Using the Outreach API to Get Accounts in PHP
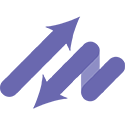
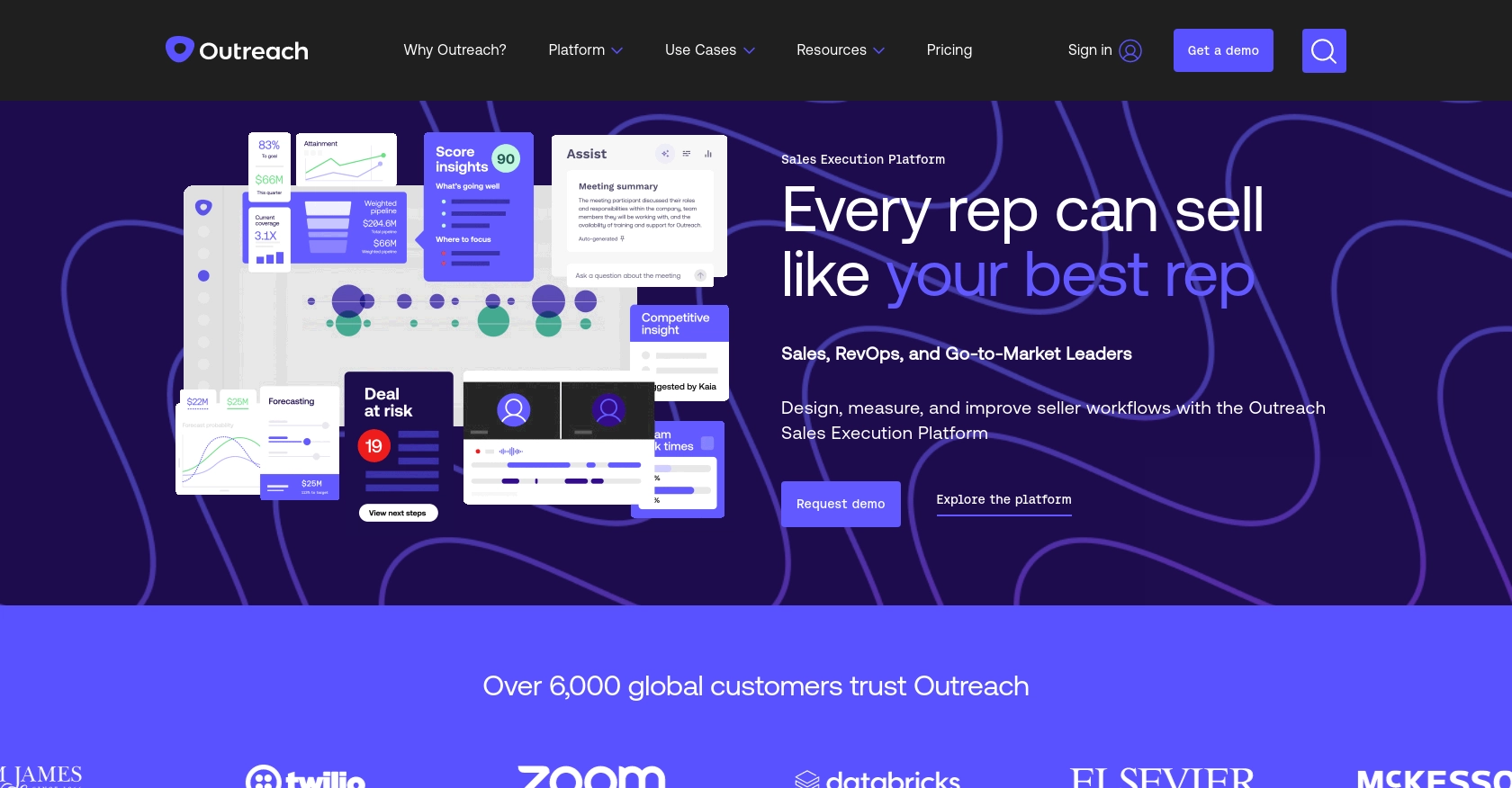
Introduction to Outreach API Integration
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve productivity. It offers a suite of tools designed to enhance communication, automate workflows, and provide valuable insights into sales performance.
Developers may want to integrate with the Outreach API to access and manage account data, enabling them to build custom solutions that enhance sales operations. For example, a developer could use the Outreach API to retrieve account information and synchronize it with other CRM systems, ensuring that sales teams have up-to-date data at their fingertips.
Setting Up Your Outreach Test/Sandbox Account
Before diving into the Outreach API integration, it's essential to set up a test or sandbox account. This allows developers to experiment with API calls without affecting live data. Outreach provides a development environment where you can safely test your integrations.
Creating an Outreach Developer Account
If you don't already have an Outreach account, start by signing up for a developer account on the Outreach website. This will give you access to the necessary tools and resources to begin your integration journey.
- Visit the Outreach Developer Portal.
- Sign up for a developer account by following the on-screen instructions.
- Once registered, log in to your account to access the development dashboard.
Creating an Outreach App for OAuth Authentication
To interact with the Outreach API, you'll need to create an app within your developer account. This app will provide the OAuth credentials required for authentication.
- Navigate to the "My Apps" section in your Outreach developer dashboard.
- Click on "Create New App" and fill in the required details such as app name and description.
- Specify the redirect URI(s) that your application will use. This is crucial for the OAuth flow.
- Select the necessary OAuth scopes that your application will require. Ensure you choose scopes related to account data access.
- Save your app to generate the client ID and client secret. Note that the client secret will only be displayed once, so store it securely.
Obtaining OAuth Tokens
With your app created, you can now obtain OAuth tokens to authenticate API requests. Follow these steps to complete the OAuth flow:
- Redirect users to the following URL for authorization:
- After user consent, Outreach will redirect to your specified URI with an authorization code.
- Exchange this code for an access token by making a POST request:
- Store the access token securely, as it will be used for subsequent API calls. Remember, the token is valid for a limited period and can be refreshed using the refresh token provided.
https://api.outreach.io/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code&scope=YOUR_SCOPES
curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=YOUR_CLIENT_ID \
-d client_secret=YOUR_CLIENT_SECRET \
-d redirect_uri=YOUR_REDIRECT_URI \
-d grant_type=authorization_code \
-d code=AUTHORIZATION_CODE
By following these steps, you'll have a fully configured Outreach sandbox environment, ready for testing and development. For more detailed information, refer to the Outreach OAuth documentation.
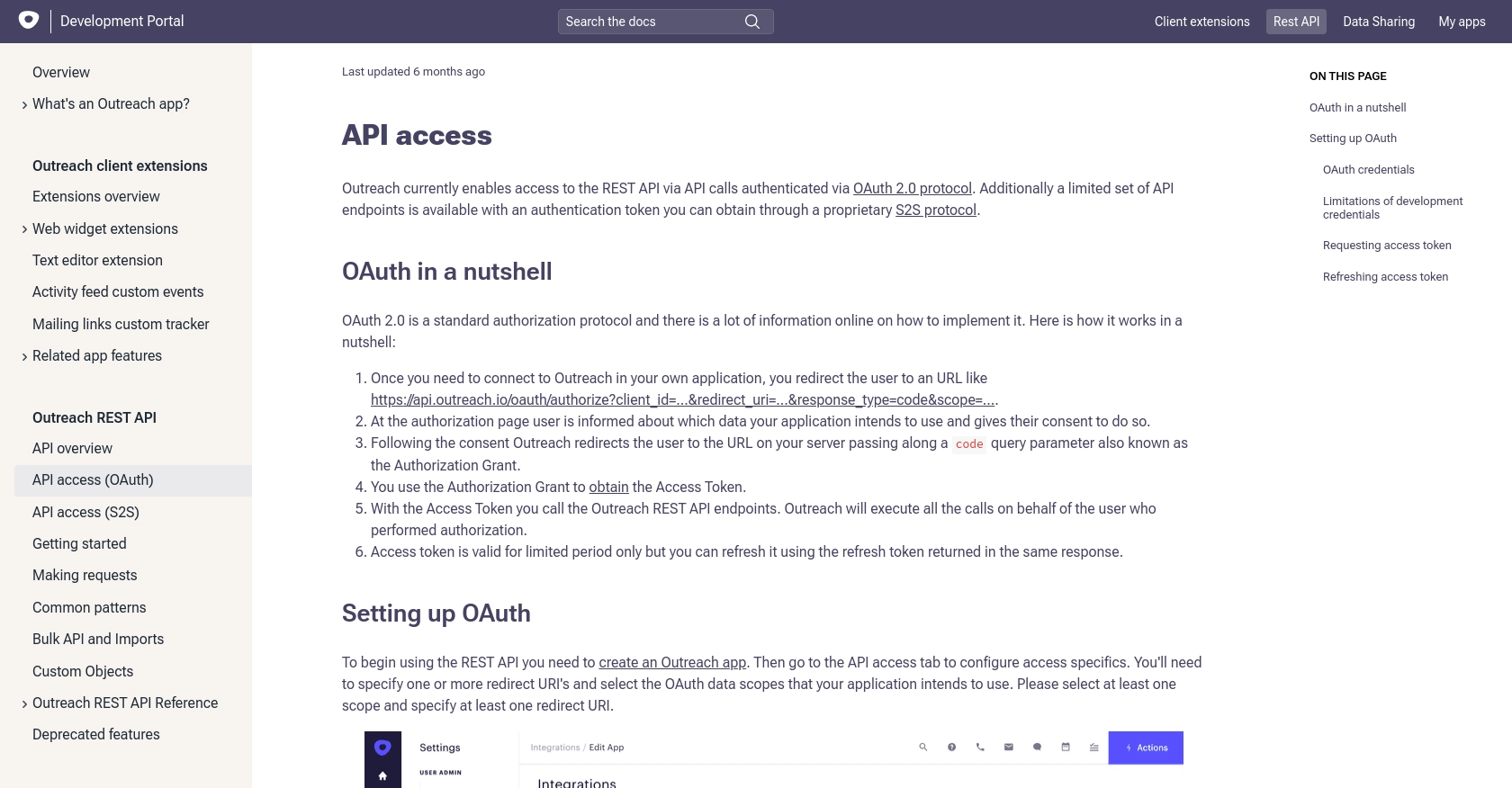
sbb-itb-96038d7
Making API Calls to Retrieve Accounts Using Outreach API in PHP
Now that you have set up your Outreach app and obtained the necessary OAuth tokens, it's time to make API calls to retrieve account data. This section will guide you through the process of using PHP to interact with the Outreach API and fetch account information.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or later and the cURL
extension enabled. You can verify this by running the following command:
php -v
To install the cURL
extension, use:
sudo apt-get install php-curl
Installing Required PHP Dependencies
For making HTTP requests, we'll use the GuzzleHTTP
library. Install it via Composer:
composer require guzzlehttp/guzzle
Fetching Accounts from Outreach API
Create a PHP script named get_outreach_accounts.php
and add the following code to retrieve accounts:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$response = $client->request('GET', 'https://api.outreach.io/api/v2/accounts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/vnd.api+json'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['data'] as $account) {
echo 'Account Name: ' . $account['attributes']['name'] . "\n";
echo 'Industry: ' . $account['attributes']['industry'] . "\n";
echo 'Website: ' . $account['attributes']['websiteUrl'] . "\n\n";
}
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth process.
Running the PHP Script
Execute the script from the command line:
php get_outreach_accounts.php
You should see a list of accounts with their names, industries, and website URLs.
Handling API Response and Errors
It's crucial to handle potential errors when making API calls. The Outreach API may return various HTTP status codes indicating success or failure. Here's how you can manage these responses:
try {
$response = $client->request('GET', 'https://api.outreach.io/api/v2/accounts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/vnd.api+json'
]
]);
$data = json_decode($response->getBody(), true);
// Process data...
} catch (\GuzzleHttp\Exception\ClientException $e) {
echo 'Request failed: ' . $e->getMessage();
}
Refer to the Outreach API documentation for more details on error codes and handling.
Verifying API Call Success
After running the script, verify the retrieved data by checking the accounts in your Outreach sandbox environment. Ensure the data matches the expected output.
By following these steps, you can efficiently retrieve account data from Outreach using PHP, enabling seamless integration with your applications.
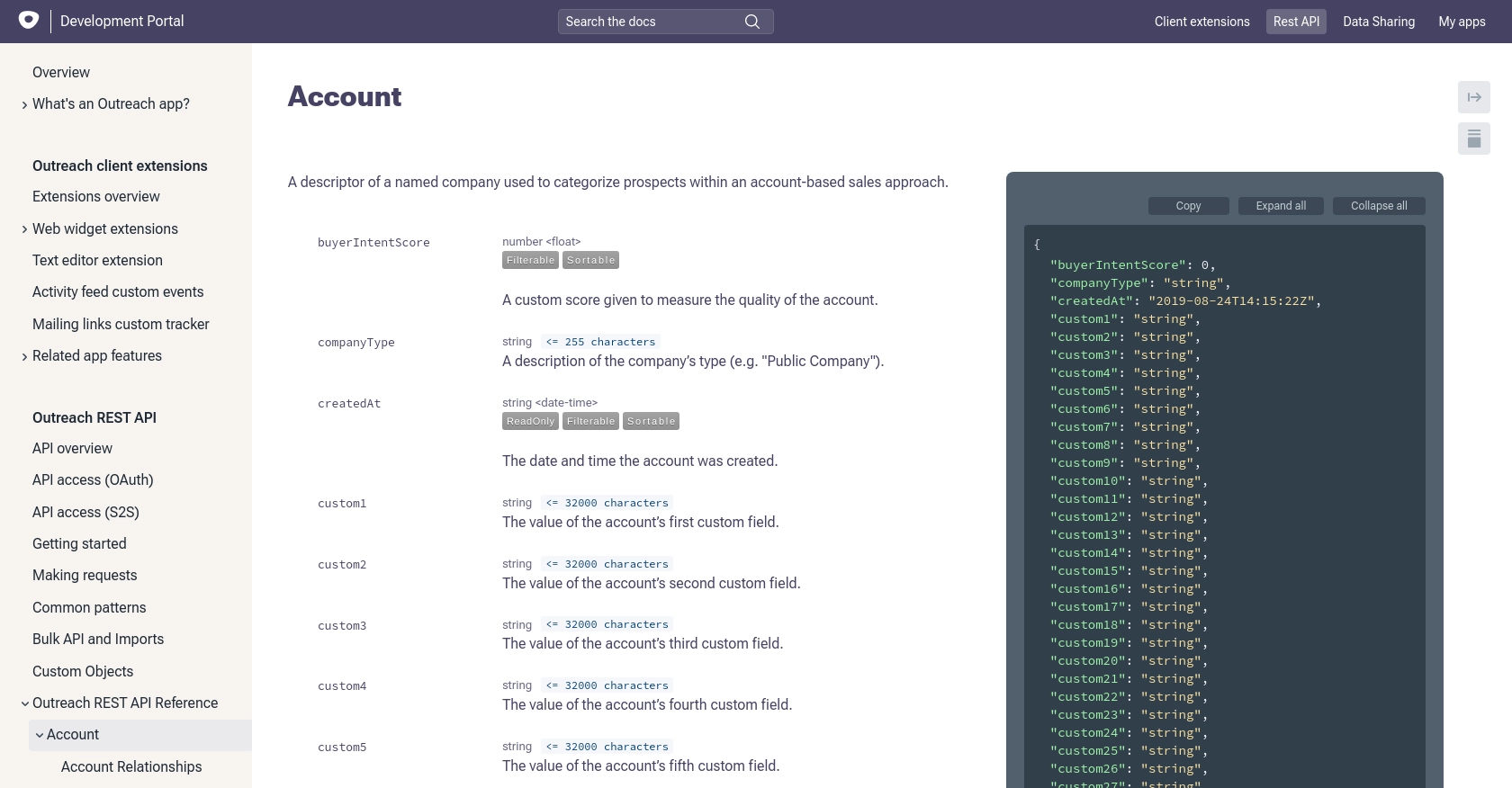
Best Practices for Using Outreach API in PHP
When integrating with the Outreach API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth tokens and client secrets securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: The Outreach API has a rate limit of 10,000 requests per hour. Monitor the
X-RateLimit-Remaining
header in API responses to avoid hitting this limit. Implement exponential backoff strategies to handle 429 errors gracefully. - Refresh Tokens Appropriately: Access tokens are short-lived. Use the refresh token to obtain new access tokens before they expire, ensuring uninterrupted API access.
- Standardize Data Fields: Transform and standardize data fields when synchronizing with other systems to maintain consistency across platforms.
Leveraging Endgrate for Seamless API Integrations
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Outreach. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of API integrations.
- Build Once, Use Everywhere: Develop a single integration that works across multiple platforms, reducing redundancy and effort.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience with minimal setup.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Conclusion on Outreach API Integration with PHP
Integrating with the Outreach API using PHP allows developers to access and manage account data efficiently, enhancing sales operations and ensuring data consistency across platforms. By following best practices and leveraging tools like Endgrate, you can create robust and scalable integrations that meet your business needs.
Start your integration journey today and unlock the full potential of the Outreach platform for your sales teams.
Read More
Ready to get started?