Using the Outreach API to Get Users in Javascript
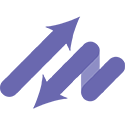
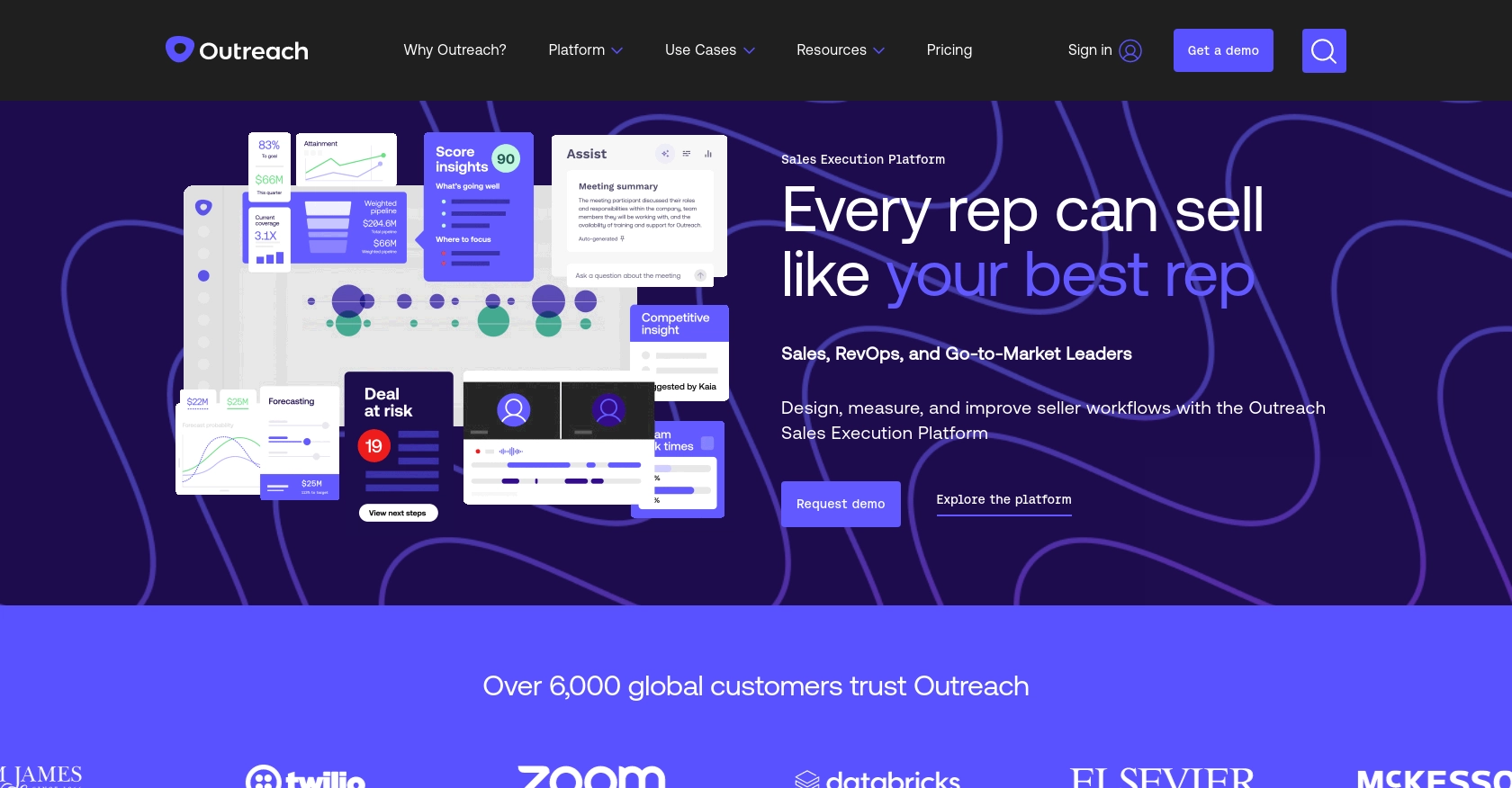
Introduction to Outreach API
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve productivity. With features like email tracking, task automation, and advanced analytics, Outreach is a popular choice for sales teams looking to enhance their outreach efforts.
Integrating with the Outreach API allows developers to access and manage user data, automate workflows, and enhance the functionality of their applications. For example, a developer might use the Outreach API to retrieve user information and integrate it with a CRM system, ensuring that sales teams have up-to-date data at their fingertips.
Setting Up Your Outreach Test/Sandbox Account
Before you can start integrating with the Outreach API, you need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your production data. Outreach provides a development environment specifically for this purpose.
Create an Outreach Developer Account
If you don't have an Outreach account, you can sign up for a developer account on the Outreach website. This account will give you access to the necessary tools and resources to begin your integration.
- Visit the Outreach Developer Portal.
- Follow the instructions to create a new account.
- Once your account is created, log in to access the developer dashboard.
Configure OAuth for Outreach API Access
The Outreach API uses OAuth 2.0 for authentication. You'll need to create an Outreach app to obtain the necessary credentials for API access.
- Navigate to the "My Apps" section in the Outreach Developer Portal.
- Click on "Create a New App" and fill in the required details.
- Specify your redirect URI and select the OAuth scopes your application will need. Make sure to include scopes related to user data access.
- Save your app configuration to generate your client ID and client secret.
Note: Keep your client secret secure, as it will only be displayed once. If needed, you can regenerate it later.
Obtain OAuth Access Token
With your app set up, you can now obtain an OAuth access token to authenticate your API requests.
// Example code to obtain an access token
const axios = require('axios');
async function getAccessToken() {
const response = await axios.post('https://api.outreach.io/oauth/token', {
client_id: 'YOUR_CLIENT_ID',
client_secret: 'YOUR_CLIENT_SECRET',
redirect_uri: 'YOUR_REDIRECT_URI',
grant_type: 'authorization_code',
code: 'AUTHORIZATION_CODE'
});
return response.data.access_token;
}
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_REDIRECT_URI
, and AUTHORIZATION_CODE
with your actual values. This will return an access token that you can use to authenticate your API calls.
For more detailed information on setting up OAuth, refer to the Outreach OAuth Documentation.
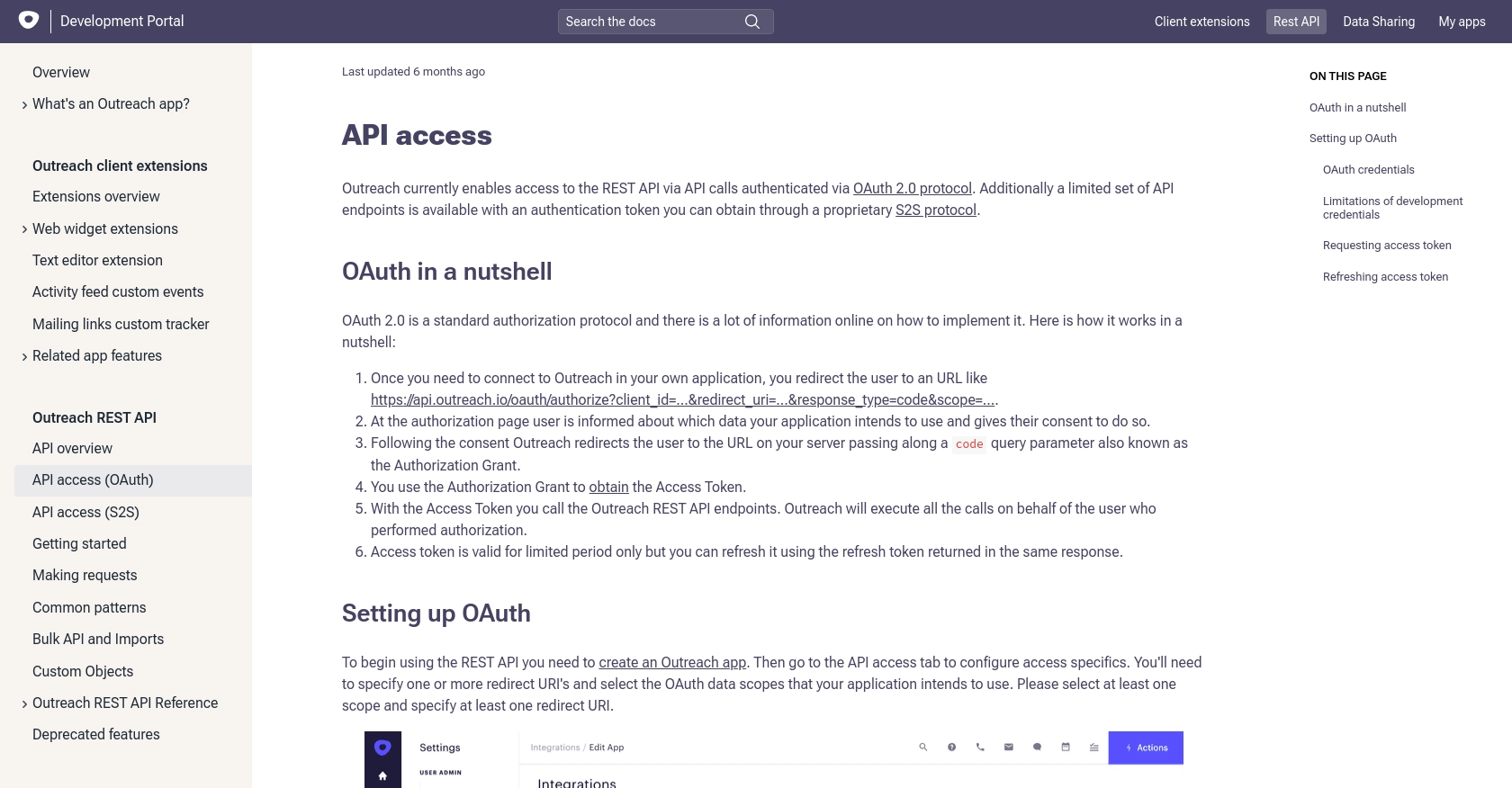
sbb-itb-96038d7
Making API Calls to Retrieve Users with Outreach API in JavaScript
To interact with the Outreach API and retrieve user data, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Outreach API
Before making API calls, ensure you have Node.js installed on your machine. You'll also need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Retrieve Users from Outreach API
Once your environment is set up, you can write the code to fetch user data from the Outreach API. Here's an example of how to do this:
const axios = require('axios');
async function getUsers() {
try {
const accessToken = 'YOUR_ACCESS_TOKEN'; // Replace with your actual access token
const response = await axios.get('https://api.outreach.io/api/v2/users', {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/vnd.api+json'
}
});
console.log(response.data);
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
getUsers();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth process. This code will make a GET request to the Outreach API to retrieve user data and log it to the console.
Verifying Successful API Requests and Handling Errors
After running the code, verify the output by checking the console for user data. If the request is successful, you should see a list of users. If there's an error, the catch block will log the error details.
Common error codes include:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 403 Forbidden: Ensure your OAuth scopes include the necessary permissions.
- 429 Too Many Requests: The Outreach API has a rate limit of 10,000 requests per hour. If exceeded, wait for the reset time indicated in the response headers.
For more details on error handling, refer to the Outreach API Documentation.
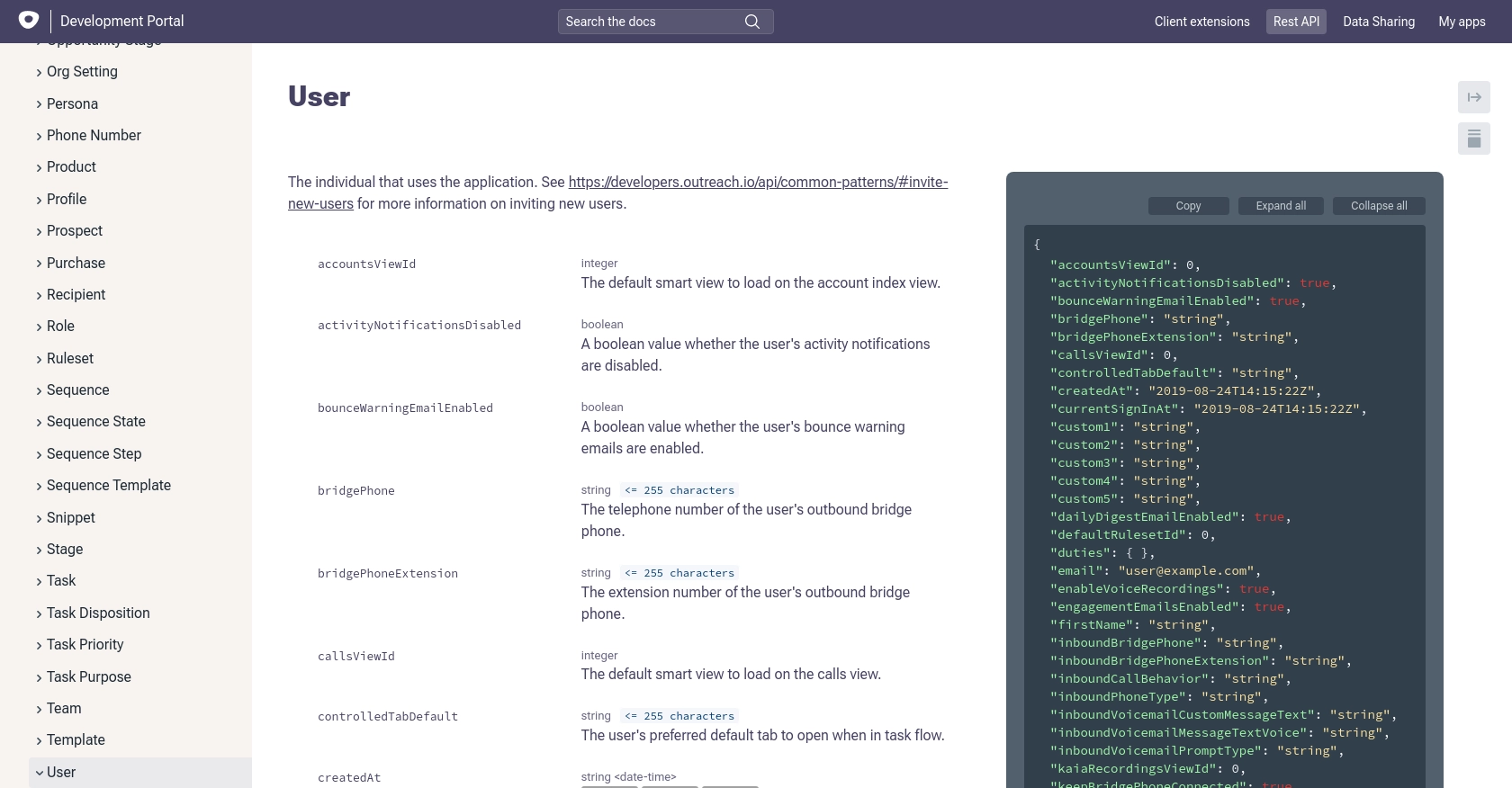
Best Practices for Using the Outreach API in JavaScript
When working with the Outreach API, it's essential to follow best practices to ensure secure and efficient integration. Here are some key recommendations:
- Secure Storage of Credentials: Store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Efficient Token Management: Keep track of token expiration times and use refresh tokens to obtain new access tokens when needed. This ensures uninterrupted API access.
- Handling Rate Limits: Be mindful of the Outreach API's rate limit of 10,000 requests per hour. Implement logic to handle 429 errors and respect the reset time provided in the response headers.
- Data Transformation and Standardization: Transform and standardize data fields as needed to ensure compatibility with your application or other integrated systems.
Leveraging Endgrate for Seamless Outreach API Integrations
Integrating multiple APIs can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Outreach. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?