Using the Re:amaze API to Get Conversations (with Python examples)
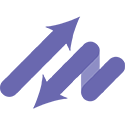
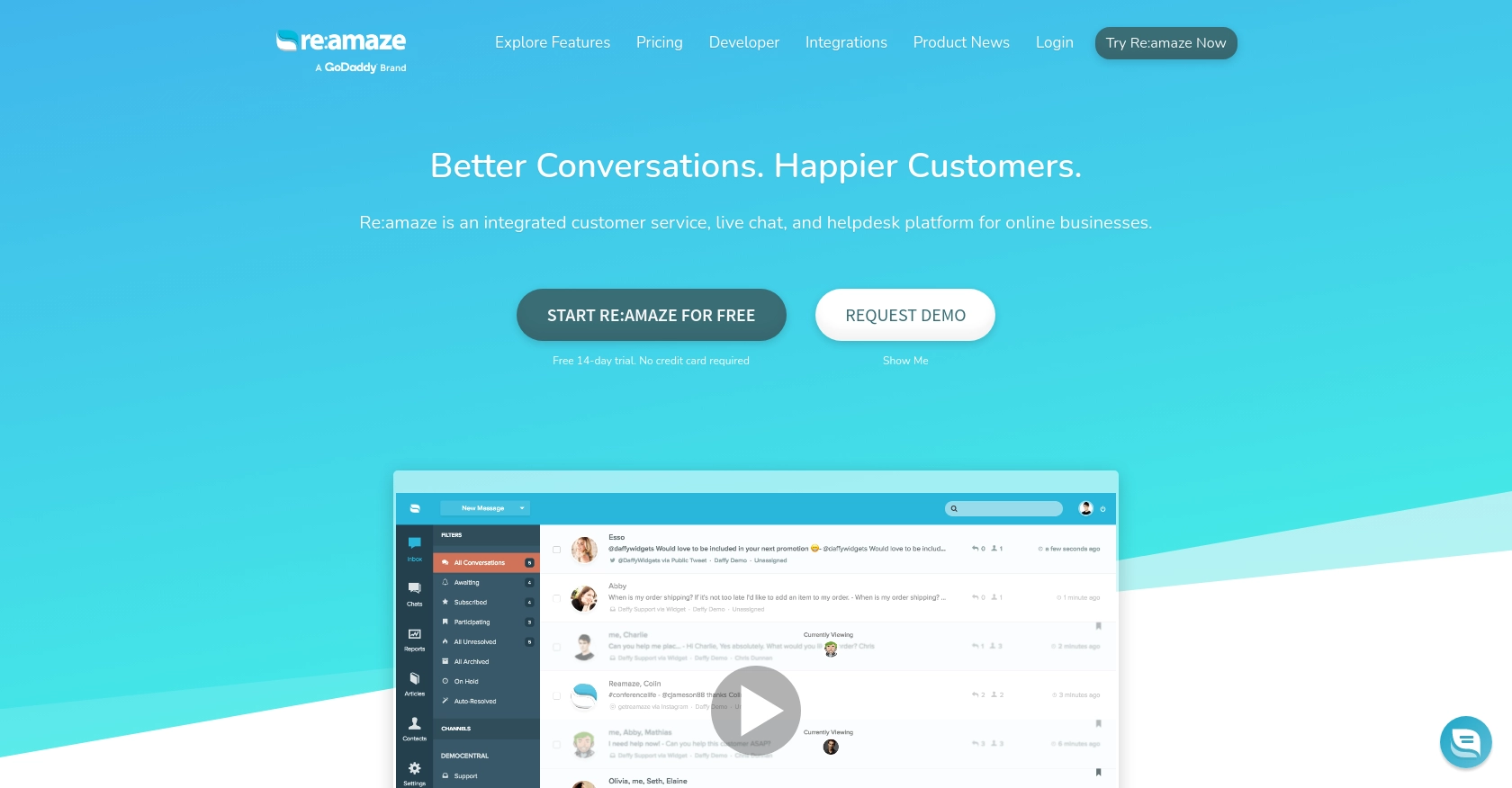
Introduction to Re:amaze API Integration
Re:amaze is a versatile customer support platform that offers a unified inbox for managing customer interactions across various channels, including email, chat, and social media. It is designed to enhance customer service efficiency and improve team collaboration through its robust set of features, such as live chat, automated messaging, and chatbots.
For developers, integrating with the Re:amaze API can streamline the process of accessing and managing customer conversations programmatically. This integration is particularly useful for automating support workflows, such as retrieving customer inquiries to analyze response times or integrating with other business tools for a seamless customer service experience.
In this article, we will explore how to use Python to interact with the Re:amaze API to retrieve conversations. This will enable developers to efficiently manage customer interactions and improve their support operations.
Setting Up Your Re:amaze Test Account for API Integration
Before diving into the Re:amaze API, it's essential to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Re:amaze offers a straightforward process to get started with their platform.
Creating a Free Re:amaze Account
To begin, you'll need to create a Re:amaze account. Follow these steps to set up your account:
- Visit the Re:amaze website and click on "Try Re:amaze Now" to sign up.
- Fill in the required information, such as your email and password, or use Google or Microsoft to sign up quickly.
- Once registered, you'll have access to the Re:amaze dashboard where you can explore its features.
Generating Your Re:amaze API Token
To interact with the Re:amaze API, you'll need an API token. This token authenticates your requests and allows you to access the API securely. Here's how to generate it:
- Log in to your Re:amaze account and navigate to the "Settings" section.
- Under the "Developer" menu, click on "API Token."
- Select "Generate New Token" to create a unique API token for your account.
- Copy and securely store this token, as you'll need it for API authentication.
Understanding Re:amaze API Authentication
The Re:amaze API uses HTTP Basic Auth for authentication. Ensure your requests are made over SSL/HTTPS for security. Include your login email and API token in the request headers to authenticate successfully.
import requests
# Define your Re:amaze brand and API token
brand = "your_brand"
api_token = "your_api_token"
login_email = "your_email"
# Example of setting up headers for authentication
headers = {
"Accept": "application/json"
}
# Example API call
response = requests.get(
f"https://{brand}.reamaze.io/api/v1/conversations",
headers=headers,
auth=(login_email, api_token)
)
print(response.json())
Replace your_brand
, your_api_token
, and your_email
with your actual Re:amaze brand, API token, and login email.
With your Re:amaze account and API token ready, you're all set to start making API calls and integrating Re:amaze into your applications.
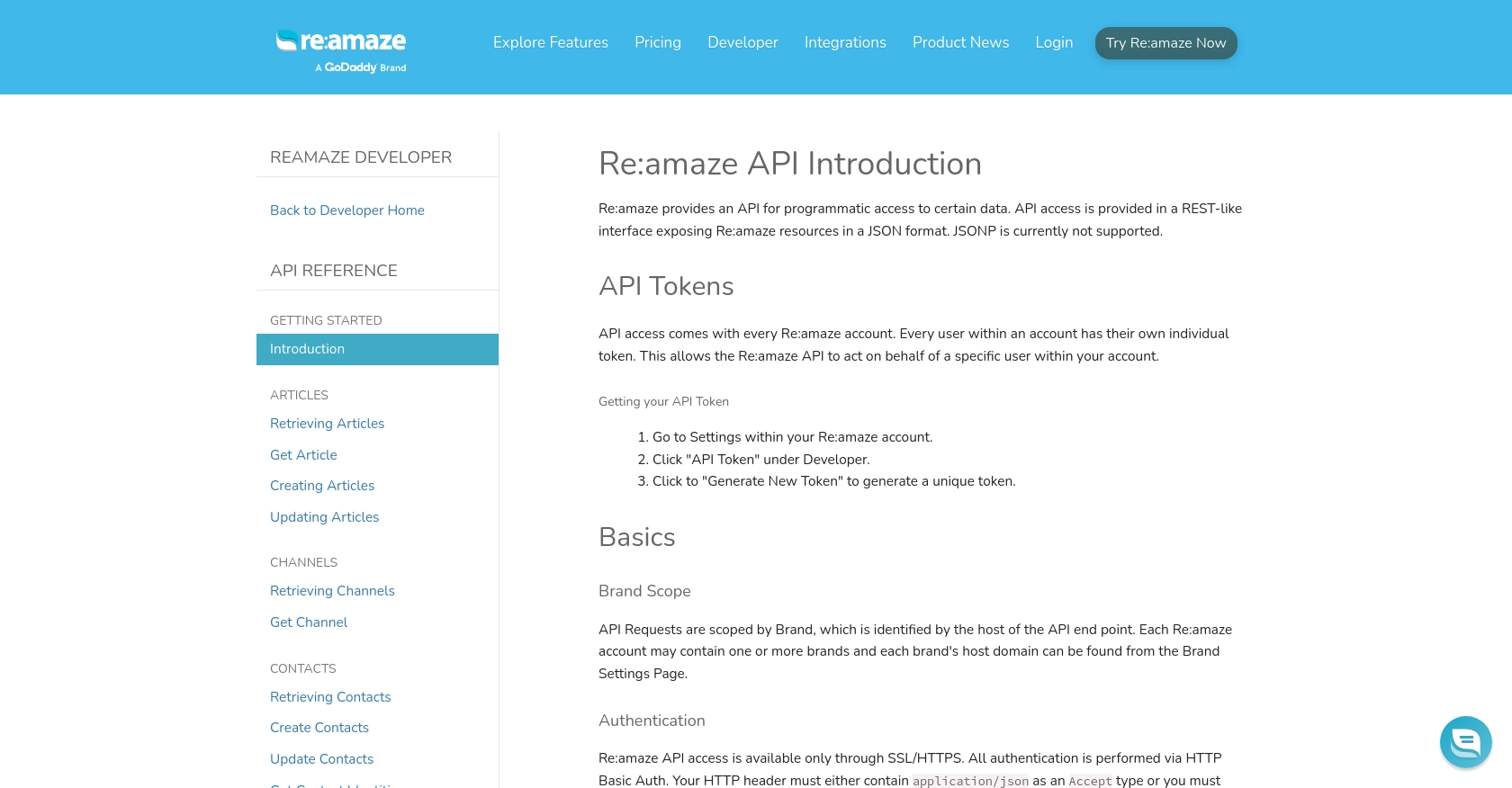
sbb-itb-96038d7
Executing API Calls to Retrieve Conversations from Re:amaze Using Python
Now that you have your Re:amaze account and API token set up, it's time to dive into making API calls to retrieve conversations. This section will guide you through the process of using Python to interact with the Re:amaze API, ensuring you can efficiently manage customer interactions.
Setting Up Your Python Environment for Re:amaze API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Making a GET Request to Retrieve Conversations from Re:amaze
With your environment ready, you can now write a Python script to fetch conversations from Re:amaze. Create a file named get_conversations.py
and add the following code:
import requests
# Define your Re:amaze brand, API token, and login email
brand = "your_brand"
api_token = "your_api_token"
login_email = "your_email"
# Set up the API endpoint and headers
endpoint = f"https://{brand}.reamaze.io/api/v1/conversations"
headers = {
"Accept": "application/json"
}
# Make a GET request to the Re:amaze API
response = requests.get(endpoint, headers=headers, auth=(login_email, api_token))
# Check if the request was successful
if response.status_code == 200:
conversations = response.json()
print("Conversations retrieved successfully:")
for conversation in conversations["conversations"]:
print(f"Subject: {conversation['subject']}, Status: {conversation['status']}")
else:
print(f"Failed to retrieve conversations. Status code: {response.status_code}")
Replace your_brand
, your_api_token
, and your_email
with your actual Re:amaze brand, API token, and login email.
Understanding the Response and Handling Errors
When you run the script, it will make a GET request to the Re:amaze API to fetch conversations. If successful, it will print the subject and status of each conversation. If the request fails, it will display the status code, helping you diagnose any issues.
Re:amaze API uses HTTP status codes to indicate success or failure. A status code of 200 indicates success, while a 429 status code means you've hit the rate limit. For more details, refer to the Re:amaze API documentation.
Verifying API Call Success in Re:amaze Dashboard
After running the script, you can verify the retrieved conversations by checking your Re:amaze dashboard. The data returned by the API should match the conversations visible in your account.
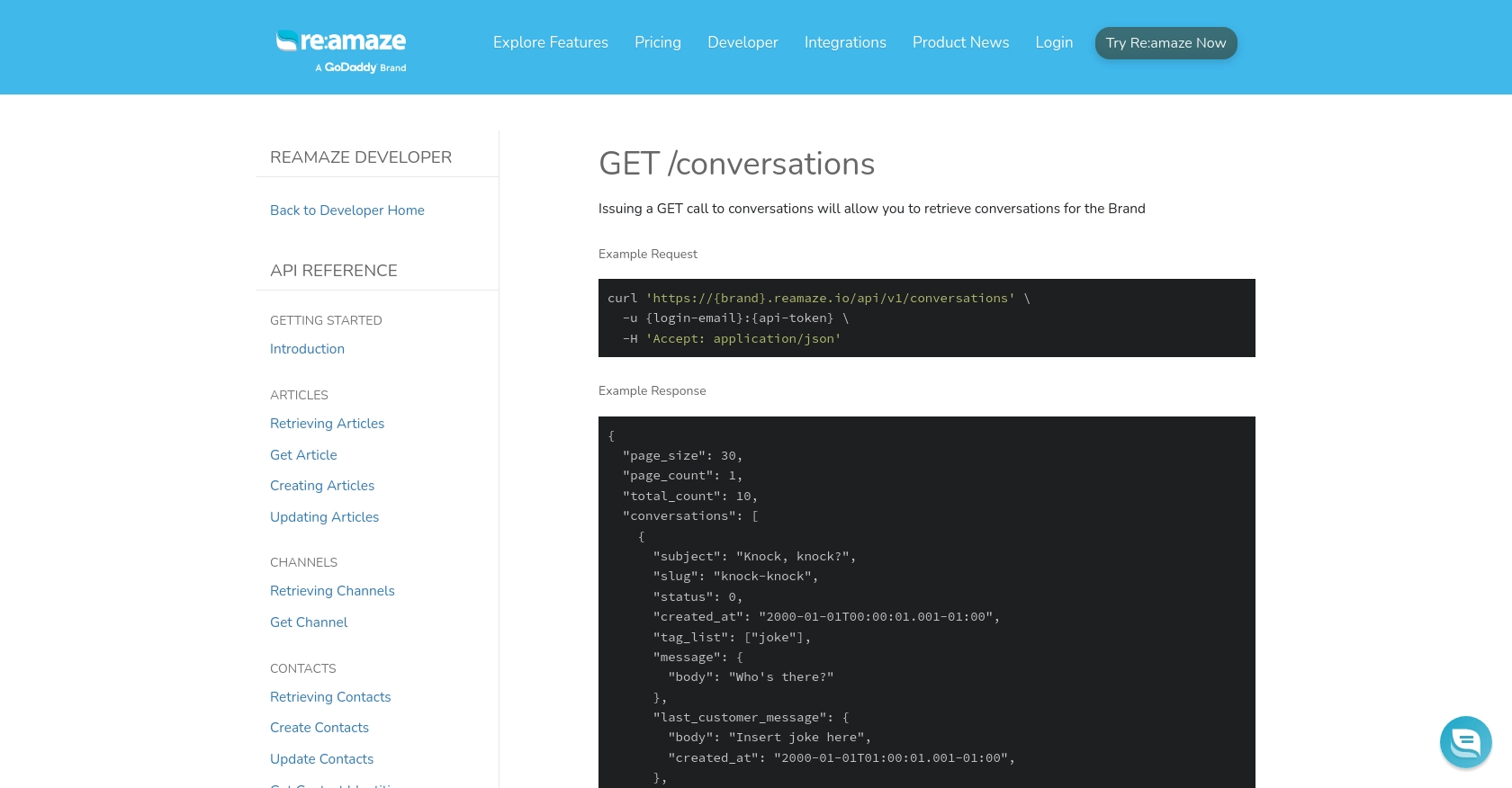
Best Practices for Using the Re:amaze API in Python
Integrating with the Re:amaze API can significantly enhance your customer support capabilities, but it's crucial to follow best practices to ensure a seamless experience. Here are some recommendations:
- Securely Store API Credentials: Always store your API token and login credentials securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: The Re:amaze API enforces rate limits to ensure fair usage. If you encounter a 429 status code, implement exponential backoff strategies to retry requests after a delay. For more details, refer to the Re:amaze API documentation.
- Standardize Data Fields: When retrieving conversations, ensure that data fields are standardized and transformed as needed for your application. This will help maintain consistency across different systems.
Enhancing Integration Efficiency with Endgrate
While integrating with the Re:amaze API directly can be beneficial, using a tool like Endgrate can further streamline the process. Endgrate allows you to manage multiple integrations through a single API endpoint, saving you time and resources.
- Focus on Core Product Development: By outsourcing integrations to Endgrate, you can concentrate on enhancing your core product offerings.
- Build Once, Deploy Anywhere: Endgrate enables you to create a single integration that works across various platforms, reducing the need for redundant development efforts.
- Provide a Seamless Experience: With Endgrate, you can offer your customers an intuitive and efficient integration experience, enhancing overall satisfaction.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
Ready to get started?