Using the Zoho Books API to Create or Update Items in Python
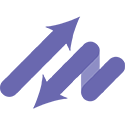
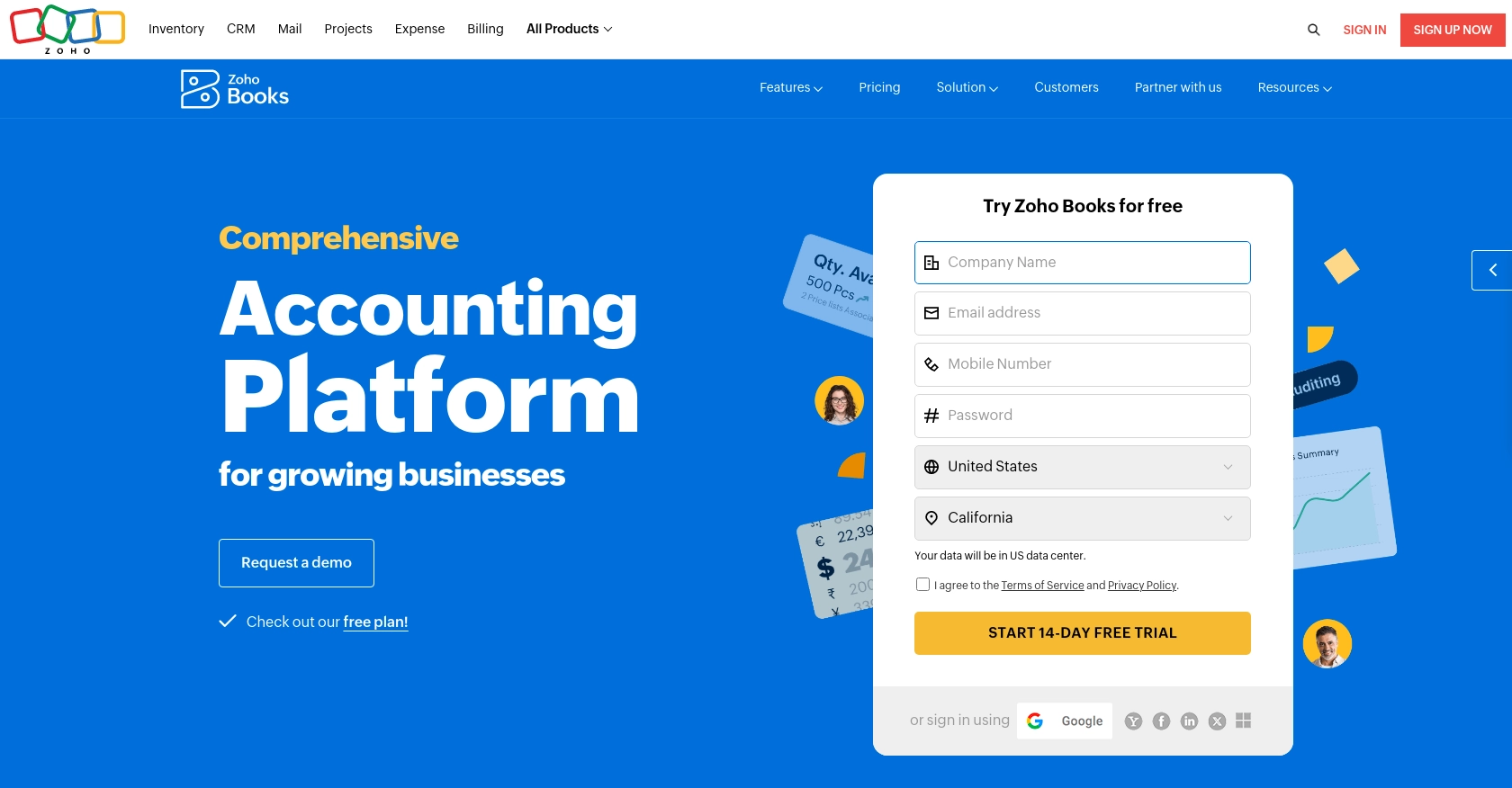
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software designed to streamline financial management for businesses. It offers a wide range of features, including invoicing, expense tracking, and inventory management, making it a popular choice for small to medium-sized enterprises.
Integrating with the Zoho Books API allows developers to automate and enhance financial operations. For example, you can use the API to create or update items in your inventory directly from your application, ensuring that your product data remains accurate and up-to-date.
In this article, we'll explore how to interact with the Zoho Books API using Python to manage items efficiently. This integration can help developers save time and reduce manual errors by automating item management tasks.
Setting Up Your Zoho Books Test Account
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Create a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. Follow the instructions to create your account and log in.
Register a New Client in Zoho Developer Console
To use the Zoho Books API, you must register your application to obtain the necessary OAuth credentials. Follow these steps:
- Go to the Zoho Developer Console.
- Click on Add Client ID to register a new application.
- Fill in the required details, such as the client name, homepage URL, and authorized redirect URIs.
- Upon successful registration, you'll receive a Client ID and Client Secret. Keep these credentials secure.
Generate OAuth Tokens for Zoho Books API
With your client credentials, you can now generate OAuth tokens to authenticate API requests:
- Redirect users to the following URL to obtain a grant token:
- After user consent, Zoho will redirect to your specified URI with a code parameter.
- Exchange the grant token for an access token by making a POST request:
- Store the access_token and refresh_token received in the response for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.settings.CREATE,ZohoBooks.settings.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
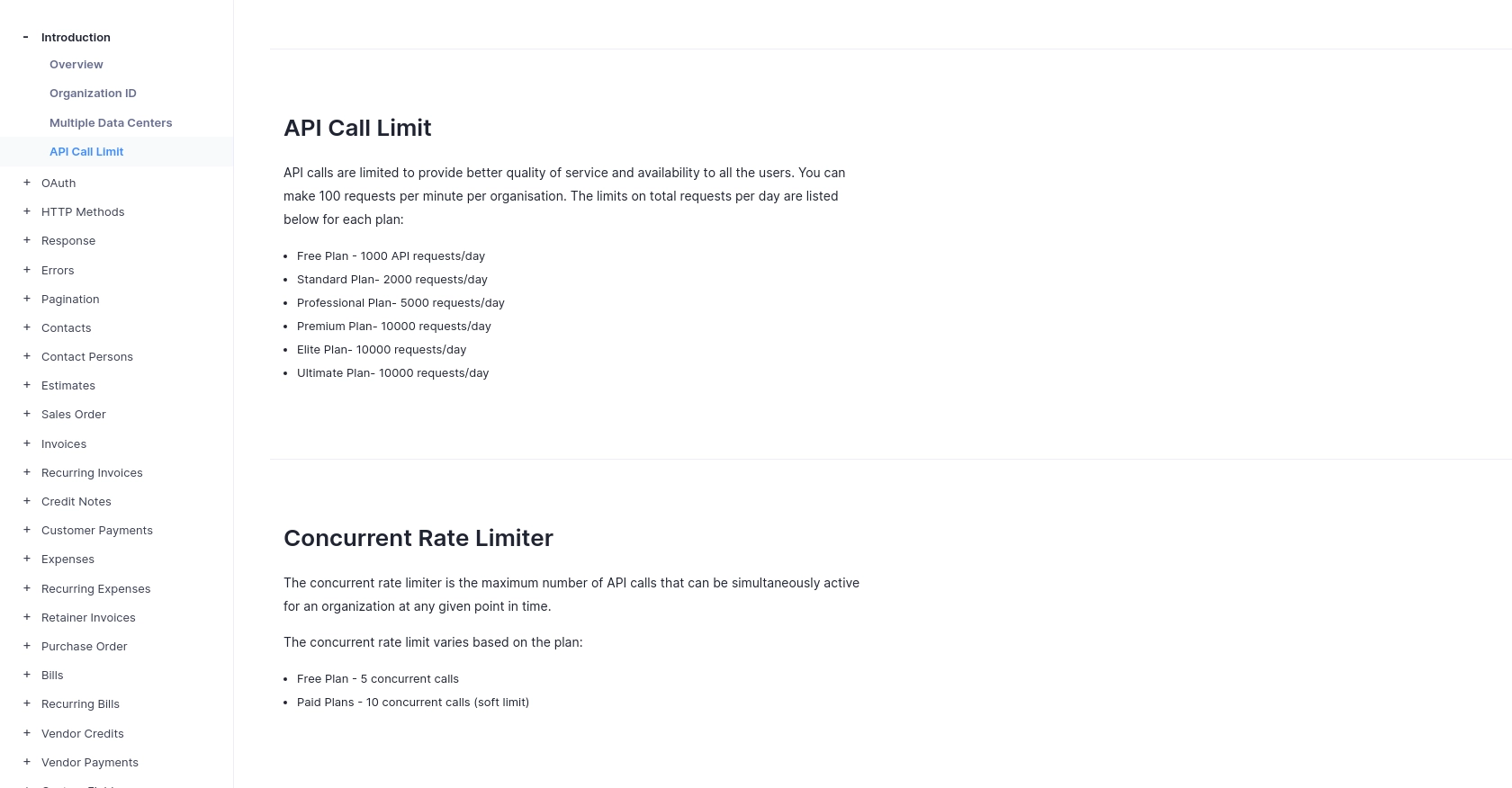
sbb-itb-96038d7
Making API Calls to Zoho Books for Item Management Using Python
To interact with the Zoho Books API and manage items, you'll need to use Python to make HTTP requests. This section will guide you through the process of creating and updating items using the Zoho Books API.
Prerequisites for Zoho Books API Integration with Python
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Install the requests
library to handle HTTP requests:
pip install requests
Creating an Item in Zoho Books Using Python
To create an item in Zoho Books, follow these steps:
- Create a new Python file named
create_item.py
and add the following code:
import requests
# Define the API endpoint and headers
url = "https://www.zohoapis.com/books/v3/items?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the item data
item_data = {
"name": "Hard Drive",
"rate": 120,
"description": "500GB",
"sku": "s12345",
"product_type": "goods"
}
# Make the POST request to create the item
response = requests.post(url, json=item_data, headers=headers)
# Check the response
if response.status_code == 201:
print("Item created successfully:", response.json())
else:
print("Failed to create item:", response.json())
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token.
Run the script using the command:
python create_item.py
Upon success, you should see a confirmation message with the item details.
Updating an Item in Zoho Books Using Python
To update an existing item, follow these steps:
- Create a new Python file named
update_item.py
and add the following code:
import requests
# Define the API endpoint and headers
item_id = "ITEM_ID_TO_UPDATE"
url = f"https://www.zohoapis.com/books/v3/items/{item_id}?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the updated item data
updated_data = {
"name": "Hard Drive Pro",
"rate": 150,
"description": "1TB"
}
# Make the PUT request to update the item
response = requests.put(url, json=updated_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Item updated successfully:", response.json())
else:
print("Failed to update item:", response.json())
Replace ITEM_ID_TO_UPDATE
, YOUR_ORG_ID
, and YOUR_ACCESS_TOKEN
with the respective item ID, organization ID, and access token.
Run the script using the command:
python update_item.py
Upon success, you should see a confirmation message with the updated item details.
Handling Errors and Verifying API Requests
It's crucial to handle errors effectively when making API calls. Zoho Books uses HTTP status codes to indicate the result of an API call:
- 200: OK
- 201: Created
- 400: Bad Request
- 401: Unauthorized
- 404: Not Found
- 429: Rate Limit Exceeded
- 500: Internal Server Error
Refer to the Zoho Books Error Documentation for more details.
Verify the success of your API requests by checking the response data and confirming changes in your Zoho Books account.
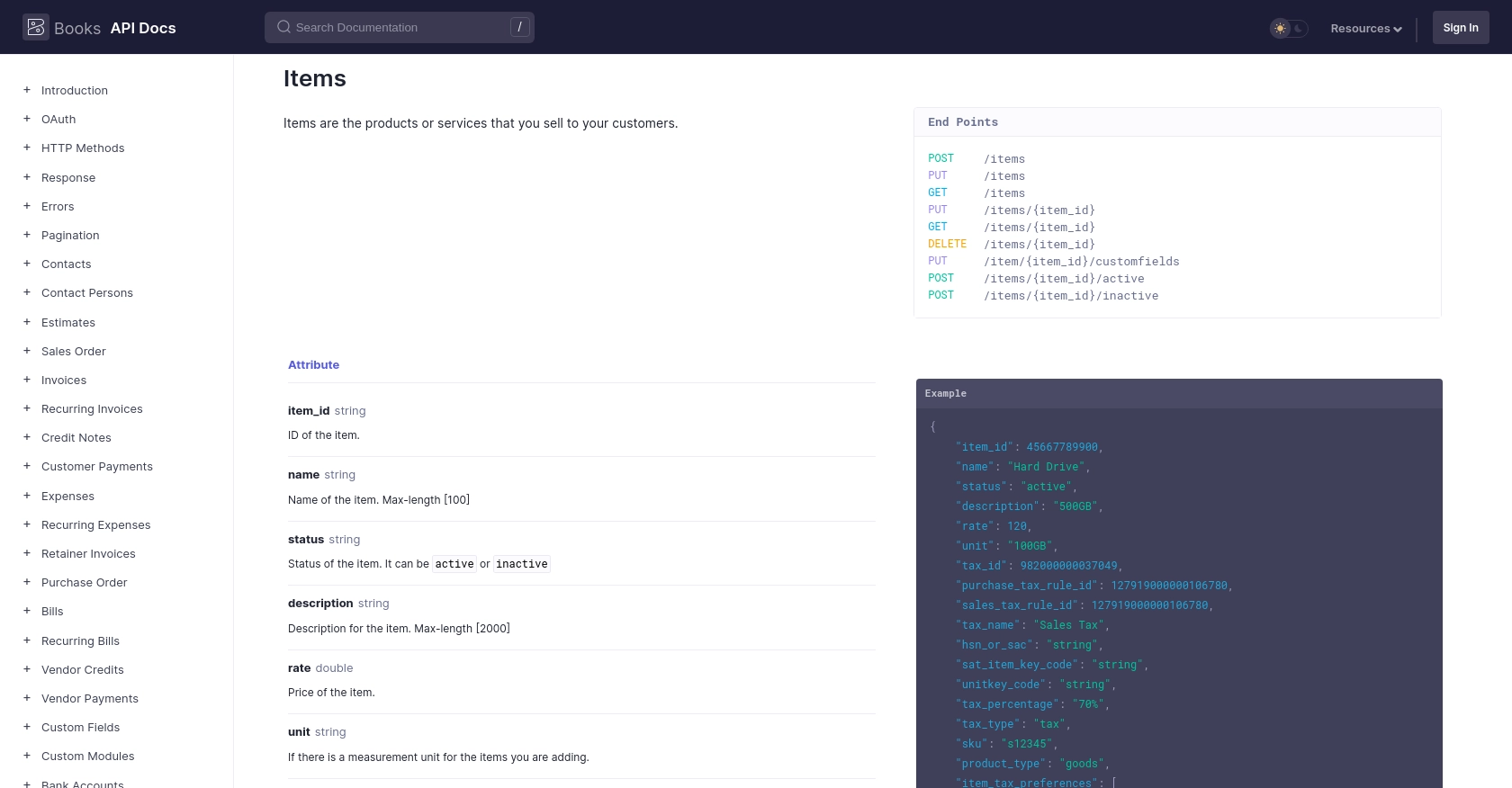
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using Python provides a powerful way to automate and streamline your financial operations. By following the steps outlined in this article, you can efficiently create and update items in your Zoho Books account, reducing manual effort and minimizing errors.
Best Practices for Secure and Efficient Zoho Books API Usage
- Securely Store OAuth Credentials: Ensure that your Client ID, Client Secret, and tokens are stored securely and not exposed in your codebase. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Zoho Books imposes a rate limit of 100 requests per minute per organization. Implement logic to handle rate limit errors (HTTP status code 429) by retrying requests after a delay. For more details, refer to the Zoho Books API Call Limit documentation.
- Standardize Data Fields: Ensure consistency in data fields when creating or updating items. This helps maintain data integrity across your applications.
- Monitor API Usage: Regularly monitor your API usage to ensure compliance with Zoho Books' limits and to optimize your integration's performance.
Streamline Your Integrations with Endgrate
While integrating with Zoho Books directly can be effective, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Zoho Books.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy a seamless integration experience for your customers. Visit Endgrate to learn more about how you can enhance your integration strategy.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/items/#overview
Ready to get started?