Using the Sap Business One API to Create or Update Purchase Orders (with Python examples)
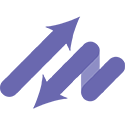
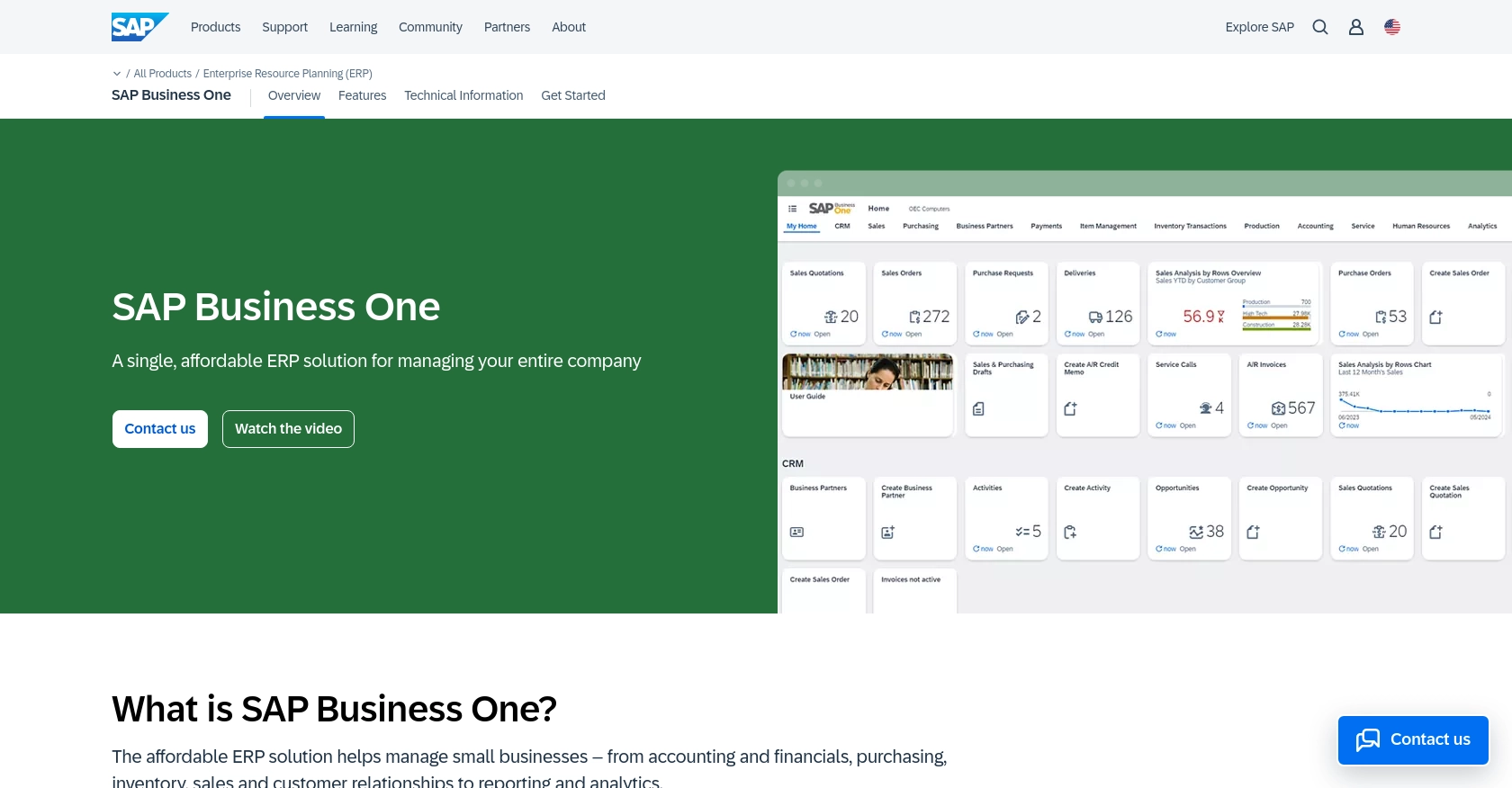
Introduction to SAP Business One API for Purchase Orders
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers a suite of tools to manage various business functions, including finance, sales, and inventory, all within a single platform. By leveraging the SAP Business One API, developers can seamlessly integrate external applications to automate and enhance business processes.
Connecting with the SAP Business One API allows developers to create or update purchase orders programmatically, streamlining procurement workflows. For example, a developer might automate the creation of purchase orders based on inventory levels, ensuring timely restocking and reducing manual data entry.
Setting Up a Test or Sandbox Account for SAP Business One API
Before you can start integrating with the SAP Business One API, it's essential to set up a test or sandbox environment. This allows you to safely experiment with API calls without affecting your live data. SAP Business One provides a comprehensive sandbox environment for developers to test their integrations.
Creating a SAP Business One Sandbox Account
To begin, you need to create a sandbox account with SAP Business One. Follow these steps to set up your account:
- Visit the SAP Business One Service Layer documentation to understand the requirements and capabilities of the sandbox environment.
- Contact your SAP Business One representative or partner to request access to a sandbox environment. They will provide you with the necessary credentials and access details.
- Once you have access, log in to the SAP Business One sandbox environment using the credentials provided.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method to secure API requests. Follow these steps to configure authentication:
- Navigate to the administration panel within your SAP Business One sandbox account.
- Create a new application by providing the necessary details such as application name, description, and redirect URI.
- Generate the client ID and client secret for your application. These credentials will be used to authenticate API requests.
- Ensure that your application has the necessary permissions to create and update purchase orders. This typically involves setting the appropriate scopes within the application settings.
Obtaining API Keys for SAP Business One
In addition to OAuth credentials, you may need API keys for certain operations. Here's how to obtain them:
- Within your SAP Business One sandbox account, navigate to the API management section.
- Generate a new API key, ensuring it has the required permissions for purchase order operations.
- Store the API key securely, as it will be needed for making authenticated API calls.
With your sandbox account and authentication configured, you're ready to start making API calls to create or update purchase orders using the SAP Business One API.
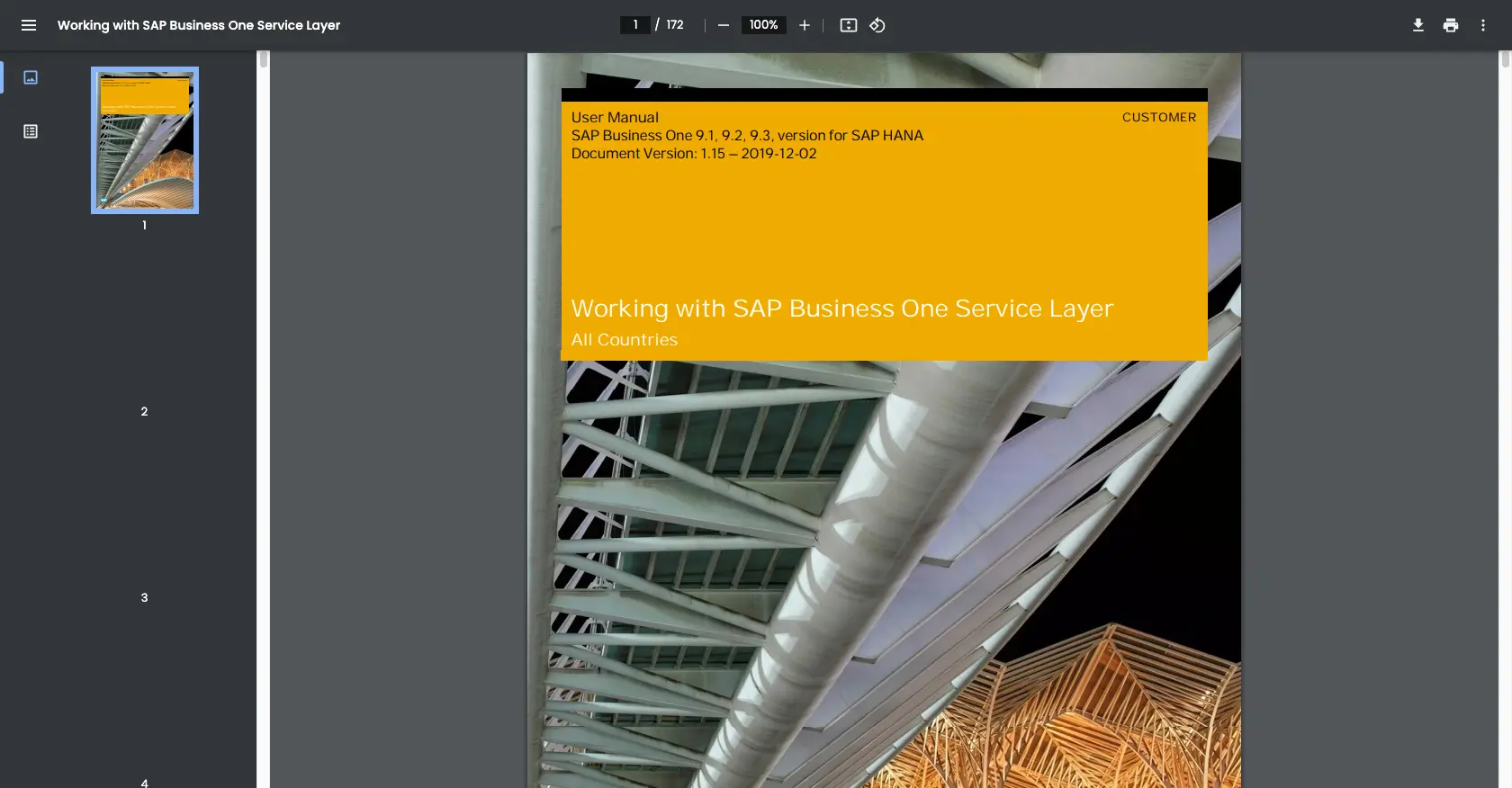
sbb-itb-96038d7
Making API Calls to Create or Update Purchase Orders with SAP Business One API Using Python
To interact with the SAP Business One API for creating or updating purchase orders, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for SAP Business One API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Install the requests
library, which is essential for making HTTP requests:
pip install requests
Creating a Purchase Order with SAP Business One API Using Python
To create a purchase order, you'll need to make a POST request to the SAP Business One API. Here's a sample code snippet:
import requests
import json
# Set the API endpoint
url = "https://your-sap-business-one-instance.com/b1s/v1/PurchaseOrders"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the purchase order data
purchase_order = {
"CardCode": "C20000",
"DocDate": "2023-10-01",
"DocumentLines": [
{
"ItemCode": "A00001",
"Quantity": 10,
"Price": 100
}
]
}
# Send the POST request
response = requests.post(url, headers=headers, data=json.dumps(purchase_order))
# Check the response
if response.status_code == 201:
print("Purchase Order Created Successfully")
else:
print("Failed to Create Purchase Order:", response.json())
Replace Your_Access_Token
with the token obtained during authentication. This code sends a POST request to create a purchase order with specified details. Ensure the data structure matches the API's requirements.
Updating a Purchase Order with SAP Business One API Using Python
To update an existing purchase order, use a PATCH request. Here's an example:
# Set the API endpoint for the specific purchase order
url = "https://your-sap-business-one-instance.com/b1s/v1/PurchaseOrders(123)"
# Define the updated data
updated_data = {
"DocumentLines": [
{
"ItemCode": "A00001",
"Quantity": 15 # Updated quantity
}
]
}
# Send the PATCH request
response = requests.patch(url, headers=headers, data=json.dumps(updated_data))
# Check the response
if response.status_code == 204:
print("Purchase Order Updated Successfully")
else:
print("Failed to Update Purchase Order:", response.json())
Ensure you replace 123
with the actual purchase order ID you wish to update. This code updates the quantity of an item in the purchase order.
Verifying API Call Success and Handling Errors
After making API calls, verify the success by checking the response status code. A status code of 201
indicates successful creation, while 204
indicates a successful update. For error handling, check the response content for error messages and codes.
Refer to the SAP Business One Service Layer documentation for detailed error codes and troubleshooting tips.
Conclusion and Best Practices for SAP Business One API Integration
Integrating with the SAP Business One API to create or update purchase orders can significantly enhance your business processes by automating procurement tasks and reducing manual intervention. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your requests, and interact with the API using Python.
Best Practices for Secure and Efficient SAP Business One API Usage
- Securely Store Credentials: Always store your API keys and access tokens securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle retries gracefully.
- Data Validation and Transformation: Ensure that the data you send to the API is validated and transformed according to the API's requirements to prevent errors.
- Error Handling: Implement robust error handling to capture and log errors, allowing for easier troubleshooting and maintenance.
Streamlining Integrations with Endgrate
While integrating with SAP Business One API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs.
Read More
Ready to get started?