Using the Pipedrive API to Create or Update Products in Python
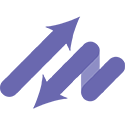
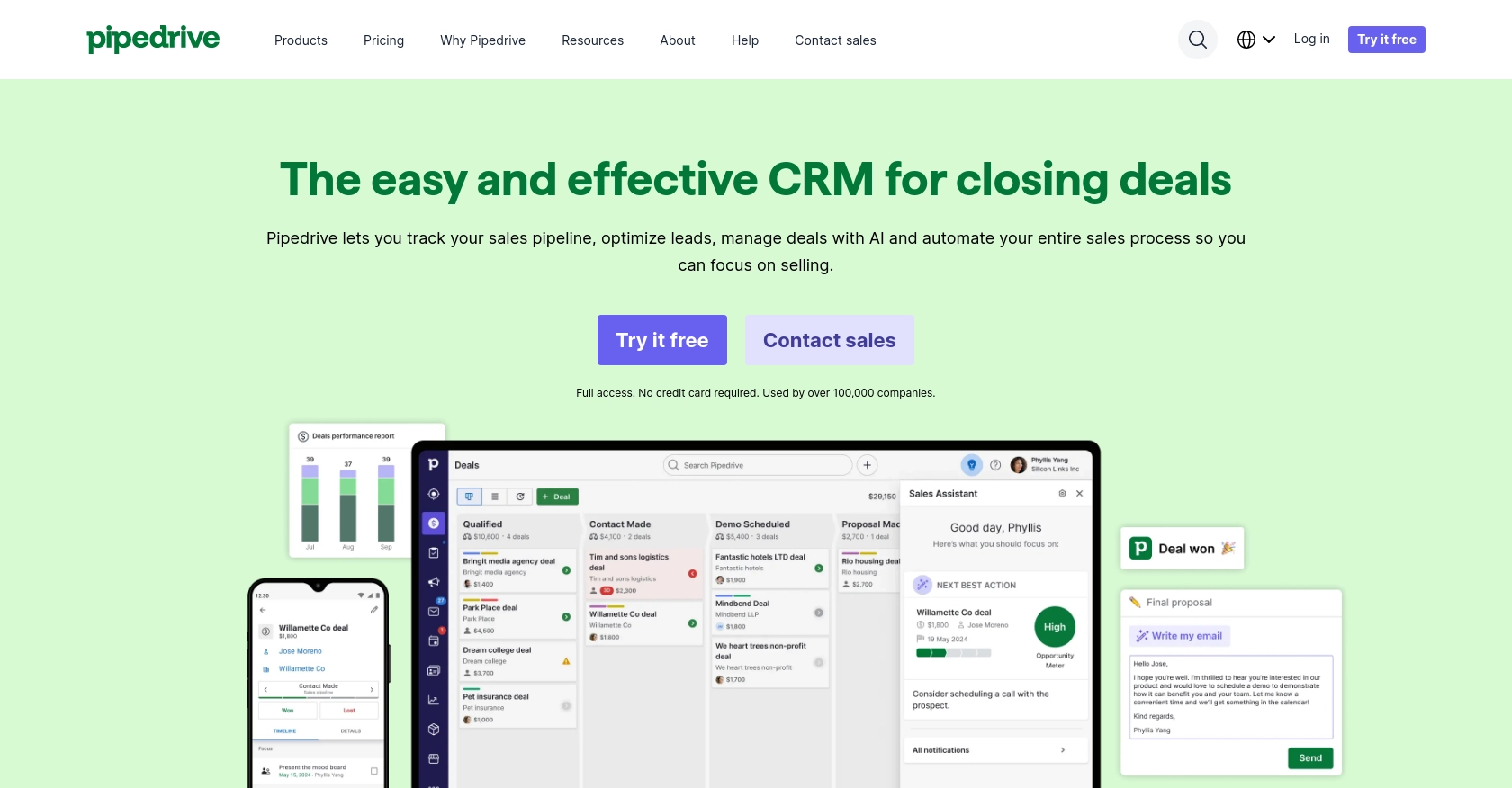
Introduction to Pipedrive API for Product Management
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage deals, and optimize their sales pipelines.
For developers, integrating with the Pipedrive API offers the opportunity to automate and enhance product management tasks. By using the API, you can create or update products within Pipedrive, streamlining inventory management and ensuring that product data is always up-to-date. This can be particularly useful for businesses that need to synchronize product information across multiple platforms.
In this article, we will explore how to use Python to interact with the Pipedrive API, focusing on creating and updating products. This integration can help developers automate product management tasks, saving time and reducing the risk of errors.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you'll need to set up a developer sandbox account. This account provides a risk-free environment to test and develop your applications without affecting live data.
Steps to Create a Pipedrive Developer Sandbox Account
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This account is similar to a regular Pipedrive company account but is limited to five seats.
- Once your request is approved, you'll receive access to the Developer Hub, where you can manage your apps and perform testing.
Creating a Pipedrive App for OAuth Authentication
Since the Pipedrive API uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials.
- Log in to your Pipedrive Developer Hub.
- Navigate to the "Apps" section and click on "Create an App."
- Fill in the required details, such as the app name and description.
- Once the app is created, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Configuring OAuth Scopes and Permissions
To interact with products in Pipedrive, you need to configure the appropriate OAuth scopes:
- Ensure that your app requests scopes related to product management, such as reading and writing product data.
- Users will need to grant these permissions during the OAuth flow, so only request the scopes necessary for your integration.
With your sandbox account and app set up, you're ready to start making API calls to create or update products in Pipedrive using Python.
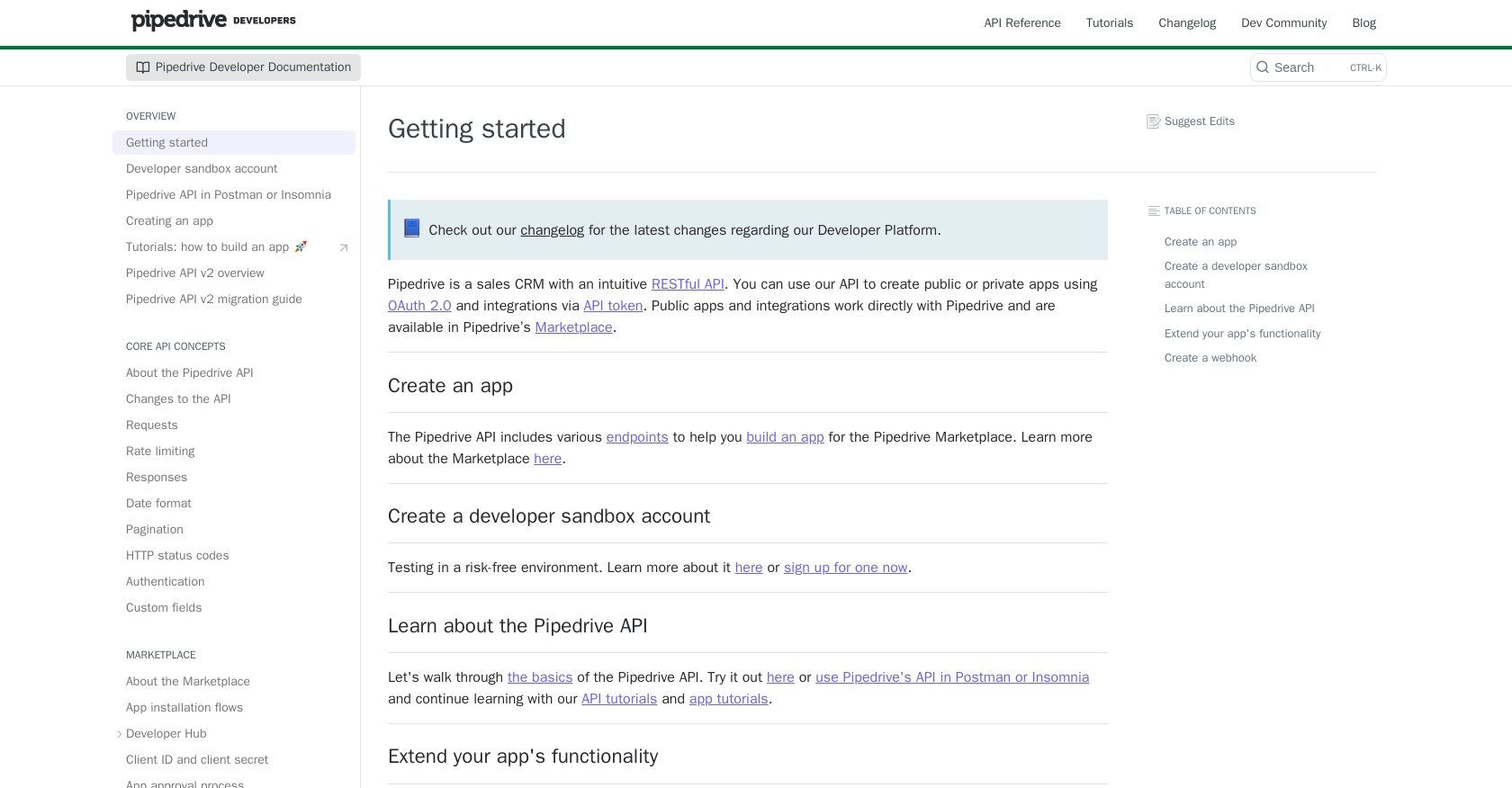
sbb-itb-96038d7
Making API Calls to Create or Update Products in Pipedrive Using Python
To interact with the Pipedrive API for creating or updating products, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for Pipedrive API Integration
Before you begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
pip install requests
Creating a Product in Pipedrive Using Python
To create a product in Pipedrive, you need to make a POST request to the Pipedrive API endpoint. Here's an example of how to do this:
import requests
# Define the API endpoint and headers
url = "https://api.pipedrive.com/v1/products"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the product data
product_data = {
"name": "New Product",
"code": "NP001",
"description": "This is a new product.",
"unit": "piece",
"tax": 20
}
# Make the POST request
response = requests.post(url, json=product_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Product created successfully:", response.json())
else:
print("Failed to create product:", response.json())
Replace Your_Access_Token
with the token obtained from your OAuth app setup. If successful, the response will include details of the newly created product.
Updating a Product in Pipedrive Using Python
To update an existing product, use the PATCH method. Here's an example:
import requests
# Define the API endpoint and headers
product_id = "12345" # Replace with your product ID
url = f"https://api.pipedrive.com/v1/products/{product_id}"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the updated product data
updated_data = {
"name": "Updated Product Name",
"description": "Updated description."
}
# Make the PATCH request
response = requests.patch(url, json=updated_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Product updated successfully:", response.json())
else:
print("Failed to update product:", response.json())
Ensure you replace product_id
with the actual ID of the product you wish to update. A successful response will confirm the update.
Handling API Responses and Errors
It's crucial to handle API responses and potential errors gracefully. The Pipedrive API uses standard HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: Authentication failed.
- 429 Too Many Requests: Rate limit exceeded.
For more detailed error handling, refer to the Pipedrive HTTP Status Codes documentation.
Verifying API Requests in the Pipedrive Sandbox
After making API calls, verify the changes in your Pipedrive sandbox account. Check the product list to ensure new products are added or existing ones are updated as expected.
By following these steps, you can efficiently manage products in Pipedrive using Python, enhancing your integration capabilities and streamlining product management tasks.
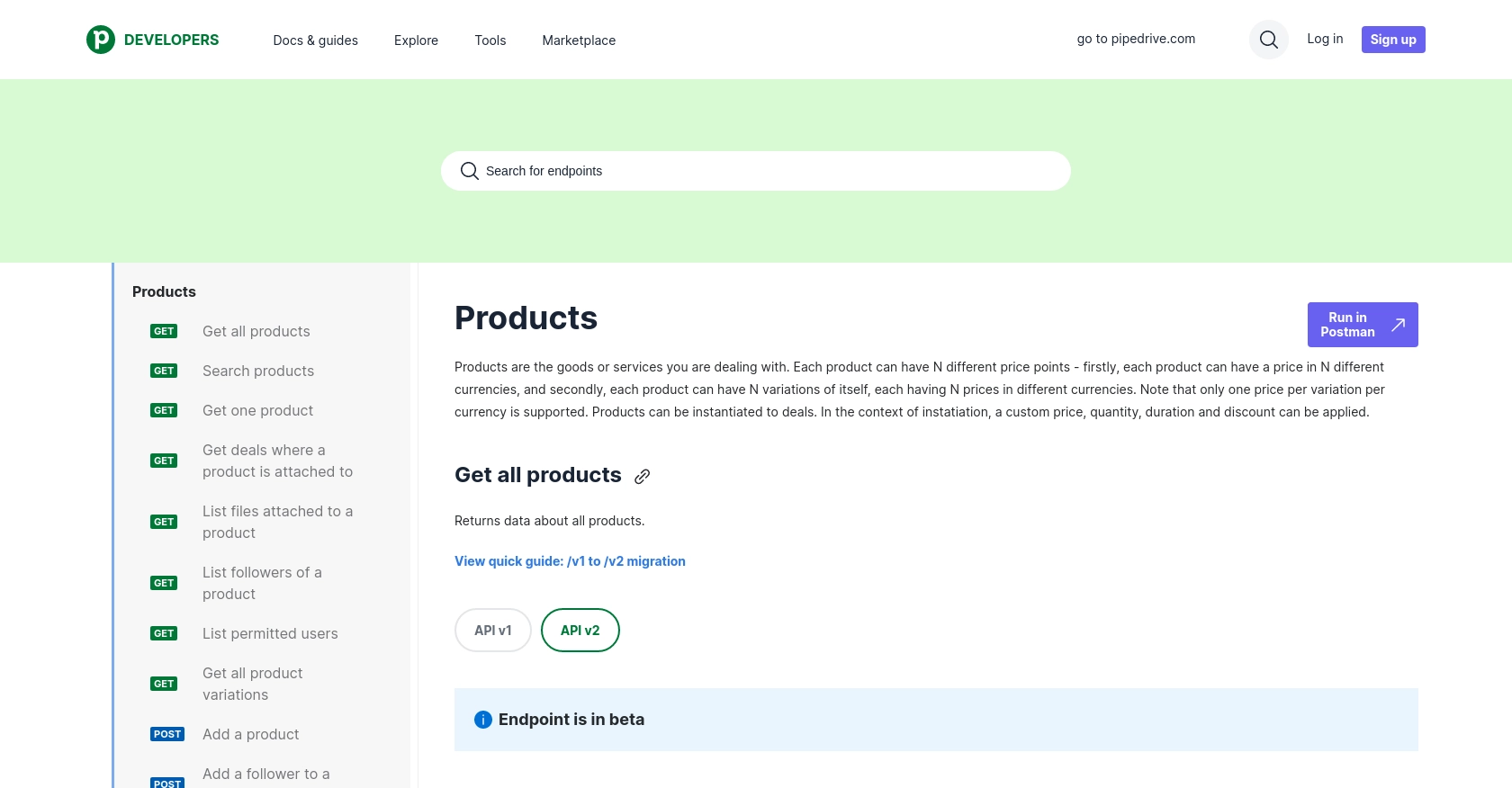
Conclusion and Best Practices for Pipedrive API Integration
Integrating with the Pipedrive API using Python allows developers to efficiently manage product data, ensuring that inventory information is always current and synchronized across platforms. By automating these tasks, businesses can save time and reduce the risk of manual errors, ultimately enhancing their sales processes.
Best Practices for Secure and Efficient Pipedrive API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handling Rate Limits: Be mindful of Pipedrive's rate limits, which allow up to 80 requests per 2 seconds per access token for the Essential plan. Implement error handling for HTTP 429 status codes and consider using webhooks to reduce unnecessary API calls. For more details, refer to the Pipedrive Rate Limiting documentation.
- Data Standardization: Ensure consistent data formats when creating or updating products. This includes standardizing fields like currency and tax rates to maintain data integrity across systems.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Pipedrive, allowing you to focus on your core product while outsourcing integration complexities. By building once for each use case, you can offer an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration efforts and enhance your product's capabilities.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Products
- https://developers.pipedrive.com/docs/api/v1/ProductFields
Ready to get started?