Using the Pipedrive API to Get Leads in PHP
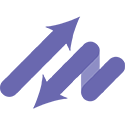
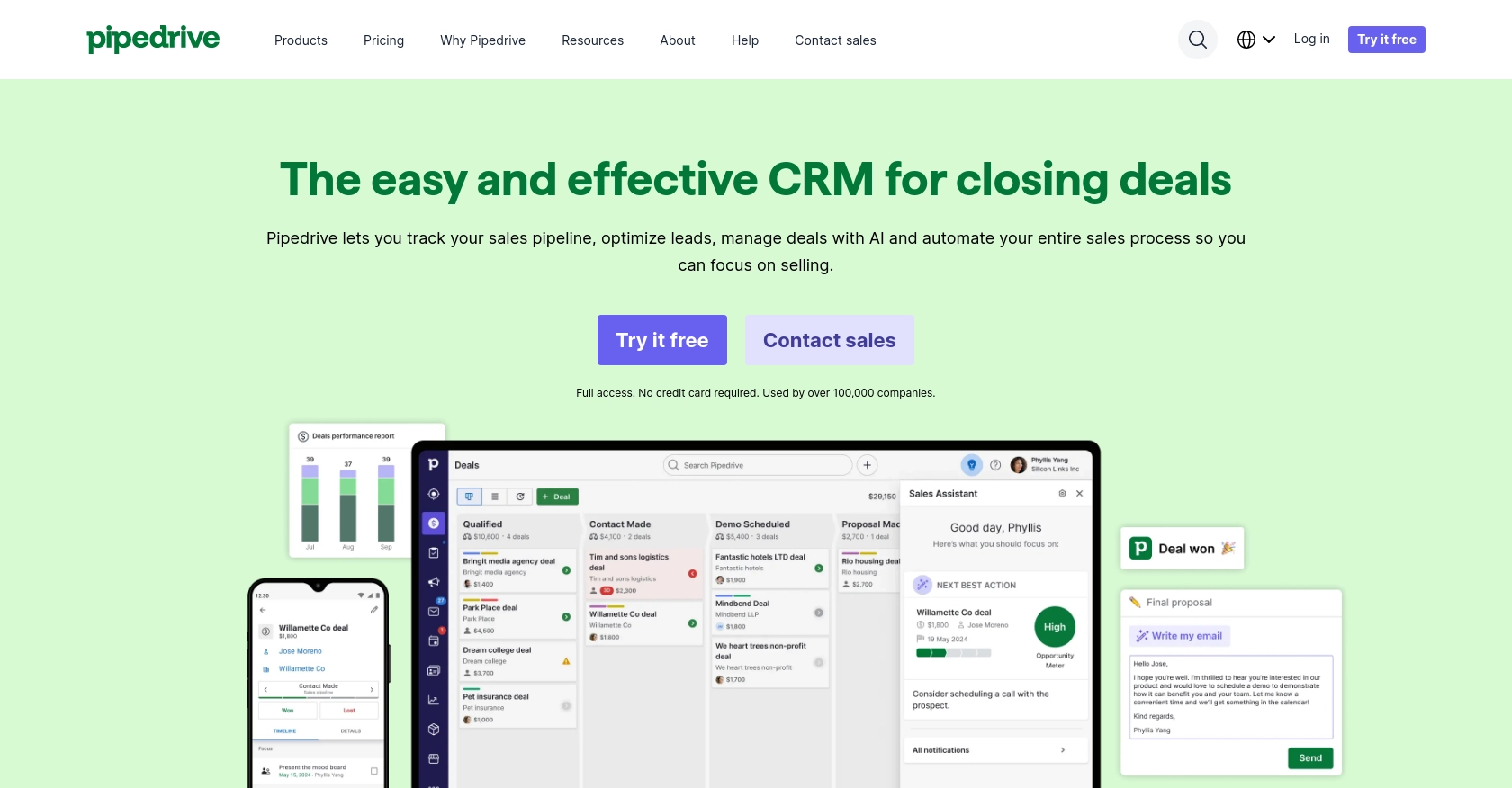
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes efficiently. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage deals, and automate workflows, making it a popular choice for businesses aiming to enhance their sales operations.
Developers may want to integrate with Pipedrive's API to streamline sales processes by automating lead management. For example, using the Pipedrive API, a developer can retrieve leads and integrate them into a custom dashboard, providing sales teams with real-time insights and improving decision-making.
Setting Up Your Pipedrive Developer Sandbox Account
Before diving into the integration process, it's essential to set up a Pipedrive developer sandbox account. This environment allows you to test and develop your application in a risk-free setting, ensuring that your integration works seamlessly without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To begin, you'll need to create a developer sandbox account with Pipedrive. This account mimics a regular Pipedrive company account but is specifically designed for development and testing purposes.
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to your sandbox account.
- Once approved, you will have access to a safe environment with sample data to test your integration.
Generating OAuth Credentials for Pipedrive API
Pipedrive uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to generate your client ID and client secret:
- Log in to your Pipedrive sandbox account.
- Navigate to the Developer Hub and register your app.
- During registration, you will receive a client ID and client secret, which are crucial for the OAuth flow.
- Ensure your app has the appropriate scopes and permissions for accessing leads data.
For more detailed instructions, refer to the Pipedrive App Creation Guide.
Importing Sample Data into Your Sandbox
To effectively test your integration, you can import sample data into your sandbox account:
- Go to the Pipedrive web app and click on “...” (More) > Import data > From a spreadsheet.
- Choose a template spreadsheet with sample data to import.
- This data will help you simulate real-world scenarios while testing your API calls.
With your sandbox account set up and OAuth credentials in hand, you're ready to start integrating with the Pipedrive API to manage leads efficiently.
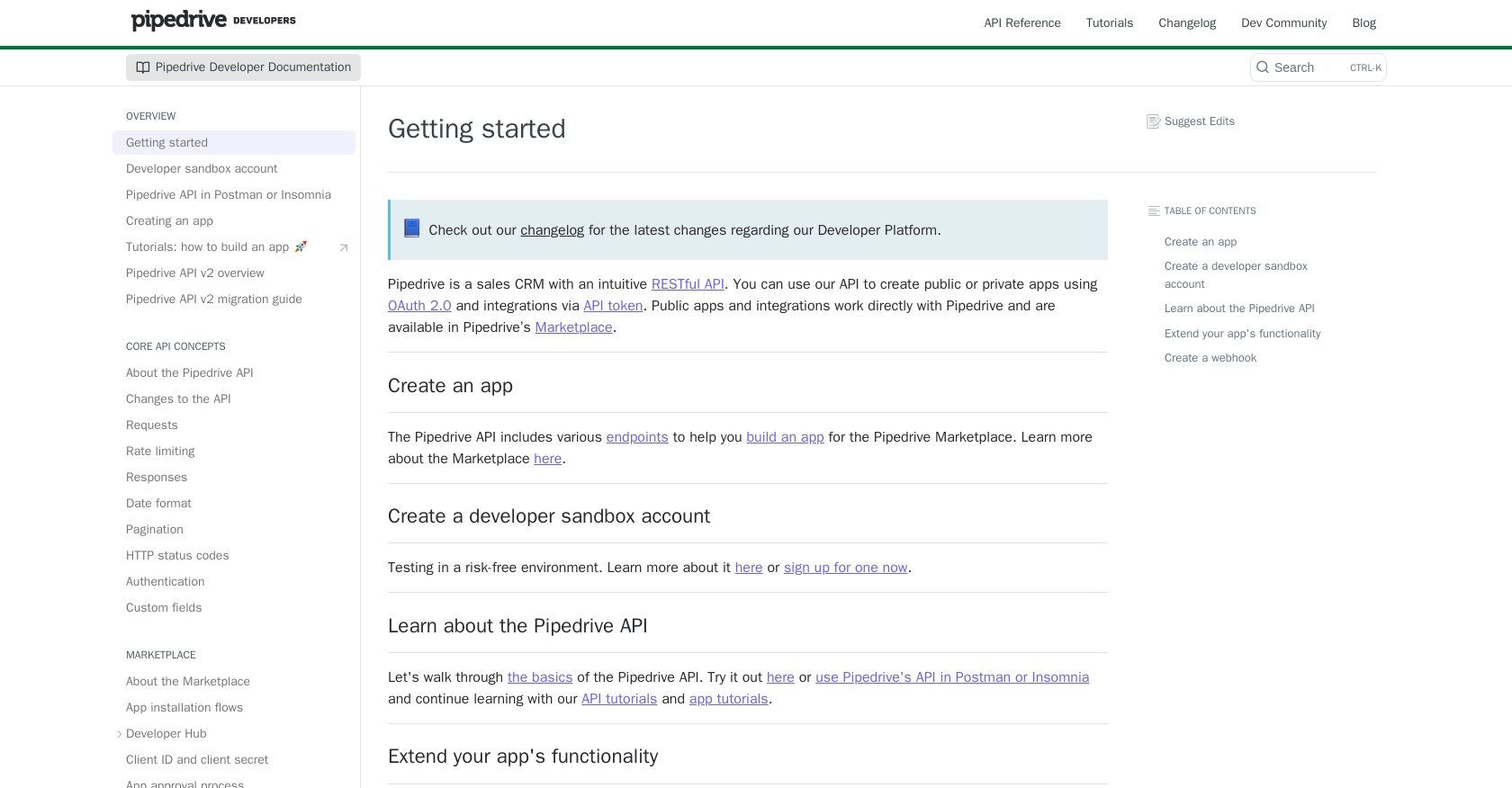
sbb-itb-96038d7
Making API Calls to Retrieve Leads from Pipedrive Using PHP
To interact with the Pipedrive API and retrieve leads, you need to set up your PHP environment and make HTTP requests to the appropriate endpoints. This section will guide you through the process of making API calls using PHP, ensuring you can efficiently access lead data from Pipedrive.
Setting Up Your PHP Environment for Pipedrive API Integration
Before making API calls, ensure your PHP environment is correctly configured:
- Ensure you have PHP 7.4 or later installed on your system.
- Install the
cURL
extension for PHP, which is essential for making HTTP requests.
To verify your PHP version and installed extensions, use the following command:
php -v
php -m | grep curl
Installing Required PHP Dependencies
To handle HTTP requests, you can use the Guzzle
library, a popular PHP HTTP client:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Leads from Pipedrive
With your environment set up, you can now write PHP code to make API calls to Pipedrive:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your OAuth access token
try {
$response = $client->request('GET', 'https://api.pipedrive.com/v1/leads', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['data'] as $lead) {
echo 'Lead Title: ' . $lead['title'] . "\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
In this code:
- We use the
Guzzle
client to make a GET request to the Pipedrive leads endpoint. - The
Authorization
header includes the OAuth access token. - We parse the JSON response and loop through the leads to display their titles.
Handling API Response and Errors
After making the API call, it's crucial to handle the response and any potential errors:
- Check the HTTP status code to ensure the request was successful (status code 200).
- Handle exceptions to catch any errors during the request.
Refer to the Pipedrive HTTP Status Codes documentation for more details on handling different response codes.
Verifying API Call Success in Pipedrive Sandbox
To verify that your API call was successful, log in to your Pipedrive sandbox account and check the Leads section. The leads retrieved by your PHP script should match those displayed in the sandbox environment.
By following these steps, you can effectively integrate with the Pipedrive API using PHP, enabling seamless lead management and data retrieval.
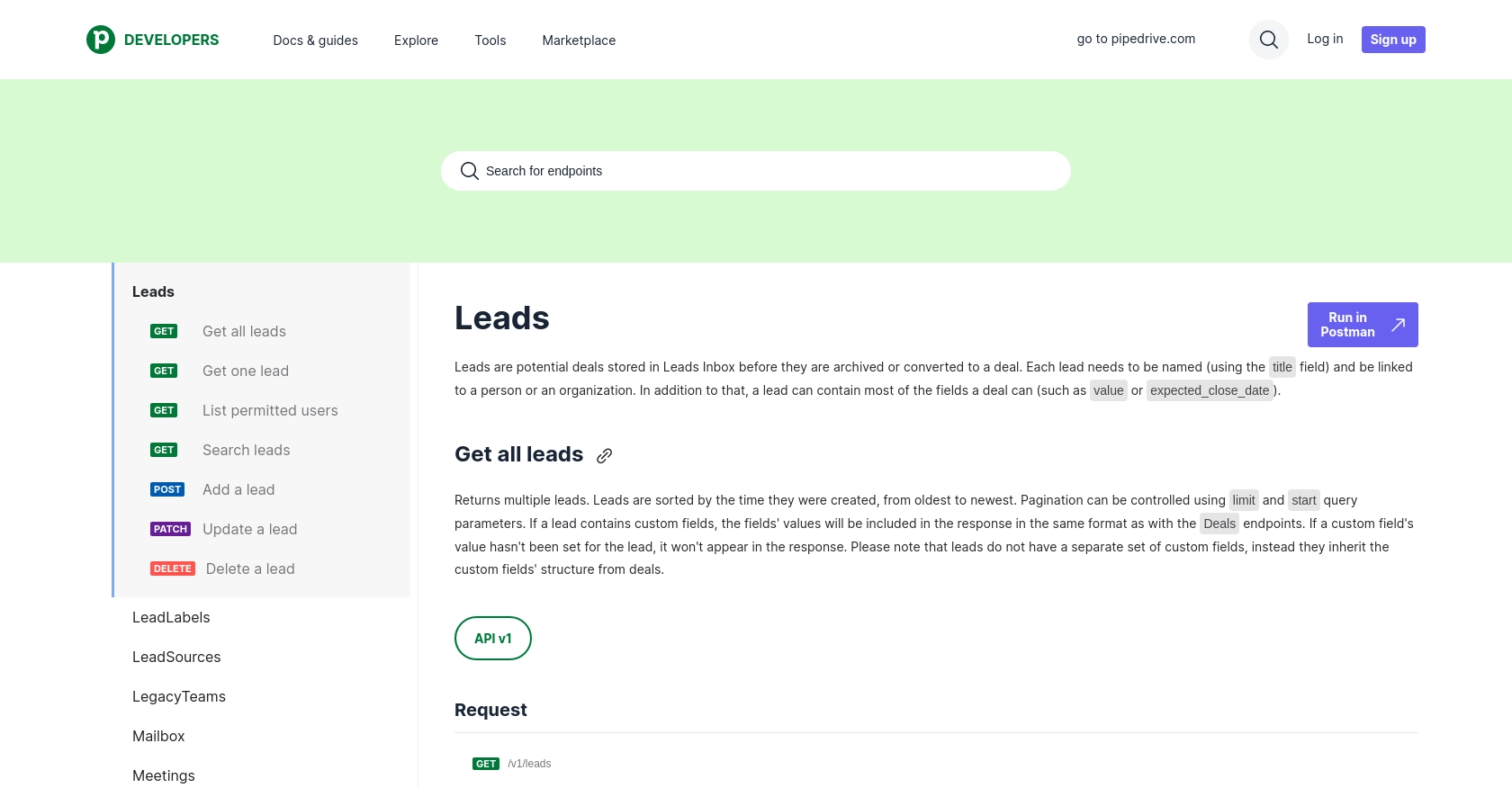
Best Practices for Pipedrive API Integration in PHP
Integrating with the Pipedrive API using PHP can significantly enhance your sales processes by automating lead management and providing real-time insights. Here are some best practices to ensure a smooth and efficient integration:
Securely Storing OAuth Credentials
- Store your OAuth credentials, such as the client ID and client secret, securely in environment variables or a secure vault.
- Avoid hardcoding sensitive information directly in your codebase to prevent unauthorized access.
Handling Pipedrive API Rate Limits
Pipedrive imposes rate limits on API requests to ensure fair usage. For OAuth apps, the rate limit is up to 480 requests per 2 seconds per access token, depending on your plan. To manage rate limits effectively:
- Implement exponential backoff strategies to retry requests when rate limits are exceeded.
- Monitor the
x-ratelimit-remaining
header to track your remaining requests.
For more details, refer to the Pipedrive Rate Limiting documentation.
Transforming and Standardizing Data Fields
- Ensure data consistency by transforming and standardizing fields before storing them in your database.
- Map Pipedrive fields to your application's data model to maintain uniformity across systems.
Utilizing Endgrate for Simplified Integrations
If managing multiple integrations becomes overwhelming, consider using Endgrate. With Endgrate, you can streamline your integration process by connecting to multiple platforms through a single API endpoint. This allows you to focus on your core product while outsourcing complex integrations.
Explore how Endgrate can save you time and resources by visiting Endgrate.
By following these best practices, you can ensure a robust and efficient integration with the Pipedrive API, enhancing your sales operations and providing valuable insights to your team.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Leads
Ready to get started?