Using the Zoho CRM API to Get Custom Objects in Javascript
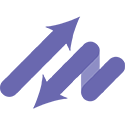
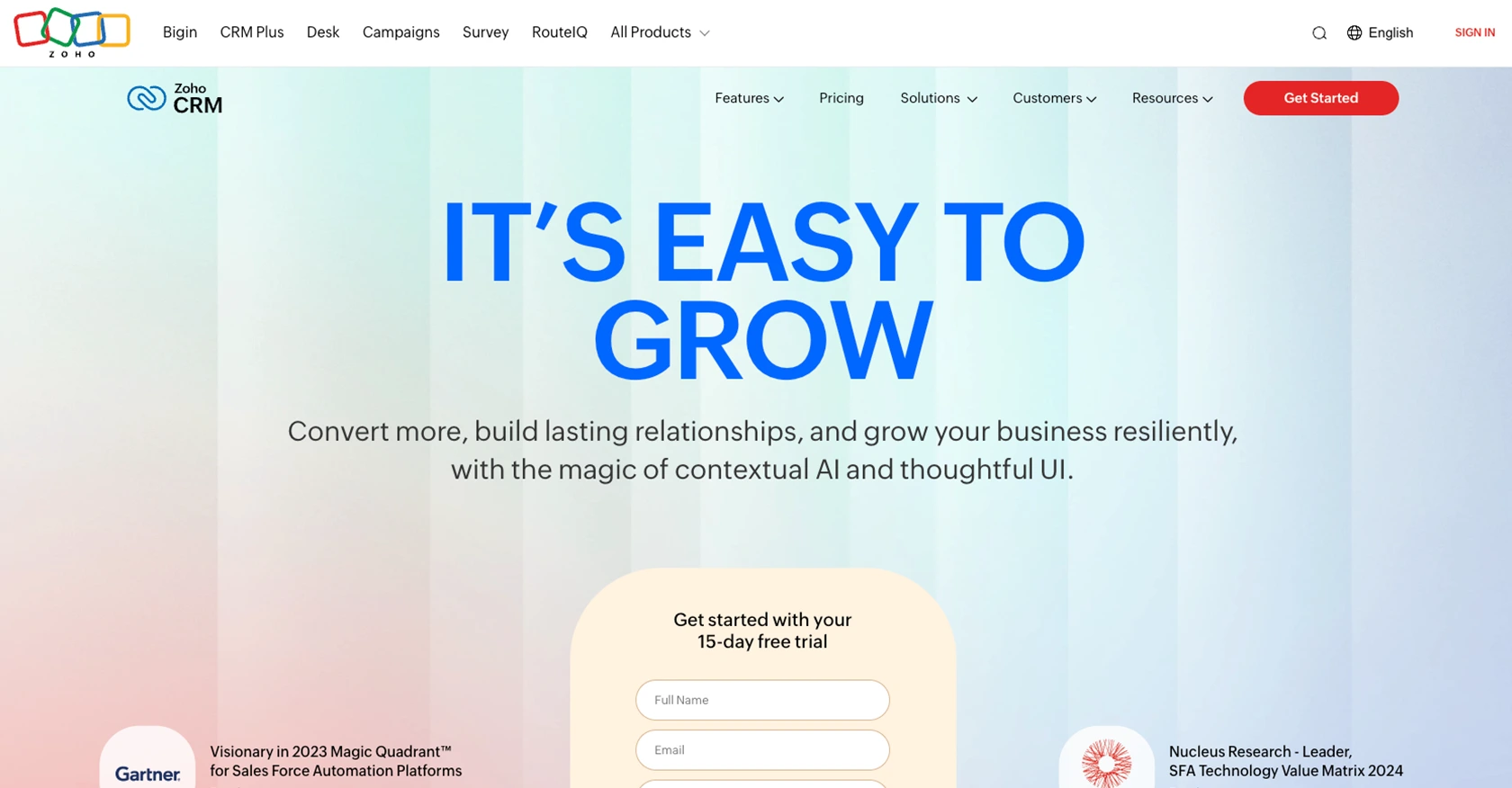
Introduction to Zoho CRM and Custom Objects
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and customer support in a unified manner. Known for its flexibility and extensive features, Zoho CRM is a popular choice for businesses aiming to enhance their customer interactions and streamline operations.
Integrating with Zoho CRM's API allows developers to access and manipulate data within the CRM, enabling automation and customization of workflows. For example, a developer might want to retrieve custom objects from Zoho CRM to analyze customer-specific data or to integrate with other business applications, enhancing the overall efficiency of business processes.
This article will guide you through using JavaScript to interact with Zoho CRM's API, specifically focusing on retrieving custom objects. By following this tutorial, you will learn how to set up the necessary environment, authenticate using OAuth, and execute API calls to access custom objects within Zoho CRM.
Setting Up Your Zoho CRM Test/Sandbox Account
Creating a Zoho CRM Account for API Integration
Before you can start interacting with Zoho CRM's API, you need to set up a Zoho CRM account. If you don't have one, you can sign up for a free trial or use the free version available on the Zoho CRM website. This will allow you to explore the platform and test API integrations without any initial cost.
- Visit the Zoho CRM sign-up page.
- Fill in the required details and follow the instructions to create your account.
- Once your account is created, log in to access the Zoho CRM dashboard.
Registering a Client Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials. Follow these steps to register your client application:
- Go to the Zoho Developer Console.
- Select the appropriate client type for your application. For JavaScript applications, choose "Java Script".
- Enter the following details:
- Client Name: A name for your application.
- Homepage URL: The URL of your web page.
- Authorized Redirect URIs: A valid URL where Zoho will redirect with a grant token after successful authentication.
- Click on Create to register your application.
Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Generating OAuth Tokens for Zoho CRM API Access
With your client application registered, you can now generate OAuth tokens to authenticate API requests:
- Make an authorization request to Zoho's OAuth server using the following URL format:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoCRM.modules.ALL&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
- After user consent, Zoho will redirect to your specified URI with an authorization code.
- Exchange the authorization code for access and refresh tokens by making a POST request to:
https://accounts.zoho.com/oauth/v2/token?grant_type=authorization_code&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&code=AUTHORIZATION_CODE
Store the access token securely as it will be used to authenticate your API calls. Note that access tokens expire after an hour, but you can use the refresh token to obtain new access tokens without user intervention.
For more details on OAuth authentication, refer to the Zoho CRM OAuth documentation.
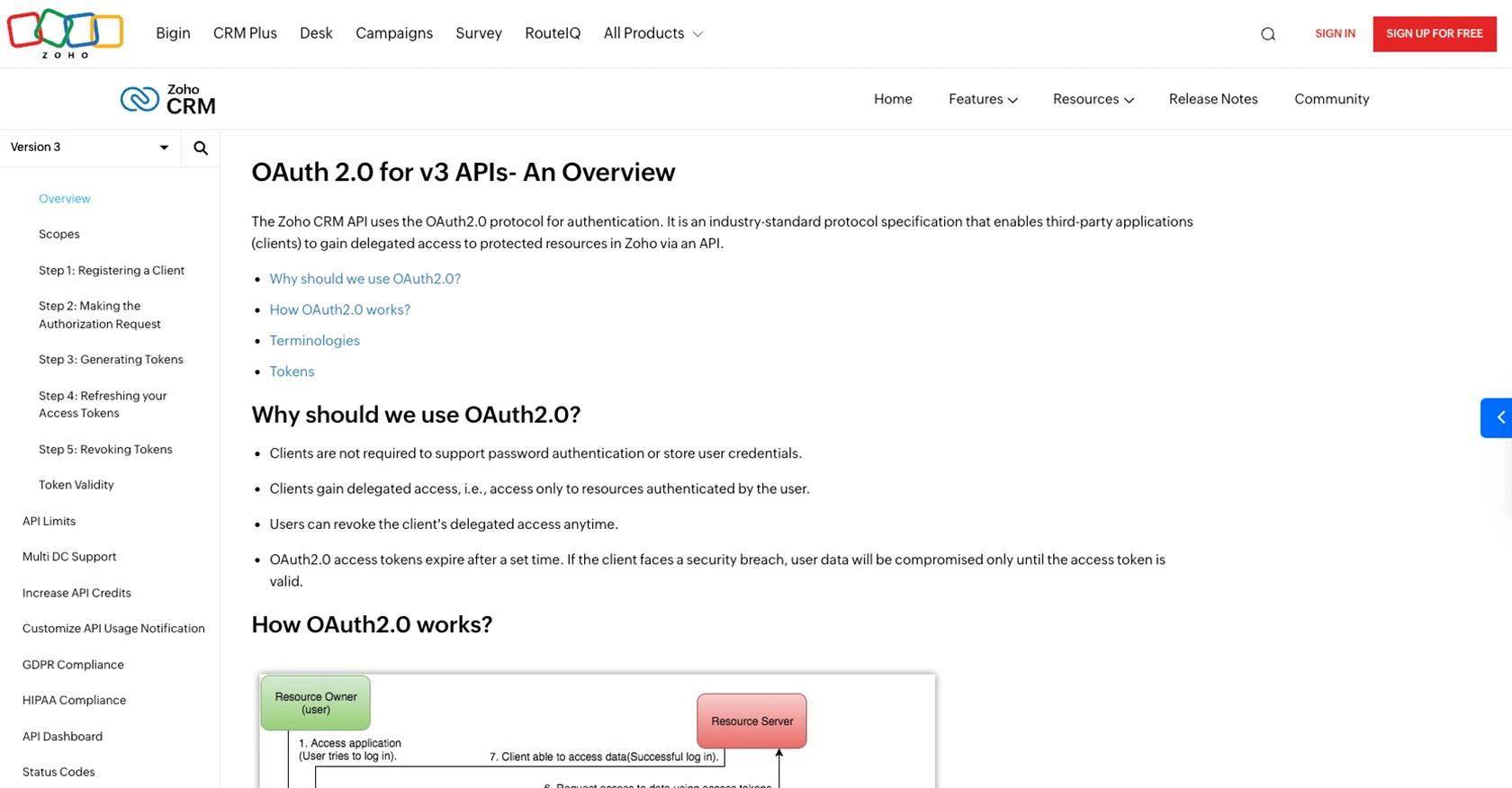
sbb-itb-96038d7
Making API Calls to Retrieve Custom Objects from Zoho CRM Using JavaScript
To interact with Zoho CRM's API and retrieve custom objects using JavaScript, you'll need to set up your environment and write the necessary code to make API requests. This section will guide you through the process, including setting up JavaScript dependencies, writing the code to make API calls, and handling responses and errors.
Setting Up Your JavaScript Environment for Zoho CRM API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code or any other of your choice.
Once Node.js is installed, you can use npm (Node Package Manager) to install the necessary packages:
npm install axios
The axios
library will be used to make HTTP requests to Zoho CRM's API.
Writing JavaScript Code to Retrieve Custom Objects from Zoho CRM
With your environment set up, you can now write the JavaScript code to retrieve custom objects from Zoho CRM. Create a new JavaScript file, for example, getCustomObjects.js
, and add the following code:
const axios = require('axios');
// Replace with your actual access token
const accessToken = 'YOUR_ACCESS_TOKEN';
// Replace 'CustomModule' with your actual custom module API name
const moduleName = 'CustomModule';
// Zoho CRM API endpoint for retrieving records
const endpoint = `https://www.zohoapis.com/crm/v3/${moduleName}`;
async function getCustomObjects() {
try {
const response = await axios.get(endpoint, {
headers: {
Authorization: `Zoho-oauthtoken ${accessToken}`
}
});
console.log('Custom Objects:', response.data);
} catch (error) {
console.error('Error fetching custom objects:', error.response ? error.response.data : error.message);
}
}
getCustomObjects();
In this code, replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth authentication process. Also, replace CustomModule
with the API name of the custom module you wish to access.
Executing the JavaScript Code and Handling API Responses
To execute the code, run the following command in your terminal:
node getCustomObjects.js
If successful, the console will display the custom objects retrieved from Zoho CRM. If there are any errors, they will be logged to the console, helping you diagnose issues such as invalid tokens or incorrect module names.
Verifying API Call Success and Handling Errors
After running the code, verify the success of your API call by checking the console output. The data should match the custom objects within your Zoho CRM sandbox account. If you encounter errors, refer to the following common error codes and their resolutions:
- 401 Unauthorized: Ensure your access token is valid and not expired. Refresh the token if necessary.
- 404 Not Found: Check that the module name is correct and exists in your Zoho CRM account.
- 429 Too Many Requests: You have exceeded the API rate limit. Wait and try again later.
For more detailed error information, refer to the Zoho CRM API status codes documentation.
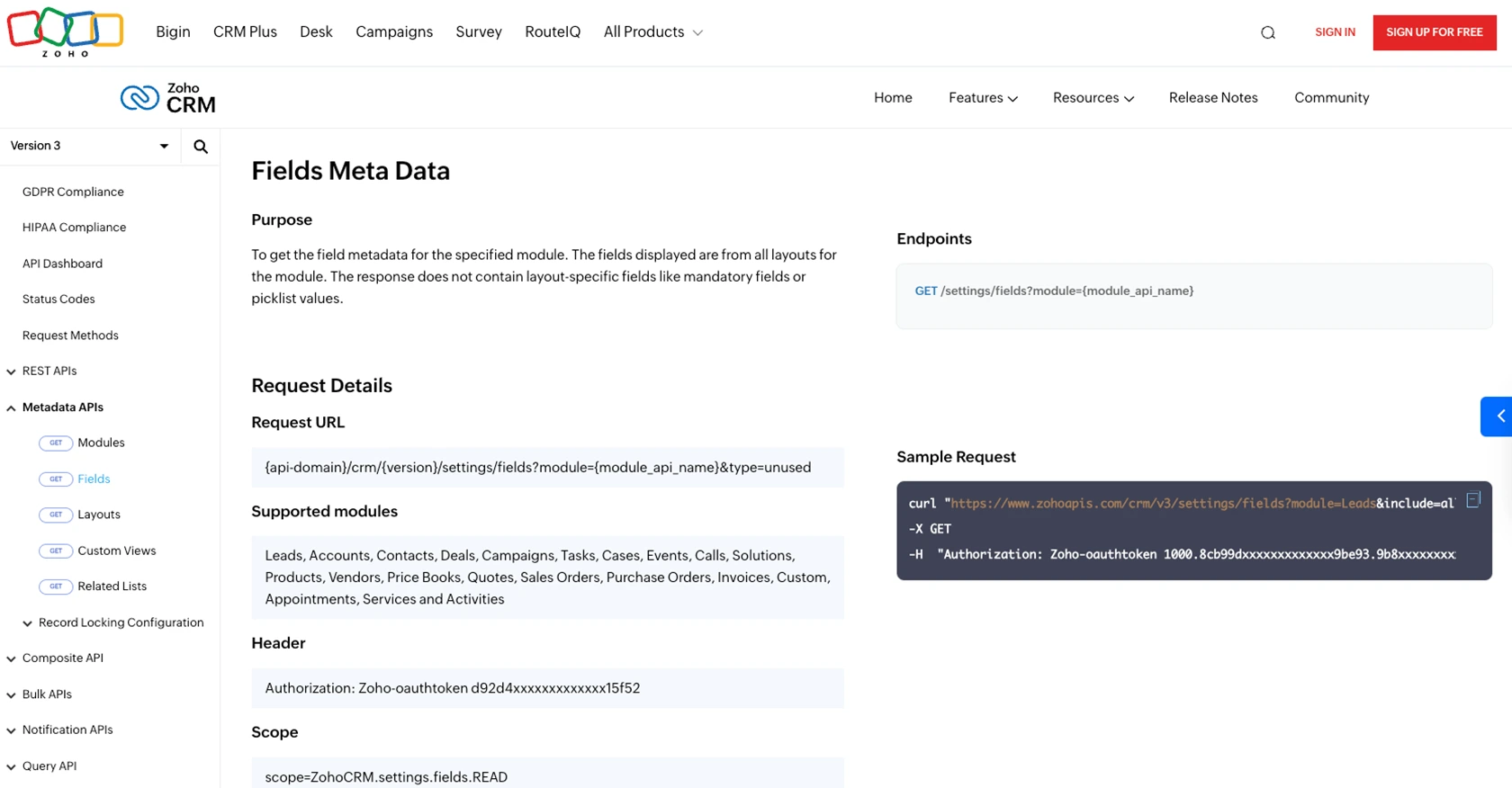
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with Zoho CRM's API using JavaScript provides a powerful way to enhance your business processes by automating data retrieval and manipulation. By following the steps outlined in this guide, you can efficiently access custom objects and integrate them with other business applications, thereby improving operational efficiency.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, such as the Client ID, Client Secret, and access tokens, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handling Rate Limits: Zoho CRM imposes API rate limits to ensure fair usage. Be mindful of these limits and implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Zoho CRM API limits documentation.
- Data Transformation and Standardization: When integrating data from Zoho CRM with other systems, ensure that data fields are transformed and standardized to maintain consistency across platforms.
Streamlining Integrations with Endgrate
While building integrations with Zoho CRM can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Zoho CRM. This allows developers to build once for each use case and leverage an intuitive integration experience for their customers.
By using Endgrate, you can save time and resources, allowing you to focus on your core product while ensuring seamless integrations. Explore how Endgrate can simplify your integration needs by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/module-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?