Using the Stamped API to Get Products in Javascript
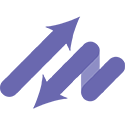
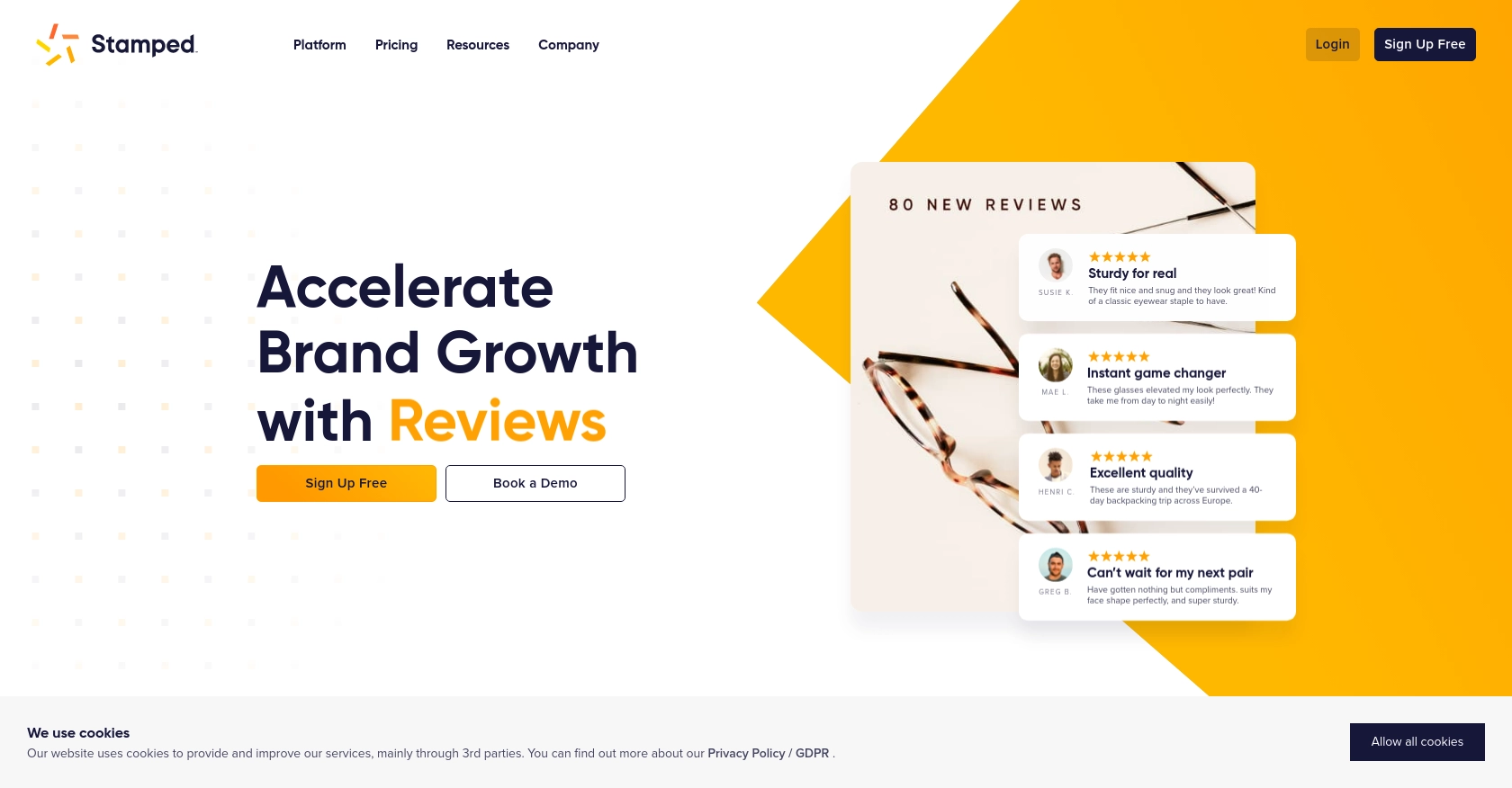
Introduction to Stamped API
Stamped is a powerful platform that enables businesses to enhance their customer engagement through reviews, loyalty programs, and more. With its robust suite of tools, Stamped empowers over 75,000 brands to drive faster, more efficient growth by leveraging customer feedback and loyalty.
For developers, integrating with the Stamped API offers the opportunity to seamlessly connect and manage product data, customer reviews, and loyalty operations. By accessing the Stamped API, developers can automate processes such as retrieving product information, which can be crucial for maintaining up-to-date product catalogs or syncing data across platforms.
In this article, we will explore how to use JavaScript to interact with the Stamped API to get product details. This integration can be particularly useful for developers looking to enhance their e-commerce platforms by displaying real-time product information directly from Stamped.
Setting Up Your Stamped API Test Account
Before you can start interacting with the Stamped API using JavaScript, you'll need to set up a test account. This involves obtaining the necessary API keys and configuring your environment to authenticate requests.
Create a Stamped Account
To access the Stamped API, you must have a Professional or Enterprise plan. If you don't already have an account, visit the Stamped website to sign up for the appropriate plan. Follow the instructions to complete your registration.
Generate API Keys for Authentication
Once your account is set up, you'll need to generate API keys to authenticate your requests:
- Log in to your Stamped account and navigate to the API Keys section in the Control Panel.
- Here, you'll find your public and private API keys. These keys are essential for authenticating your API requests.
- Note down your public API key and private API key, as you'll need them to authenticate your requests using HTTP Basic Auth.
Configure Authentication for API Requests
Stamped API uses HTTP Basic Auth for authentication. Here's how to configure it:
- Use your public API key as the username.
- Use your private API key as the password.
This setup will allow you to authenticate your requests securely when interacting with the Stamped API.
Understanding Store Hash
Your Stamped account may contain multiple stores. Most API endpoints require a {{storeHash}}
variable. You can find this Store Hash in the API Keys section of your dashboard. Ensure you have this information ready, as it will be used in your API calls.
With your Stamped account and API keys ready, you can now proceed to make API calls to retrieve product information using JavaScript.
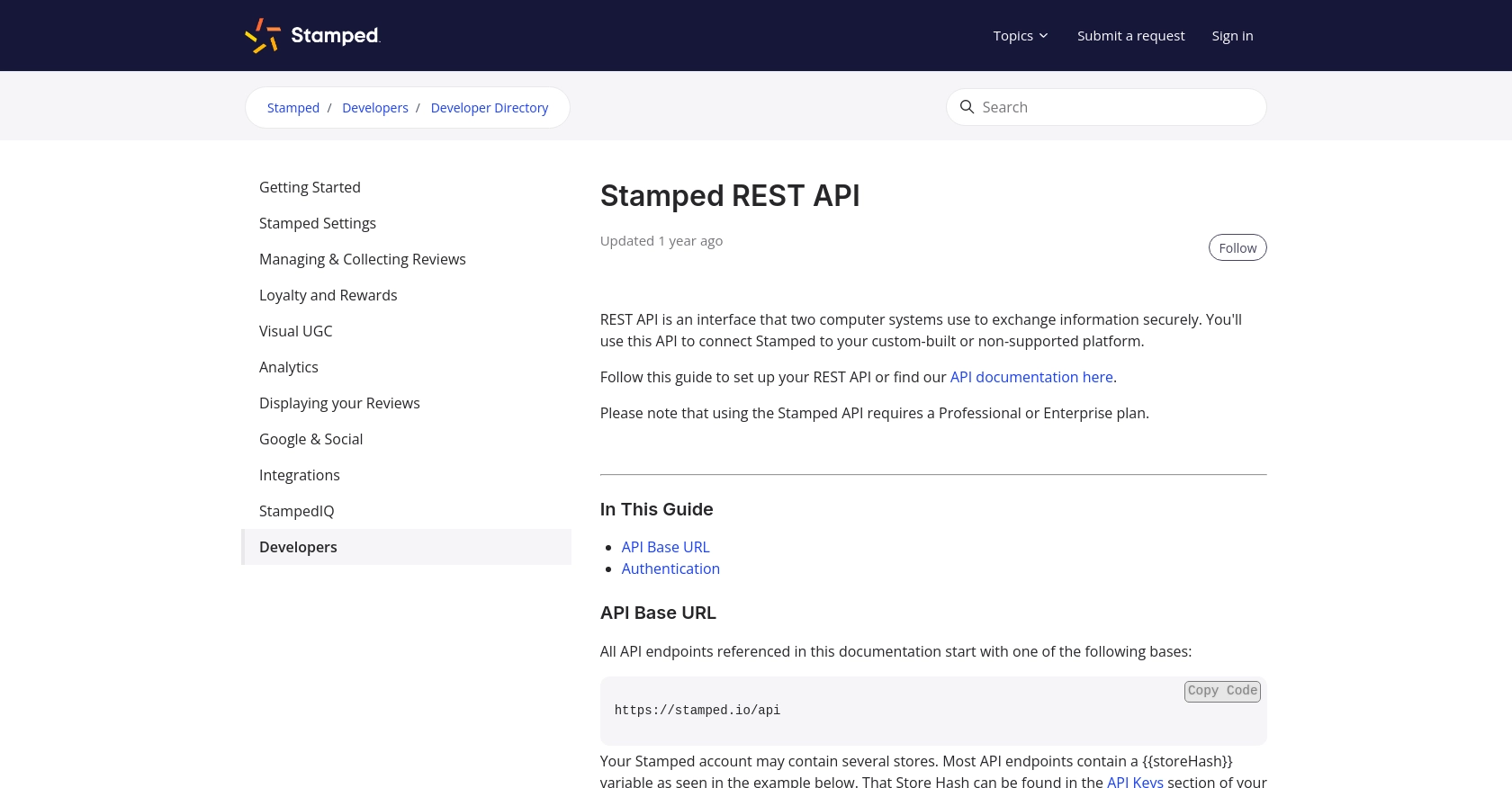
sbb-itb-96038d7
Making API Calls to Retrieve Product Information from Stamped Using JavaScript
To interact with the Stamped API and retrieve product details using JavaScript, you'll need to set up your environment and write the necessary code to make API requests. This section will guide you through the process of making authenticated API calls and handling responses effectively.
Prerequisites for Using JavaScript with Stamped API
Before you begin, ensure you have the following:
- A modern web browser or Node.js environment for running JavaScript code.
- Your public and private API keys from your Stamped account.
- The
{{storeHash}}
for your store, which is required for API endpoints.
Installing Required Dependencies
If you're using Node.js, you may need to install the axios
library to handle HTTP requests. Run the following command to install it:
npm install axios
Writing JavaScript Code to Get Product Details from Stamped API
Below is an example of how to use JavaScript to make a GET request to the Stamped API to retrieve product information:
const axios = require('axios');
// Replace with your actual store hash, product ID, and API keys
const storeHash = 'your_store_hash';
const productId = 'your_product_id';
const publicKey = 'your_public_api_key';
const privateKey = 'your_private_api_key';
// Set the API endpoint
const endpoint = `https://stamped.io/api/v3/merchant/shops/${storeHash}/products/${productId}`;
// Configure the request headers for HTTP Basic Auth
const auth = {
username: publicKey,
password: privateKey
};
// Make the GET request to the Stamped API
axios.get(endpoint, { auth })
.then(response => {
// Handle the response data
console.log('Product Details:', response.data);
})
.catch(error => {
// Handle errors
console.error('Error fetching product details:', error.response ? error.response.data : error.message);
});
Understanding the API Response
Upon a successful request, the API will return product details in JSON format. You can access and manipulate this data as needed for your application. If the request fails, ensure you check the error message for details on what went wrong.
Verifying API Call Success in Stamped Dashboard
To verify that your API call was successful, you can cross-check the product details returned by the API with the information available in your Stamped dashboard. This ensures that the data retrieved is accurate and up-to-date.
Handling Errors and Common Error Codes
When making API calls, it's essential to handle potential errors gracefully. Common HTTP error codes you might encounter include:
- 400 Bad Request: The request was invalid. Check your parameters and try again.
- 401 Unauthorized: Authentication failed. Verify your API keys and credentials.
- 404 Not Found: The specified product or endpoint does not exist. Check your store hash and product ID.
- 500 Internal Server Error: An error occurred on the server. Try the request again later.
By handling these errors, you can ensure a robust integration with the Stamped API.
For more detailed information on the API, refer to the Stamped API documentation.
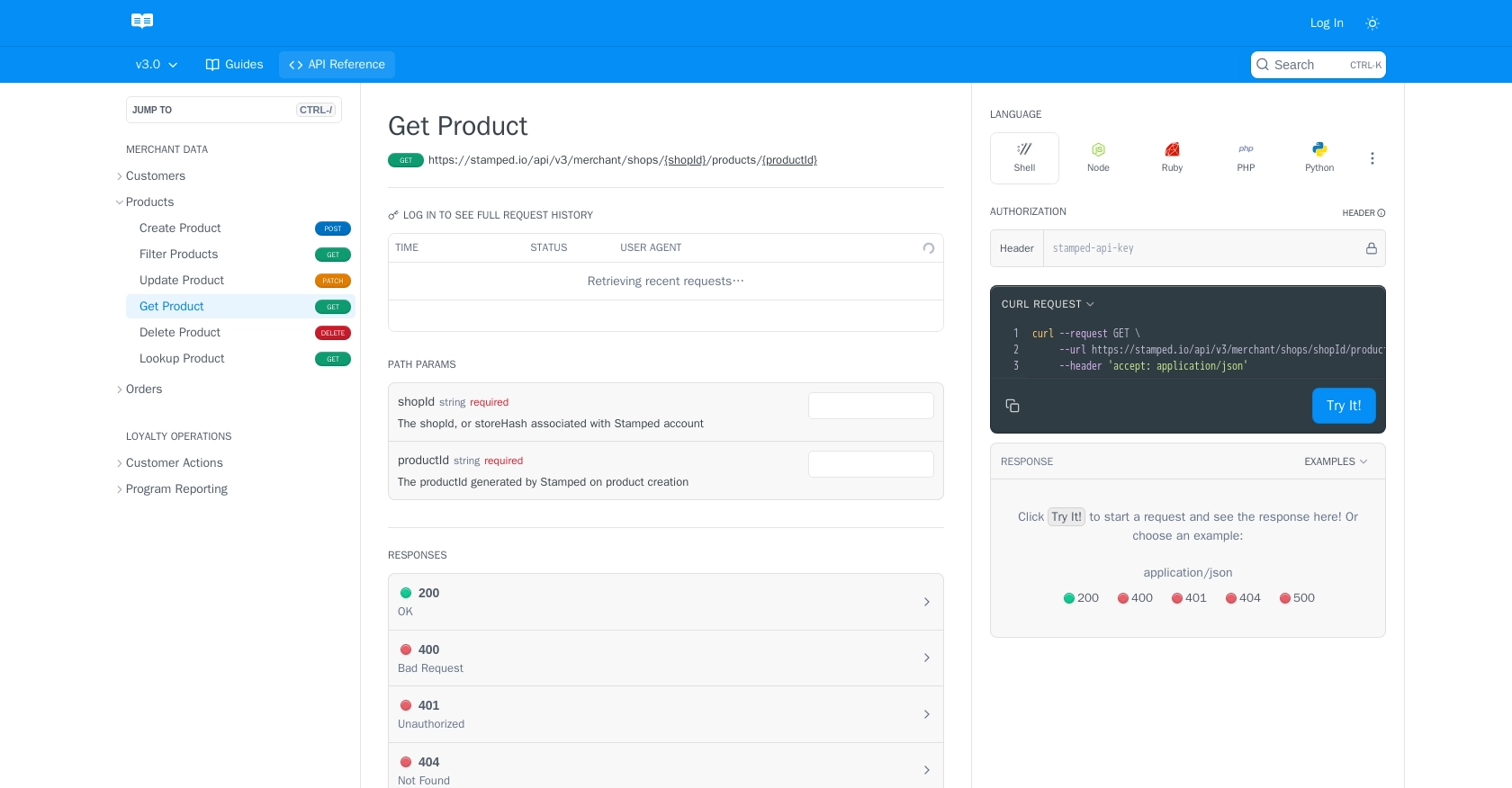
Conclusion and Best Practices for Using Stamped API with JavaScript
Integrating with the Stamped API using JavaScript provides a powerful way to enhance your e-commerce platform by accessing real-time product information. By following the steps outlined in this article, you can efficiently retrieve product details and ensure your application remains up-to-date with the latest data from Stamped.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Credentials: Always store your API keys securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Be mindful of any rate limits imposed by the Stamped API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized to fit your application's requirements. This can help maintain consistency across different data sources.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Stamped can be beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can make a significant difference.
Endgrate offers a unified API endpoint that connects to multiple platforms, including Stamped, allowing you to streamline your integration processes. By using Endgrate, you can focus on your core product development while outsourcing the complexities of managing various integrations.
Explore how Endgrate can simplify your integration needs and provide an intuitive experience for your customers by visiting Endgrate.
Read More
Ready to get started?