Using the Typeform API to Get Responses (with PHP examples)
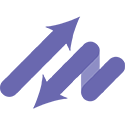
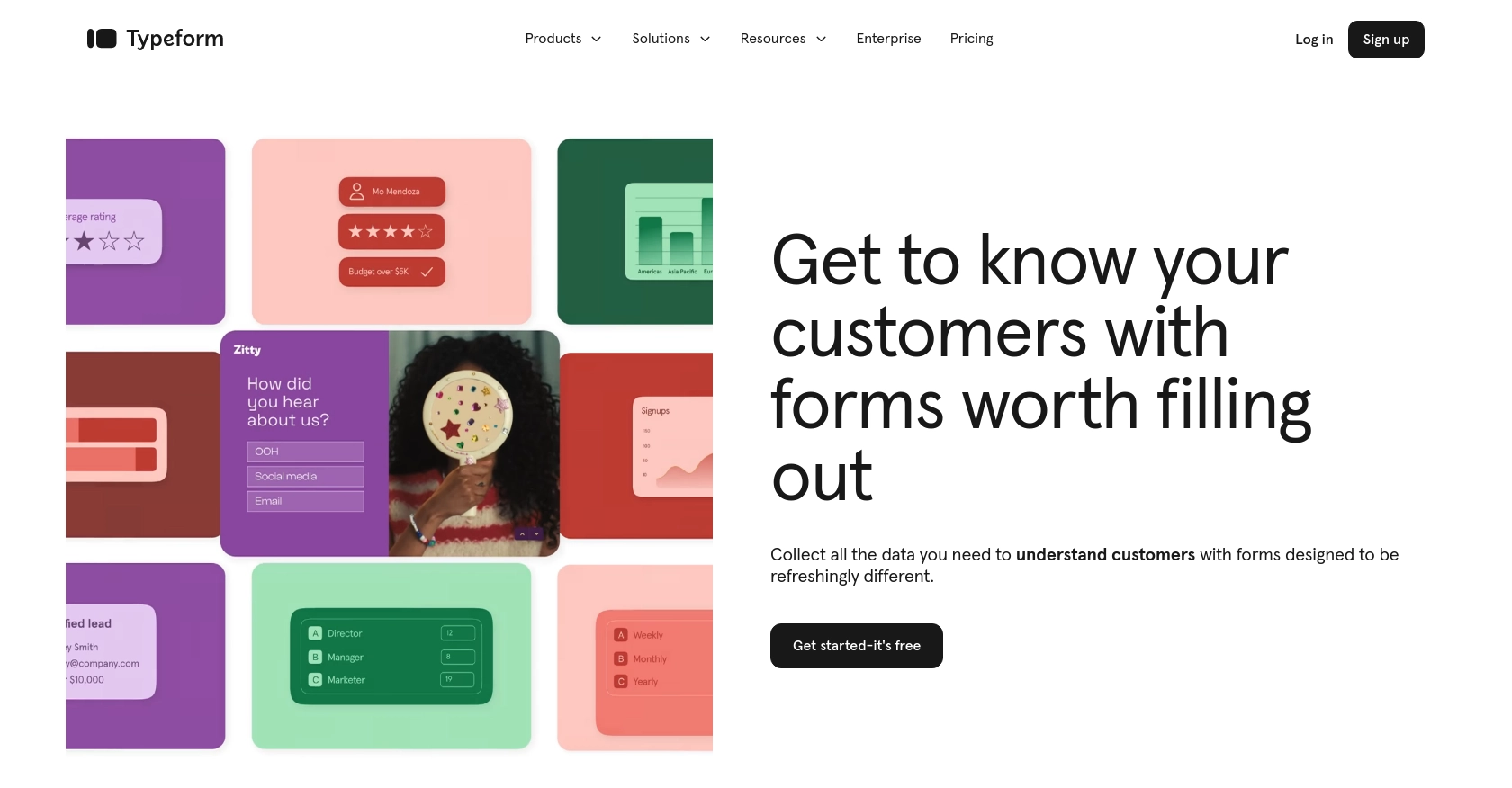
Introduction to Typeform API Integration
Typeform is a versatile online form builder that allows businesses to create engaging and interactive forms, surveys, and quizzes. Known for its user-friendly interface and customizable design options, Typeform helps organizations gather valuable data and insights from their audience.
Integrating with Typeform's API enables developers to automate data retrieval and streamline workflows. For example, you can use the Typeform API to automatically fetch survey responses and analyze them in real-time, enhancing decision-making processes and improving customer engagement strategies.
Setting Up Your Typeform Developer Account and Application
Before you can start using the Typeform API to retrieve responses, you need to set up a developer account and create an application within Typeform. This process involves registering your application to obtain the necessary credentials for OAuth 2.0 authentication.
Step-by-Step Guide to Creating a Typeform Developer Account
- Sign Up or Log In: If you don't already have a Typeform account, visit the Typeform website and sign up for a free account. If you already have an account, simply log in.
- Access Developer Apps: Once logged in, navigate to the upper-left corner and click the icon drop-down menu next to your organization name. Under the Organization section, click on Developer Apps.
- Register a New App: Click on Register a new app. Fill in the required fields, including the App Name, App Website, and Redirect URI(s). The Redirect URI is where Typeform will redirect users after they log in and grant access to your app.
-
Save Your Credentials: After registering your app, you'll receive a
client_id
andclient_secret
. Make sure to copy and store these credentials securely, as they are essential for the OAuth 2.0 authentication process.
Configuring OAuth 2.0 for Typeform API Access
Typeform uses OAuth 2.0 to secure its API endpoints. Follow these steps to configure OAuth 2.0 for your application:
-
Authorization Request: Direct users to the following URL to initiate the OAuth flow:
Replacehttps://api.typeform.com/oauth/authorize?client_id={your_client_id}&redirect_uri={your_redirect_uri}&scope=responses:read
{your_client_id}
and{your_redirect_uri}
with your actual client ID and redirect URI. -
Exchange Authorization Code for Access Token: After users grant access, Typeform will redirect them to your specified redirect URI with an authorization code. Use this code to request an access token:
Replacecurl --request POST \ --url https://api.typeform.com/oauth/token \ --data-urlencode 'grant_type=authorization_code' \ --data-urlencode 'code={authorization_code}' \ --data-urlencode 'client_id={your_client_id}' \ --data-urlencode 'client_secret={your_client_secret}' \ --data-urlencode 'redirect_uri={your_redirect_uri}'
{authorization_code}
,{your_client_id}
,{your_client_secret}
, and{your_redirect_uri}
with the appropriate values.
Once you have completed these steps, your application will be ready to interact with the Typeform API using the access token obtained through the OAuth 2.0 process. For more detailed information, refer to the Typeform Applications Documentation and OAuth 2.0 Scopes Documentation.
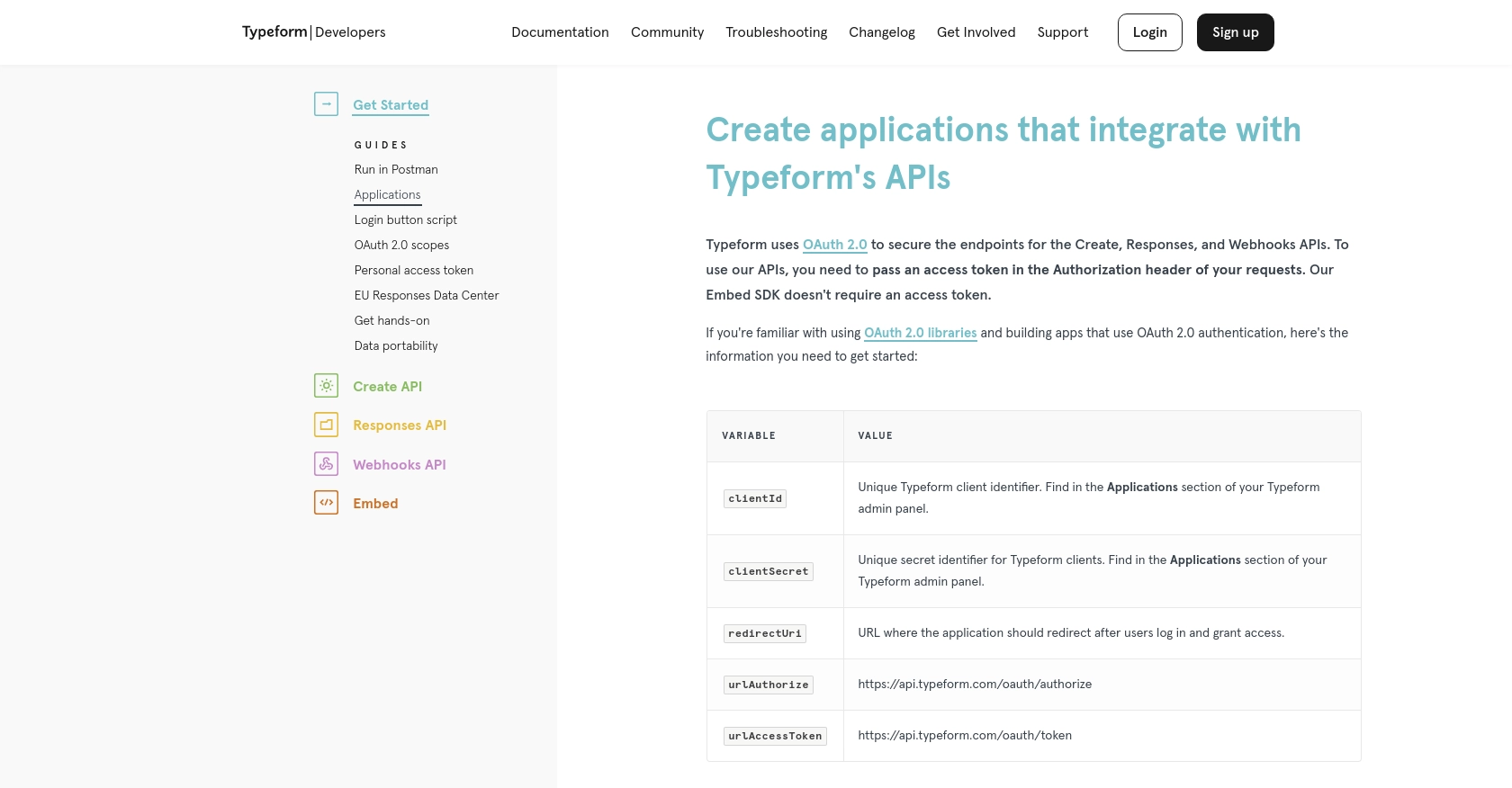
sbb-itb-96038d7
How to Make API Calls to Retrieve Typeform Responses Using PHP
Once you have set up your Typeform application and obtained an access token, you can start making API calls to retrieve responses from your Typeform forms. This section will guide you through the process of using PHP to interact with the Typeform API and fetch form responses.
Prerequisites for Using PHP with Typeform API
Before proceeding, ensure you have the following installed on your server:
- PHP 7.4 or higher
- cURL extension for PHP
These tools will allow you to make HTTP requests and handle JSON data effectively.
Fetching Typeform Responses with PHP
To retrieve responses from a specific Typeform, you need to make a GET request to the Typeform API endpoint. Here's a step-by-step guide:
-
Set the API Endpoint and Headers: Define the API endpoint URL and set the necessary headers, including the Authorization header with your access token.
Replace$endpoint = "https://api.typeform.com/forms/{form_id}/responses"; $headers = [ "Authorization: Bearer {your_access_token}", "Content-Type: application/json" ];
{form_id}
with your actual form ID and{your_access_token}
with your access token. -
Make the GET Request: Use cURL to send the GET request to the Typeform API.
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $endpoint); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch);
-
Handle the API Response: Parse the JSON response to access the form responses.
$data = json_decode($response, true); if (isset($data['items'])) { foreach ($data['items'] as $response) { echo "Response ID: " . $response['response_id'] . "\n"; // Access other response details as needed } } else { echo "No responses found or an error occurred."; }
By following these steps, you can successfully retrieve and process responses from your Typeform forms using PHP. For more details on the API parameters and response structure, refer to the Typeform Responses API Documentation.
Handling Errors and Debugging
When making API calls, it's crucial to handle potential errors gracefully. The Typeform API may return various HTTP status codes indicating the success or failure of your request. Common error codes include:
- 400 Bad Request: Indicates a malformed request. Check your request parameters.
- 401 Unauthorized: Indicates an issue with your access token. Ensure it's valid and not expired.
- 405 Method Not Allowed: Indicates an incorrect HTTP method. Ensure you're using GET for retrieving responses.
- 500 Internal Server Error: Indicates a server-side issue. Try again later or contact Typeform support.
Implement error handling in your PHP code to manage these scenarios effectively and ensure a robust integration with the Typeform API.
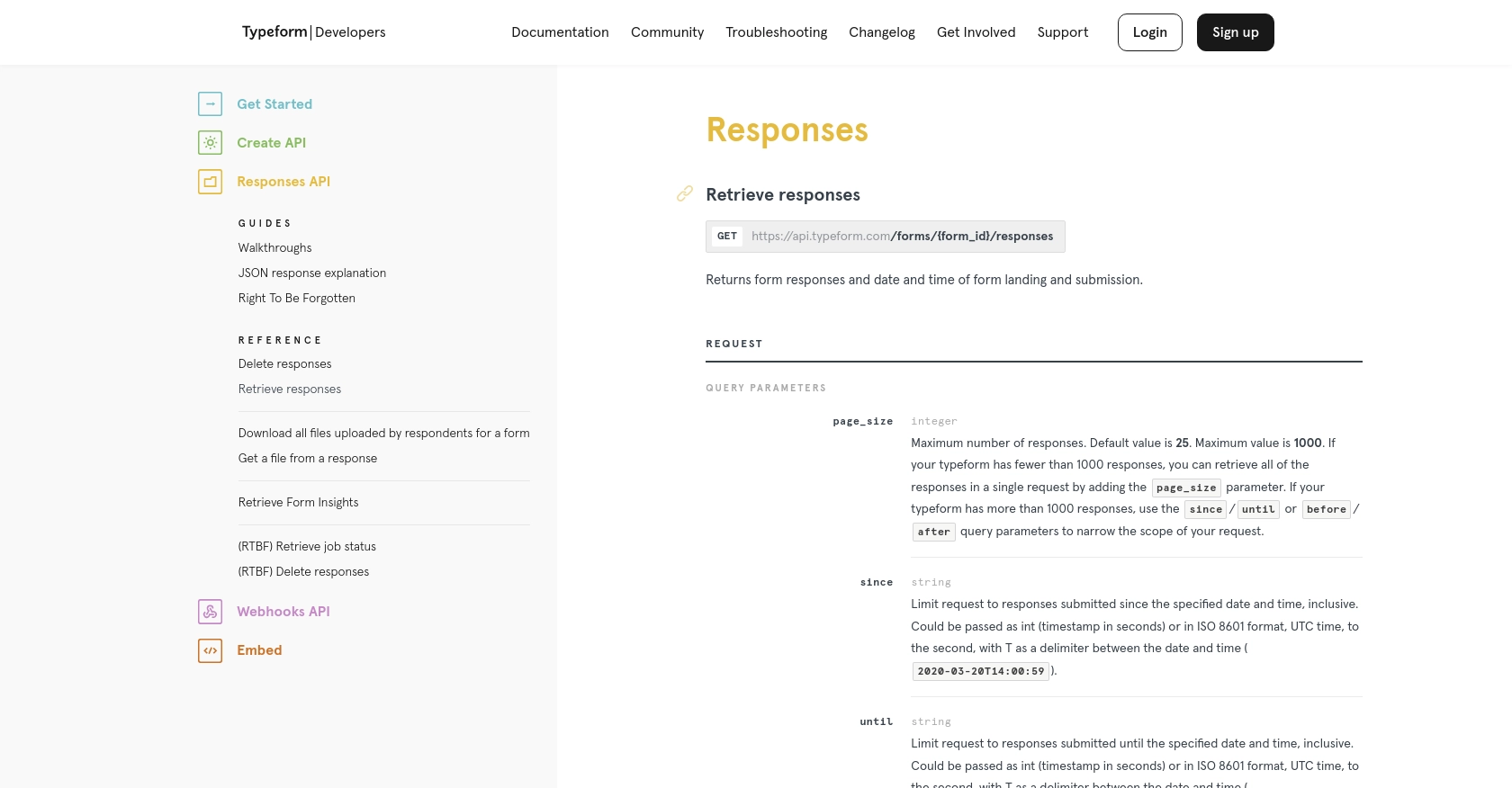
Best Practices for Secure and Efficient Typeform API Integration
When integrating with the Typeform API, it's essential to follow best practices to ensure secure and efficient data handling. Here are some recommendations:
- Secure Storage of Credentials: Always store your
client_id
,client_secret
, and access tokens securely. Avoid hardcoding them in your source code or committing them to version control systems. Use environment variables or secure vaults to manage sensitive information. - Implement Rate Limiting: Be mindful of Typeform's API rate limits to avoid throttling. Implement logic to handle rate limit responses and retry requests after the specified wait time.
- Data Transformation and Standardization: When retrieving responses, consider transforming and standardizing data fields to match your application's data model. This will facilitate seamless integration and data analysis.
- Error Handling and Logging: Implement robust error handling to manage API errors gracefully. Log errors for debugging and monitoring purposes to ensure smooth operation and quick resolution of issues.
Streamlining Typeform API Integrations with Endgrate
Integrating multiple APIs can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Typeform. With Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the intricacies of API integrations.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms, reducing redundancy and maintenance efforts.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience, improving satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover a more efficient way to manage your API integrations.
Read More
Ready to get started?