How to Get Report with the AppFolio API in PHP
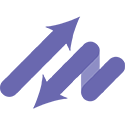
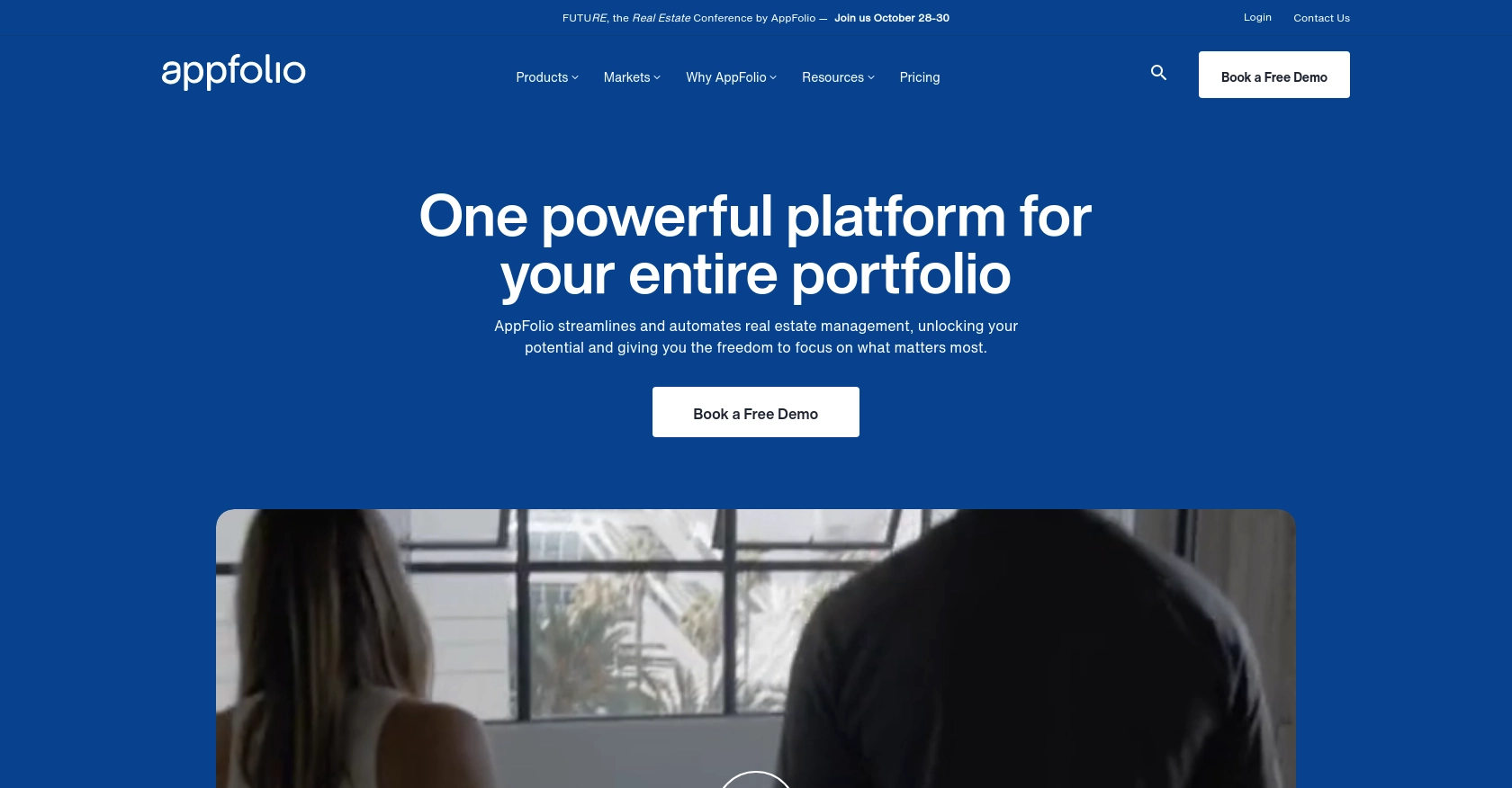
Introduction to AppFolio API Integration
AppFolio is a comprehensive property management software that offers a wide range of solutions for real estate professionals. It provides tools for marketing, leasing, accounting, and reporting, making it a popular choice for property managers looking to streamline their operations.
Developers may want to integrate with the AppFolio API to automate and enhance reporting capabilities. For example, a developer could use the AppFolio API to automatically generate and retrieve property management reports, which can then be used for data analysis and decision-making.
This article will guide you through the process of using PHP to interact with the AppFolio API, specifically focusing on retrieving reports. By following this tutorial, you'll learn how to efficiently access and manage property data using AppFolio's robust platform.
Setting Up Your AppFolio Test Account for API Integration
Before you can start interacting with the AppFolio API using PHP, you'll need to set up your AppFolio account. This involves creating reports and scheduling them to be sent via email, as AppFolio's integration process relies on handling CSV reports sent through email.
Accessing the AppFolio Property Manager Portal
To begin, log in to your AppFolio Property Manager Portal. You can access the portal by visiting the following link: Go to AppFolio Property Manager Portal.
Creating and Sending Reports in CSV Format
- Once logged in, create the appropriate report you wish to automate.
- Click on Actions and select Email to send the report in CSV format to the designated email address.
- Next, click on Actions again and choose Save Layout. Save the layout with a name of your choice for future use.
Scheduling Reports for Automated Delivery
- Navigate back to your Property Manager portal and go to Reporting → Scheduled Reports.
- Create a new scheduled report and set it to send daily to the same email address as before, ensuring the format is CSV.
- The body and subject of the email can be customized as needed.
By following these steps, you will have set up your AppFolio account to automatically send reports, which can then be processed using PHP to extract and analyze the data.
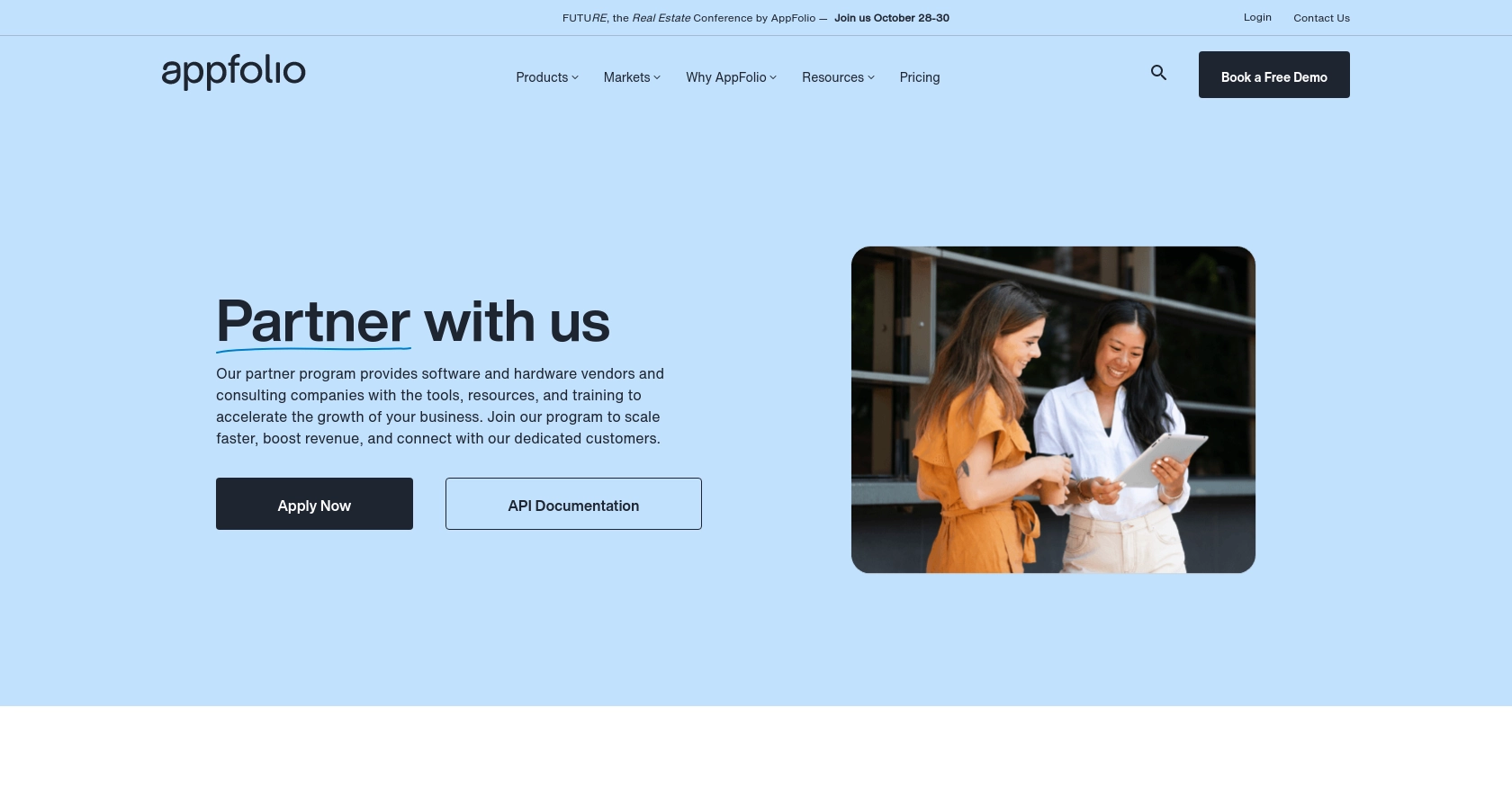
sbb-itb-96038d7
Making API Calls to Retrieve Reports from AppFolio Using PHP
To interact with the AppFolio API and retrieve reports, you'll need to use PHP to process the CSV reports sent via email. This section will guide you through setting up your PHP environment and handling the CSV data effectively.
Setting Up Your PHP Environment for AppFolio API Integration
Before you start coding, ensure you have the following prerequisites installed on your system:
- PHP 7.4 or higher
- Composer for dependency management
You'll also need to install the phpmailer/phpmailer
package to handle email processing. Run the following command in your terminal:
composer require phpmailer/phpmailer
Processing CSV Reports Sent from AppFolio
Once you've set up your environment, you can create a PHP script to process the CSV reports. The script will connect to your email server, retrieve the emails containing the reports, and parse the CSV data.
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
// Load Composer's autoloader
require 'vendor/autoload.php';
// Email configuration
$email = 'your-email@example.com';
$password = 'your-email-password';
$host = 'your-email-host';
// Connect to the email server
$mail = new PHPMailer(true);
$mail->isSMTP();
$mail->Host = $host;
$mail->SMTPAuth = true;
$mail->Username = $email;
$mail->Password = $password;
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
try {
// Fetch emails
$mail->setFrom($email);
$mail->addAddress($email);
$mail->Subject = 'AppFolio Report';
$mail->Body = 'Please find the attached report.';
// Process the CSV attachment
$attachments = $mail->getAttachments();
foreach ($attachments as $attachment) {
if (pathinfo($attachment['name'], PATHINFO_EXTENSION) === 'csv') {
$csvData = file_get_contents($attachment['path']);
$rows = array_map('str_getcsv', explode("\n", $csvData));
$header = array_shift($rows);
foreach ($rows as $row) {
$reportData = array_combine($header, $row);
// Process each row of data
print_r($reportData);
}
}
}
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
In this script, we use PHPMailer to connect to the email server and retrieve the emails containing the CSV reports. The script then reads the CSV file, parses the data, and processes each row for further analysis or storage.
Verifying Successful Data Retrieval from AppFolio
After running the script, you should verify that the data has been correctly retrieved and processed. Check the output in your terminal to ensure the CSV data is displayed as expected. You can also log the data to a file or database for further analysis.
Handling Errors and Troubleshooting
While processing the CSV reports, you may encounter errors such as connection issues or malformed CSV data. Ensure you handle exceptions in your PHP script to catch and log any errors. This will help you troubleshoot and resolve issues efficiently.
By following these steps, you can automate the retrieval and processing of AppFolio reports using PHP, enhancing your property management capabilities.
Conclusion and Best Practices for AppFolio API Integration Using PHP
Integrating with the AppFolio API using PHP offers a powerful way to automate and enhance property management reporting. By following the steps outlined in this guide, you can efficiently retrieve and process reports, enabling data-driven decision-making for your real estate operations.
Best Practices for Secure and Efficient AppFolio API Integration
- Secure Email Credentials: Always store email credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by your email provider to avoid disruptions in report retrieval.
- Data Standardization: Ensure that the CSV data is standardized and validated before processing to maintain data integrity.
- Error Handling: Implement robust error handling to catch and log any issues during email retrieval or CSV processing.
Enhancing Integration Capabilities with Endgrate
For developers looking to streamline multiple integrations, Endgrate offers a unified API solution that simplifies the process. By using Endgrate, you can save time and resources, allowing you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can help you manage integrations more efficiently by visiting Endgrate.
Read More
Ready to get started?