Using the Commerce7 API to Create or Update Customers in PHP
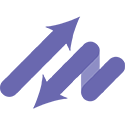
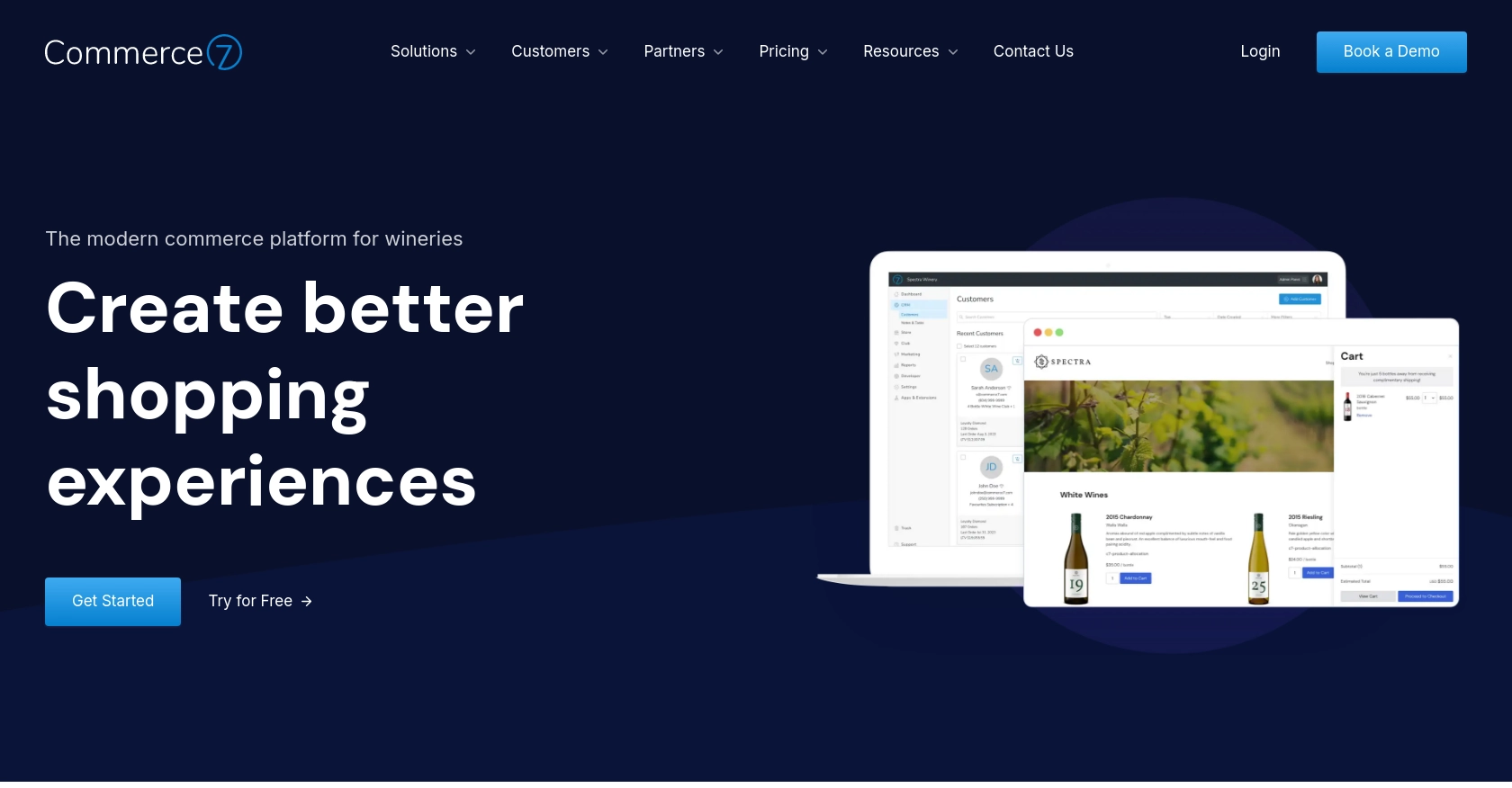
Introduction to Commerce7 API Integration
Commerce7 is a robust e-commerce platform tailored for the wine industry, offering a suite of tools to enhance customer engagement and streamline operations. Its comprehensive features include customer management, order processing, and club memberships, making it a preferred choice for wineries looking to optimize their business processes.
Integrating with the Commerce7 API allows developers to efficiently manage customer data, automate marketing efforts, and enhance customer experiences. For example, you can use the API to create or update customer records directly from your CRM system, ensuring that your customer data is always up-to-date and accessible across platforms.
Setting Up a Commerce7 Test/Sandbox Account for API Integration
Before you can start integrating with the Commerce7 API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Commerce7 provides a dedicated environment for developers to test their integrations.
Creating a Commerce7 Developer Account
To begin, you'll need to create a Commerce7 developer account. Follow these steps:
- Visit the Commerce7 Developer Center.
- Click on the Add App button to start the process of creating a new app.
- Fill in the necessary details for your app, including the app name and description.
- Once your app is created, you'll receive an App ID and an App Secret Key. Keep these credentials secure as they are essential for authenticating API requests.
Configuring API Access and Authentication
Commerce7 uses a custom authentication method for API access. Here's how to configure it:
- Log into the Commerce7 App Development Center and select your app.
- Under the app settings, choose the API endpoints you need access to. This ensures your app has the necessary permissions.
- For authentication, use Basic Auth with the following credentials:
- Username: Your App ID
- Password: Your App Secret Key
- Ensure you have the tenant ID for the client you are working with. This is the first part of the URL when logged into the Commerce7 Admin (e.g.,
https://yourtenant.admin.platform.commerce7.com
).
Generating API Keys and Access Tokens
Once your app is set up, generate the necessary API keys and access tokens:
- Navigate to the app dashboard in the App Development Center.
- Locate the section for API keys and generate a new key if needed.
- Store the API key securely, as it will be used in your API requests to authenticate and authorize access.
With your Commerce7 sandbox account and API credentials ready, you can now proceed to make API calls to create or update customer records using PHP.
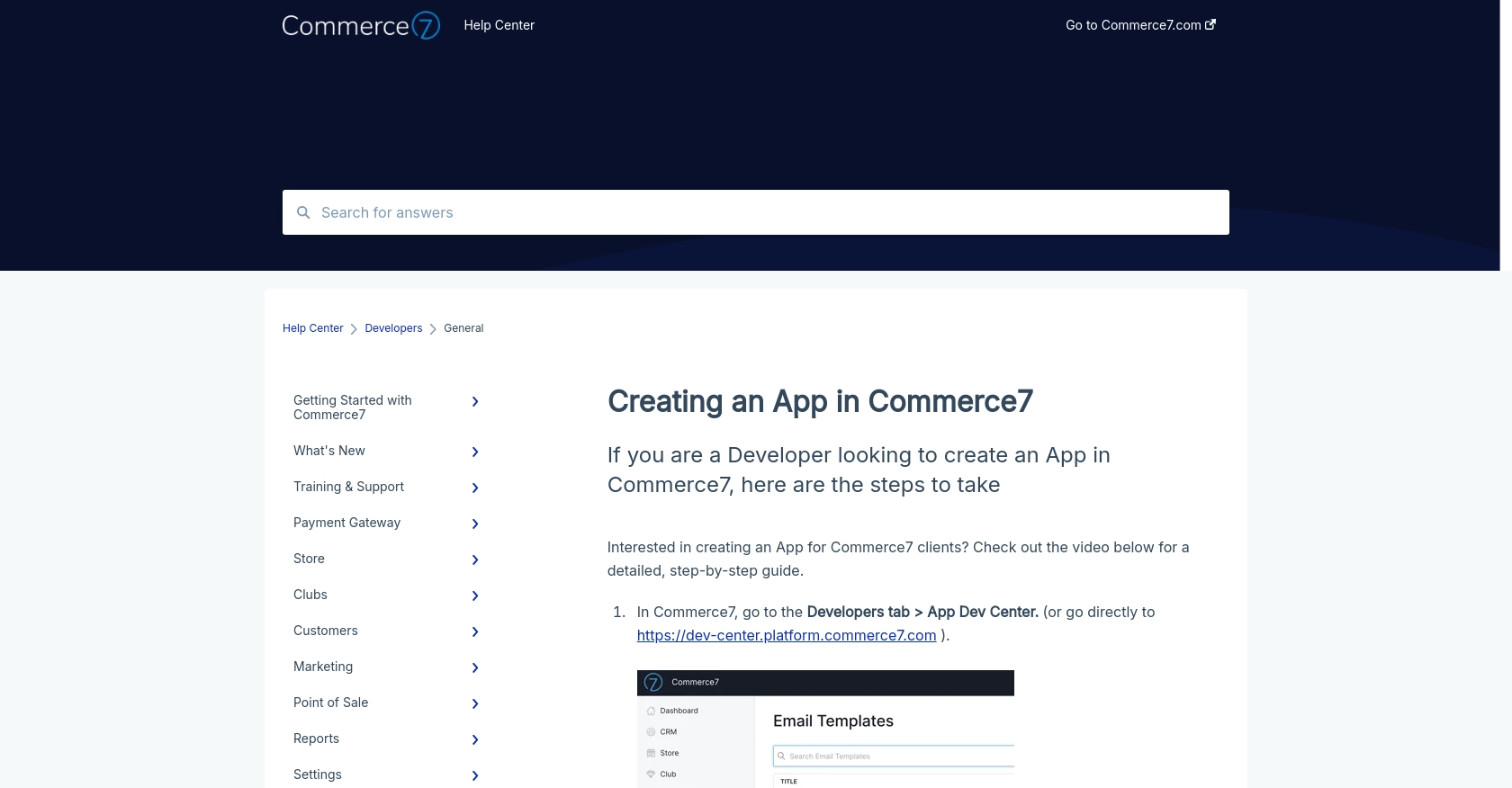
sbb-itb-96038d7
Making API Calls to Commerce7 for Customer Management Using PHP
To interact with the Commerce7 API for creating or updating customer records, you'll need to use PHP. This section will guide you through setting up your PHP environment, making API calls, and handling responses effectively.
Setting Up Your PHP Environment for Commerce7 API Integration
Before you begin, ensure your development environment is ready:
- Install PHP version 7.4 or higher.
- Ensure you have Composer installed for managing dependencies.
- Install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command:
composer require guzzlehttp/guzzle
Creating a Customer with the Commerce7 API Using PHP
To create a new customer in Commerce7, use the following PHP script:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://api.commerce7.com/v1/customer', [
'auth' => ['Your_App_ID', 'Your_App_Secret_Key'],
'json' => [
'firstName' => 'Andrew',
'lastName' => 'Kamphuis',
'birthDate' => '1973-11-15',
'city' => 'Vancouver',
'stateCode' => 'BC',
'zipCode' => 'V6A1C2',
'countryCode' => 'CA',
'emailMarketingStatus' => 'Subscribed',
'phones' => [['phone' => '+16046135343']],
'emails' => [['email' => 'andrewkamphuis@gmail.com']]
]
]);
echo $response->getBody();
Replace Your_App_ID
and Your_App_Secret_Key
with your actual credentials. This script sends a POST request to the Commerce7 API to create a customer with the specified details.
Updating a Customer in Commerce7 Using PHP
To update an existing customer, use the following PHP script:
$response = $client->put('https://api.commerce7.com/v1/customer/{customer_id}', [
'auth' => ['Your_App_ID', 'Your_App_Secret_Key'],
'json' => [
'birthDate' => '1973-11-15'
]
]);
echo $response->getBody();
Replace {customer_id}
with the actual ID of the customer you wish to update. This script updates the customer's birth date.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors:
- Check the HTTP status code to verify the request's success. A status code of 200 indicates success.
- Handle errors by catching exceptions. Guzzle throws exceptions for HTTP errors, which you can catch and manage accordingly.
try {
$response = $client->post('https://api.commerce7.com/v1/customer', [...]);
echo $response->getBody();
} catch (\GuzzleHttp\Exception\RequestException $e) {
echo $e->getMessage();
}
Verifying API Requests in Commerce7 Sandbox
To ensure your API requests are successful, verify the changes in your Commerce7 sandbox account. Check if the customer records are created or updated as expected.
By following these steps, you can efficiently manage customer data in Commerce7 using PHP, ensuring seamless integration and data consistency across platforms.
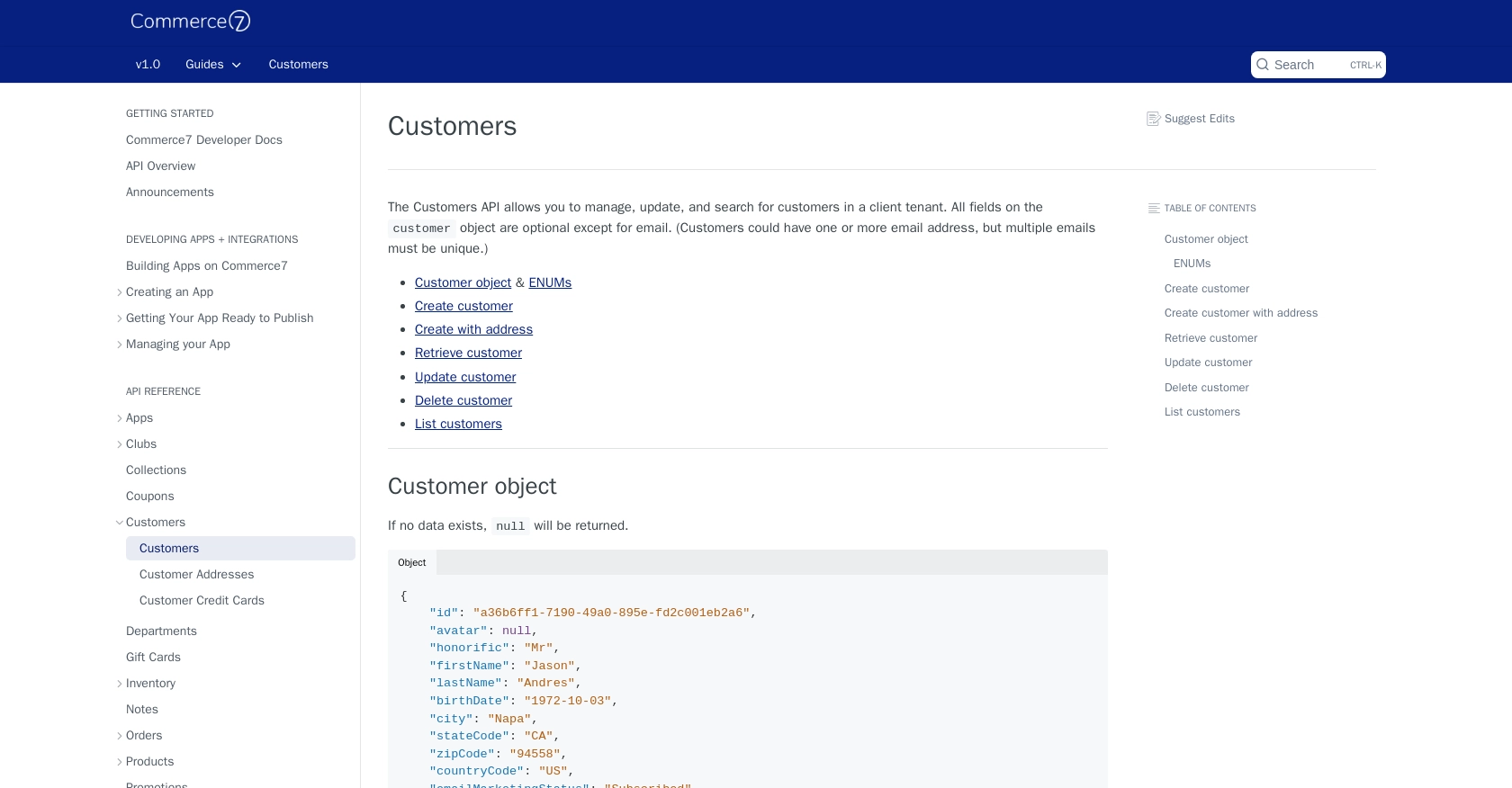
Conclusion and Best Practices for Commerce7 API Integration Using PHP
Integrating with the Commerce7 API using PHP provides a powerful way to manage customer data efficiently, ensuring seamless operations and enhanced customer experiences. By following the steps outlined in this guide, you can create and update customer records with ease, leveraging Commerce7's robust platform capabilities.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Always store your App ID and App Secret Key securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Commerce7 imposes a rate limit of 100 requests per minute per tenant. Implement logic to handle rate limits gracefully, such as retry mechanisms or request throttling. For more details, refer to the Commerce7 API documentation.
- Data Transformation and Standardization: Ensure that data formats, such as dates and currency, are consistent with Commerce7's requirements. Convert time zones to UTC and handle currency in cents as needed.
- Error Handling: Implement robust error handling to manage exceptions and HTTP errors. Use try-catch blocks to capture and respond to issues effectively.
Enhancing Integration Capabilities with Endgrate
While integrating with Commerce7 directly is effective, using a tool like Endgrate can further streamline your integration processes. Endgrate offers a unified API endpoint that connects to multiple platforms, including Commerce7, allowing you to manage integrations more efficiently.
By leveraging Endgrate, you can save time and resources, focusing on your core product development while ensuring a seamless integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
With these best practices and tools, you can optimize your use of the Commerce7 API, ensuring reliable and efficient customer data management for your business.
Read More
Ready to get started?