Using the Mailchimp API to Get Members (with Javascript examples)
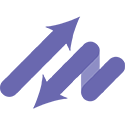
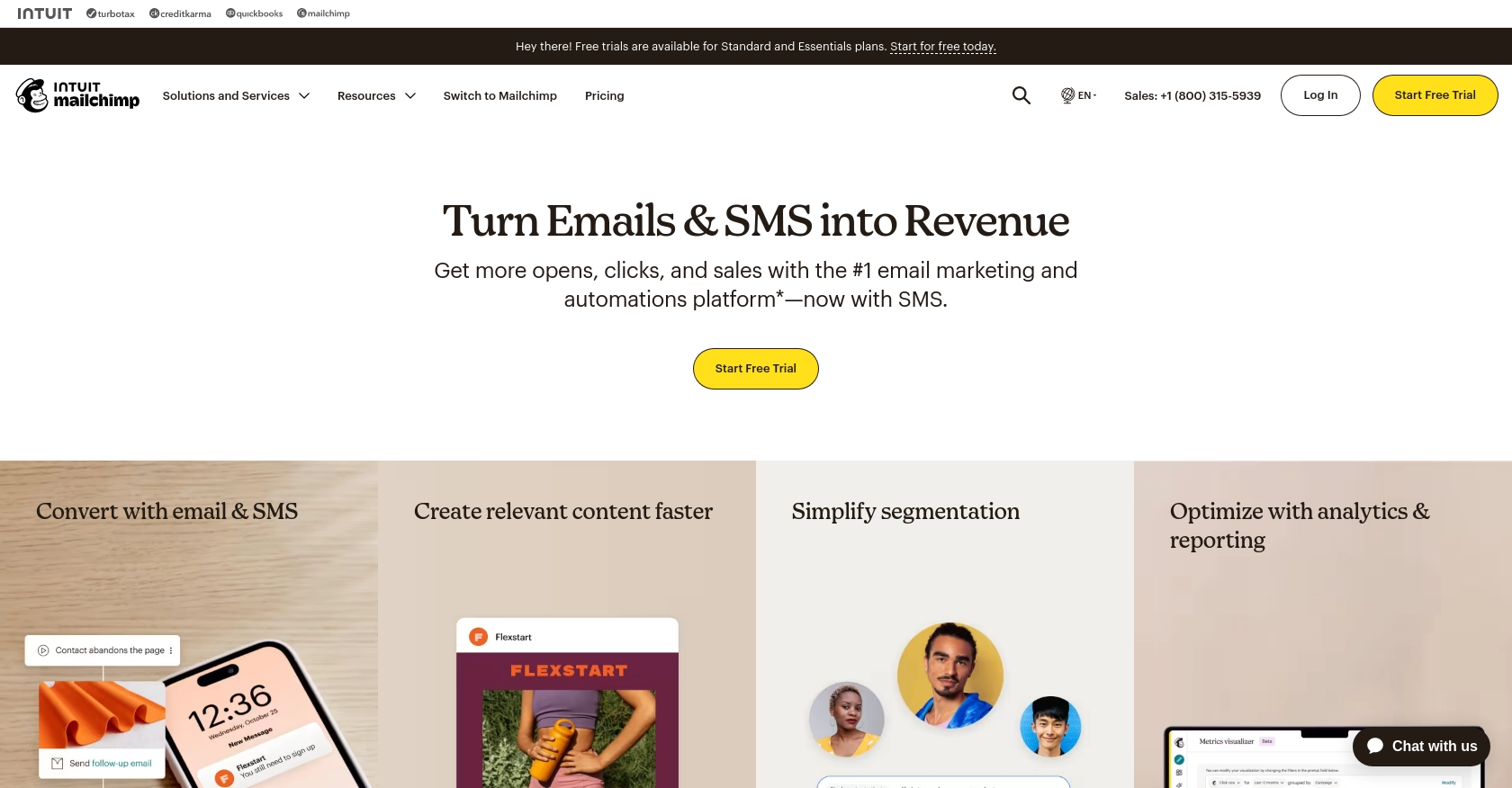
Introduction to Mailchimp API Integration
Mailchimp is a powerful marketing automation platform that provides businesses with the tools to create, send, and analyze email campaigns. Known for its user-friendly interface and robust features, Mailchimp is a popular choice for businesses looking to enhance their marketing efforts.
Developers may want to integrate with Mailchimp's API to manage audience data, automate marketing workflows, and synchronize email activities with their applications. For example, using the Mailchimp API, a developer can retrieve member information from a specific audience list to personalize marketing campaigns or analyze subscriber behavior.
Setting Up Your Mailchimp Test Account and API Key
Before you can start using the Mailchimp API, you'll need to set up a Mailchimp account and generate an API key. This key will allow you to authenticate your requests and interact with Mailchimp's services programmatically.
Creating a Mailchimp Account
If you don't already have a Mailchimp account, follow these steps to create one:
- Visit the Mailchimp website and click on the "Sign Up Free" button.
- Fill in the required information, including your email, username, and password, then click "Sign Up."
- Follow the instructions in the confirmation email to activate your account.
Generating Your Mailchimp API Key
Once your account is set up, you can generate an API key to authenticate your API requests:
- Log in to your Mailchimp account and navigate to the "Account" section.
- Under "Extras," select "API keys."
- Click on "Create A Key" to generate a new API key.
- Copy the generated API key and store it securely, as it provides full access to your Mailchimp account.
For more details, refer to the Mailchimp API Quick Start Guide.
Understanding Mailchimp OAuth Authentication
If your integration requires accessing Mailchimp data on behalf of other users, you should implement OAuth 2.0 authentication:
- Register your application in the Mailchimp Developer portal.
- Follow the OAuth 2.0 flow to obtain an access token for each user.
For more information, see the Mailchimp OAuth Guide.
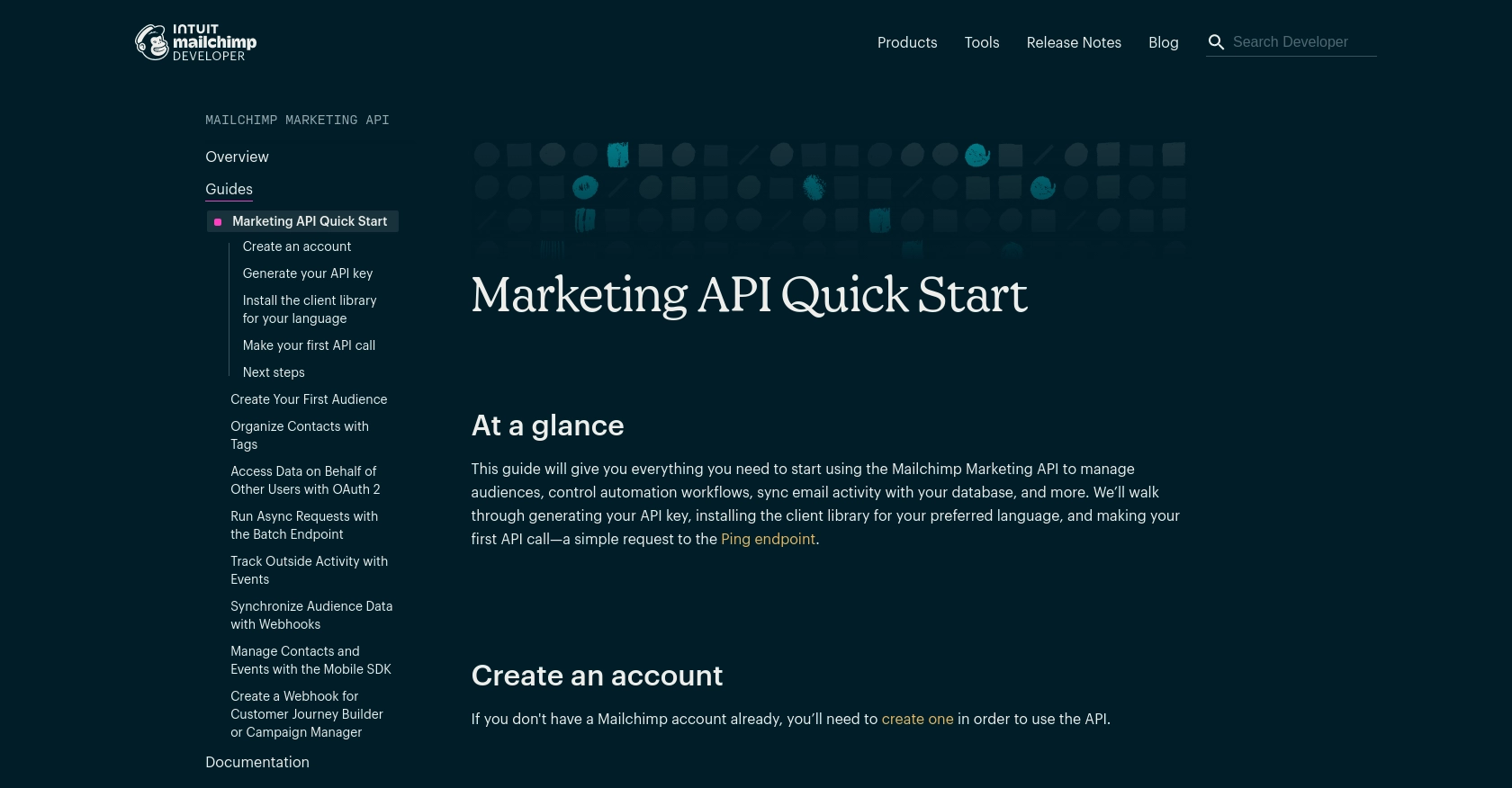
sbb-itb-96038d7
Making API Calls to Retrieve Members from Mailchimp Using JavaScript
To interact with the Mailchimp API and retrieve member information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code to make the API call, and handling the response.
Setting Up Your JavaScript Environment for Mailchimp API Integration
Before you begin, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side API interactions.
- Download and install Node.js from the official website.
- Once installed, you can use npm (Node Package Manager) to install necessary packages.
Installing Required Packages for Mailchimp API Requests
To make HTTP requests in JavaScript, you can use the popular axios
library. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch Members from Mailchimp
Now that your environment is set up, you can write the JavaScript code to interact with the Mailchimp API. Create a new file named getMailchimpMembers.js
and add the following code:
const axios = require('axios');
// Replace with your Mailchimp API key and server prefix
const apiKey = 'YOUR_API_KEY';
const serverPrefix = 'YOUR_SERVER_PREFIX';
const listId = 'YOUR_LIST_ID';
// Set the API endpoint
const endpoint = `https://${serverPrefix}.api.mailchimp.com/3.0/lists/${listId}/members`;
// Configure the request headers
const headers = {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
};
// Make a GET request to the Mailchimp API
axios.get(endpoint, { headers })
.then(response => {
console.log('Members:', response.data.members);
})
.catch(error => {
console.error('Error fetching members:', error.response.data);
});
Replace YOUR_API_KEY
, YOUR_SERVER_PREFIX
, and YOUR_LIST_ID
with your actual Mailchimp API key, server prefix, and list ID.
Running the JavaScript Code to Retrieve Mailchimp Members
To execute the code, run the following command in your terminal:
node getMailchimpMembers.js
If successful, you should see a list of members from your specified Mailchimp audience list printed in the console.
Handling Errors and Verifying API Call Success
When making API calls, it's crucial to handle potential errors and verify the success of your requests. The code above includes a catch
block to log any errors that occur during the request. Common error codes include:
- 401 Unauthorized: Check your API key and ensure it's correct.
- 404 Not Found: Verify the list ID and server prefix.
- 429 Too Many Requests: You have exceeded the API rate limits. Refer to the Mailchimp API limits documentation for more details.
For more information on handling errors, refer to the Mailchimp API documentation.
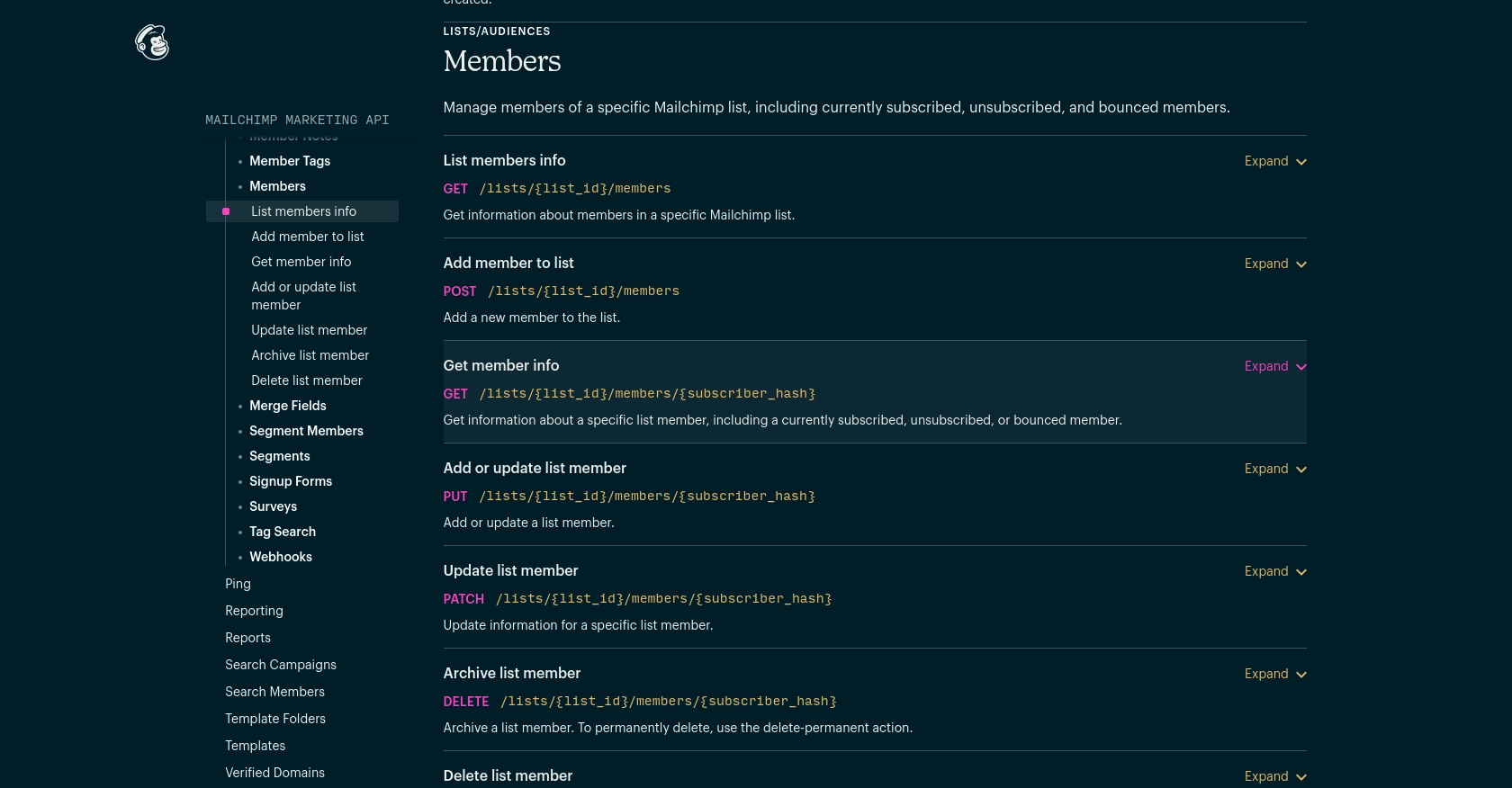
Conclusion and Best Practices for Mailchimp API Integration
Integrating with the Mailchimp API using JavaScript allows developers to efficiently manage audience data and automate marketing workflows. By following the steps outlined in this guide, you can successfully retrieve member information and enhance your marketing strategies.
Best Practices for Secure and Efficient Mailchimp API Usage
- Secure API Keys: Store your API keys securely and avoid exposing them in client-side code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Mailchimp's API rate limits, which allow up to 10 simultaneous connections. Implement retry logic and cache frequent requests to avoid hitting these limits. For more details, refer to the Mailchimp API limits documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your applications.
Enhancing Your Integration Experience with Endgrate
For developers looking to streamline their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can simplify your Mailchimp API integrations and beyond.
Read More
Ready to get started?