How to Get Purchase Orders with the Xero API in PHP
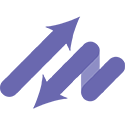
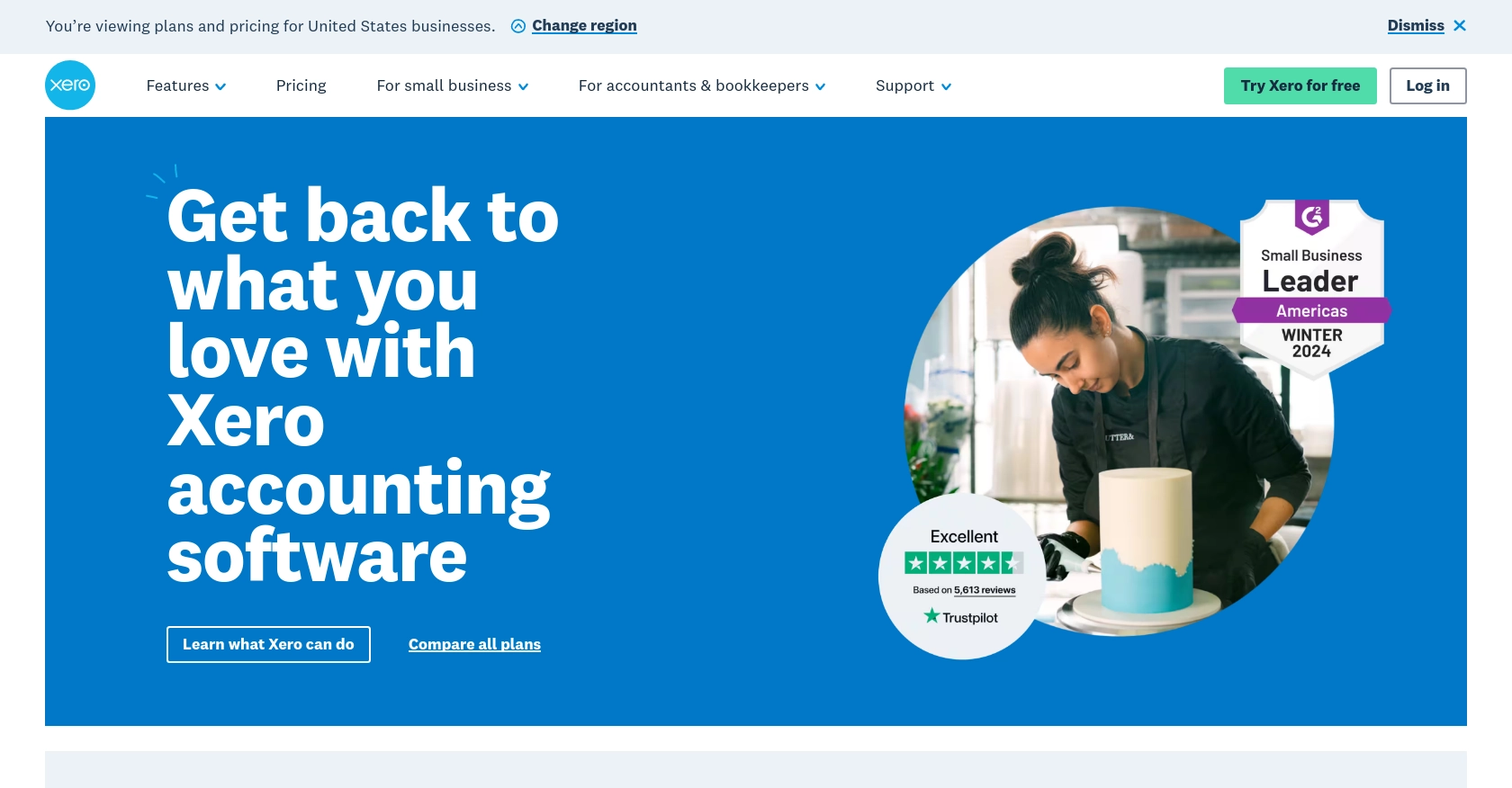
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to simplify financial management for small and medium-sized businesses. It offers a wide range of features, including invoicing, bank reconciliation, and financial reporting, making it a popular choice for businesses looking to streamline their accounting processes.
Integrating with Xero's API allows developers to automate and enhance financial workflows by accessing and managing accounting data programmatically. For example, a developer might use the Xero API to retrieve purchase orders, enabling seamless integration with inventory management systems or financial reporting tools.
This article will guide you through the process of using PHP to interact with the Xero API, specifically focusing on retrieving purchase orders. By following this tutorial, you'll learn how to efficiently access and manage purchase order data within the Xero platform using PHP.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with the Xero API using PHP, you'll need to set up a sandbox account. This will allow you to test your API calls without affecting live data. Xero provides a demo company that you can use for this purpose.
Follow these steps to set up your Xero sandbox account:
- Create a Xero Account: If you don't already have a Xero account, sign up for a free trial on the Xero website. If you already have an account, simply log in.
- Access the Demo Company: Once logged in, navigate to the dashboard and select the option to use the demo company. This will provide you with a sandbox environment to test your API interactions.
Creating a Xero App for OAuth 2.0 Authentication
To interact with the Xero API, you'll need to create an app in the Xero Developer portal. This app will provide the necessary credentials for OAuth 2.0 authentication.
- Register Your App: Go to the Xero Developer portal and click on "New App". Fill in the required details, such as the app name and company URL.
- Set Redirect URI: Specify a redirect URI for your app. This is where users will be redirected after they authorize your app. For testing purposes, you can use
http://localhost
. - Obtain Client ID and Secret: After creating the app, you'll receive a client ID and client secret. Keep these credentials secure, as they are necessary for authenticating API requests.
Configuring OAuth 2.0 Scopes for Xero API Access
To access purchase orders, you'll need to configure the appropriate scopes for your app. Scopes define the level of access your app has to the Xero API.
- Navigate to Scopes: In the Xero Developer portal, go to your app's settings and find the "Scopes" section.
- Select Required Scopes: Ensure that you select the scopes necessary for accessing purchase orders. Refer to the Xero OAuth 2.0 Scopes documentation for more details.
With your sandbox account and app set up, you're now ready to start making API calls to retrieve purchase orders using PHP. In the next section, we'll walk through the process of making these calls and handling the responses.
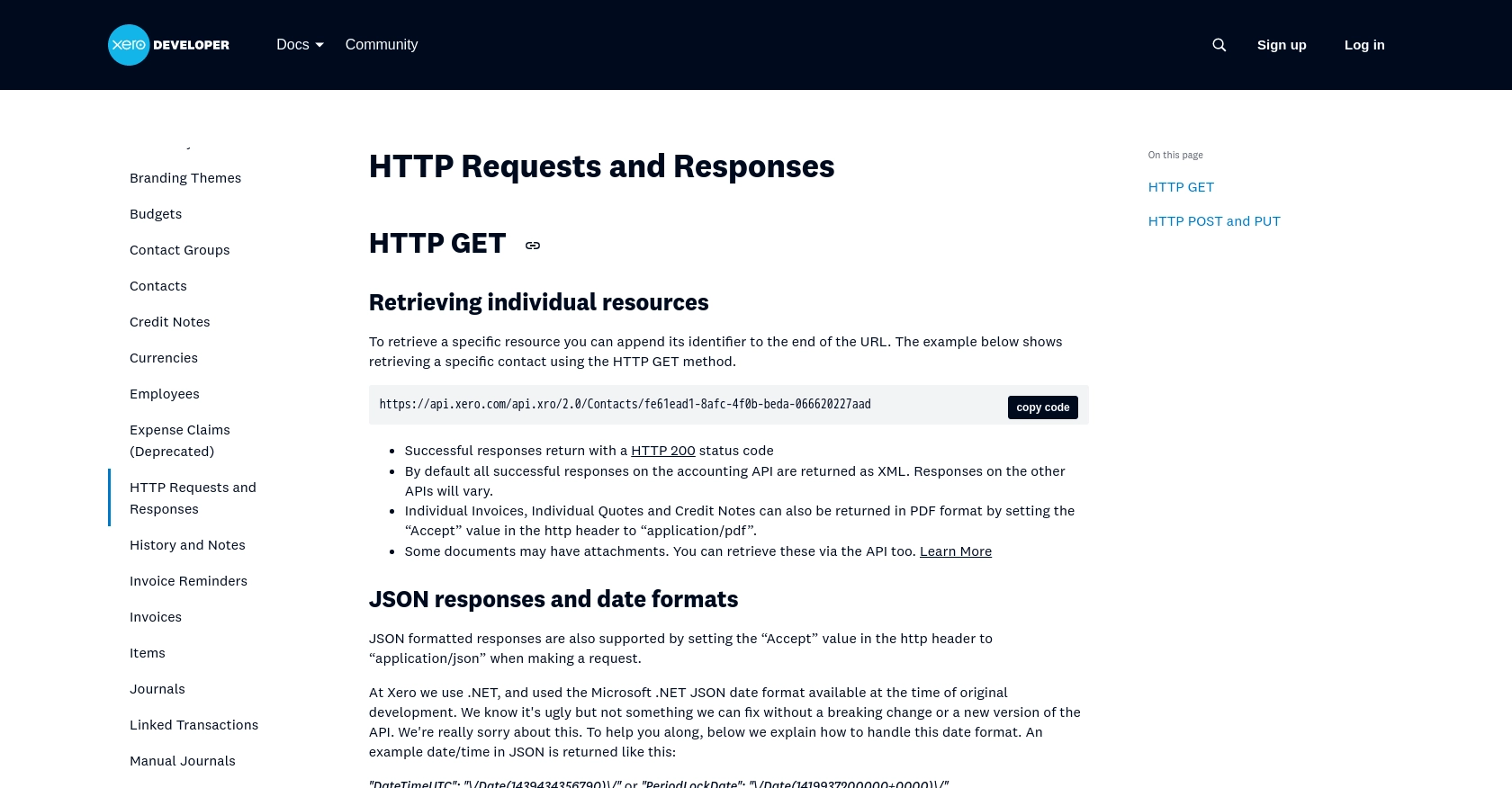
sbb-itb-96038d7
Making API Calls to Retrieve Purchase Orders from Xero Using PHP
Now that you have set up your Xero sandbox account and created an app for OAuth 2.0 authentication, it's time to make API calls to retrieve purchase orders using PHP. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to interact with the Xero API, and handling responses.
Setting Up Your PHP Environment for Xero API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP 7.4 or later and the Composer package manager to manage dependencies.
- Install PHP: Make sure PHP is installed on your system. You can download it from the official PHP website.
- Install Composer: Download and install Composer from the Composer website. This tool will help you manage the required libraries.
Installing Required PHP Libraries for Xero API
To interact with the Xero API, you'll need the Guzzle HTTP client and the Xero PHP SDK. Use Composer to install these libraries.
composer require guzzlehttp/guzzle
composer require xeroapi/xero-php-oauth2
Writing PHP Code to Retrieve Purchase Orders from Xero
With the necessary libraries installed, you can now write the PHP code to retrieve purchase orders from Xero. Create a new PHP file and add the following code:
require 'vendor/autoload.php';
use XeroAPI\XeroPHP\AccountingApi;
use XeroAPI\XeroPHP\Configuration;
use XeroAPI\XeroPHP\ApiException;
use GuzzleHttp\Client;
// Set up the configuration
$config = Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
// Create a new API client
$apiInstance = new AccountingApi(
new Client(),
$config
);
try {
// Fetch purchase orders
$result = $apiInstance->getPurchaseOrders('YOUR_TENANT_ID');
print_r($result);
} catch (ApiException $e) {
echo 'Exception when calling AccountingApi->getPurchaseOrders: ', $e->getMessage(), PHP_EOL;
}
Replace YOUR_ACCESS_TOKEN
and YOUR_TENANT_ID
with your actual access token and tenant ID obtained from the Xero Developer portal.
Verifying API Call Success and Handling Errors
After running the PHP script, you should see the purchase orders from your Xero sandbox account printed in the console. If the request is successful, the data will match the purchase orders in your sandbox environment.
In case of errors, the ApiException
will catch them, and you can handle them accordingly. Refer to the Xero API documentation for more information on error codes and their meanings.
By following these steps, you can efficiently retrieve purchase orders from Xero using PHP, allowing you to integrate this data into your applications or workflows seamlessly.
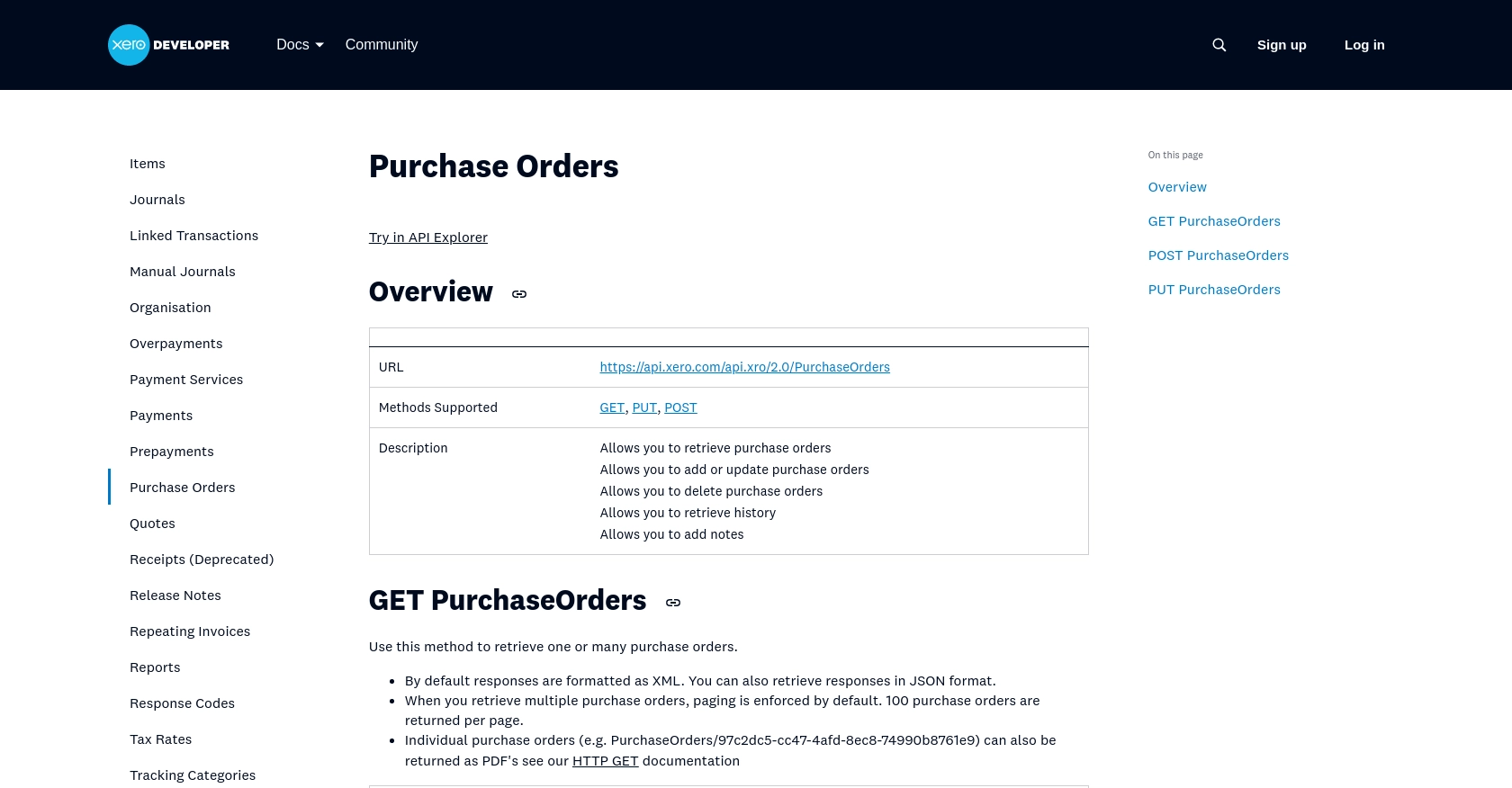
Conclusion: Best Practices for Xero API Integration Using PHP
Integrating with the Xero API using PHP provides a powerful way to automate and enhance your financial workflows. By following the steps outlined in this article, you can efficiently retrieve purchase orders and integrate them into your applications or systems.
Best Practices for Secure and Efficient Xero API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of Xero's rate limits to avoid throttling. According to the Xero API documentation, ensure your application handles rate limit responses gracefully.
- Transform and Standardize Data: When integrating purchase order data, ensure that you transform and standardize fields to match your application's requirements.
- Monitor API Changes: Regularly check the Xero API documentation for updates or changes that may affect your integration.
By adhering to these best practices, you can ensure a robust and secure integration with Xero's API, enhancing your application's capabilities and providing a seamless experience for your users.
Streamline Your Integration Process with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case, reducing the complexity of maintaining multiple integrations. Focus on your core product while Endgrate handles the intricacies of API connections.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/purchaseorders
Ready to get started?