Using the Zendesk Support API to Get Tickets (with Python examples)
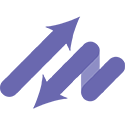
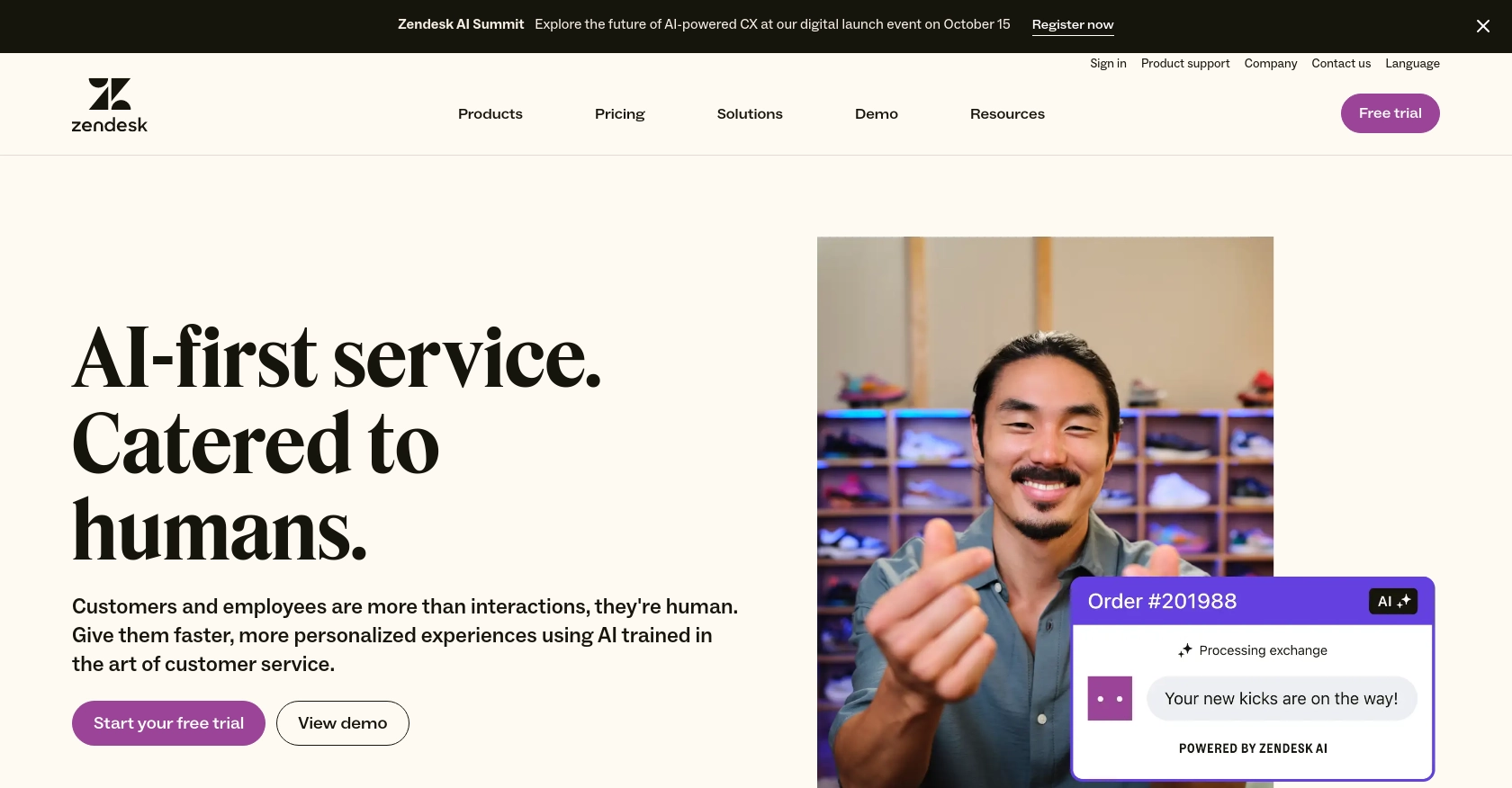
Introduction to Zendesk Support API
Zendesk Support is a powerful customer service platform that enables businesses to manage customer interactions across various channels. It offers a comprehensive suite of tools for ticketing, reporting, and customer engagement, making it a popular choice for organizations looking to enhance their customer support operations.
Developers often integrate with the Zendesk Support API to automate and streamline support processes. For example, you might want to retrieve tickets using the Zendesk Support API to analyze customer queries and improve response times. This integration can help in building custom dashboards or automating ticket management workflows.
In this article, we will explore how to use Python to interact with the Zendesk Support API, specifically focusing on retrieving tickets. This guide will provide step-by-step instructions and code examples to help you efficiently access and manage tickets within the Zendesk platform.
Setting Up Your Zendesk Support Test Account
Before diving into the Zendesk Support API, it's essential to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Zendesk offers a trial account that you can use to explore its features and functionalities.
Creating a Zendesk Support Trial Account
- Visit the Zendesk registration page and sign up for a free trial account.
- Fill out the required information, including your name, email address, and company details.
- Once registered, you will receive a confirmation email. Follow the instructions in the email to activate your account.
- Log in to your Zendesk Support account to access the dashboard.
Configuring OAuth Authentication for Zendesk Support API
Zendesk Support API uses OAuth for secure authentication. Follow these steps to set up OAuth for your application:
- Navigate to the Admin Center in your Zendesk dashboard.
- Click on Apps and integrations in the sidebar, then select APIs > Zendesk API.
- Go to the OAuth Clients tab and click Add OAuth client.
- Fill in the required fields:
- Client Name: Enter a name for your application.
- Description: Optionally, provide a short description.
- Redirect URLs: Enter the URL where Zendesk should redirect after authorization.
- Click Save to generate your OAuth credentials. Make sure to copy the Client ID and Client Secret as they will be used in your API requests.
For more detailed instructions on OAuth setup, refer to the Zendesk OAuth documentation.
Generating an Access Token
With your OAuth client set up, you can now generate an access token to authenticate your API requests:
- Direct users to the Zendesk authorization page using the following URL format:
https://{subdomain}.zendesk.com/oauth/authorizations/new?response_type=code&client_id={your_client_id}&redirect_uri={your_redirect_url}&scope=read
- After authorization, Zendesk will redirect to your specified URL with an authorization code.
- Exchange the authorization code for an access token by making a POST request to:
import requests url = "https://{subdomain}.zendesk.com/oauth/tokens" payload = { "grant_type": "authorization_code", "code": "{authorization_code}", "client_id": "{your_client_id}", "client_secret": "{your_client_secret}", "redirect_uri": "{your_redirect_url}" } response = requests.post(url, json=payload) access_token = response.json().get("access_token") print(access_token)
Use the access token in your API requests by including it in the Authorization header as follows:
headers = {
"Authorization": "Bearer {access_token}"
}
For more information on OAuth flows, visit the Zendesk Developer Docs.
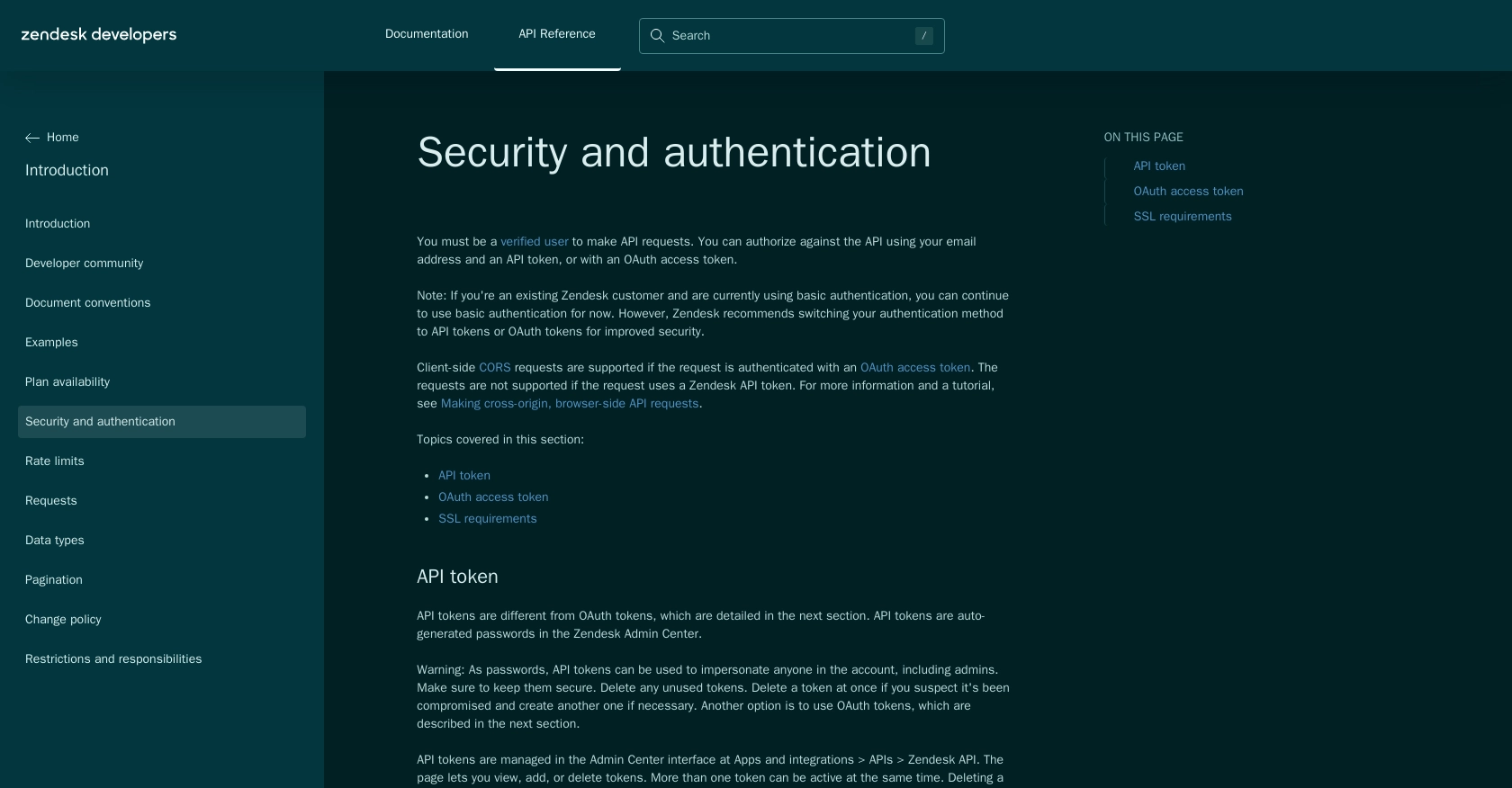
sbb-itb-96038d7
Making API Calls to Retrieve Zendesk Support Tickets Using Python
Understanding Python and Required Dependencies for Zendesk Support API
To interact with the Zendesk Support API using Python, ensure you have Python 3.11.1 or later installed on your machine. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Setting Up Your Python Environment for Zendesk Support API Calls
Begin by creating a new Python file, get_zendesk_tickets.py
, to manage your API interactions. This file will contain the code necessary to authenticate and retrieve tickets from Zendesk Support.
Writing the Python Code to Retrieve Tickets from Zendesk Support
Use the following code to make a GET request to the Zendesk Support API and retrieve tickets:
import requests
# Define the API endpoint and headers
url = "https://{subdomain}.zendesk.com/api/v2/tickets.json"
headers = {
"Authorization": "Bearer {access_token}",
"Content-Type": "application/json"
}
# Make the GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
tickets = response.json().get("tickets", [])
for ticket in tickets:
print(f"Ticket ID: {ticket['id']}, Subject: {ticket['subject']}")
else:
print(f"Failed to retrieve tickets: {response.status_code} - {response.text}")
Replace {subdomain}
with your Zendesk subdomain and {access_token}
with the access token obtained during the OAuth setup.
Verifying Successful API Requests in Zendesk Support
After running the script, you should see a list of ticket IDs and their subjects printed in the console. To verify the request's success, log in to your Zendesk Support dashboard and ensure the tickets match the output.
Handling Errors and Understanding Zendesk Support API Error Codes
If the request fails, the script will print an error message with the status code and response text. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it hasn't expired.
- 404 Not Found: Verify the API endpoint URL and subdomain.
- 429 Too Many Requests: You've exceeded the rate limit. Refer to the Zendesk rate limits documentation for guidance.
For more detailed error handling, consult the Zendesk API documentation.
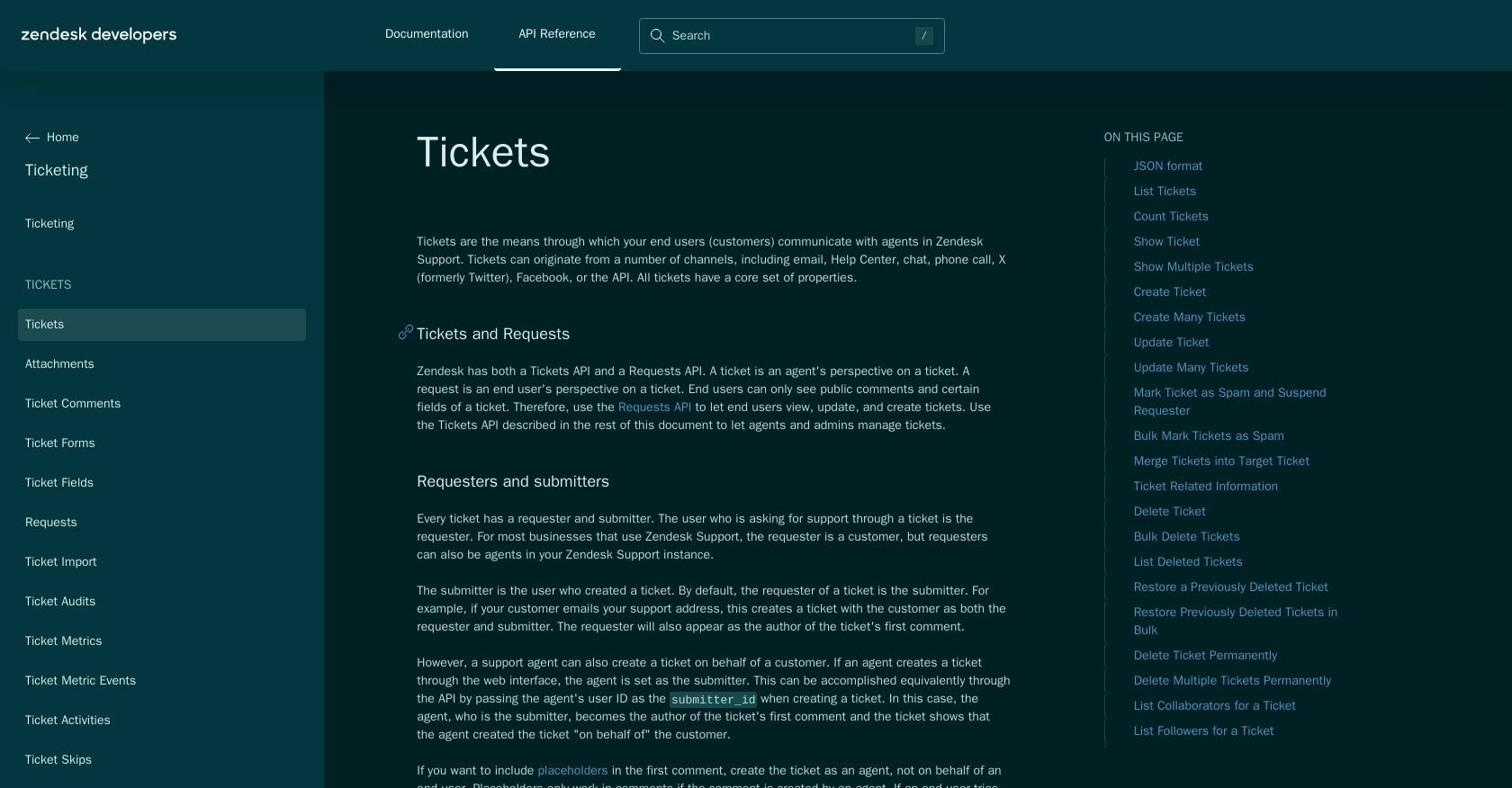
Best Practices for Using Zendesk Support API with Python
When working with the Zendesk Support API, it's crucial to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials and access tokens securely. Avoid hardcoding them in your scripts. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of Zendesk's rate limits. If you receive a 429 error, implement a retry mechanism that respects the
Retry-After
header. For more details, refer to the Zendesk rate limits documentation. - Data Transformation and Standardization: When retrieving data, ensure you transform and standardize it to fit your application's needs. This might involve converting date formats or normalizing field names.
- Error Handling: Implement robust error handling to manage different response codes and scenarios. This will help in maintaining a smooth user experience and debugging issues effectively.
Enhancing Integration Efficiency with Endgrate
Integrating multiple platforms can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Zendesk Support. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
To learn more about how Endgrate can streamline your integration processes, visit Endgrate.
Read More
- https://endgrate.com/provider/zendesksupport
- https://developer.zendesk.com/api-reference/introduction/security-and-auth/
- https://support.zendesk.com/hc/en-us/articles/4408845965210-Using-OAuth-authentication-with-your-application
- https://developer.zendesk.com/api-reference/introduction/rate-limits/
- https://developer.zendesk.com/api-reference/introduction/pagination/
- https://developer.zendesk.com/api-reference/ticketing/tickets/tickets/
Ready to get started?