Using the Zoho CRM API to Get Custom Objects in Python
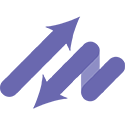
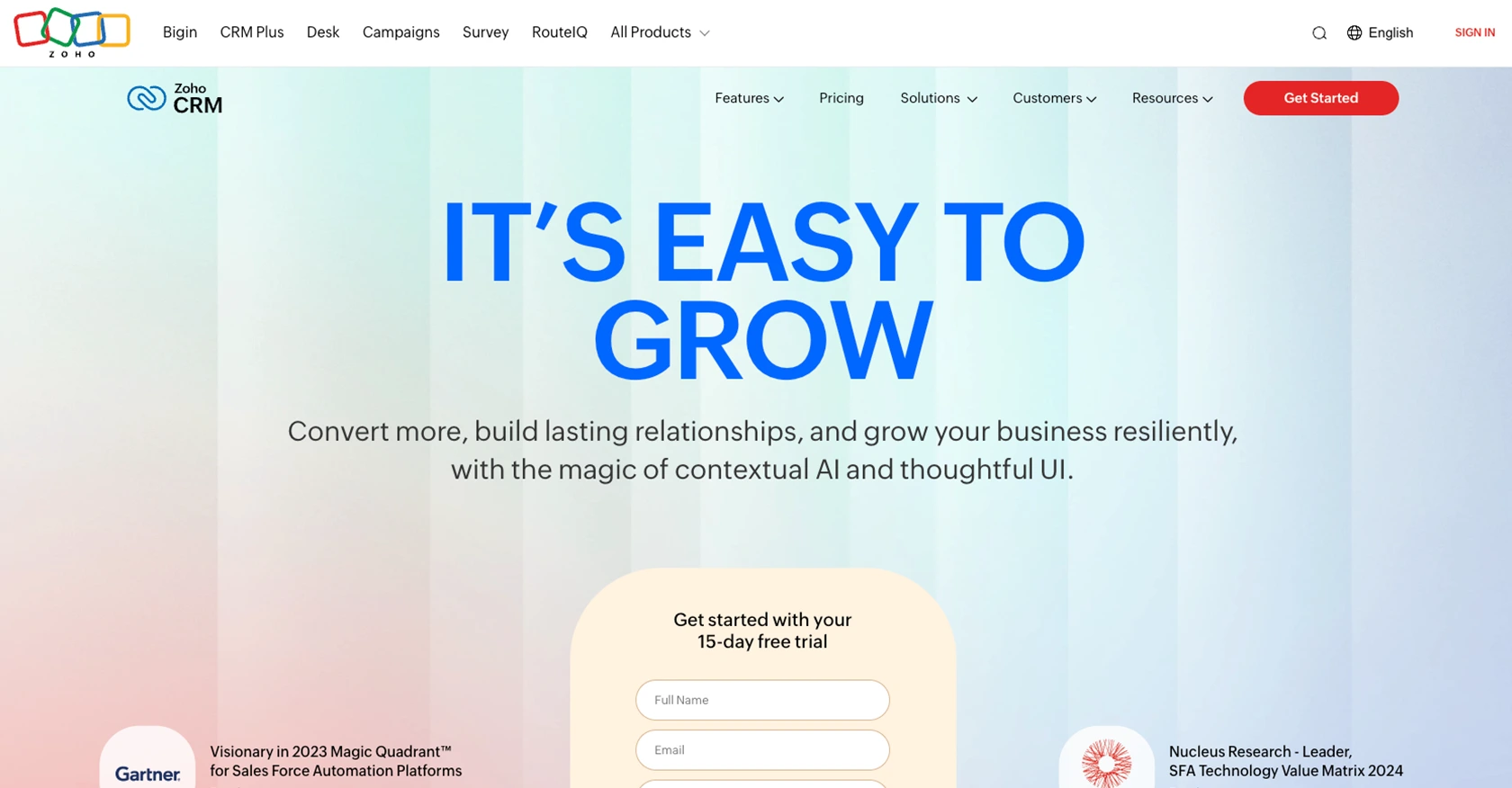
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a single system. Known for its flexibility and scalability, Zoho CRM offers a wide range of features including lead management, workflow automation, analytics, and more.
Developers often integrate with Zoho CRM to enhance business processes by accessing and manipulating customer data programmatically. For example, using the Zoho CRM API, a developer can retrieve custom objects to analyze customer interactions and improve sales strategies.
This article will guide you through using Python to interact with Zoho CRM's API, specifically focusing on retrieving custom objects. By following this tutorial, you'll learn how to efficiently access and manage data within Zoho CRM, enabling you to automate and streamline your business operations.
Setting Up Your Zoho CRM Test/Sandbox Account
Before diving into the integration process, it's essential to set up a Zoho CRM test or sandbox account. This environment allows you to safely experiment with the API without affecting live data. Zoho CRM provides a developer-friendly sandbox that mimics the production environment, enabling you to test your integrations thoroughly.
Creating a Zoho CRM Account
If you don't already have a Zoho CRM account, follow these steps to create one:
- Visit the Zoho CRM website and click on "Sign Up For Free."
- Fill in the required details, such as your name, email, and password, and click "Sign Up."
- Verify your email address by clicking on the link sent to your inbox.
- Log in to your new Zoho CRM account.
Accessing the Zoho CRM Sandbox
To access the sandbox environment, follow these steps:
- Log in to your Zoho CRM account.
- Navigate to the "Setup" section by clicking on the gear icon in the top-right corner.
- Under "Developer Space," select "Sandbox."
- Click "Create Sandbox" and follow the prompts to set up your sandbox environment.
Registering a Zoho CRM App for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register an application to obtain the necessary credentials. Here's how to do it:
- Go to the Zoho API Console.
- Click "Add Client" and choose the appropriate client type (e.g., Web Based, Self Client).
- Fill in the required details, such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Click "Create" to generate your Client ID and Client Secret.
Ensure you save these credentials securely, as they will be used to authenticate API requests.
Generating OAuth Tokens
With your app registered, you can now generate OAuth tokens:
- Direct users to the Zoho authorization URL with your Client ID and requested scopes.
- Upon user consent, Zoho will redirect to your specified URI with an authorization code.
- Exchange this code for access and refresh tokens by making a POST request to Zoho's token endpoint.
import requests
# Set the token endpoint and payload
token_url = "https://accounts.zoho.com/oauth/v2/token"
payload = {
"grant_type": "authorization_code",
"client_id": "Your_Client_ID",
"client_secret": "Your_Client_Secret",
"redirect_uri": "Your_Redirect_URI",
"code": "Authorization_Code"
}
# Make the POST request to get tokens
response = requests.post(token_url, data=payload)
tokens = response.json()
print(tokens)
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, and Authorization_Code
with your actual credentials and code.
With your sandbox account and OAuth setup complete, you're ready to start interacting with Zoho CRM's API using Python.
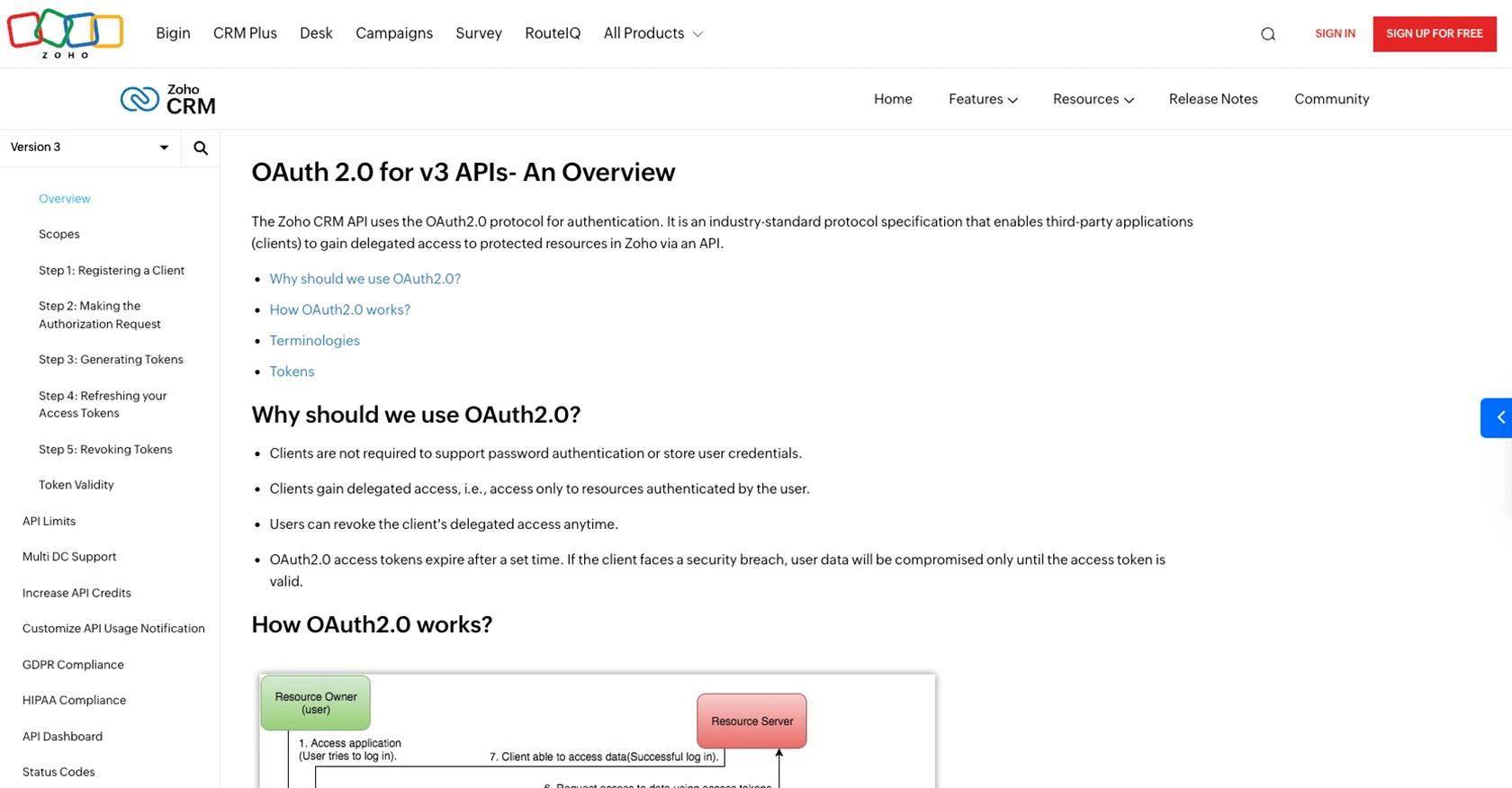
sbb-itb-96038d7
Making API Calls to Retrieve Custom Objects from Zoho CRM Using Python
With your Zoho CRM sandbox and OAuth setup complete, you can now proceed to make API calls to retrieve custom objects. This section will guide you through the process of setting up your Python environment, writing the code to interact with Zoho CRM's API, and handling the responses effectively.
Setting Up Your Python Environment for Zoho CRM API Integration
Before making API calls, ensure your Python environment is ready. You'll need Python 3.11.1 and the requests
library to handle HTTP requests. Install the library using pip:
pip install requests
Writing Python Code to Fetch Custom Objects from Zoho CRM
To retrieve custom objects, you'll need to specify the module you want to access. Replace MODULE_HERE
with your desired custom object module in the API endpoint URL. Here's a sample code snippet to get you started:
import requests
# Define the API endpoint and headers
module_name = "Your_Custom_Module"
endpoint = f"https://www.zohoapis.com/crm/v3/{module_name}"
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
print("Custom Objects Retrieved Successfully:", data)
else:
print("Failed to Retrieve Custom Objects:", response.status_code, response.text)
Replace Your_Custom_Module
and Your_Access_Token
with your actual module name and access token.
Handling API Responses and Error Codes from Zoho CRM
After making the API call, it's crucial to handle the response correctly. If the request is successful, the response will contain the custom objects data. In case of errors, Zoho CRM provides specific status codes to help you identify the issue:
- 401 Unauthorized: Check if your access token is valid and has the necessary scopes.
- 403 Forbidden: Ensure your user has permission to access the module.
- 404 Not Found: Verify the module name is correct.
- 500 Internal Server Error: Contact Zoho support if the issue persists.
Refer to the Zoho CRM Status Codes Documentation for more details.
Verifying API Call Success in Zoho CRM Sandbox
To confirm that your API call was successful, log in to your Zoho CRM sandbox account and navigate to the module you accessed. Check if the data retrieved matches the records in the sandbox environment.
By following these steps, you can efficiently interact with Zoho CRM's API to retrieve custom objects, enabling you to automate and enhance your business processes.
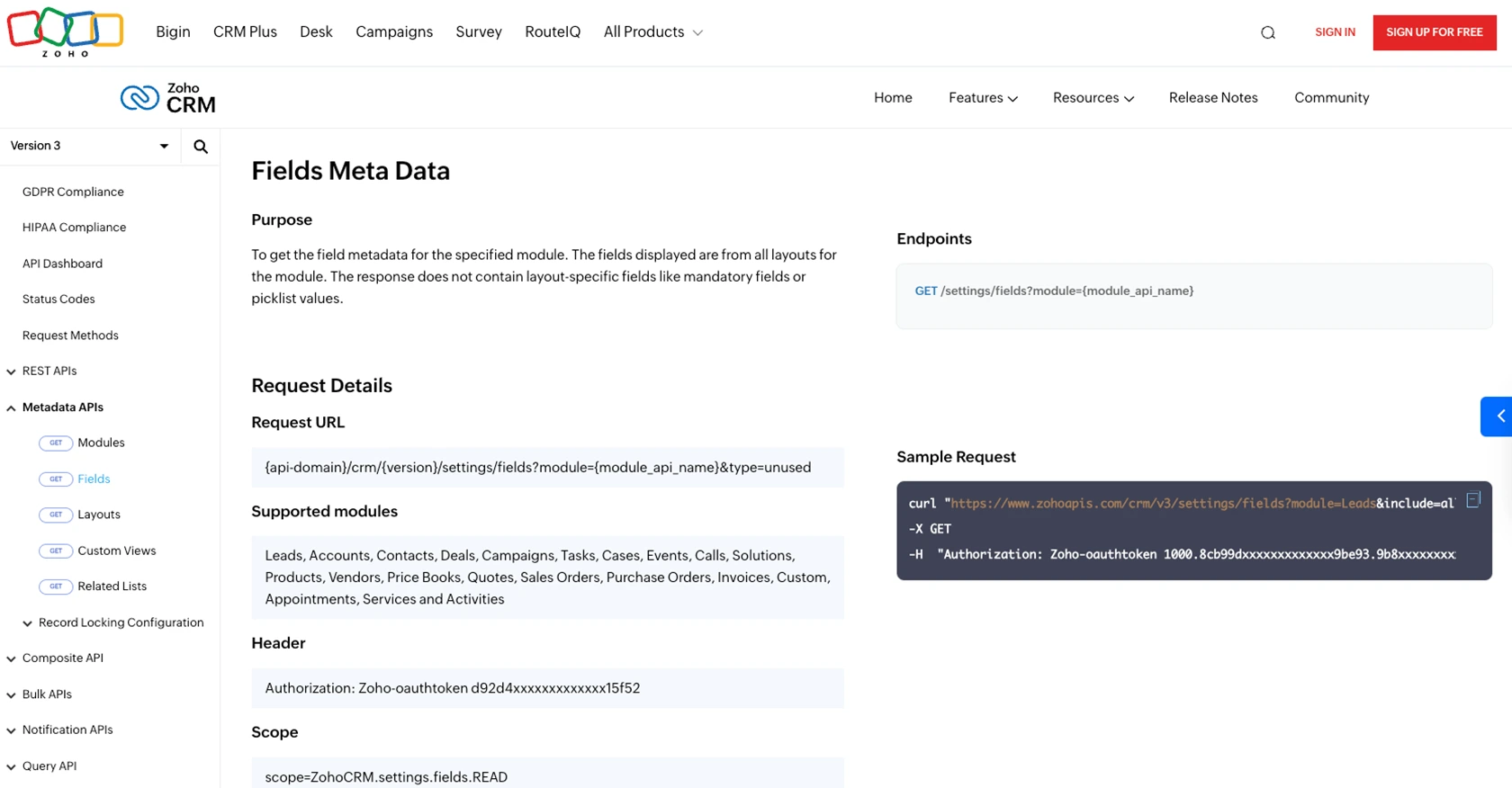
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with Zoho CRM's API to retrieve custom objects using Python can significantly enhance your business operations by automating data management and improving workflow efficiency. By following the steps outlined in this guide, you can effectively set up your environment, authenticate using OAuth, and make successful API calls.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your source code or exposing them in public repositories.
- Handle Rate Limiting: Zoho CRM imposes API rate limits. Monitor your API usage and implement logic to handle rate limit responses gracefully. Refer to the Zoho CRM API Limits Documentation for more details.
- Refresh Tokens Regularly: Since access tokens expire, ensure you implement a mechanism to refresh tokens using the refresh token to maintain uninterrupted API access.
- Data Transformation and Standardization: When retrieving data, consider transforming and standardizing fields to match your application's data model for consistency and ease of use.
Streamline Your Integrations with Endgrate
While integrating with Zoho CRM's API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Zoho CRM. By leveraging Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your users.
Explore how Endgrate can help you save time and resources by visiting Endgrate and discover the benefits of outsourcing your integration needs.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/module-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?