Using the Front API to Create Or Update Accounts (with Javascript examples)
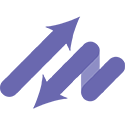
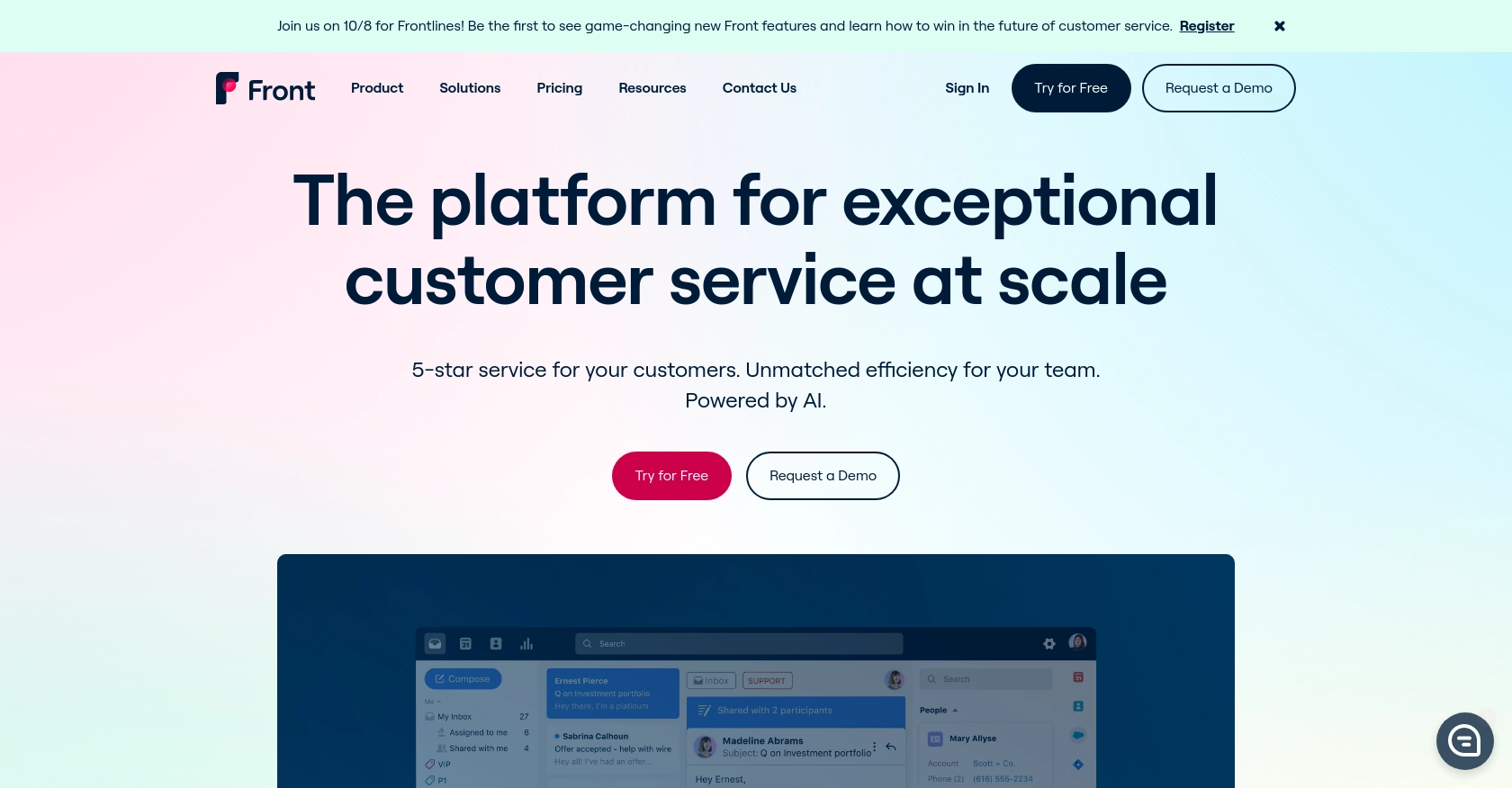
Introduction to Front API Integration
Front is a powerful communication platform that centralizes emails, messages, and other communication channels into a single collaborative workspace. It is designed to enhance team productivity by streamlining communication and providing tools for efficient collaboration.
Developers may want to integrate with Front's API to automate account management processes, such as creating or updating customer accounts. For example, a developer could use the Front API to automatically update account details from an external CRM system, ensuring that all customer information is current and synchronized across platforms.
Setting Up a Front Developer Account for API Integration
Before you can start using the Front API to create or update accounts, you'll need to set up a developer account. This will allow you to test your integration in a safe environment without affecting production data.
Creating a Front Developer Account
If you don't already have a Front account, you can sign up for a free developer account. This account provides access to a sandbox environment where you can experiment with the API.
- Visit the Front Developer Portal.
- Click on the sign-up link and follow the instructions to create your developer account.
- Once your account is set up, you'll have access to the developer environment.
Generating an API Token for Authentication
Front's API uses API tokens for authentication. Here's how you can generate one:
- Log in to your Front developer account.
- Navigate to the API tokens section in your account settings.
- Click on "Create API Token" and select the appropriate scopes for your integration.
- Copy the generated API token and store it securely, as you'll need it to authenticate your API requests.
For more detailed instructions, refer to the Front API documentation.
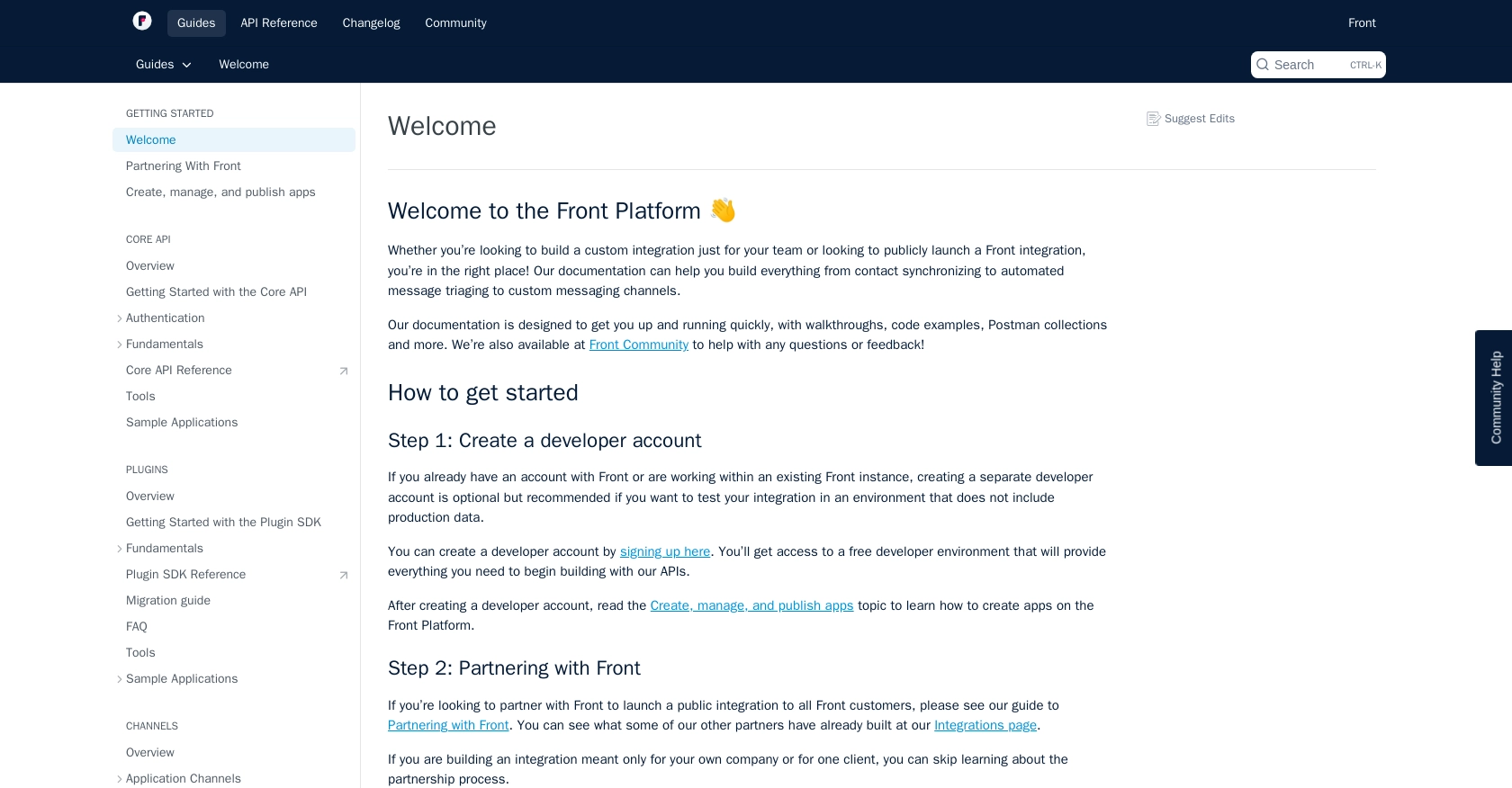
sbb-itb-96038d7
Making API Calls to Create or Update Accounts with Front API Using JavaScript
To interact with the Front API for creating or updating accounts, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Front API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Creating a New Account with Front API
To create a new account, you'll use the Front API's Create Account endpoint. Here's a sample JavaScript code snippet to perform this action:
const axios = require('axios');
const createAccount = async () => {
const url = 'https://api2.frontapp.com/accounts';
const token = 'YOUR_API_TOKEN'; // Replace with your actual API token
const accountData = {
name: 'New Account Name',
description: 'Description of the new account'
};
try {
const response = await axios.post(url, accountData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Account created successfully:', response.data);
} catch (error) {
console.error('Error creating account:', error.response.data);
}
};
createAccount();
Replace YOUR_API_TOKEN
with the API token you generated earlier. This code sends a POST request to the Front API to create a new account with the specified name and description.
Updating an Existing Account with Front API
To update an existing account, use the Update Account endpoint. Here's how you can do it with JavaScript:
const updateAccount = async (accountId) => {
const url = `https://api2.frontapp.com/accounts/${accountId}`;
const token = 'YOUR_API_TOKEN'; // Replace with your actual API token
const updateData = {
description: 'Updated description for the account'
};
try {
const response = await axios.patch(url, updateData, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
console.log('Account updated successfully:', response.data);
} catch (error) {
console.error('Error updating account:', error.response.data);
}
};
updateAccount('ACCOUNT_ID'); // Replace with the actual account ID
Replace ACCOUNT_ID
with the ID of the account you wish to update. This code sends a PATCH request to modify the account's description.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and errors appropriately. The above examples include basic error handling using try-catch
blocks. You can further enhance this by checking the status codes and implementing retry logic if necessary.
For more details on error codes and handling, refer to the Front API documentation.
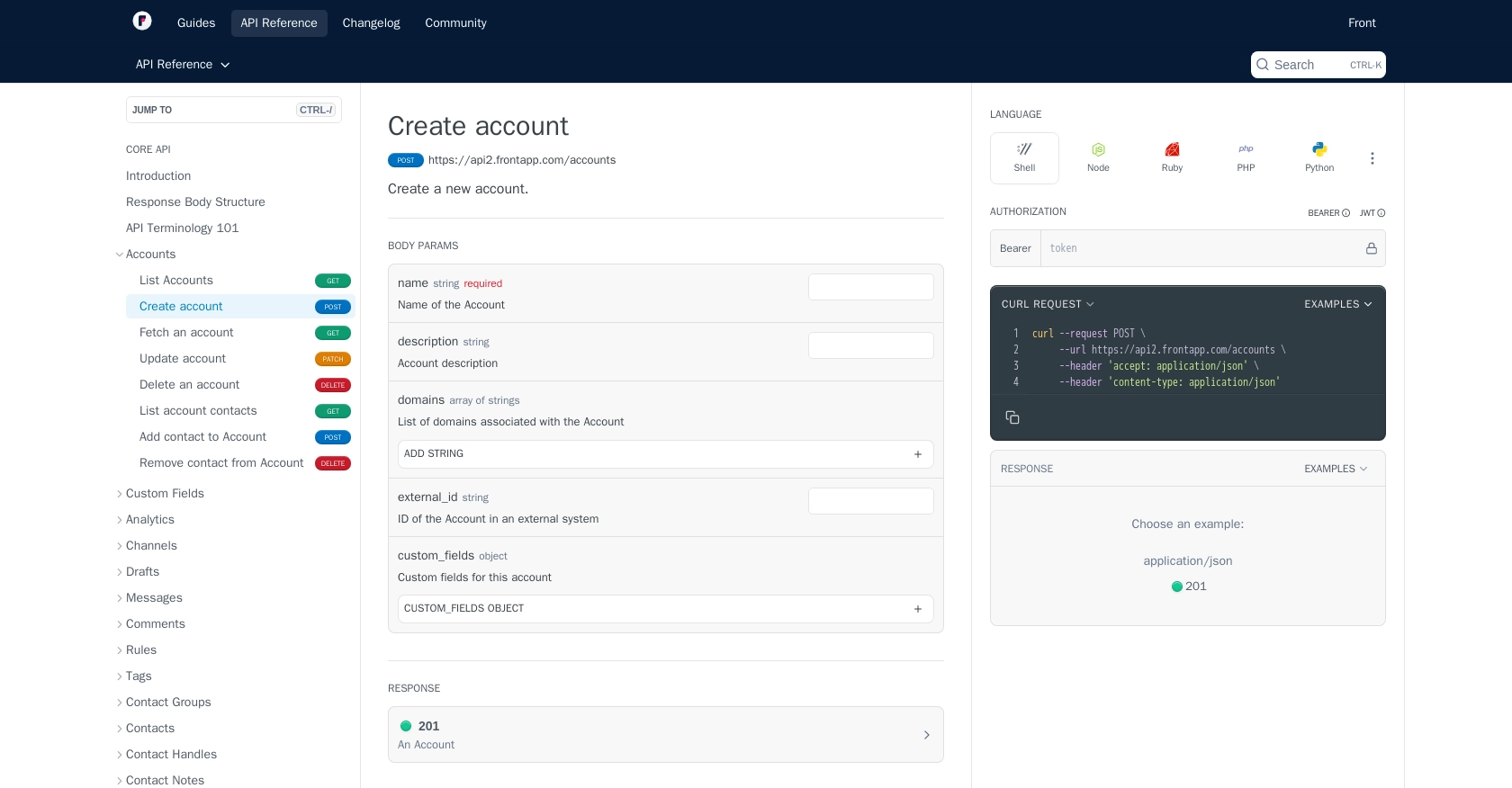
Conclusion and Best Practices for Using Front API in JavaScript
Integrating with the Front API to create or update accounts can significantly streamline your account management processes, ensuring data consistency across platforms. By automating these tasks, developers can save time and reduce the risk of manual errors.
Best Practices for Secure and Efficient Front API Integration
- Secure Storage of API Tokens: Always store your API tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Front's API has a rate limit of 50 requests per minute for the Starter plan. Implement logic to handle 429 status codes and use the
Retry-After
header to manage retries effectively. For more details, refer to the Front API rate limiting documentation. - Data Standardization: Ensure that data fields are standardized before sending them to the API. This helps maintain consistency and prevents errors during data processing.
- Error Handling: Implement robust error handling to manage different HTTP status codes. This includes logging errors and providing meaningful feedback to users or systems.
Enhancing Integration with Endgrate
For developers looking to simplify and scale their integration efforts, consider using Endgrate. With Endgrate, you can streamline your integration processes, allowing you to focus on your core product development. Endgrate offers a unified API endpoint that connects to multiple platforms, including Front, providing an intuitive integration experience for your customers.
By leveraging Endgrate, you can build once for each use case and avoid the complexity of managing multiple integrations. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
- https://endgrate.com/provider/front
- https://dev.frontapp.com/docs/welcome
- https://dev.frontapp.com/docs/core-api-getting-started
- https://dev.frontapp.com/docs/oauth
- https://dev.frontapp.com/docs/rate-limiting
- https://dev.frontapp.com/docs/pagination
- https://dev.frontapp.com/reference/create-account
- https://dev.frontapp.com/reference/update-account
Ready to get started?