Using the Sap Business One API to Create or Update Vendors in Javascript
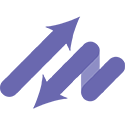
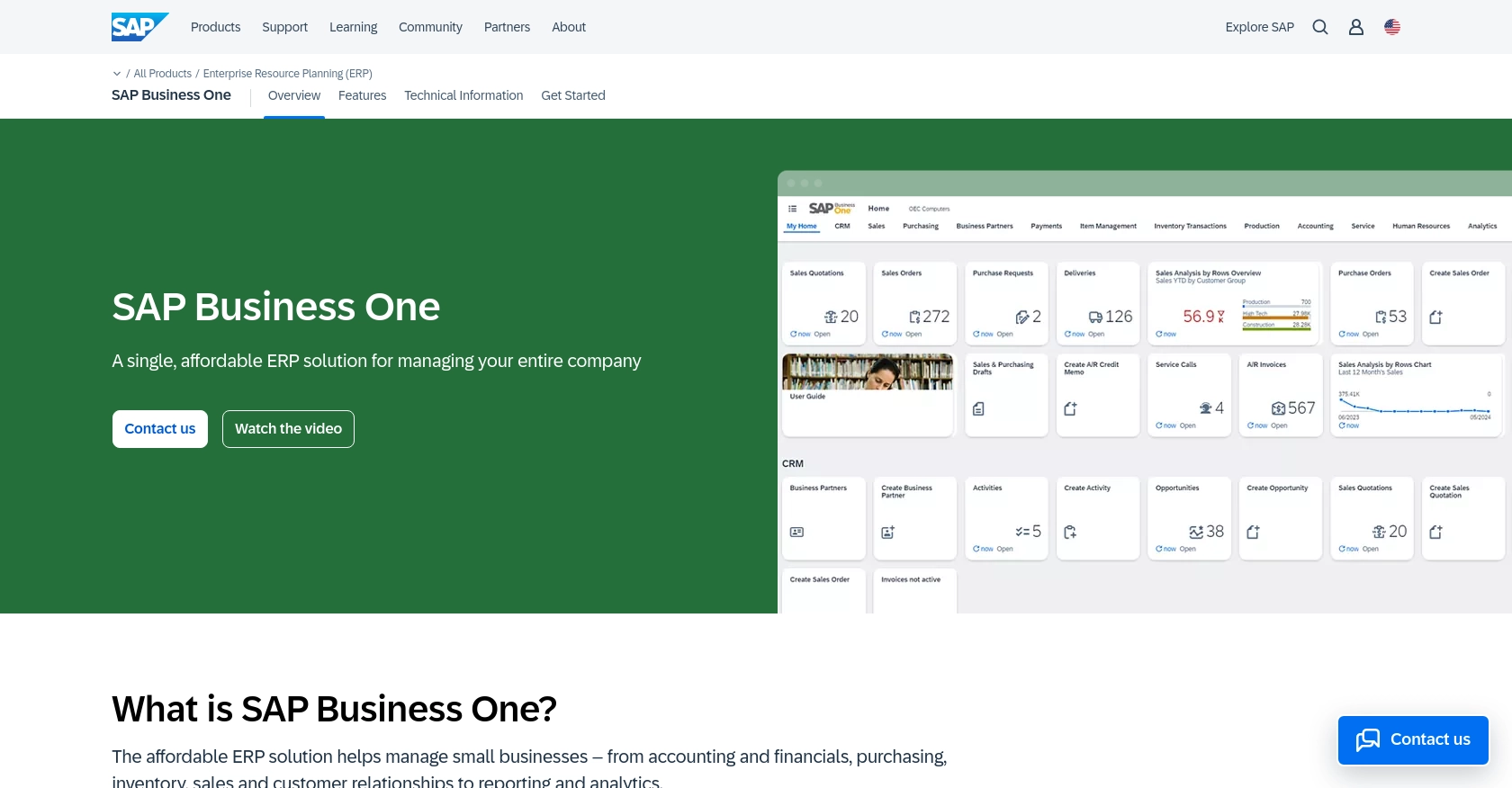
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and supply chain management. Its robust API capabilities allow developers to integrate and automate various business processes seamlessly.
Connecting with the SAP Business One API enables developers to efficiently manage vendor data, such as creating or updating vendor information. For example, a developer might automate the process of updating vendor details from an external supplier database, ensuring that the ERP system remains up-to-date with minimal manual intervention.
Setting Up a Test or Sandbox Account for SAP Business One API
To begin working with the SAP Business One API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Creating a SAP Business One Sandbox Account
If you don't already have access to a SAP Business One sandbox, you can request one through SAP's official channels. This typically involves contacting your SAP representative or using the SAP Partner Portal to gain access to a trial or demo environment.
Configuring OAuth-Based Authentication for SAP Business One API
SAP Business One uses a custom authentication method. To authenticate your API requests, you need to create an app within your sandbox account. Follow these steps:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer section, which is where API interactions are managed.
- Create a new application by providing the necessary details such as application name and description.
- Once the application is created, you will receive a Client ID and Client Secret. Keep these credentials secure as they are required for API authentication.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need to generate API keys for specific operations. Here's how you can do it:
- Within your sandbox account, navigate to the API Management section.
- Locate the option to generate a new API key and follow the prompts to create one.
- Store the API key securely, as it will be used in your API requests.
With your sandbox account and authentication credentials set up, you're ready to start making API calls to create or update vendor information using JavaScript.
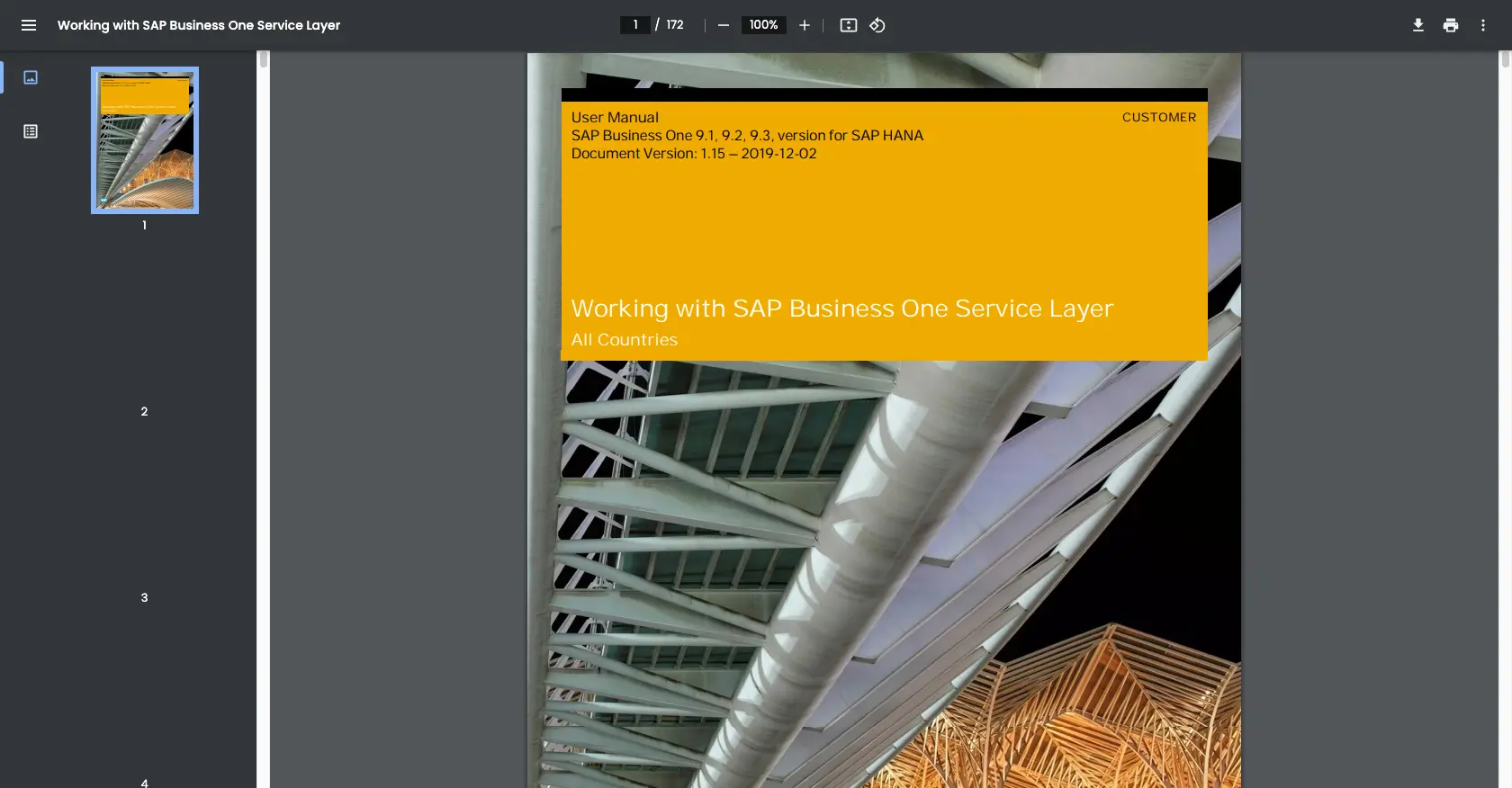
sbb-itb-96038d7
Making API Calls to SAP Business One for Vendor Management Using JavaScript
To interact with the SAP Business One API for creating or updating vendors, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your development environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for SAP Business One API
Before you start coding, ensure you have the following prerequisites installed:
- Node.js (version 14.x or higher)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, you can set up your project by running the following commands in your terminal:
mkdir sap-business-one-api
cd sap-business-one-api
npm init -y
npm install axios
The axios
library will be used to make HTTP requests to the SAP Business One API.
Writing JavaScript Code to Create or Update Vendors in SAP Business One
Now, let's write the JavaScript code to create or update vendor information using the SAP Business One API. Create a file named vendorManagement.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Vendors';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
// Vendor data to be created or updated
const vendorData = {
CardCode: 'V001',
CardName: 'New Vendor',
CardType: 'Supplier'
};
// Function to create or update vendor
async function manageVendor() {
try {
const response = await axios.post(endpoint, vendorData, { headers });
console.log('Vendor created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating vendor:', error.response ? error.response.data : error.message);
}
}
manageVendor();
Replace Your_Access_Token
with the token obtained from your SAP Business One sandbox account. The vendorData
object contains the vendor information you want to create or update.
Executing the JavaScript Code and Verifying the API Call
Run the code using the following command in your terminal:
node vendorManagement.js
If the request is successful, you should see a confirmation message in the console. To verify, check your SAP Business One sandbox account to ensure the vendor information has been updated or created.
Handling Errors and Understanding SAP Business One API Responses
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a try-catch
block to catch and log errors. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 500 Internal Server Error: An error occurred on the server.
Refer to the SAP Business One Service Layer documentation for more detailed information on error codes and handling.
Best Practices for Using the SAP Business One API
When working with the SAP Business One API, it's essential to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store Credentials: Always store your API credentials, such as the Client ID, Client Secret, and API keys, securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and transformed as needed to match the SAP Business One data model. This helps maintain data integrity and consistency.
Streamlining Integrations with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including SAP Business One. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product by outsourcing integrations to Endgrate, reducing development time and costs.
- Build Once, Use Everywhere: Create a single integration for each use case, and apply it across multiple platforms without redundant development efforts.
- Enhance Customer Experience: Offer your customers an intuitive and seamless integration experience with minimal effort.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?