear is a powerful CRM platform designed to enhance customer engagement and streamline communication for businesses. It offers a comprehensive suite of tools that allow brands to manage customer interactions effectively, providing insights and personalized experiences.
Developers may want to integrate with Endear's API to automate and enhance their customer relationship management processes. For example, using the Endear API, developers can create notes associated with customer interactions, allowing teams to maintain detailed records of customer communications and preferences.
This article will guide you through the process of using Python to create notes with the Endear API, providing step-by-step instructions to help you seamlessly integrate this functionality into your application.
Setting Up Your Endear Test Account for API Integration
Before you can start creating notes with the Endear API, you need to set up a test account. This will allow you to safely experiment with the API without affecting live data. Follow these steps to get started:
Creating an Endear Account
If you don't already have an Endear account, you'll need to create one. Visit the Endear website and sign up for a free trial or demo account. This will give you access to the platform's features and allow you to explore its capabilities.
Generating an API Key for Endear
Once your account is set up, you'll need to generate an API key to authenticate your requests. Follow these steps:
- Navigate to your Endear account settings.
- Click on Integrations from the menu.
- Select Add Integration and choose API.
- Fill in the required details and submit the form to generate your API key.
Make sure to store your API key securely, as it will be used to authenticate your API requests.
Authenticating API Requests with Endear
With your API key in hand, you can now authenticate your API requests. Endear uses API key-based authentication, which requires you to include the key in the request headers. Here's how you can do it:
import requests
# Define the API endpoint and headers
url = "https://api.endearhq.com/graphql"
headers = {
"Content-Type": "application/json",
"X-Endear-Api-Key": "Your_API_Key"
}
# Example request payload
payload = {
"query": "query { currentIntegration { id } }"
}
# Make the POST request
response = requests.post(url, json=payload, headers=headers)
# Check the response
print(response.json())
Replace Your_API_Key
with the API key you generated earlier. This setup will allow you to authenticate and interact with the Endear API.
For more details on authentication, refer to the Endear Authentication Documentation.
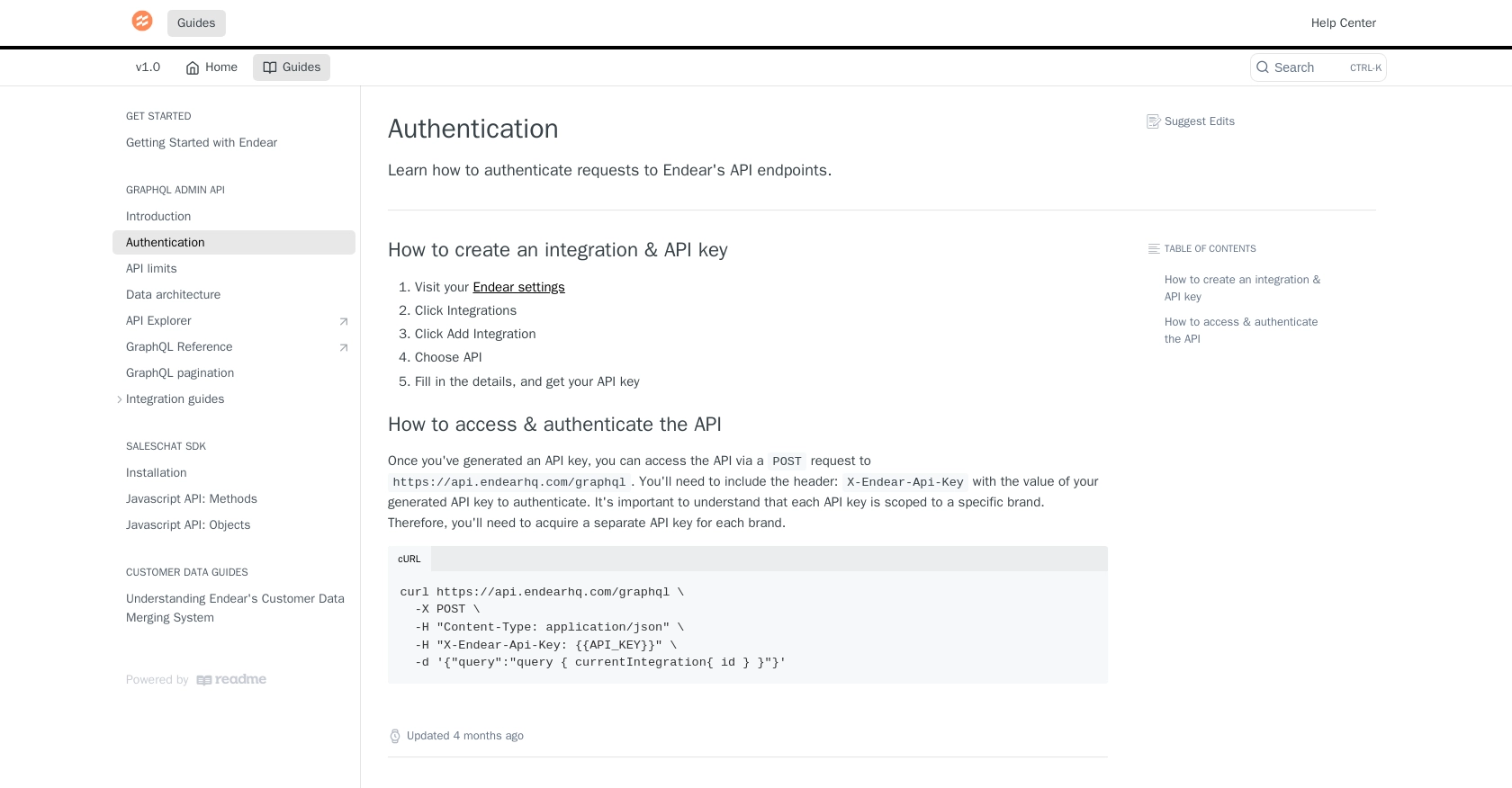
sbb-itb-96038d7
How to Make the Actual API Call to Create Notes with Endear API in Python
In this section, we'll walk through the process of making an API call to create notes using the Endear API with Python. This involves setting up your Python environment, writing the code to interact with the API, and handling the response.
Setting Up Your Python Environment for Endear API Integration
Before you start coding, ensure you have the necessary tools and libraries installed. You'll need:
- Python 3.11.1 or later
- The
requests
library for making HTTP requests
Install the requests
library using pip:
pip install requests
Writing Python Code to Create Notes with Endear API
Now, let's write the Python code to create a note using the Endear API. Create a file named create_endear_note.py
and add the following code:
import requests
# Define the API endpoint and headers
url = "https://api.endearhq.com/graphql"
headers = {
"Content-Type": "application/json",
"X-Endear-Api-Key": "Your_API_Key"
}
# Define the GraphQL mutation for creating a note
payload = {
"query": """
mutation {
createNote(input: {
note: {
title: "Meeting Notes",
content: "Discussed project milestones and deadlines.",
customerId: "123456"
}
}) {
note {
id
title
content
}
}
}
"""
}
# Make the POST request
response = requests.post(url, json=payload, headers=headers)
# Check the response
if response.status_code == 200:
print("Note Created Successfully:", response.json())
else:
print("Failed to Create Note:", response.status_code, response.text)
Replace Your_API_Key
with the API key you generated earlier. This code sends a GraphQL mutation to create a note with specified details.
Verifying the API Call and Handling Errors
After running the script, you should see a response indicating the note was created successfully. If the request fails, the script will print the error code and message.
To verify the note creation, you can check your Endear test account to ensure the note appears as expected.
Handle errors by checking the response status code. Common error codes include:
- 401 Unauthorized: Check your API key and authentication headers.
- 429 Too Many Requests: You've hit the rate limit of 120 requests per minute. Consider implementing a retry mechanism.
For more details on error codes, refer to the Endear Rate Limits and Error Codes Documentation.
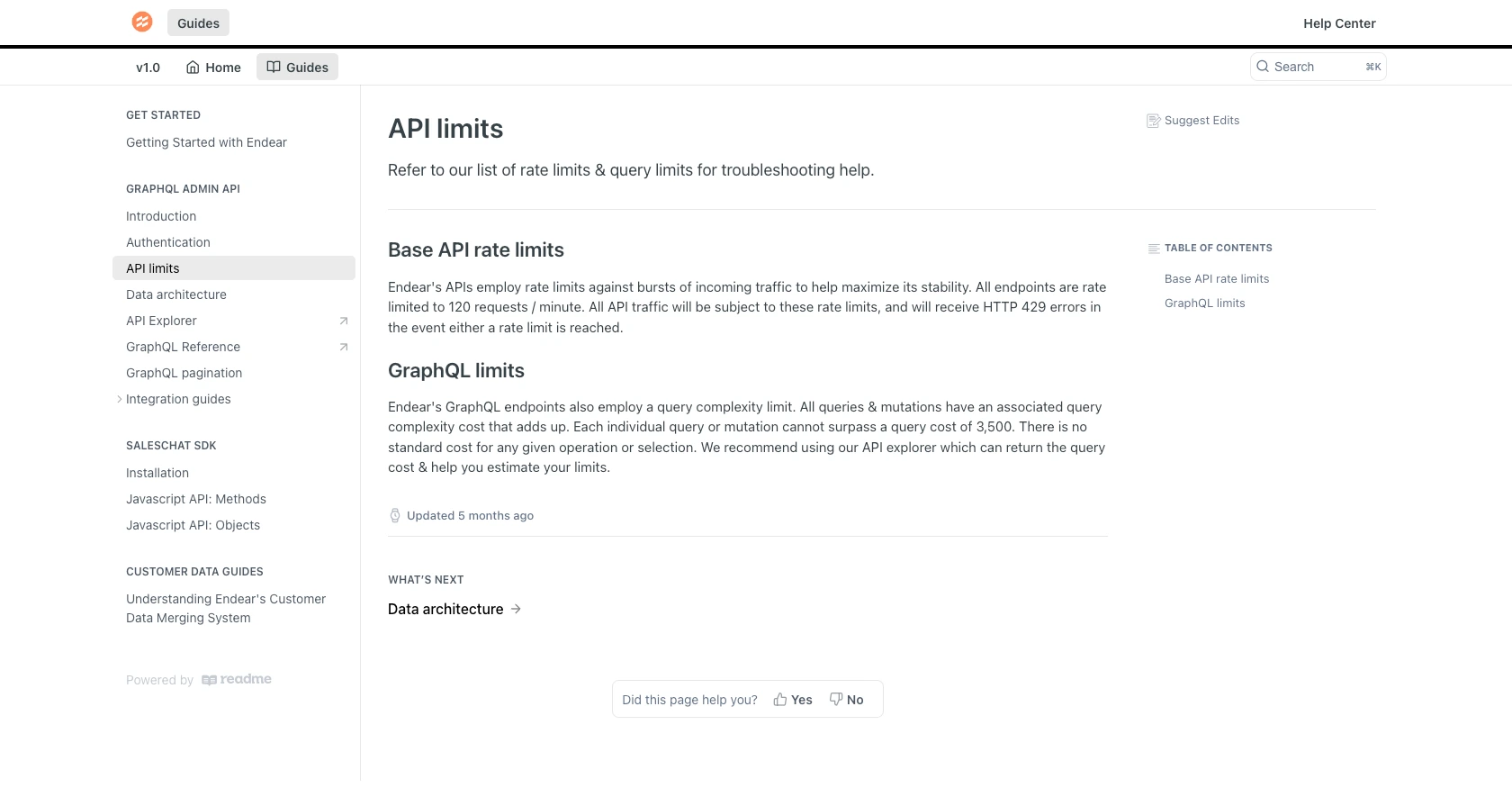
Conclusion and Best Practices for Using Endear API in Python
Integrating with the Endear API to create notes using Python can significantly enhance your customer relationship management processes. By automating note creation, you ensure that your team has access to detailed records of customer interactions, which can improve communication and customer satisfaction.
Best Practices for Secure and Efficient Endear API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Endear's rate limit of 120 requests per minute. Implement retry mechanisms and exponential backoff strategies to handle HTTP 429 errors gracefully.
- Data Standardization: Ensure that data fields are standardized across your application to maintain consistency and avoid data conflicts.
Enhance Your Integration Strategy with Endgrate
While integrating with Endear's API can be straightforward, managing multiple integrations across different platforms can be complex and time-consuming. This is where Endgrate can help.
Endgrate offers a unified API endpoint that simplifies the integration process, allowing you to focus on your core product. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration strategy and save valuable time and resources.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Note
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?