Using the Nutshell API to Create or Update Contacts in Python
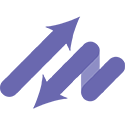
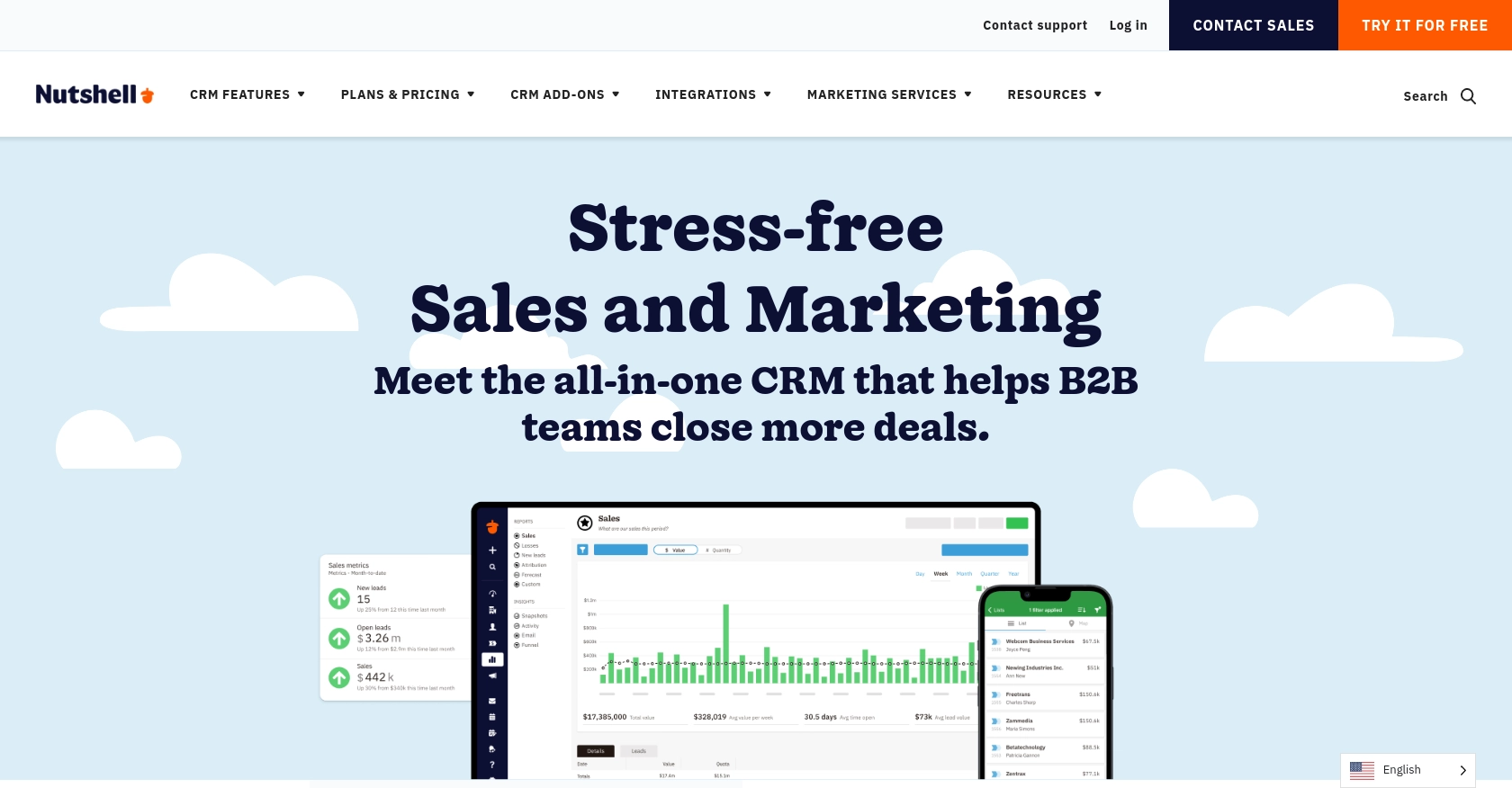
Introduction to Nutshell CRM
Nutshell is a powerful CRM platform designed to help businesses manage their sales processes efficiently. It offers a comprehensive suite of tools for tracking leads, managing contacts, and analyzing sales data, making it an ideal choice for businesses looking to streamline their customer relationship management.
Developers might want to integrate with Nutshell's API to automate and enhance their CRM workflows. For example, using the Nutshell API, a developer can create or update contact information directly from an external application, ensuring that the CRM data remains accurate and up-to-date.
This article will guide you through using Python to interact with the Nutshell API, focusing on creating or updating contacts. By following this tutorial, you'll learn how to efficiently manage contact data within the Nutshell platform using Python.
Setting Up Your Nutshell Test Account and API Access
Before you can start integrating with the Nutshell API, you'll need to set up a test account and obtain the necessary credentials for authentication. This section will guide you through the process of creating a Nutshell account and generating an API key for secure access.
Creating a Nutshell Account
If you don't already have a Nutshell account, you can sign up for a free trial on the Nutshell website. This will give you access to the platform's features and allow you to test API interactions without any initial cost.
- Visit the Nutshell website and click on the "Sign Up" button.
- Fill out the registration form with your details and submit it to create your account.
- Once your account is set up, log in to access the Nutshell dashboard.
Generating an API Key for Nutshell
To interact with the Nutshell API, you'll need an API key, which acts as a password for authenticating your requests. Follow these steps to generate your API key:
- Log in to your Nutshell account and navigate to the "Setup" tab.
- Click on "API" to access the API settings page.
- Select "Create New API Key" and provide a name for your key to identify it later.
- Set the permissions for the key, ensuring it has access to the contacts you wish to manage.
- Save the API key and keep it secure, as it will be used in your API requests.
Understanding Nutshell API Authentication
The Nutshell API uses HTTP Basic authentication, requiring both a username and an API key. The username can be your company's domain or a specific user's email address, while the API key serves as the password. Ensure that your application securely stores these credentials to prevent unauthorized access.
Here's an example of how to structure your authentication header:
import requests
# Set up authentication credentials
username = 'your_email_or_domain'
api_key = 'your_api_key'
# Example of making an authenticated request
response = requests.get(
'https://app.nutshell.com/api/v1/json',
auth=(username, api_key)
)
With your Nutshell account and API key ready, you're now set to begin integrating with the Nutshell API using Python. In the next section, we'll explore how to create and update contacts through API calls.
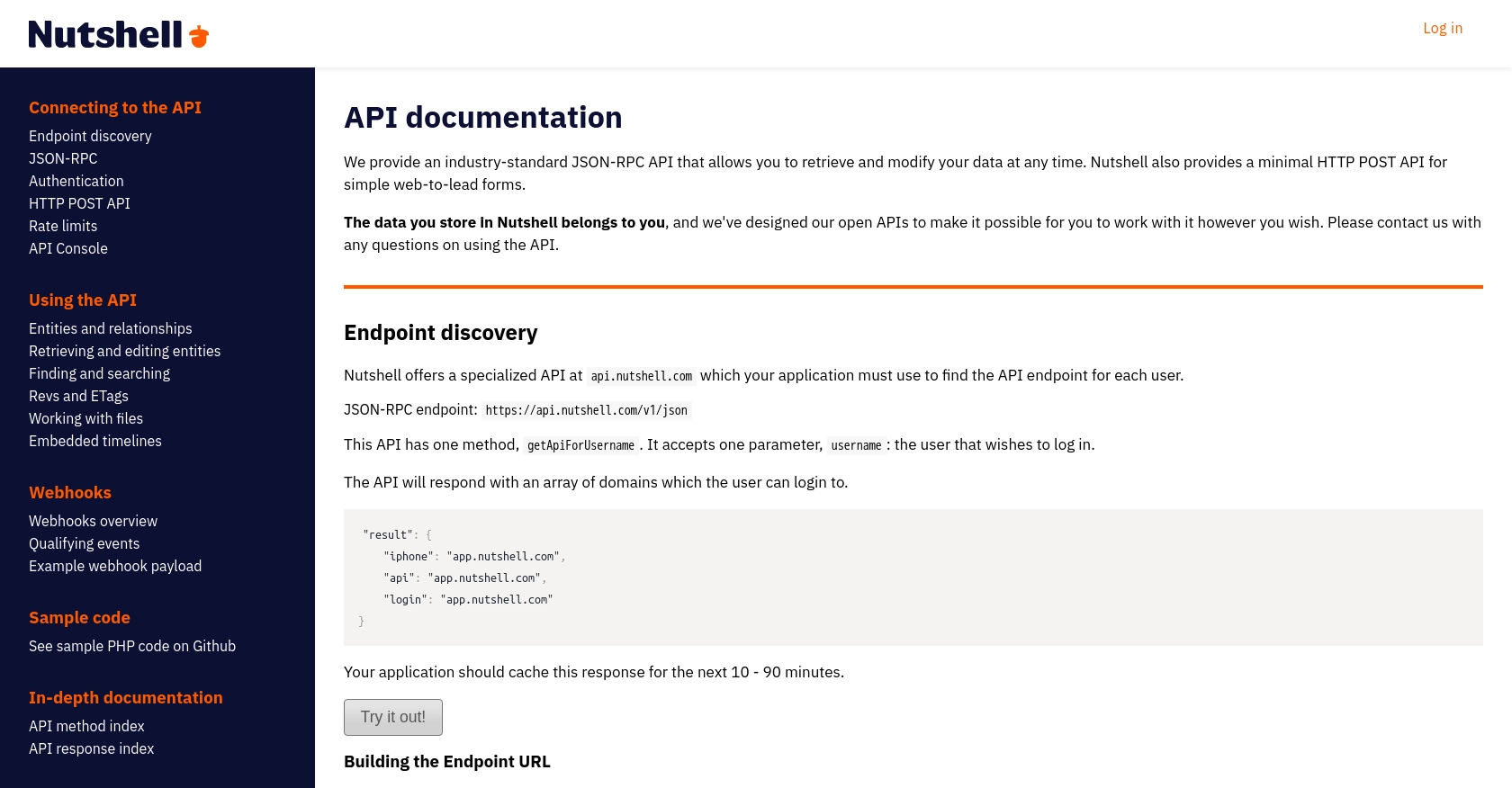
sbb-itb-96038d7
Making API Calls to Create or Update Contacts in Nutshell Using Python
In this section, we'll explore how to make API calls to create or update contacts in Nutshell using Python. We'll cover the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your Python Environment for Nutshell API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Creating a Contact in Nutshell Using Python
To create a contact in Nutshell, you'll need to send a JSON-RPC request to the Nutshell API. Here's a sample code snippet to create a new contact:
import requests
import json
# Set up authentication credentials
username = 'your_email_or_domain'
api_key = 'your_api_key'
# Define the API endpoint
url = 'https://app.nutshell.com/api/v1/json'
# Define the contact data
contact_data = {
"method": "newContact",
"params": {
"contact": {
"name": "John Doe",
"email": ["john.doe@example.com"],
"phone": ["123-456-7890"]
}
},
"id": "1"
}
# Make the API request
response = requests.post(url, auth=(username, api_key), json=contact_data)
# Check the response
if response.status_code == 200:
print("Contact created successfully:", response.json())
else:
print("Failed to create contact:", response.status_code, response.text)
Replace your_email_or_domain
and your_api_key
with your actual Nutshell credentials. The example above creates a contact named John Doe with an email and phone number.
Updating a Contact in Nutshell Using Python
To update an existing contact, use the editContact
method. Here's how you can update a contact's information:
import requests
import json
# Set up authentication credentials
username = 'your_email_or_domain'
api_key = 'your_api_key'
# Define the API endpoint
url = 'https://app.nutshell.com/api/v1/json'
# Define the contact update data
contact_update_data = {
"method": "editContact",
"params": {
"contactId": 42, # Replace with the actual contact ID
"rev": "6", # Replace with the actual revision number
"contact": {
"email": ["new.email@example.com"],
"phone": ["987-654-3210"]
}
},
"id": "2"
}
# Make the API request
response = requests.post(url, auth=(username, api_key), json=contact_update_data)
# Check the response
if response.status_code == 200:
print("Contact updated successfully:", response.json())
else:
print("Failed to update contact:", response.status_code, response.text)
Ensure you replace the contactId
and rev
with the correct values for the contact you wish to update. This example updates the contact's email and phone number.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and errors appropriately. The Nutshell API returns a 200 status code for successful requests. If the request fails, check the status code and response text for details. Common error codes include 401 for unauthorized access, indicating issues with authentication credentials.
For more information on error handling, refer to the Nutshell API documentation.
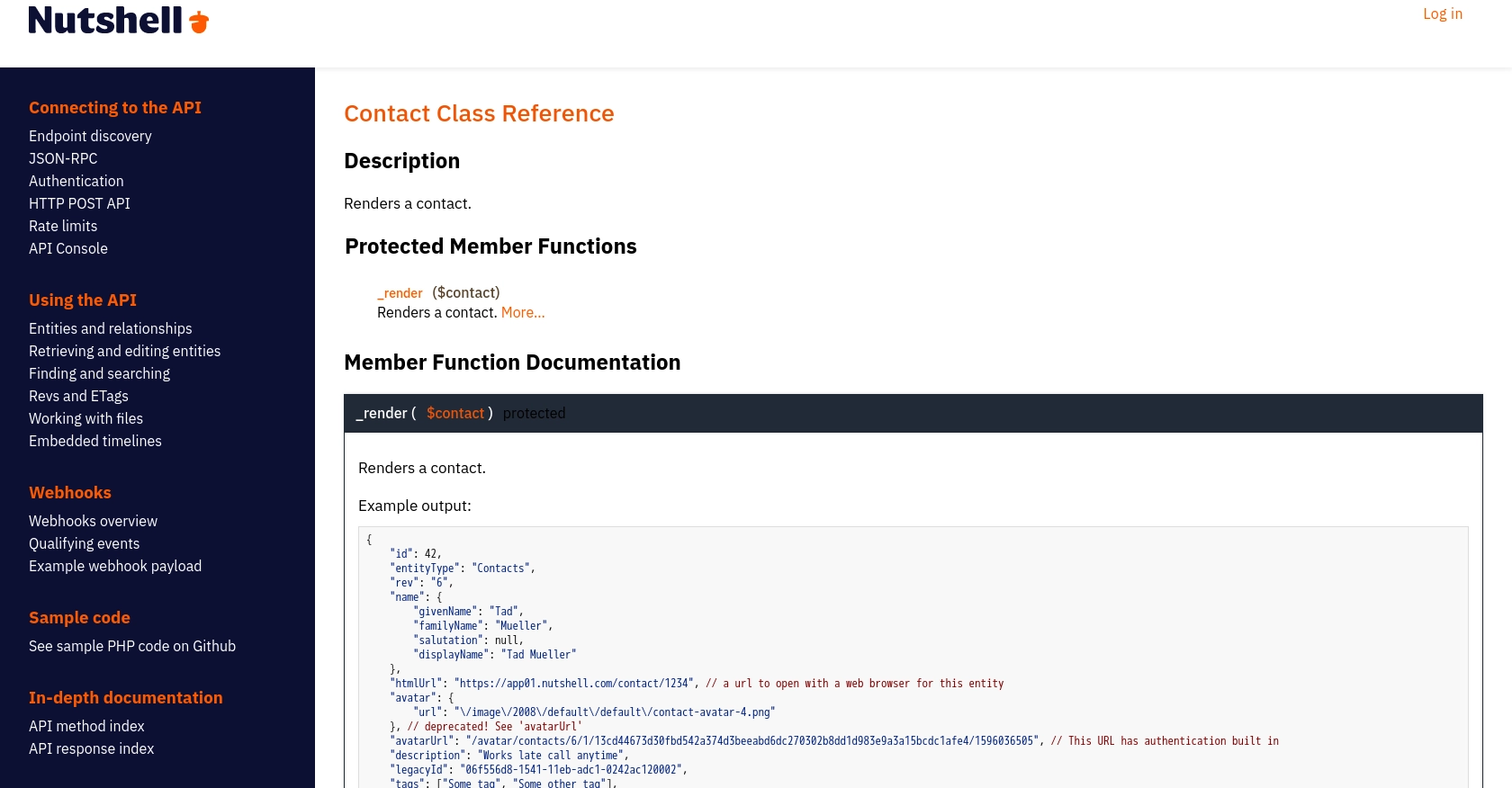
Conclusion and Best Practices for Nutshell API Integration
Integrating with the Nutshell API using Python provides a powerful way to automate and manage your CRM data efficiently. By following the steps outlined in this guide, you can create and update contacts seamlessly, ensuring your CRM remains accurate and up-to-date.
Best Practices for Secure and Efficient Nutshell API Usage
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies for retrying requests when necessary.
- Data Standardization: Ensure that data fields are standardized before sending them to the API. This helps maintain consistency across your CRM data.
- Error Handling: Implement robust error handling to manage API responses effectively. Log errors for troubleshooting and ensure your application can gracefully handle failures.
Streamline Your Integrations with Endgrate
While integrating with the Nutshell API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Nutshell.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?