How to Get Sales Orders with the Quickbooks API in Python
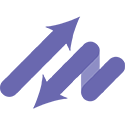
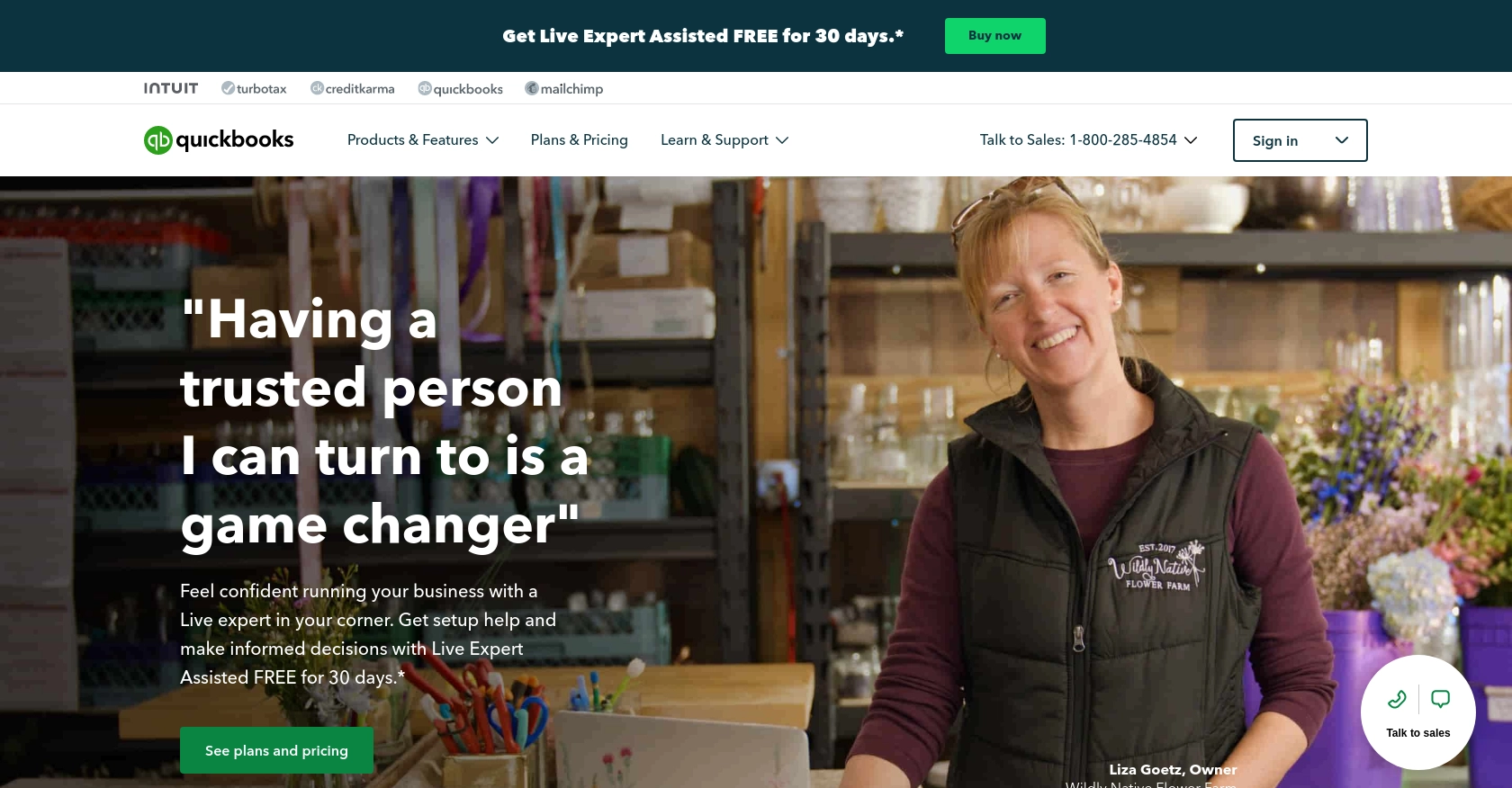
Introduction to QuickBooks API for Sales Orders
QuickBooks is a widely-used accounting software that offers a robust suite of tools for managing financial data, invoicing, payroll, and more. It is particularly popular among small to medium-sized businesses due to its user-friendly interface and comprehensive features.
For developers, integrating with the QuickBooks API can streamline financial operations by automating tasks such as retrieving sales orders. By accessing sales order data programmatically, businesses can enhance their order management processes, improve accuracy, and save time. For example, a developer might use the QuickBooks API to automatically pull sales order information into a custom dashboard, providing real-time insights into sales performance.
Setting Up Your QuickBooks Sandbox Account for API Access
Before you can start interacting with the QuickBooks API to retrieve sales orders, you need to set up a QuickBooks sandbox account. This environment allows you to test your API calls without affecting any real data, providing a safe space for development and experimentation.
Step-by-Step Guide to Creating a QuickBooks Sandbox Account
-
Sign Up for a QuickBooks Developer Account:
Visit the Intuit Developer Portal and sign up for a developer account. If you already have an account, simply log in.
-
Create a New App:
Once logged in, navigate to the "My Apps" section and click on "Create an App." Choose "QuickBooks Online and Payments" as your platform.
-
Configure Your App:
Fill in the necessary details for your app, such as the app name and description. This information helps you identify your app within the developer portal.
-
Generate OAuth Credentials:
After creating your app, go to the "Keys & OAuth" section. Here, you'll find your Client ID and Client Secret. These credentials are essential for authenticating your API requests.
-
Set Up Redirect URIs:
In the same "Keys & OAuth" section, specify your redirect URIs. These are the endpoints where QuickBooks will send users after they authorize your app.
Authenticating with OAuth 2.0 in QuickBooks
The QuickBooks API uses OAuth 2.0 for authentication, ensuring secure access to your data. Follow these steps to authenticate:
-
Obtain an Authorization Code:
Direct users to QuickBooks' authorization URL, where they can log in and grant your app access. Upon approval, QuickBooks will redirect them to your specified URI with an authorization code.
-
Exchange the Authorization Code for Tokens:
Use the authorization code to request an access token and a refresh token from QuickBooks. These tokens allow your app to make API calls on behalf of the user.
For more detailed information on setting up OAuth, refer to the Intuit Developer Documentation.
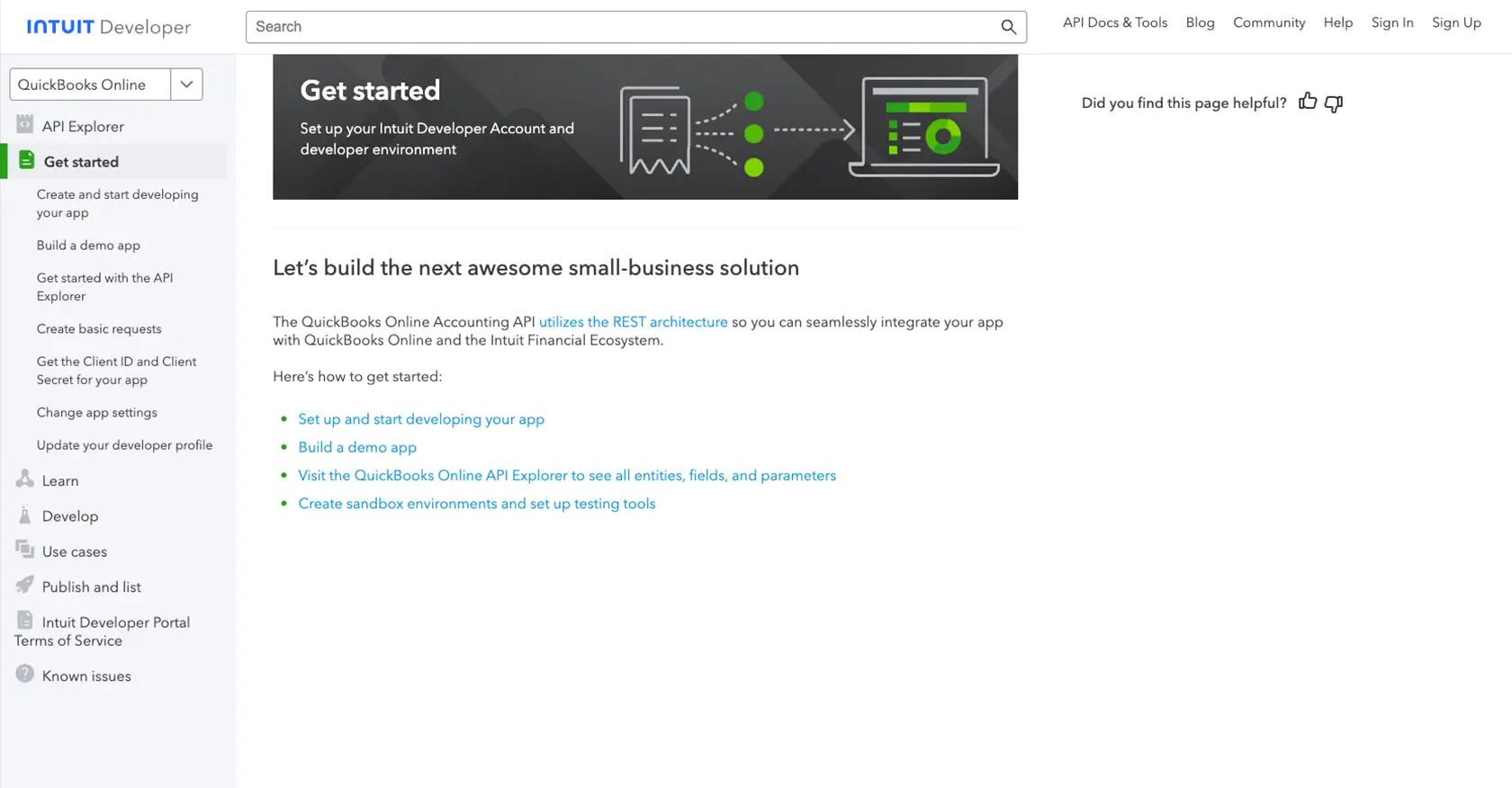
sbb-itb-96038d7
Making API Calls to Retrieve Sales Orders from QuickBooks Using Python
To interact with the QuickBooks API and retrieve sales orders, you'll need to set up your Python environment and write code to make the necessary API calls. This section will guide you through the process, ensuring you have the right tools and knowledge to successfully access sales order data.
Setting Up Your Python Environment for QuickBooks API Integration
Before you begin, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command line and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Retrieve Sales Orders from QuickBooks
With your environment set up, you can now write the Python code to interact with the QuickBooks API. Create a file named get_sales_orders.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/salesorder"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the QuickBooks API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
sales_orders = response.json()
for order in sales_orders.get("SalesOrder", []):
print(order)
else:
print(f"Failed to retrieve sales orders: {response.status_code} - {response.text}")
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token obtained during the OAuth authentication process.
Verifying Successful API Calls and Handling Errors
After running the script, you should see the sales orders printed in your terminal if the request is successful. You can verify the retrieved data by comparing it with the sales orders in your QuickBooks sandbox account.
If the request fails, the script will output an error message with the status code and response text. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 403 Forbidden: Verify that your app has the necessary permissions to access sales orders.
- 404 Not Found: Ensure the endpoint URL is correct and the resource exists.
For more detailed error handling, refer to the Intuit Developer Documentation.
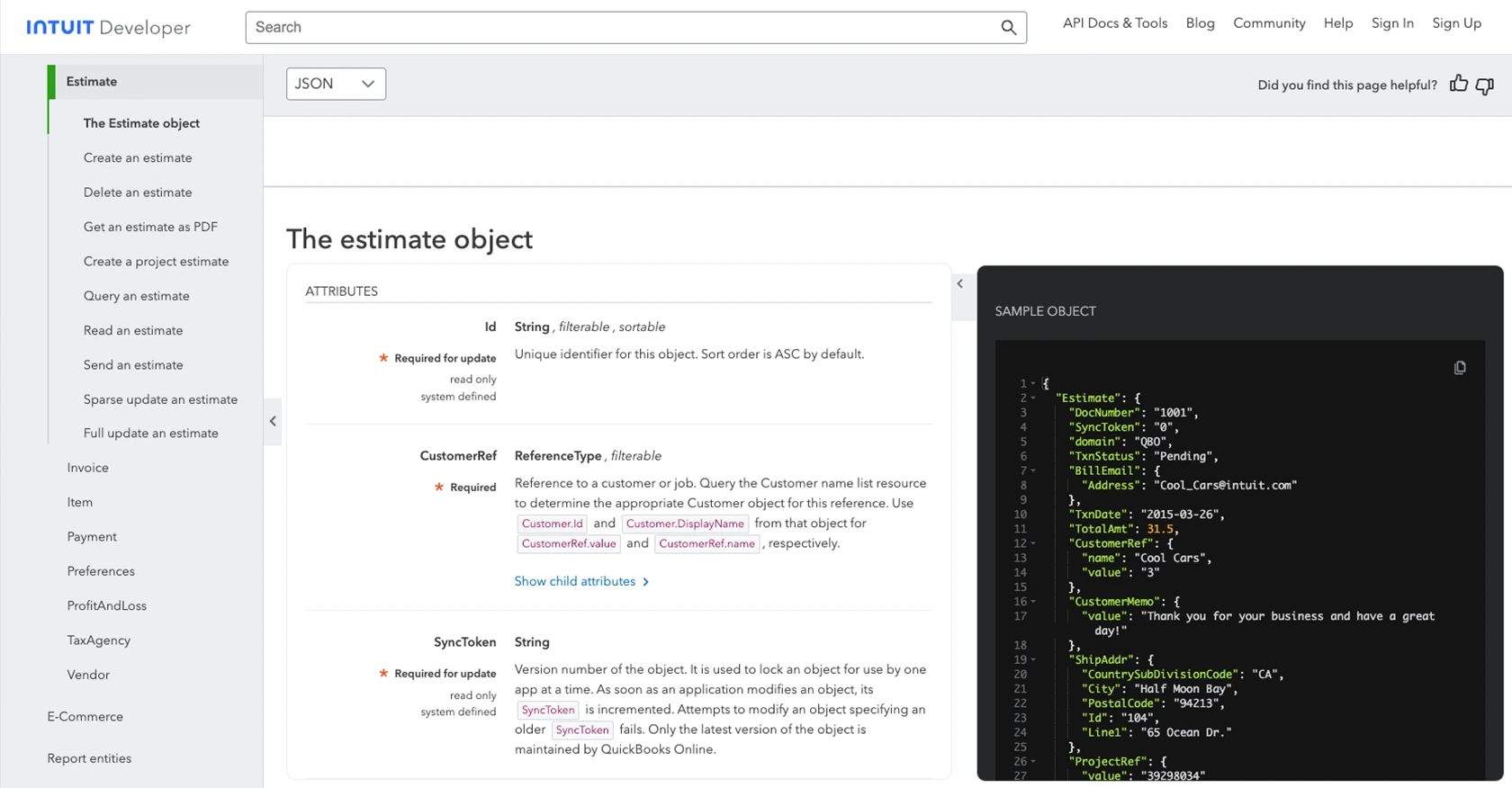
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to retrieve sales orders using Python can significantly enhance your business's financial operations. By automating the retrieval of sales order data, you can streamline processes, reduce manual errors, and gain real-time insights into your sales performance.
Best Practices for Secure and Efficient API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as the client ID, client secret, and access tokens, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: QuickBooks API imposes rate limits to ensure fair usage. Be mindful of these limits and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: When retrieving sales orders, ensure that the data fields are standardized to match your internal systems. This will facilitate seamless integration and data consistency.
Streamline Your Integrations with Endgrate
While building custom integrations with QuickBooks can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including QuickBooks. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can help you streamline your integrations and enhance your product offerings by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/estimate
Ready to get started?