Using the Younium API to Create or Update Products (with Python examples)
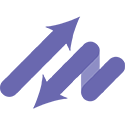
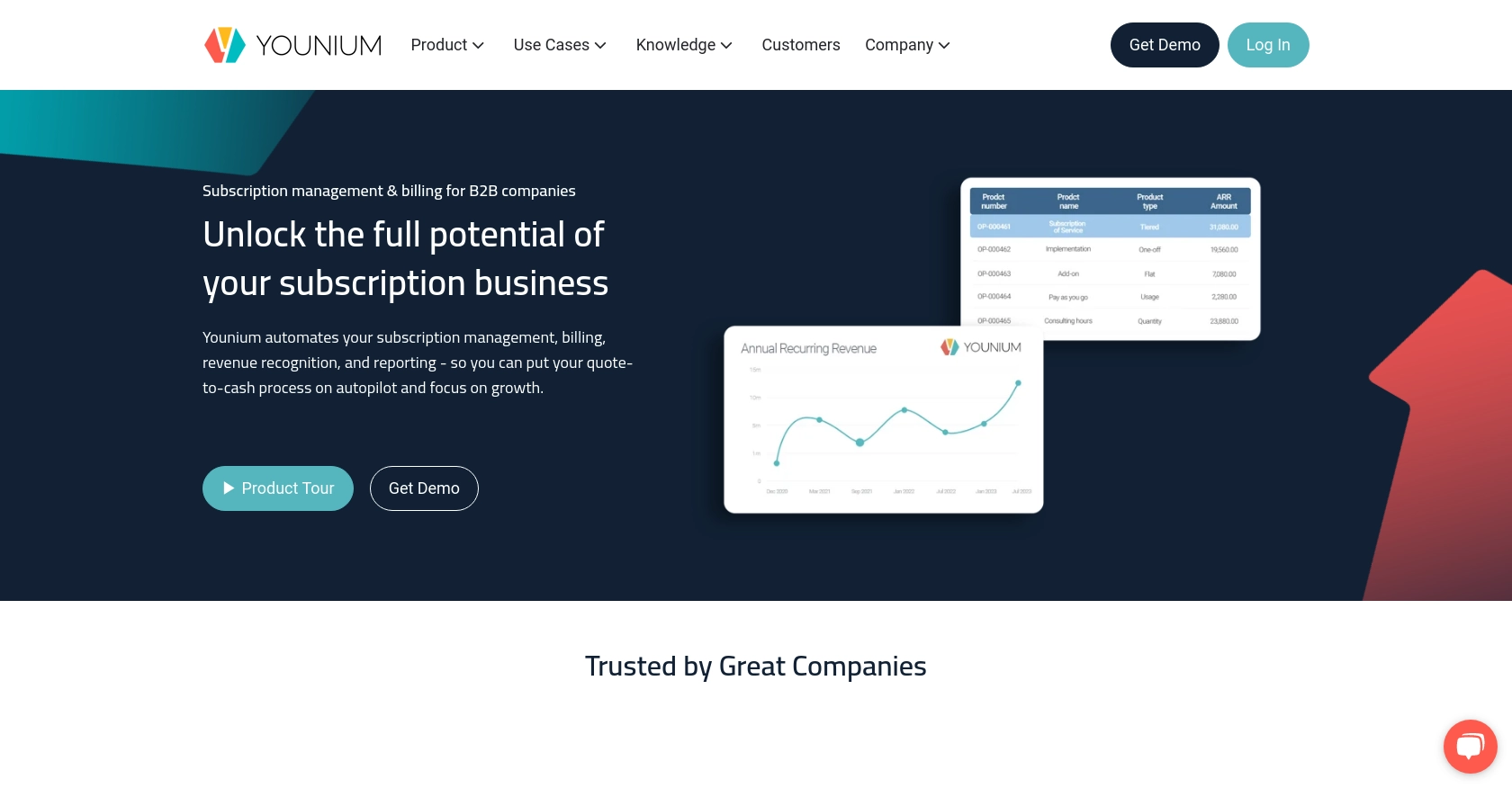
Introduction to Younium API for Product Management
Younium is a comprehensive subscription management platform designed to streamline billing, invoicing, and financial operations for B2B SaaS companies. By offering a robust API, Younium enables developers to integrate seamlessly with its platform, enhancing automation and efficiency in managing subscription-based products.
Integrating with the Younium API allows developers to create or update product information programmatically, ensuring that product catalogs remain accurate and up-to-date. For example, a developer might use the Younium API to automatically update product pricing and features based on market trends, thereby optimizing the sales process and improving customer satisfaction.
Setting Up Your Younium Sandbox Account for API Integration
Before diving into the Younium API for product management, it's essential to set up a sandbox account. This environment allows developers to test API interactions without affecting live data, ensuring a smooth integration process.
Creating a Younium Sandbox Account
To begin, you'll need to create a sandbox account on the Younium platform. Follow these steps:
- Visit the Younium Developer Portal and sign up for a developer account if you haven't already.
- Once registered, navigate to the sandbox environment section and create a new sandbox account.
- Follow the on-screen instructions to complete the setup process.
Generating API Tokens and Client Credentials
Younium uses JWT access tokens for API authentication. Here's how to generate the necessary credentials:
- Log into your Younium account and click on your name in the top right corner to access the user profile menu.
- Select “Privacy & Security” and then “Personal Tokens” from the left panel.
- Click “Generate Token” and provide a relevant description for your token.
- Click “Create” to generate your Client ID and Secret Key. Make sure to copy these values immediately, as they will not be visible again.
Acquiring a JWT Access Token
With your Client ID and Secret Key, you can now acquire a JWT access token:
import requests
# Set the API endpoint for token generation
url = "https://api.sandbox.younium.com/auth/token"
# Define the request headers and body
headers = {"Content-Type": "application/json"}
body = {
"clientId": "Your_Client_ID",
"secret": "Your_Secret_Key"
}
# Make a POST request to acquire the JWT token
response = requests.post(url, headers=headers, json=body)
# Extract the access token from the response
token_data = response.json()
access_token = token_data.get("accessToken")
print("Access Token:", access_token)
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. This script will return a JWT access token valid for 24 hours.
Handling Authentication Errors
If you encounter errors during authentication, refer to the following common issues:
- 401 Unauthorized: Indicates an expired, missing, or incorrect access token.
- 403 Forbidden: Occurs if the legal entity is incorrect or if the user lacks necessary permissions.
For more details, consult the Younium Authentication Documentation.
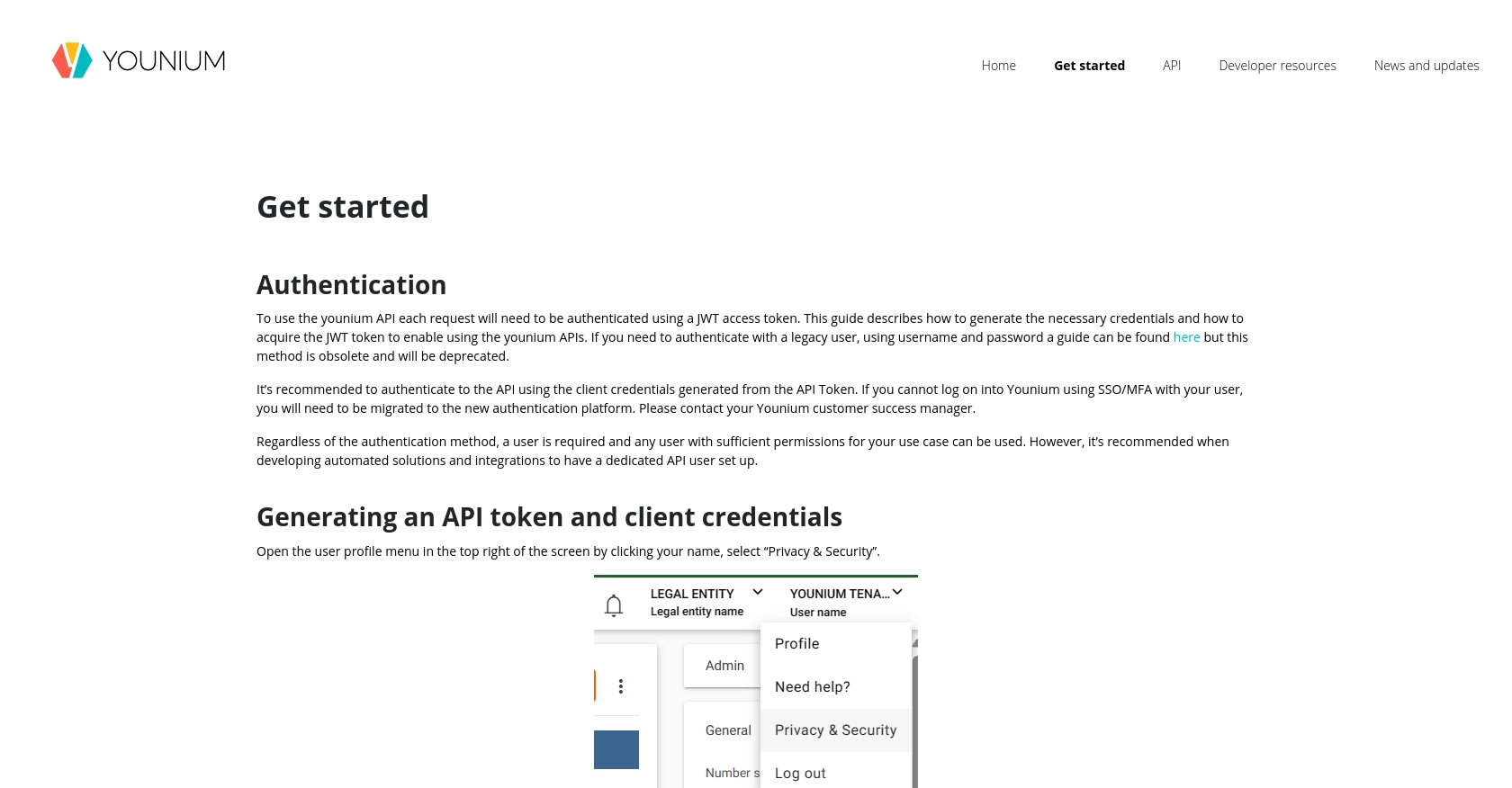
sbb-itb-96038d7
Making API Calls to Younium for Product Management with Python
To interact with the Younium API for creating or updating products, you'll need to make authenticated API calls using Python. This section will guide you through the process, including setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Younium API Integration
Before you begin, ensure you have Python 3.x installed on your machine along with the requests
library, which is essential for making HTTP requests. You can install it using pip:
pip install requests
Creating or Updating Products Using the Younium API
With your environment ready, you can now proceed to create or update products in Younium. Below is a Python example demonstrating how to perform these actions:
import requests
# Define the API endpoint for creating or updating products
url = "https://api.sandbox.younium.com/products"
# Set the request headers including the JWT access token
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1"
}
# Define the product data to be created or updated
product_data = {
"name": "New Product",
"description": "This is a new product",
"price": 100.0,
"currency": "USD"
}
# Make a POST request to create or update the product
response = requests.post(url, headers=headers, json=product_data)
# Check the response status
if response.status_code == 201:
print("Product created or updated successfully.")
else:
print("Failed to create or update product:", response.json())
Replace Your_Access_Token
with the JWT token you obtained earlier. This script sends a POST request to the Younium API to create or update a product with the specified details.
Verifying API Call Success in Younium Sandbox
After executing the API call, you can verify the success of the operation by checking the Younium sandbox environment. The newly created or updated product should appear in your product catalog.
Handling Errors and Common Issues with Younium API
When making API calls, you might encounter errors. Here are some common issues and their solutions:
- 400 Bad Request: Indicates malformed request data. Ensure all required fields are correctly formatted.
- 401 Unauthorized: Check if the access token is correct and not expired.
- 403 Forbidden: Verify the legal entity and user permissions.
For more detailed error handling, refer to the Younium API Documentation.
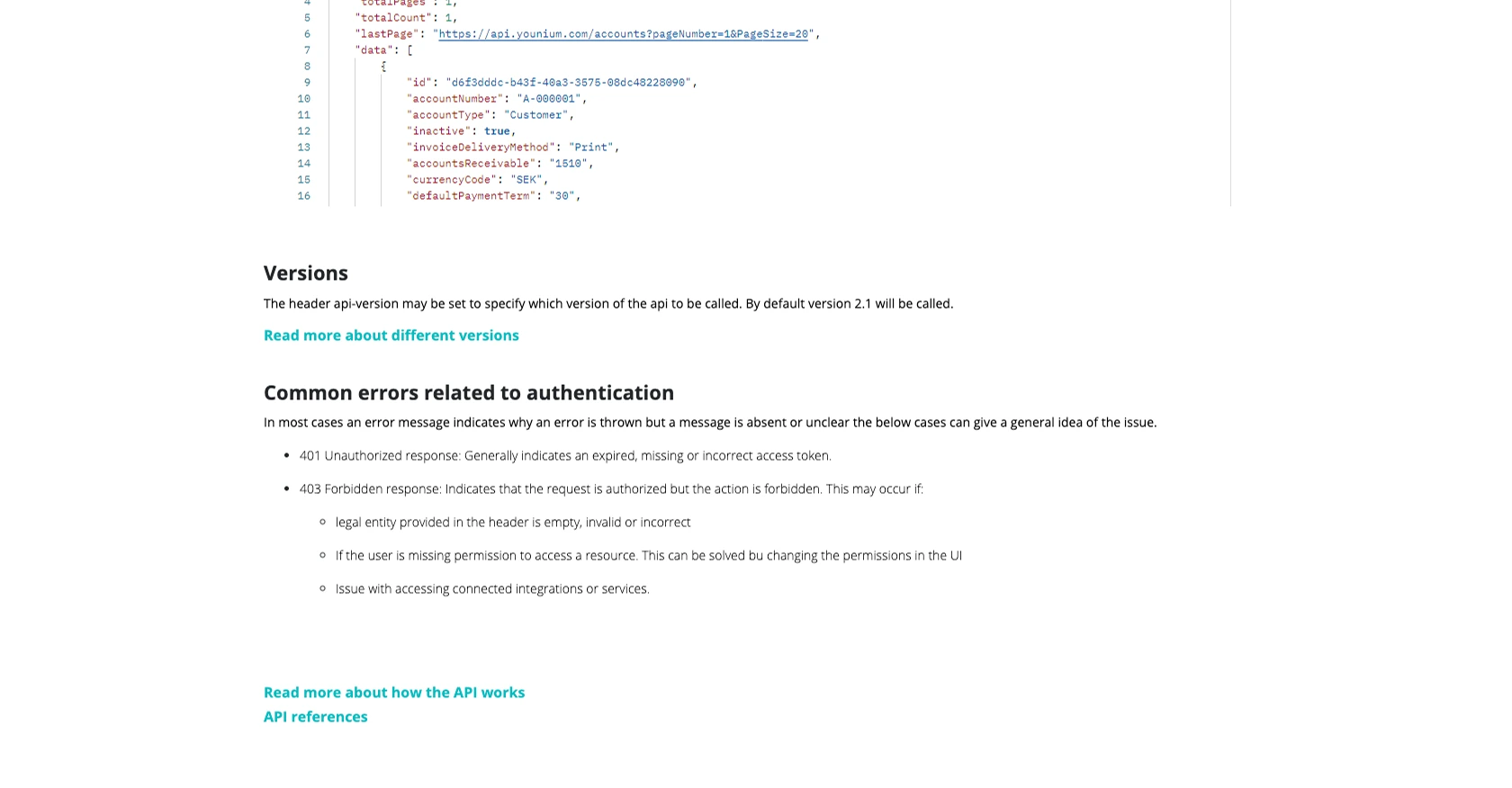
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API for product management can significantly enhance the efficiency and accuracy of managing subscription-based products. By automating the creation and updating of product information, developers can ensure that product catalogs are always current, which can lead to improved sales processes and customer satisfaction.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your Client ID, Secret Key, and JWT tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Be mindful of any rate limits imposed by the Younium API. Implement retry logic and exponential backoff strategies to handle rate limit responses gracefully.
- Data Standardization: Ensure that product data is standardized before making API calls. This includes consistent formatting of product names, descriptions, and pricing.
- Error Handling: Implement robust error handling to manage API response codes effectively. This includes logging errors and providing meaningful feedback to users or systems.
Streamlining Integrations with Endgrate
While integrating with the Younium API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration processes, allowing developers to focus on core product development rather than managing individual integrations.
By leveraging Endgrate, you can save time and resources, build once for each use case, and provide an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?