How to Get Records with the Postgres API in Python
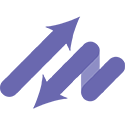
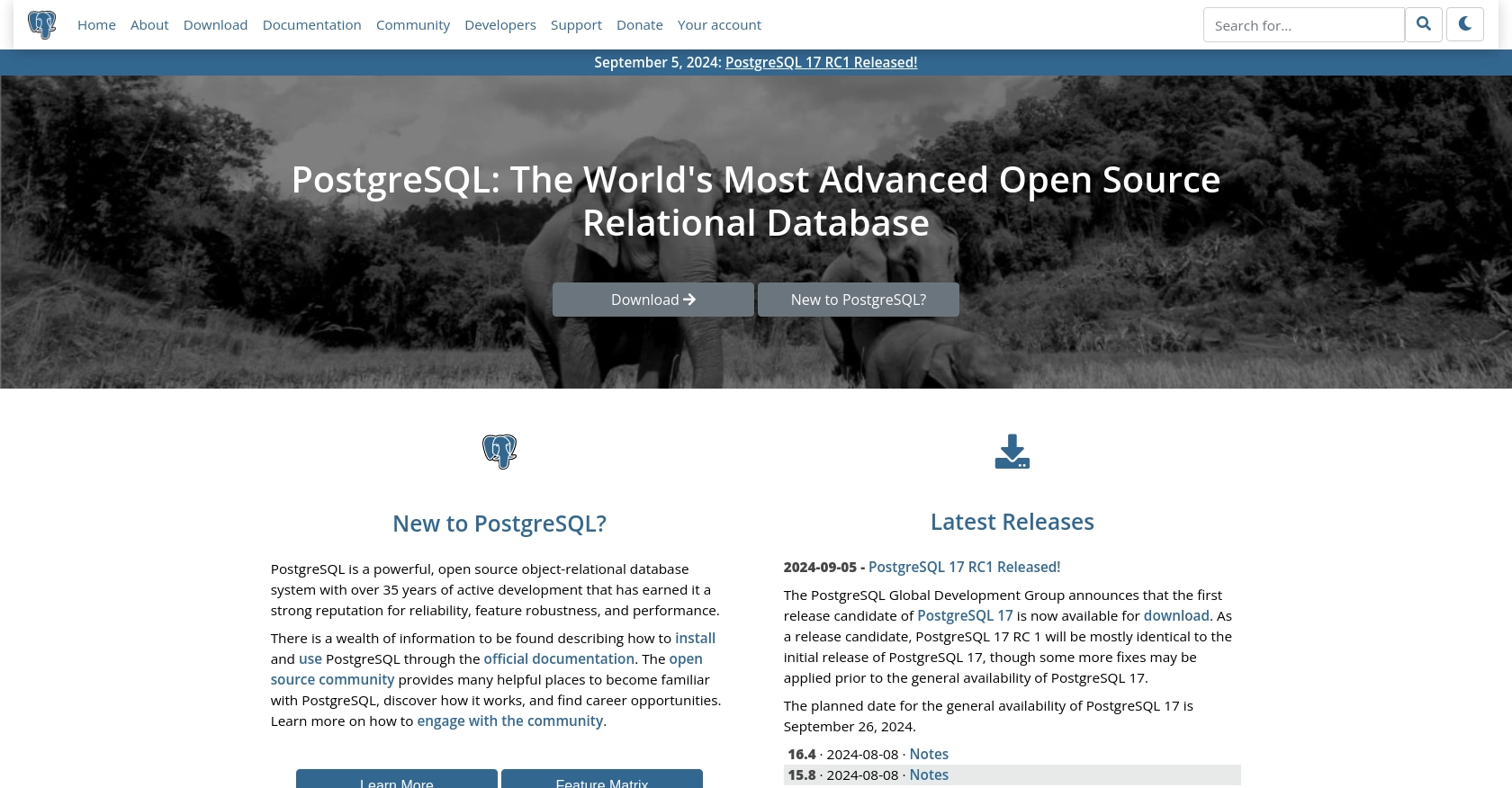
Introduction to PostgreSQL API Integration
PostgreSQL, often referred to as Postgres, is a powerful, open-source relational database management system known for its robustness, scalability, and compliance with SQL standards. It is widely used by developers and businesses to manage and store data efficiently.
Integrating with the PostgreSQL API allows developers to interact programmatically with their databases, enabling automation and seamless data retrieval. For example, a developer might want to use the PostgreSQL API to fetch records for data analysis or to integrate with other applications, enhancing the overall functionality of their software solutions.
This article will guide you through the process of using Python to interact with the PostgreSQL API, focusing on retrieving records efficiently and effectively.
Setting Up Your PostgreSQL Test Environment
Before you can start interacting with the PostgreSQL API using Python, you need to set up a test environment. This involves creating a PostgreSQL database and configuring it for API access. This setup will allow you to safely test your API calls without affecting your production data.
Creating a PostgreSQL Database
To begin, you'll need to have PostgreSQL installed on your local machine or server. You can download the latest version from the official PostgreSQL website. Follow the installation instructions specific to your operating system.
Once installed, you can create a new database using the following command in your terminal:
createdb test_database
This command creates a new database named test_database
which you will use for testing purposes.
Configuring User Access
Next, you need to set up a user account that can access the database. You can create a new user with the following command:
CREATE USER test_user WITH PASSWORD 'securepassword';
Make sure to replace 'securepassword'
with a strong password of your choice. Grant the necessary privileges to this user:
GRANT ALL PRIVILEGES ON DATABASE test_database TO test_user;
Connecting to the Database
With your database and user set up, you can now connect to the database using Python. You'll need the psycopg2
library, which is a popular PostgreSQL adapter for Python. Install it using pip:
pip install psycopg2-binary
Here's a sample code snippet to connect to your PostgreSQL database:
import psycopg2
try:
connection = psycopg2.connect(
dbname="test_database",
user="test_user",
password="securepassword",
host="localhost"
)
print("Connection to PostgreSQL established successfully.")
except Exception as e:
print(f"An error occurred: {e}")
finally:
if connection:
connection.close()
Replace the connection parameters with your actual database details. This code will establish a connection to your PostgreSQL database, allowing you to execute API calls and retrieve records.
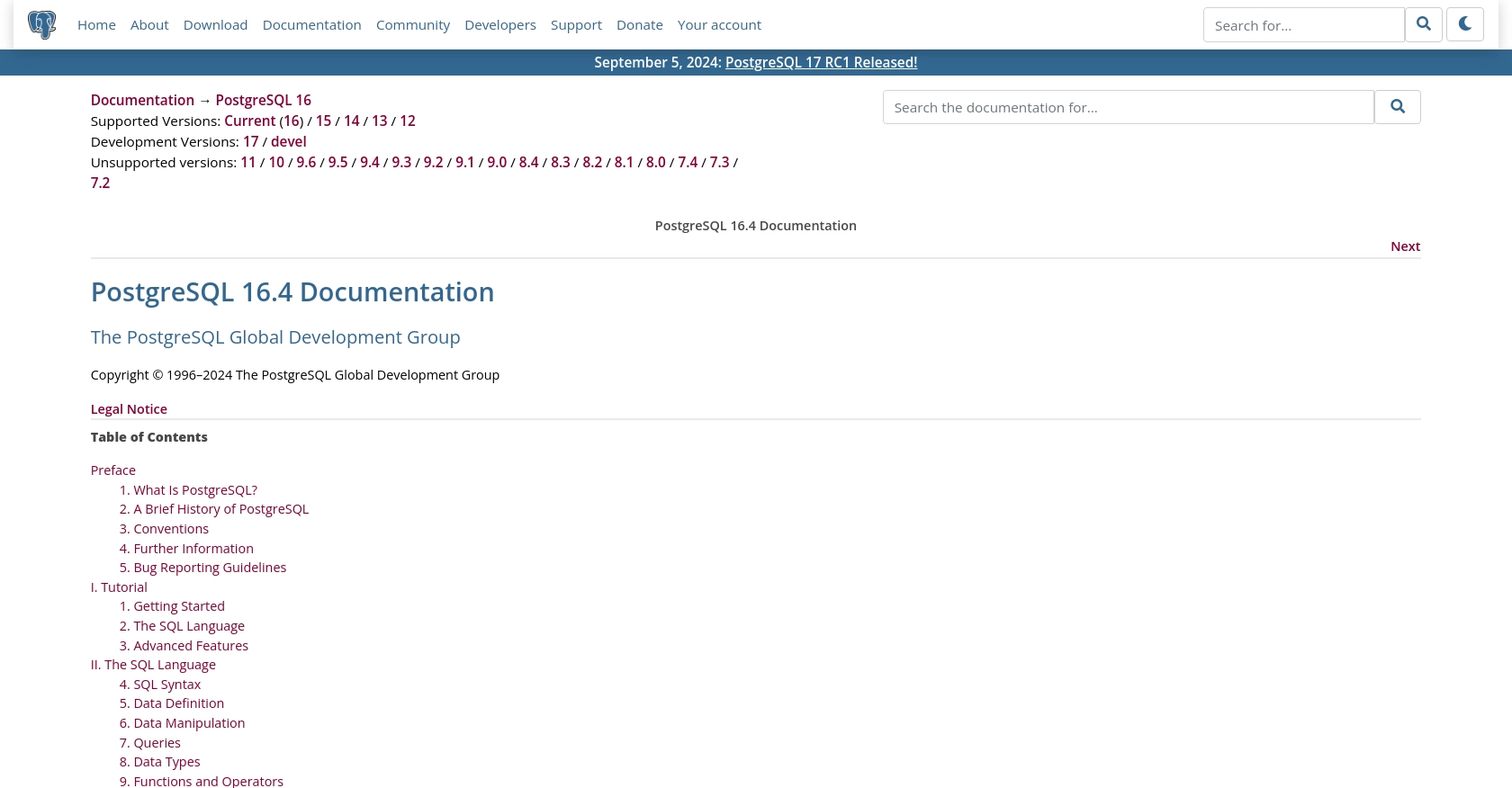
sbb-itb-96038d7
Making API Calls to Retrieve Records from PostgreSQL Using Python
To effectively interact with your PostgreSQL database and retrieve records, you need to understand how to make API calls using Python. This section will guide you through the process of setting up your Python environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for PostgreSQL API Calls
Before making API calls, ensure you have Python 3.x installed on your machine. Additionally, you'll need the psycopg2
library, which facilitates communication between Python and PostgreSQL. Install it using the following command:
pip install psycopg2-binary
Writing Python Code to Fetch Records from PostgreSQL
Once your environment is set up, you can write a Python script to connect to your PostgreSQL database and retrieve records. Below is a sample code snippet demonstrating how to achieve this:
import psycopg2
def fetch_records():
try:
# Establish a connection to the PostgreSQL database
connection = psycopg2.connect(
dbname="test_database",
user="test_user",
password="securepassword",
host="localhost"
)
cursor = connection.cursor()
# Execute a query to fetch records
query = "SELECT * FROM your_table_name;"
cursor.execute(query)
# Fetch all records from the executed query
records = cursor.fetchall()
# Print the retrieved records
for record in records:
print(record)
except Exception as e:
print(f"An error occurred: {e}")
finally:
if connection:
cursor.close()
connection.close()
# Call the function to fetch records
fetch_records()
Replace your_table_name
with the actual name of the table you want to query. This script connects to the PostgreSQL database, executes a SQL query to fetch all records from the specified table, and prints the results.
Verifying Successful API Calls and Handling Errors
After running your script, verify that the records are correctly retrieved by checking the output in your terminal. If the records match those in your database, the API call was successful.
In case of errors, the script will print an error message. Common issues include incorrect database credentials or network connectivity problems. Ensure your connection parameters are accurate and that your PostgreSQL server is running.
Best Practices for Error Handling and Optimization
- Use try-except blocks to catch and handle exceptions gracefully.
- Close database connections and cursors in the
finally
block to ensure resources are released. - Optimize queries for performance, especially when dealing with large datasets.
By following these steps, you can efficiently retrieve records from your PostgreSQL database using Python, enabling seamless integration and data management in your applications.
Conclusion: Best Practices for PostgreSQL API Integration with Python
Integrating with the PostgreSQL API using Python can significantly enhance your application's data management capabilities. By following the steps outlined in this article, you can efficiently retrieve records and ensure seamless interaction with your PostgreSQL database.
Storing User Credentials Securely
- Always store database credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Avoid hardcoding sensitive information directly in your codebase.
Handling PostgreSQL API Rate Limits and Errors
- Implement error handling to manage exceptions gracefully and provide meaningful feedback to users.
- Be aware of any rate limits imposed by your database setup and plan your API calls accordingly to avoid disruptions.
Optimizing Data Retrieval and Transformation
- Optimize SQL queries to improve performance, especially when dealing with large datasets.
- Consider transforming and standardizing data fields to ensure consistency across your application.
Streamlining Integrations with Endgrate
While integrating with PostgreSQL directly is powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. By leveraging Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?