How to Get Companies with the FreshDesk API in Javascript
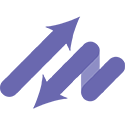
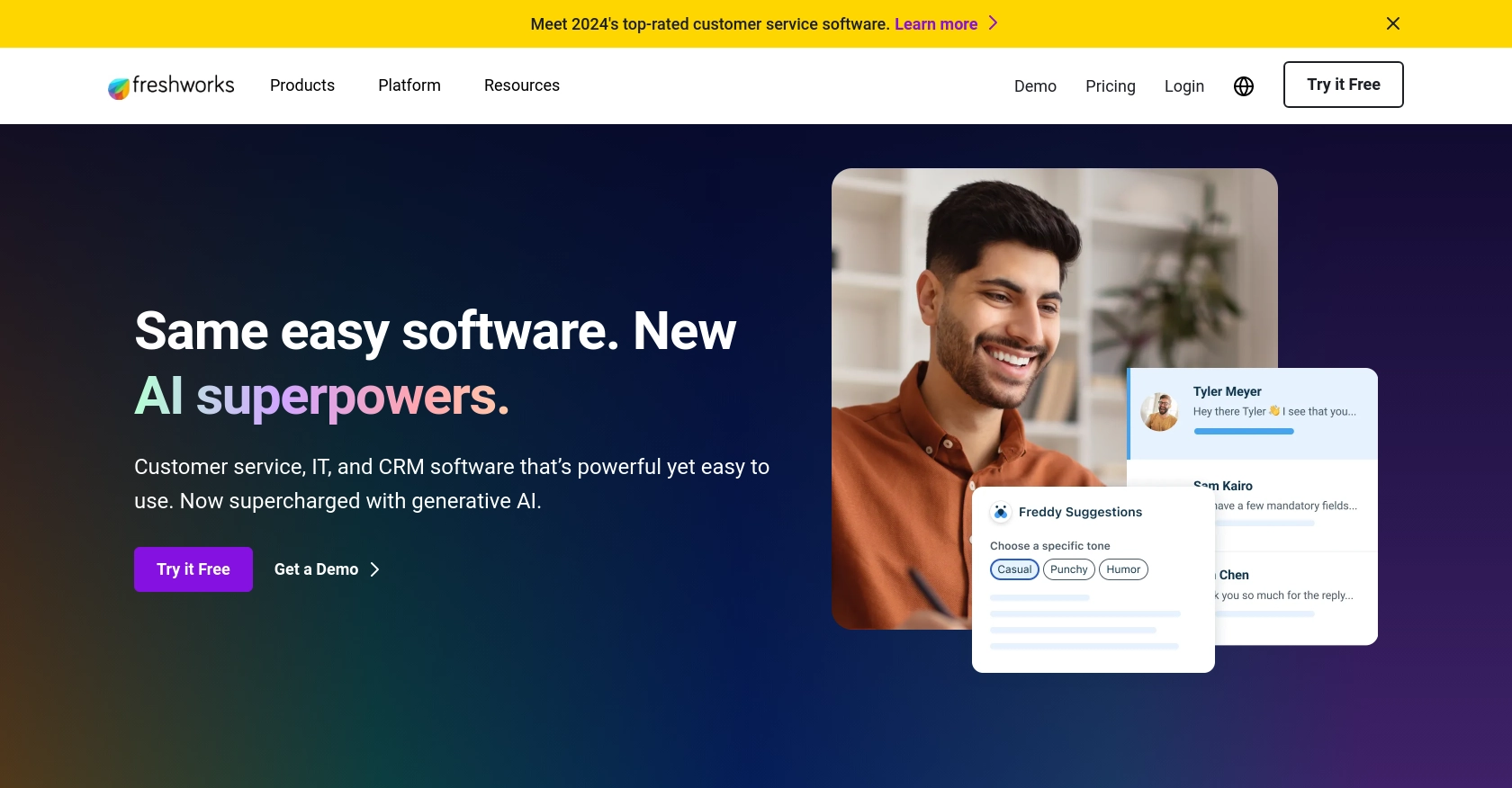
Introduction to FreshDesk API Integration
FreshDesk is a popular customer support software that provides businesses with a comprehensive suite of tools to manage customer interactions efficiently. It offers features such as ticketing, automation, and reporting, making it an essential platform for companies aiming to enhance their customer service experience.
Developers may want to integrate with FreshDesk's API to access and manage company data, enabling them to streamline operations and improve customer support workflows. For example, a developer might use the FreshDesk API to retrieve a list of companies associated with support tickets, allowing for better organization and prioritization of customer issues.
Setting Up Your FreshDesk Test/Sandbox Account
Before you can start integrating with the FreshDesk API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data.
Creating a FreshDesk Account
If you don't already have a FreshDesk account, you can sign up for a free trial on the FreshDesk website. This trial provides access to most features, allowing you to explore the platform and test API integrations.
- Visit the FreshDesk signup page.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating API Key for FreshDesk
FreshDesk uses a custom authentication method involving an API key. Here's how you can obtain it:
- Log in to your FreshDesk account.
- Navigate to your profile settings by clicking on your profile picture in the top right corner.
- Select "Profile Settings" from the dropdown menu.
- Scroll down to the "Your API Key" section and copy the API key provided.
Keep this API key secure, as it will be used to authenticate your API requests.
Setting Up a FreshDesk Sandbox Environment
While FreshDesk does not offer a dedicated sandbox environment, you can use your trial account to simulate a testing environment. Create sample data such as companies and tickets to test your API calls effectively.
- Create sample companies and tickets within your FreshDesk account.
- Use these samples to test API interactions without impacting real customer data.
With your FreshDesk account and API key ready, you're all set to start making API calls to retrieve company data using JavaScript.
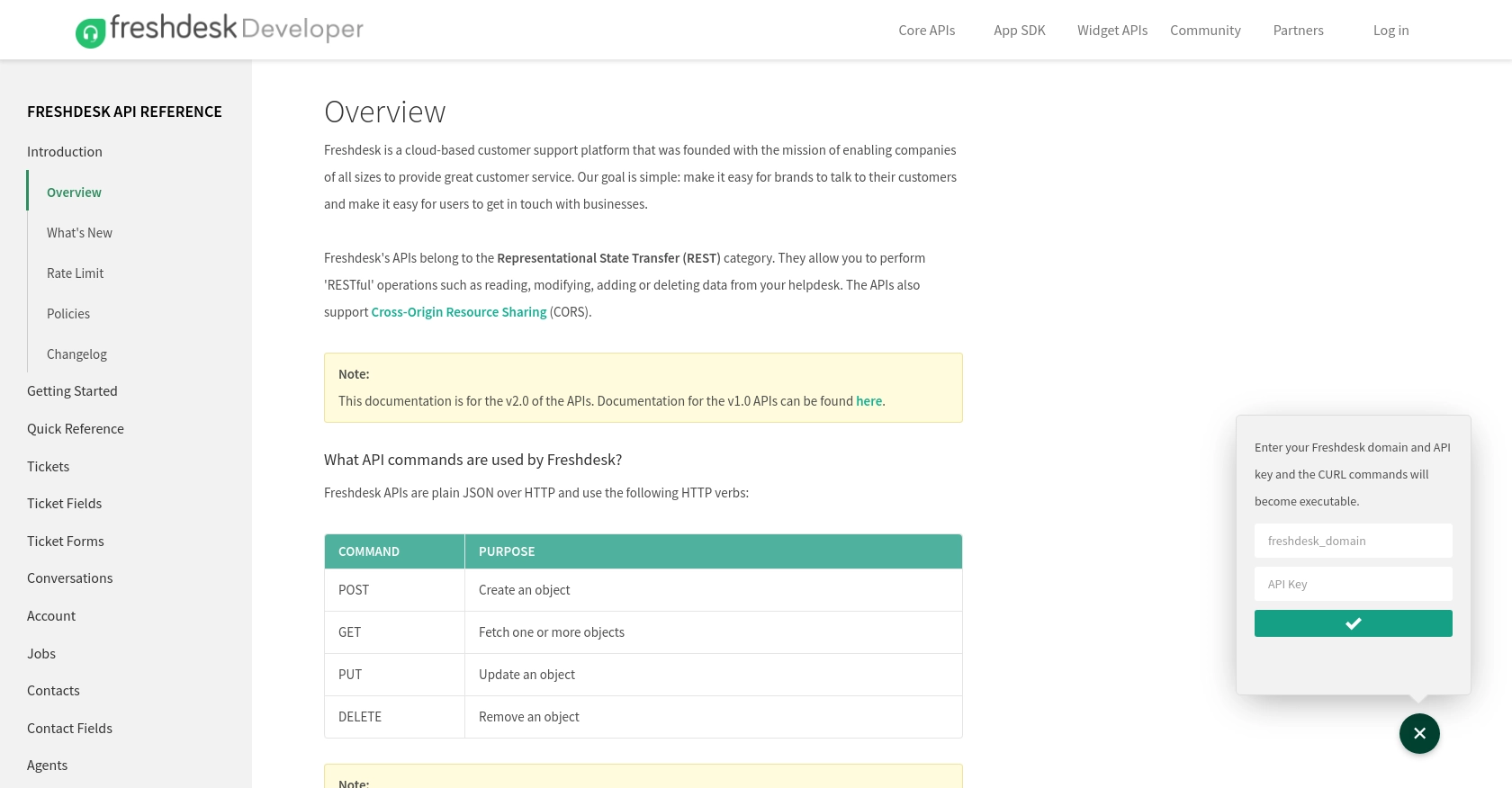
sbb-itb-96038d7
Making API Calls to Retrieve Companies with FreshDesk API in JavaScript
To interact with the FreshDesk API and retrieve company data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment and executing the necessary API calls.
Setting Up Your JavaScript Environment for FreshDesk API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor, such as Visual Studio Code, to write and execute your JavaScript code.
- The
axios
library for making HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Fetch Companies from FreshDesk
With your environment set up, you can now write the JavaScript code to retrieve companies from FreshDesk. Create a new file named getCompanies.js
and add the following code:
const axios = require('axios');
// Your FreshDesk domain and API key
const freshdeskDomain = 'yourdomain.freshdesk.com';
const apiKey = 'Your_API_Key';
// Set the API endpoint
const endpoint = `https://${freshdeskDomain}/api/v2/companies`;
// Make a GET request to the FreshDesk API
axios.get(endpoint, {
auth: {
username: apiKey,
password: 'X' // Password can be any character
}
})
.then(response => {
// Handle the response data
console.log('Companies:', response.data);
})
.catch(error => {
// Handle errors
console.error('Error fetching companies:', error.response ? error.response.data : error.message);
});
Replace Your_API_Key
with the API key you obtained from your FreshDesk account. The password field can be any character, as FreshDesk uses basic authentication with the API key as the username.
Executing and Verifying the API Call
To execute the code, run the following command in your terminal:
node getCompanies.js
If the request is successful, you should see a list of companies printed in the console. Verify the data by checking your FreshDesk account to ensure the companies match the retrieved data.
Handling Errors and Troubleshooting FreshDesk API Requests
When making API calls, it's essential to handle potential errors gracefully. The code above includes basic error handling, which logs the error message if the request fails. Common issues include incorrect API keys or network problems.
For more detailed error information, refer to the FreshDesk API documentation: FreshDesk API Documentation.
Conclusion and Best Practices for FreshDesk API Integration
Integrating with the FreshDesk API using JavaScript can significantly enhance your ability to manage and organize customer data efficiently. By retrieving company information, developers can streamline workflows and improve customer support operations.
Best Practices for Secure and Efficient FreshDesk API Usage
- Secure API Key Storage: Always store your FreshDesk API key securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of FreshDesk's API rate limits to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from FreshDesk is standardized and transformed as needed to fit your application's requirements.
Streamlining Integrations with Endgrate
While integrating with FreshDesk is straightforward, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including FreshDesk.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?