Using the Active Campaign API to Get Users (with Python examples)
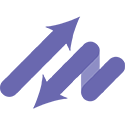
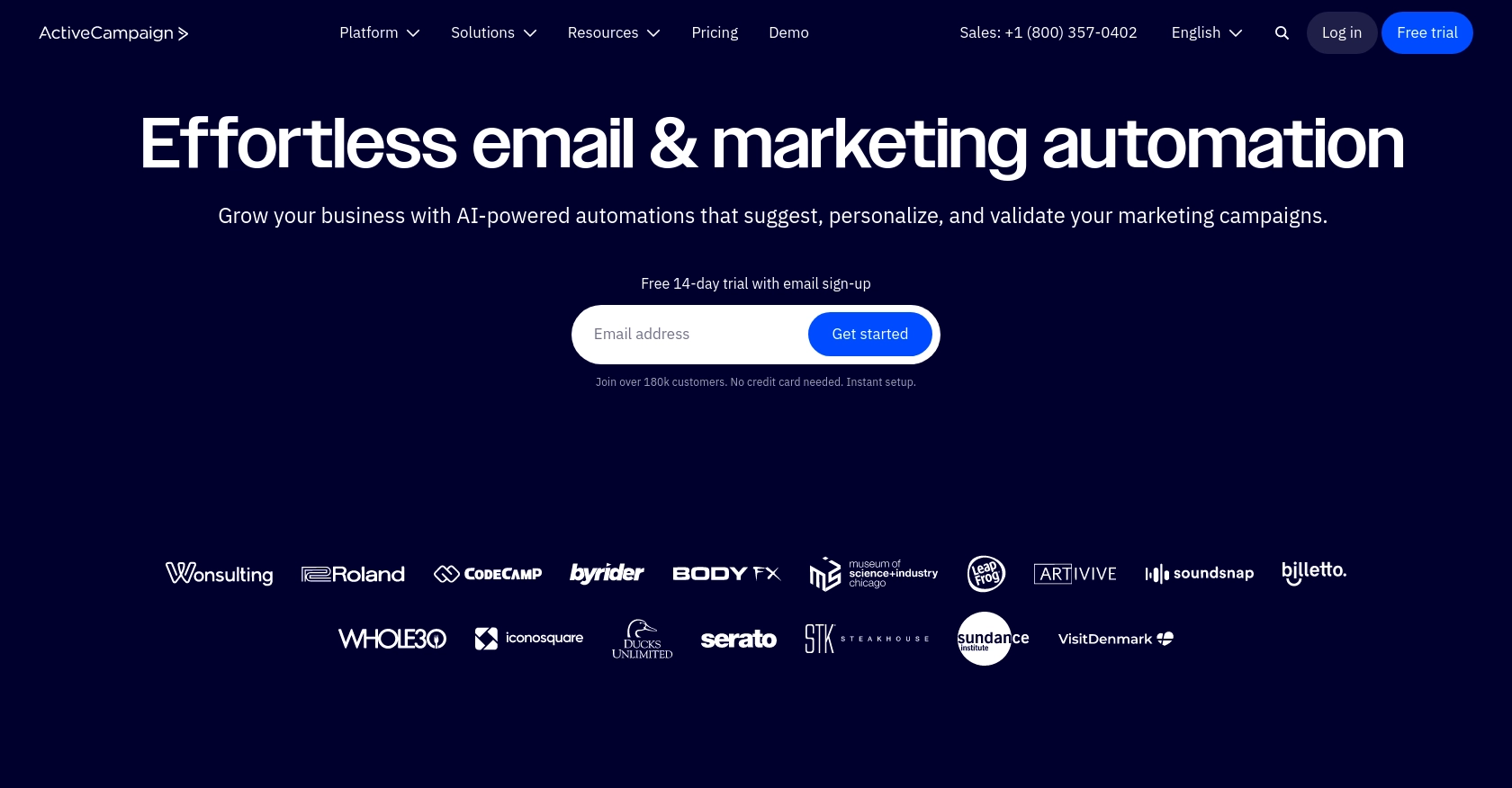
Introduction to Active Campaign API
Active Campaign is a powerful marketing automation platform that provides a suite of tools for email marketing, CRM, and sales automation. It is designed to help businesses engage with their customers more effectively by automating various marketing and sales processes.
Developers might want to integrate with Active Campaign to access user data and automate workflows. For example, using the Active Campaign API, a developer can retrieve a list of users to analyze engagement metrics or synchronize user data with other applications.
This article will guide you through using Python to interact with the Active Campaign API, specifically focusing on retrieving user information. By following this tutorial, you will learn how to efficiently access and manage user data within the Active Campaign platform.
Setting Up Your Active Campaign Test or Sandbox Account
Before you can start using the Active Campaign API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your live data. Active Campaign offers a free trial that you can use to explore its features and test integrations.
Creating an Active Campaign Account
To get started, visit the Active Campaign free trial page and sign up for an account. Follow the on-screen instructions to complete the registration process. Once your account is created, you'll have access to the Active Campaign dashboard.
Generating Your Active Campaign API Key
To interact with the Active Campaign API, you'll need an API key. Here's how to obtain it:
- Log in to your Active Campaign account.
- Navigate to the "Settings" page by clicking on your profile icon in the top right corner.
- Under the "Developer" tab, you'll find your unique API key. Make sure to keep this key secure and do not share it publicly.
For more details on authentication, refer to the Active Campaign authentication documentation.
Configuring Your API Base URL
Each Active Campaign account has a unique API base URL. To find yours:
- Go to the "Settings" page in your Active Campaign account.
- Under the "Developer" tab, locate your API URL. It will be in the format:
https://
..api-us1.com/api/3/
Ensure you use this URL for all API requests. For more information, visit the Active Campaign base URL documentation.
Testing Your API Key and Base URL
To verify that your API key and base URL are working correctly, you can make a simple API call to list all users:
import requests
# Set the API endpoint and headers
url = "https://.api-us1.com/api/3/users"
headers = {
"Api-Token": "Your_API_Key",
"accept": "application/json"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("API key and URL are working correctly.")
else:
print("There was an error with your API key or URL.")
Replace Your_API_Key
with the API key you obtained earlier. This script will help you confirm that your setup is correct.
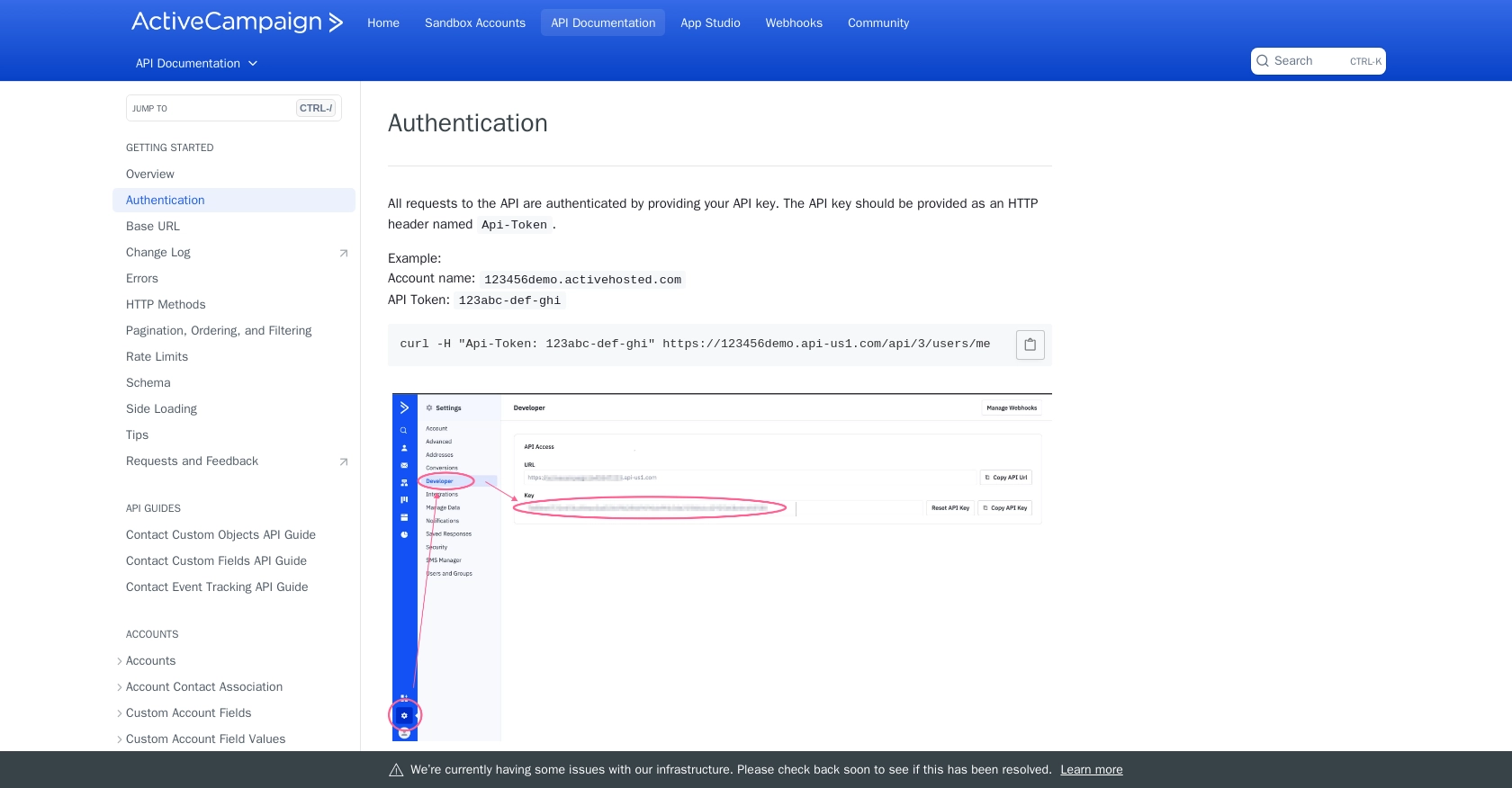
sbb-itb-96038d7
Making API Calls to Retrieve Users from Active Campaign Using Python
To interact with the Active Campaign API and retrieve user data, you'll need to make HTTP requests using Python. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Active Campaign API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Retrieve Users from Active Campaign
Create a new Python file named get_active_campaign_users.py
and add the following code:
import requests
# Set the API endpoint and headers
url = "https://.api-us1.com/api/3/users"
headers = {
"Api-Token": "Your_API_Key",
"accept": "application/json"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
users = response.json().get('users', [])
for user in users:
print(f"Username: {user['username']}, Email: {user['email']}")
else:
print(f"Error: {response.status_code} - {response.text}")
Replace Your_API_Key
with your actual API key. This script sends a GET request to the Active Campaign API to list all users and prints their usernames and emails.
Understanding the API Response and Handling Errors
When the API call is successful, the response will contain a list of users. The script extracts and prints the username and email of each user. If the request fails, the script will print the error code and message.
For more information on error codes, refer to the Active Campaign API documentation.
Verifying the API Call in Your Active Campaign Account
After running the script, you should see the list of users printed in the console. You can verify the retrieved data by comparing it with the user information in your Active Campaign account.
Handling Rate Limits and Best Practices
Active Campaign's API has a rate limit of 5 requests per second per account. To avoid hitting this limit, consider implementing a delay between requests or handling rate limit errors gracefully. For more details, see the rate limits documentation.
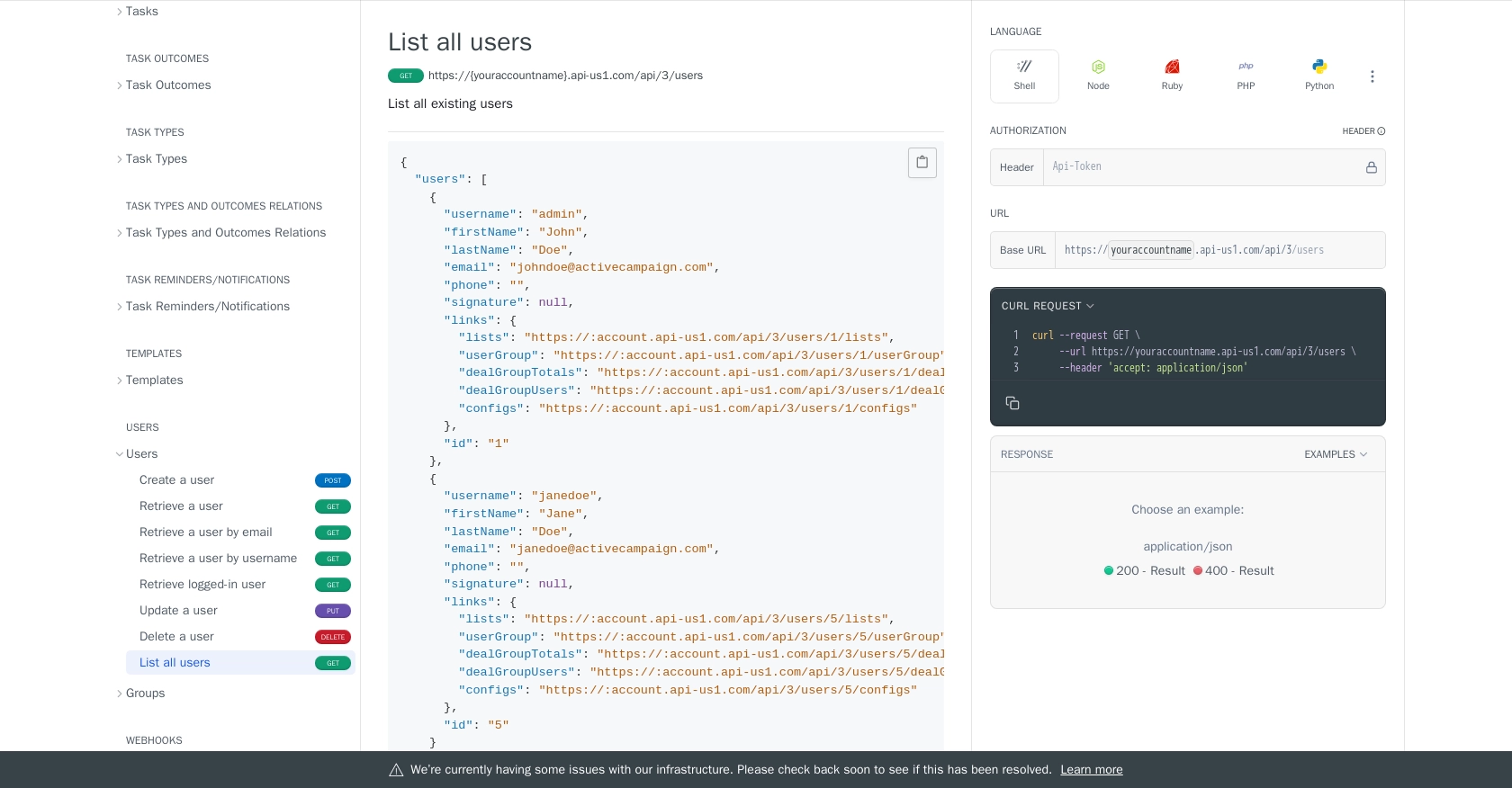
Conclusion and Best Practices for Using Active Campaign API with Python
Integrating with the Active Campaign API using Python allows developers to efficiently manage and retrieve user data, enhancing the automation of marketing and sales processes. By following the steps outlined in this guide, you can seamlessly connect to Active Campaign and leverage its powerful features.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always keep your API key confidential. Store it securely in environment variables or a secure vault, and avoid hardcoding it in your scripts.
- Handle Rate Limits Gracefully: Active Campaign enforces a rate limit of 5 requests per second per account. Implement logic to handle rate limit errors by introducing delays or retries.
- Data Standardization: Ensure that the data retrieved from Active Campaign is standardized and transformed as needed to integrate smoothly with other systems.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for troubleshooting and provide meaningful feedback to users.
Enhance Your Integration Strategy with Endgrate
While building custom integrations with Active Campaign can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing multiple integrations through a single API endpoint. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can simplify your integration processes and provide an intuitive experience for your customers. Visit Endgrate to learn more and start optimizing your integration strategy today.
Read More
- https://endgrate.com/provider/activecampaign
- https://developers.activecampaign.com/reference/authentication
- https://developers.activecampaign.com/reference/url
- https://developers.activecampaign.com/reference/pagination
- https://developers.activecampaign.com/reference/rate-limits
- https://developers.activecampaign.com/reference/list-all-users
Ready to get started?